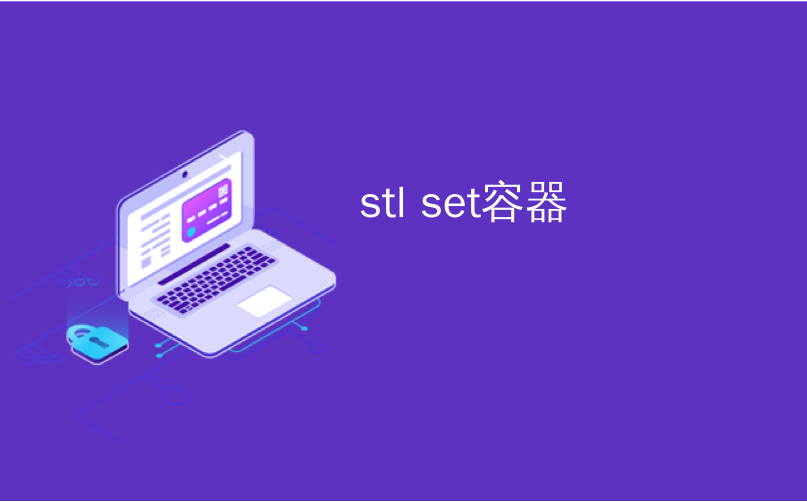
stl set容器
In this tutorial you will learn about STL Set container in C++ i.e. std::set and all functions applicable on it.
在本教程中,您将学习C ++中的STL Set容器,即std :: set及其适用的所有功能。
Set is a associative container. We know that in associative containers each element is unique. So sets are also containers that stores unique elements following in a specific order. The word associative means each value associated with a key value. For any kind of operation key will be more preferred than actual value. Here sets are special type of associative containers where value itself is key value.
Set是一个关联容器。 我们知道,在关联容器中,每个元素都是唯一的。 因此,集合也是按特定顺序存储唯一元素的容器。 单词关联是指与键值关联的每个值。 对于任何类型的操作,键将比实际值更可取。 这里的集合是特殊类型的关联容器,其中值本身是键值。
Some more facts about set are, elements in set are constant. It means that we are unable to modify once we insert the element. If we want to update element then we should delete that element and again insert with updated element. The elements in the set are always sorted.
关于集合的更多事实是,集合中的元素是恒定的。 这意味着一旦插入元素,我们将无法修改。 如果要更新元素,则应删除该元素,然后再次插入更新的元素。 集合中的元素总是被排序。
C ++ STL集 (C++ STL Set)
Let see some functions associated with sets:
让我们看一些与集合相关的函数:
Before working with functions let see iterators that can apply on list to manipulate the data in list.
在使用函数之前,让我们看看可以应用于列表的迭代器,以操作列表中的数据。
begin(): returns iterator to the beginning
begin():将迭代器返回到开头
end(): returns iterator to the end of the list
end():将迭代器返回到列表的末尾
rbegin(): returns reverse iterator to reverse beginning
rbegin():返回反向迭代器以反向开始
rend(): returns reverse iterator to reverse end.
rend():将反向迭代器返回到反向端点。
These iterators we can use in our programs.
我们可以在程序中使用这些迭代器。
First thing we need to include is set header file. Which is #include<set>
我们需要包括的第一件事是设置头文件。 这是#include <set>
Inserting element into set:
将元素插入集合:
There are different ways we can insert elements into set.
我们可以通过多种方式将元素插入集合。
Note: In any method below when we insert an element into set it automatically inserted at proper position based on ascending sorted order.
注意:在下面的任何方法中,当我们将元素插入到集合中时,它会根据升序排序自动插入到适当的位置。
Method 1: Insert directly by passing element. setName.insert(element);
方法1:通过传递元素直接插入。 setName.insert(element);
Method 2: Using iterator. This returns iterator at inserted position. setName.insert (iterator,value)
方法2:使用迭代器。 这将在插入位置返回迭代器。 setName.insert(迭代器,值)
Method 3: Copying from another container.
