This tutorial will help you to know about concept of TCP/IP Socket Programming in C and C++ along with client server program example.
本教程将帮助您了解C和C ++中的TCP / IP套接字编程的概念以及客户端服务器程序示例。
什么是插座? (What is Socket?)
We know that in Computer Networks, communication between server and client using TCP/IP protocol is connection oriented (which buffers and bandwidth are reserved for client). Server will get so many hits from different clients, and then server has to identify each client uniquely to reply every request. To achieve this we use “ip address of client (32 bit) + port number (16 bit) of the process”. This is called Socket (48 bit). Any network communication should goes through socket.
我们知道在计算机网络中,使用TCP / IP协议的服务器和客户端之间的通信是面向连接的(缓冲区和带宽是为客户端保留的)。 服务器将从不同的客户端获得如此多的点击,然后服务器必须唯一地标识每个客户端以回复每个请求。 为此,我们使用“客户端的IP地址(32位)+进程的端口号(16位)”。 这称为套接字(48位)。 任何网络通信都应通过套接字进行。
客户端-服务器通信中的过程 (Procedure in Client-Server Communication)
Socket: Create a new communication
套接字:创建新的通信
Bind: Attach a local address to a socket
绑定:将本地地址附加到套接字
Listen: Announce willingness to accept connections
听:宣布愿意接受联系
Accept: Block caller until a connection request arrives
接受:阻止呼叫者,直到连接请求到达
Connect: Actively attempt to establish a connection
连接:积极尝试建立连接
Send: Send some data over connection
发送:通过连接发送一些数据
Receive: Receive some data over connection
接收:通过连接接收一些数据
Close: Release the connection
关闭:释放连接
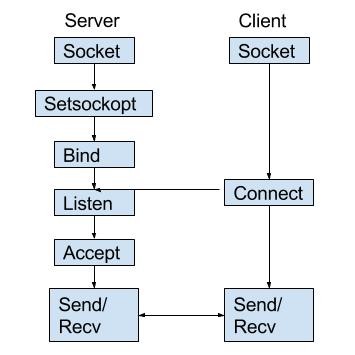
使用C和C ++的套接字编程的客户端服务器程序 (Client Server Program Using Socket Programming in C and C++)
Let’s see how to create server and client using C programming. Below code will work in C++ also.
让我们看看如何使用C编程来创建服务器和客户端。 下面的代码也将在C ++中工作。
We now create a server which run continuously, and if any client hit the server with a request then server will send it’s date and time. To know the success message we here printing that time and date.
现在,我们创建一个连续运行的服务器,如果有任何客户端通过请求将其击中服务器,则服务器将发送其日期和时间。 要了解成功消息,我们在此打印该时间和日期。
服务器 (server.c)
#include <stdio.h> // standard input and output library
#include <stdlib.h> // this includes functions regarding memory allocation
#include <string.h> // contains string functions
#include <errno.h> //It defines macros for reporting and retrieving error conditions through error codes
#include <time.h> //contains various functions for manipulating date and time
#include <unistd.h> //contains various constants
#include <sys/types.h> //contains a number of basic derived types that should be used whenever appropriate
#include <arpa/inet.h> // defines in_addr structure
#include <sys/socket.h> // for socket creation
#include <netinet/in.h> //contains constants and structures needed for internet domain addresses
int main()
{
time_t clock;
char dataSending[1025]; // Actually this is called packet in Network Communication, which contain data and send through.
int clintListn = 0, clintConnt = 0;
struct sockaddr_in ipOfServer;
clintListn = socket(AF_INET, SOCK_STREAM, 0); // creating socket
memset(&ipOfServer, '0', sizeof(ipOfServer));
memset(dataSending, '0', sizeof(dataSending));
ipOfServer.sin_family = AF_INET;
ipOfServer.sin_addr.s_addr = htonl(INADDR_ANY);
ipOfServer.sin_port = htons(2017); // this is the port number of running server
bind(clintListn, (struct sockaddr*)&ipOfServer , sizeof(ipOfServer));
listen(clintListn , 20);
while(1)
{
printf("\n\nHi,Iam running server.Some Client hit me\n"); // whenever a request from client came. It will be processed here.
clintConnt = accept(clintListn, (struct sockaddr*)NULL, NULL);
clock = time(NULL);
snprintf(dataSending, sizeof(dataSending), "%.24s\r\n", ctime(&clock)); // Printing successful message
write(clintConnt, dataSending, strlen(dataSending));
close(clintConnt);
sleep(1);
}
return 0;
}
Explanation
说明
1. socket() function creates a new socket inside kernel and returns an integer which used as socket descriptor.
