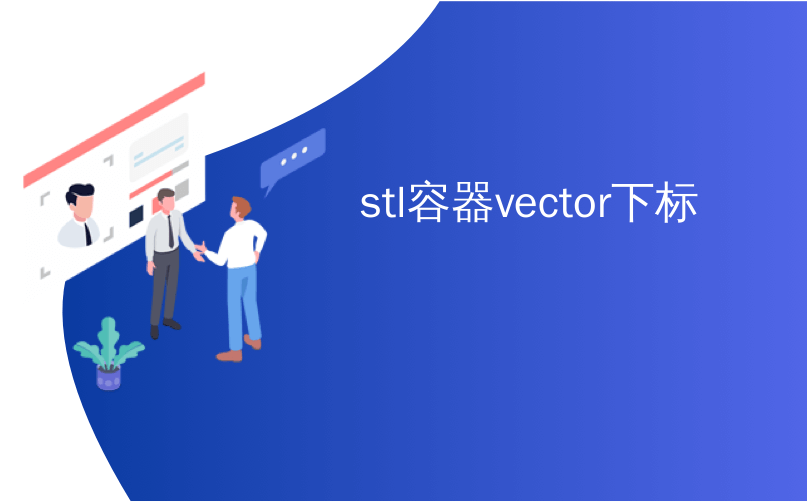
stl容器vector下标
Here you will learn about C++ STL Vector Container i.e. std::vector and various functions applicable on it.
在这里,您将了解C ++ STL矢量容器,即std :: vector及其适用的各种功能。
Vector, as we discussed earlier vector is dynamic array that grows in one direction.
正如我们前面讨论的,向量是在一个方向上增长的动态数组。

Also Read: C++ STL Array Container – std::array
另请阅读: C ++ STL数组容器– std :: array
C ++ STL向量 (C++ STL Vector)
Vectors are dynamic arrays. When we insert a new element or delete an element from vector, it has ability to resize itself automatically. Vector elements also stored in contiguous memory locations, so that they can traverse and accessed by iterators. Inserting and deleting at end takes constant , O(1) time. By using vectors we can avoid array_index_outof_bound exceptions.
向量是动态数组。 当我们插入一个新元素或从vector中删除一个元素时,它具有自动调整自身大小的能力。 向量元素也存储在连续的内存位置中,以便它们可以遍历并由迭代器访问。 最后插入和删除需要常数O(1)时间。 通过使用向量,我们可以避免array_index_outof_bound异常。
STL向量的语法 (Syntax of STL Vector)
vector <data_type> vectorName;
vector <数据类型> vectorName;
向量容器使用的功能 (Functions Used with Vector Container)
push_back(): This is used to insert element into vector. It inserts element at the end of the vector.
push_back():用于将元素插入向量。 它将元素插入向量的末尾。
[] operator: This operator returns the reference of the element positioned at index where we access like vectorName[index_position].
[]运算符:此运算符返回位于我们访问索引处的元素的引用,例如vectorName [index_position]。
at(): This operator also useful to access the element at particular position.
at():此运算符也可用于访问特定位置的元素。
front(): This returns the reference to the first element of the vector.
front():这将返回对向量的第一个元素的引用。
back(): This returns the reference to the last element of the vector.
back():这将返回对向量的最后一个元素的引用。
Example program to show above mentioned functions:
显示上述功能的示例程序:
#include<iostream>
#include<vector>
using namespace std;
int main(){
vector<int> vec; // syntax for defining a vector
// inserting elements into vector by push_back funtion
for(int i=0;i<6;i++)
vec.push_back(i);
// accessing using [] operator
for(int i=0;i<3;i++)
cout<< vec[i] << " ";
// accessing using at()
for(int i=3;i<6;i++)
cout << vec.at(i) << " ";
cout << endl;
// returning front element
cout << vec.front() << endl;
//returning last element
cout << vec.back() << endl;
return 0;
}
Output
输出量
0 1 2 3 4 5 0 5
0 1 2 3 4 5 0 5
size(): It gives the number of elements present in the vector.
size():给出向量中存在的元素数量。
max_size(): It gives the maximum number of elements that a vector can hold.
max_size():给出向量可以容纳的最大元素数。
capacity(): We said that, vector is dynamic array which grow by inserting elements. When we declare it system allocates some space to it. Particular number of elements it can hold. If we insert more than that elements, system allocates some more space for it (at new location by free-up old space).