方法3:从另一个容器复制。
Example program for inserting into set:
插入集合的示例程序:
#include<iostream>
#include<set>
using namespace std;
int main(){
set<int> s1; // declaring a set
set<int> :: iterator it; // iterator for set
for(int i=0;i<5;i++){
s1.insert(i*10); // inserting using Method1
}
it= s1.begin();
s1.insert(it,99); // inserting using Method2
int ary[]= { 23, 34, 45, 56};
s1.insert(ary, ary+4); // inserting using Method3
//checking by printing
for(it= s1.begin(); it!=s1.end(); it++)
cout << *it << " ";
// We can observe that output will be print in sorted order. That is the property of set
return 0;
}
Output
输出量
0 10 20 23 30 34 40 45 56 99
0 10 20 23 30 34 40 45 56 99
Some more functions applicable on set are:
一些适用于集合的功能是:
erase(): We can erase an element by specifying value or pointing to iterator.
delete():我们可以通过指定值或指向迭代器来擦除元素。
swap(): swaps elements of set1 to set2 and set2 to set1.
swap():将set1的元素交换为set2,将set2的元素交换为set1。
clear(): removes all elements in the list. It results list of size 0.
clear():删除列表中的所有元素。 结果列表大小为0。
Example program to show usage of above functions:
显示上述功能用法的示例程序:
#include<iostream>
#include<set>
using namespace std;
int main(){
set<int> s1;
set<int> :: iterator it;
for(int i=0; i<5; i++)
s1.insert(i+10);
s1.erase(12); // deleting element 12
cout << "deleting element 12 --> ";
for(it= s1.begin(); it!=s1.end(); it++)
cout << *it << " ";
cout << endl;
set<int> s2;
for(int i=0;i<4;i++)
s2.insert(i);
cout << "set1 elements before swapping --> ";
for(it= s1.begin(); it!= s1.end(); it++)
cout<< *it << " ";
cout << endl;
cout << "set2 elements before swapping --> ";
for(it= s2.begin(); it!= s2.end(); it++)
cout<< *it << " ";
cout << endl;
s1.swap(s2); // swapping operation
cout << "set1 elements after swapping --> ";
for(it= s1.begin(); it!= s1.end(); it++)
cout<< *it << " ";
cout << endl;
cout << "set2 elements after swapping --> ";
for(it= s2.begin(); it!= s2.end(); it++)
cout<< *it << " ";
cout << endl;
s1.clear(); // clearing list1
s1.empty() ? cout <<"list is empty" << endl: cout << "list is not empty" << endl;
// ternary operation which resutls list is empty or not
return 0;
}
Output
输出量
deleting element 12 –> 10 11 13 14 set1 elements before swapping –> 10 11 13 14 set2 elements before swapping –> 0 1 2 3 set1 elements after swapping –> 0 1 2 3 set2 elements after swapping –> 10 11 13 14 list is empty
删除元素12 –> 交换前的1011 13 14 set1元素–>交换前的10 11 13 14 set2元素–>交换后的0 1 2 3 set1元素–>交换后的0 1 2 3 set2元素–> 10 11 13 14 list是空的
Some more functions are:
其他一些功能是:
empty(): returns a Boolean value whether set is empty or not.
empty():返回布尔值,无论set是否为空。
size(): returns the size of the list.
size():返回列表的大小。
max_size(): returns the maximum size a set can have.
max_size():返回集合可以具有的最大大小。
find(): It returns iterator to the element.
find():将迭代器返回到元素。
count(x): Returns how many times elements “x” present in set.
count(x):返回集合中元素“ x”出现的次数。
Example program to show usage of above functions:
显示上述功能用法的示例程序:
#include<iostream>
#include<set>
using namespace std;
int main(){
set<int> s1;
set<int> :: iterator it;
for(int i=0; i<5; i++)
s1.insert(i+10);
s1.empty() ? cout <<"list is empty" << endl: cout << "list is not empty" << endl;
cout << "size of the list is " << s1.size() << endl;
cout << "maximum size of the list is " << s1.max_size() << endl;
cout << "finding elemnt 12 in list" << endl;
it= s1.find(12);
cout << *it << endl;
s1.insert(12);
if(s1.count(22))
cout << "number 22 is in the list " << endl;
else
cout << "22 is not in the list";
return 0;
}
Output
输出量
list is not empty size of the list is 5 maximum size of the list is 461168601842738790 finding elemnt 12 in list 12 22 is not in the list
list不为空列表的 大小为5列表的 最大大小为461168601842738790 查找列表 12中的 elemnt 12 22不在列表中
Comment below if you have any queries or found any information incorrect in above tutorial for STL Set container in C++.
如果您在上面的教程中对C ++中的STL Set容器有任何疑问或发现任何不正确的信息,请在下面评论。
翻译自: https://www.thecrazyprogrammer.com/2017/08/stl-set.html
stl set容器