1. socket()函数在内核内部创建一个新的套接字,并返回一个用作套接字描述符的整数。
2. For IP4 address we are sending first argument as AF_INET. You can also see we are assigning ipOfServer = AF_INET, represents that this argument related to Internet IP addresses.
2.对于IP4地址,我们将第一个参数作为AF_INET发送。 您还可以看到我们正在分配ipOfServer = AF_INET,表示此参数与Internet IP地址有关。
3. SOCK_STREAM argument confirms that we are using TCP as a protocol in this communication, which is reliable communication.
3. SOCK_STREAM参数确认在此通信中我们正在使用TCP作为协议,这是可靠的通信。
4. Sending ‘0’ as third argument is, we are saying to kernel that use default protocol
4.发送“ 0”作为第三个参数,是对使用默认协议的内核说的
5. Next we have to bind the created socket to structure ipOfServer. For this we are calling bind() functional. Which includes port, ip addresses as details.
5.接下来,我们必须将创建的套接字绑定到结构ipOfServer。 为此,我们调用bind()函数。 其中包括端口,IP地址作为详细信息。
6. listen() also an inbuilt function the 2nd argument 20 says that maximum 20 number of clients can connect to that server. So maximum 20 queue process can be handled by server.
6. listen()也是一个内置函数,第二个参数20表示最多可以连接20个客户端。 因此,服务器最多可以处理20个队列。
7. Upto now server started. Next server waits for client request by accept() function.
7.到目前为止,服务器已启动。 下一个服务器通过accept()函数等待客户端请求。
8. accept() runs infinite loop to keep server always running. But it may eat up all CPU processing, to avoid that we have written sleep(1), which server went to sleep for 1 sec.
8. accept()运行无限循环以保持服务器始终运行。 但是它可能会耗尽所有CPU处理能力,以避免我们编写了sleep(1),该服务器进入睡眠状态1秒钟。
9. When server hit by client, it prints date and time on clients socket through descriptor returned by accept().
9.当服务器被客户端命中时,它将通过accept()返回的描述符在客户端套接字上打印日期和时间。
客户端 (client.c)
#include <sys/socket.h>
#include <sys/types.h>
#include <netinet/in.h>
#include <netdb.h>
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <unistd.h>
#include <errno.h>
#include <arpa/inet.h>
int main()
{
int CreateSocket = 0,n = 0;
char dataReceived[1024];
struct sockaddr_in ipOfServer;
memset(dataReceived, '0' ,sizeof(dataReceived));
if((CreateSocket = socket(AF_INET, SOCK_STREAM, 0))< 0)
{
printf("Socket not created \n");
return 1;
}
ipOfServer.sin_family = AF_INET;
ipOfServer.sin_port = htons(2017);
ipOfServer.sin_addr.s_addr = inet_addr("127.0.0.1");
if(connect(CreateSocket, (struct sockaddr *)&ipOfServer, sizeof(ipOfServer))<0)
{
printf("Connection failed due to port and ip problems\n");
return 1;
}
while((n = read(CreateSocket, dataReceived, sizeof(dataReceived)-1)) > 0)
{
dataReceived[n] = 0;
if(fputs(dataReceived, stdout) == EOF)
{
printf("\nStandard output error");
}
printf("\n");
}
if( n < 0)
{
printf("Standard input error \n");
}
return 0;
}
Explanation
说明
This code can connect to server and receive date and time from server.
此代码可以连接到服务器并从服务器接收日期和时间。
1. Since this communication through socket, here also, we created socket.
1.由于通过套接字进行通信,因此在这里,我们也创建了套接字。
2. Port number of the process and IP address both bundled in a structure. We connect these with socket
2.进程的端口号和IP地址都捆绑在一个结构中。 我们用插座连接这些
3. Once sockets are connected, the server sends the date and time to client socket through clients socket descriptor.
3.连接套接字后,服务器通过客户端套接字描述符将日期和时间发送到客户端套接字。
如何运行server.c和client.c文件? (How to run server.c and client.c files?)
First run server.c file and create an output file for that in Unix or Linux.
首先运行server.c文件,并在Unix或Linux中为该文件创建一个输出文件。
Type gcc server.c -o server command in terminal.