Capacity():我们说过,向量是动态数组,通过插入元素来增长。 当我们声明它时,系统会为其分配一些空间。 它可以容纳的元素的特定数量。 如果我们插入的元素多于该元素,则系统会为其分配更多的空间(在新位置释放旧空间)。
Capacity() returns how many items can be fit in the vector before it is “full”. Once full, adding new items will result in a new, larger block of memory being allocated and the existing items being copied to it.
Capacity()返回向量“满”之前可容纳多少个项目。 一旦装满,添加新项目将导致分配一个更大的新内存块,并将现有项目复制到其中。
resize(): It resize the vector, restrict to contain only some number of elements.
resize():它调整向量的大小,限制为仅包含一些元素。
empty(): This is Boolean function. Returns whether the vector is empty or not.
empty():这是布尔函数。 返回向量是否为空。
Example Program to show above functions:
显示上述功能的示例程序:
#include<iostream>
#include<vector>
using namespace std;
int main(){
vector<int> vec;
for(int i=0;i<5;i++) vec.push_back(i);
cout << "size of the vector is " << vec.size() << endl; // present number of elements
cout << "Maximum size is " << vec.max_size() << endl; // maximum number of elements can hold
cout << "Capacity of the vector is " << vec.capacity() << endl; //
vec.resize(0); // restricting vector to contain zero elements
vec.empty() ? cout << "vector is empty" << endl: cout << "vector is not empty " << endl; //checking empty conditon
return 0;
}
Output
输出量
size of the vector is 5 Maximum size is 4611686018427387903 Capacity of the vector is 8 vector is empty
向量的大小为5 最大大小为4611686018427387903 向量的容量为8 向量为空
Vector with Iterator
向量与迭代器
begin(): Returns an iterator pointing to the first element of the vector.
begin():返回指向向量第一个元素的迭代器。
end(): Returns an iterator pointing to the present last element of the vector.
end():返回一个迭代器,该迭代器指向向量的当前最后一个元素。
rbegin(): Returns a reverse iterator pointing to the last element in the vector. Used to move from last to first
rbegin():返回指向向量中最后一个元素的反向迭代器。 从最后移到第
rend(): Returns a reverse iterator pointing to the first element in the vector.
rend():返回指向向量中第一个元素的反向迭代器。
Example program:
示例程序:
#include<iostream>
#include<vector>
using namespace std;
int main(){
vector<int> vec;
vector<int> :: iterator it1;
vector<int> :: reverse_iterator it2;
for(int i=0;i<5;i++) vec.push_back(i);
// elements form start to end
cout << "elements in the vector from start to end ";
for(it1 = vec.begin(); it1!= vec.end();it1++)
cout << *it1 << " ";
cout << endl;
// elements form end to start
cout << "elements in the vector from end to start ";
for(it2 = vec.rbegin(); it2!= vec.rend();it2++)
cout << *it2 << " ";
cout << endl;
return 0;
}
Output
输出量
elements in the vector from start to end 0 1 2 3 4 elements in the vector from end to start 4 3 2 1 0
向量中从头到尾的元素0 1 2 3 4 向量中从头到尾的元素4 3 2 1 0
assign(): Assign new content to vector and resize.
Assign ():将新内容分配给vector并调整大小。
pop_back(): This removes the element at the end of the vector. So that, size of the vector also reduced by 1.
pop_back():这将删除向量结尾处的元素。 因此,向量的大小也减少了1。
insert(iterator, element): This inserts the element in vector before the position pointed by iterator. This insert method can be overloaded by third variable count. This says how many times the element to be inserted before the pointed position.
insert(iterator,element):将元素插入向量中迭代器指向的位置之前。 此插入方法可以由第三个变量计数重载。 这表示要在指向位置之前插入元素多少次。
Example program to show above methods:
显示上述方法的示例程序:
#include<iostream>
#include<vector>
using namespace std;
int main(){
vector <int> vec1;
vector <int> vec2;
vector <int> :: iterator it;
vec1.assign(4,100); // inserting element 100 into vector 4 times.