在终端中输入gcc server.c -o server命令。
Now run the server using ./server
现在使用./server运行服务器
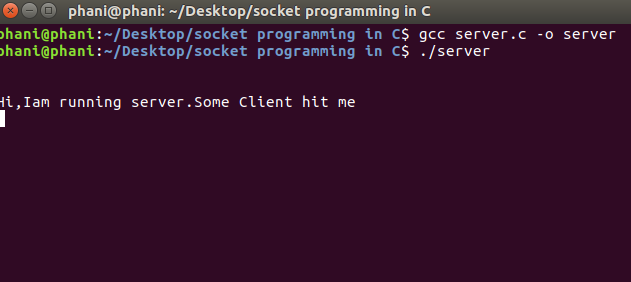
After running the server just minimize the terminal. Open new terminal. Do remaining work there.
运行服务器后,只需最小化终端即可。 打开新终端。 在那里做剩余的工作。
To know the server is running or not we can check using netstat command.
要知道服务器是否正在运行,我们可以使用netstat命令进行检查。
After opening new terminal, type sudo netstat -ntlp
打开新终端后,键入sudo netstat -ntlp
Here we can see running server with port 2017
在这里我们可以看到运行端口为2017的服务器
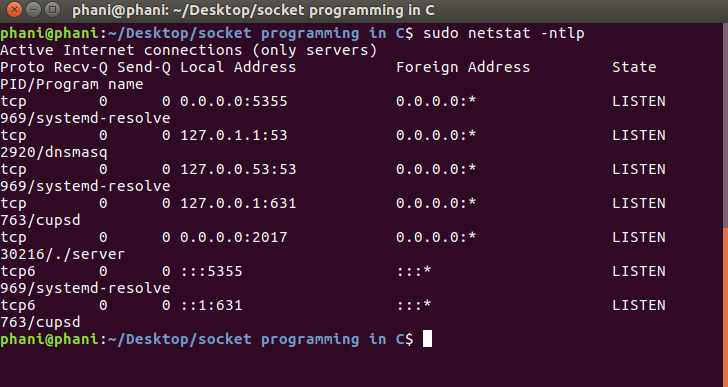
Now check with client by sending request with same port:
现在,通过使用相同端口发送请求来与客户端进行检查:
Type gcc client -o client
输入gcc client -o client
Type ./client
键入./client
After that you can see the date and time which is sent by server.
之后,您可以看到服务器发送的日期和时间。
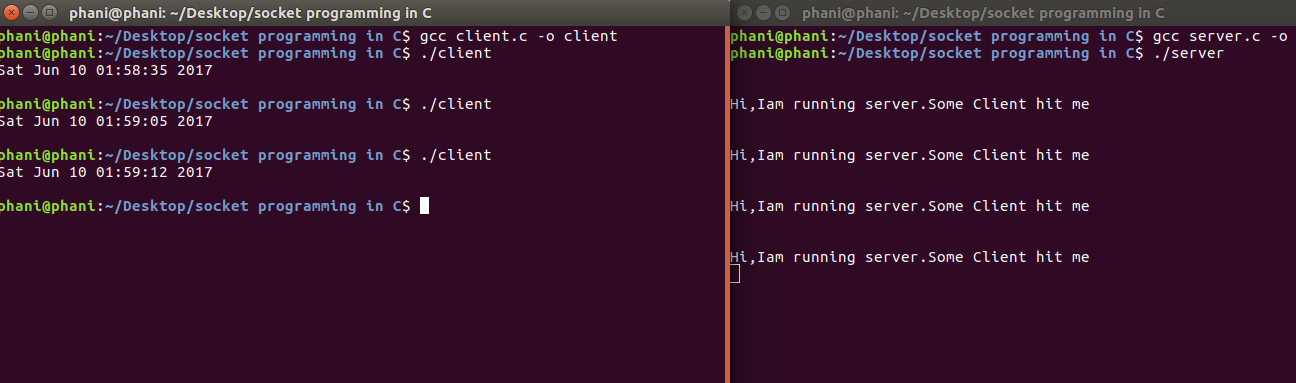
It means connection established successfully.
表示连接成功建立。
Whenever we run client program that means we are requesting the server, every time server will send date and time saying that connection established successfully.
每当我们运行客户端程序(这意味着我们在请求服务器)时,每次服务器都会发送日期和时间,表明连接已成功建立。
Comment below if you have queries or found something incorrect in above tutorial for socket programming in C and C++.
如果您在上面的教程中使用C和C ++进行套接字编程有疑问或发现不正确的地方,请在下面评论。
翻译自: https://www.thecrazyprogrammer.com/2017/06/socket-programming.html