it = vec1.begin();
vec2.assign(it+1, vec1.end()); // inserts 3 elements of vec1
cout << "Vector1 elements are " << endl;
for(int i=0;i< vec1.size();i++)
cout << vec1[i] << " ";
cout << endl;
cout << "Vector2 elements are " << endl;
for(int i=0;i< vec2.size();i++)
cout << vec2[i] << " ";
cout << endl;
vec2.push_back(10);
cout << "new value inserted into vector2. Last element is " << vec2.back() << endl;
vec2.pop_back();
cout << "after pop_back operation last element of vector2 is " << vec2.back() << endl;
vector <int> vec3(3,10);
it = vec3.begin();
it = vec3.insert(it,20); // this inserts element 20 as first element
cout << "Now first element of vec3 is " << vec3.front();
return 0;
}
Output
输出量
Vector1 elements are 100 100 100 100 Vector2 elements are 100 100 100 new value inserted into vector2. Last element is 10 after pop_back operation last element of vector2 is 100 Now first element of vec3 is 20
向量1元件 100个100 100 100 Vector2元件是 插入vector2 100 100 100 新值。 pop_back操作后, 最后一个元素为10 vector2的最后一个元素为100 现在vec3的第一个元素为20
erase(): Removes the element pointed by the iterator position. This erase method can be overloaded with extra iterator that specifies the range to be removed.
delete():删除迭代器位置指向的元素。 可以使用额外的迭代器来重载此擦除方法,该迭代器指定要删除的范围。
Example program:
示例程序:
#include<iostream>
#include<vector>
using namespace std;
int main(){
vector <int> vec;
vector <int> :: iterator it;
vec.push_back(100);
for(int i=0;i<5;i++)
vec.push_back(i);
cout << "first element before erasing is " << vec.front() << endl;
it = vec.begin();
vec.erase(it); // removes first element of the vector
cout << "first element after erasing is " << vec.front() << endl;
vec.erase(vec.begin(), vec.end()); // this removes elements in the vector from first to last
//checking vector empty or not
vec.empty() ? cout << "vector is empty " << endl : cout << "Vector is not empty " <<endl;
return 0;
}
Output
输出量
first element before erasing is 100 first element after erasing is 0 vector is empty
擦除前的第一个元素为100擦除 后的第一个元素为0 矢量为空
swap( vector1, vector2): This swaps the all elements of vector1 to vector2 and vector2 to vector1.
swap(vector1,vector2):将vector1的所有元素交换为vector2,将vector2的所有元素交换为vector1。
clear(): This removes the all elements of the vector.
clear():这将删除向量的所有元素。
Example program:
示例程序:
#include<iostream>
#include<vector>
using namespace std;
int main(){
vector <int> vec1;
vector <int> vec2;
for(int i=1;i<6;i++)
vec1.push_back(i);
for(int i=11;i<16;i++)
vec2.push_back(i);
cout << "Vector1 elements before swapping are " << endl;
for(int i=0;i<5;i++)
cout << vec1[i] << " ";
cout << endl;
cout << "Vector2 elements before swapping are " << endl;
for(int i=0;i<5;i++)
cout << vec2[i] << " ";
cout << endl;
swap(vec1,vec2);
cout << "Vector1 elements after swapping are " << endl;
for(int i=0;i<5;i++)
cout << vec1[i] << " ";
cout << endl;
cout << "Vector2 elements after swapping are " << endl;
for(int i=0;i<5;i++)
cout << vec2[i] << " ";
cout << endl;
// clearing vector1
vec1.clear();
//checking vector empty or not
vec1.empty() ? cout << "vector is empty " << endl : cout << "Vector is not empty " <<endl;
return 0;
}
Output
输出量
Vector1 elements before swapping are 1 2 3 4 5 Vector2 elements before swapping are 11 12 13 14 15 Vector1 elements after swapping are 11 12 13 14 15 Vector2 elements after swapping are 1 2 3 4 5 vector is empty
交换前的Vector1元素为 1 2 3 4 5 交换前的Vector2元素为 11 12 13 14 15 交换后的Vector1元素为 11 12 13 14 15 交换后的Vector2元素为 1 2 3 4 5 向量为空
Comment below if you have queries or found any information incorrect in above tutorial for C++ stl vector container.
如果您对上面的C ++ stl矢量容器教程有疑问或发现任何不正确的信息,请在下面评论。
翻译自: https://www.thecrazyprogrammer.com/2017/07/stl-vector.html
stl容器vector下标