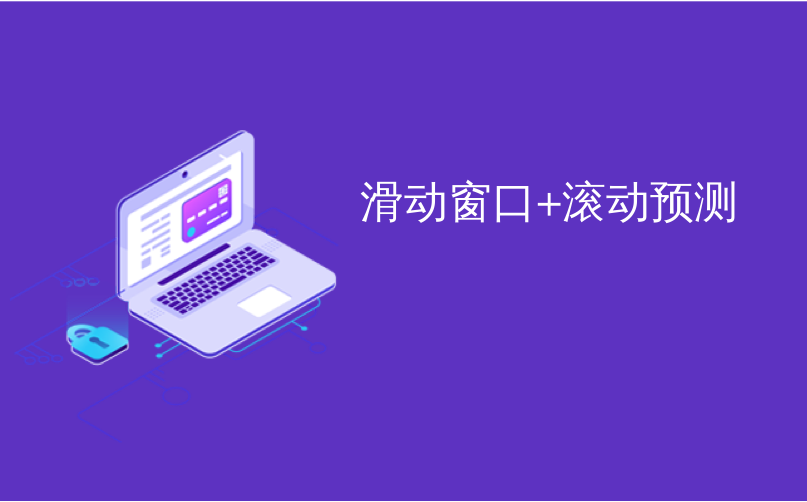
滑动窗口+滚动预测
Here you will get sliding window protocol program in C.
在这里,您将获得使用C的滑动窗口协议程序。
In computer networks sliding window protocol is a method to transmit data on a network. Sliding window protocol is applied on the Data Link Layer of OSI model. At data link layer data is in the form of frames. In Networking, Window simply means a buffer which has data frames that needs to be transmitted.
在计算机网络中,滑动窗口协议是一种在网络上传输数据的方法。 滑动窗口协议应用于OSI模型的数据链路层。 在数据链路层,数据采用帧的形式。 在联网中,窗口仅表示具有需要传输的数据帧的缓冲区。
Both sender and receiver agrees on some window size. If window size=w then after sending w frames sender waits for the acknowledgement (ack) of the first frame.
发送方和接收方都同意某些窗口大小。 如果窗口大小= w,则在发送w帧后,发送方将等待第一帧的确认(ack)。
As soon as sender receives the acknowledgement of a frame it is replaced by the next frames to be transmitted by the sender. If receiver sends a collective or cumulative acknowledgement to sender then it understands that more than one frames are properly received, for eg:- if ack of frame 3 is received it understands that frame 1 and frame 2 are received properly.
发送方一接收到帧的确认,就将其替换为发送方要发送的下一帧。 如果接收方向发送方发送了集体确认或累积确认,那么它将理解已正确接收了多个帧,例如:-如果接收到第3帧的确认,它将理解第1帧和第2帧已正确接收。
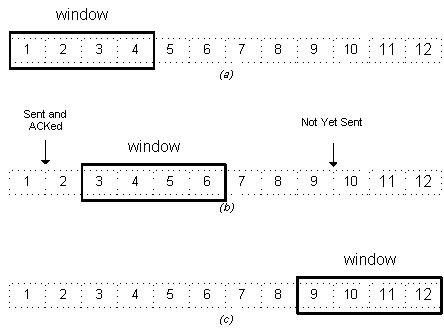
In sliding window protocol the receiver has to have some memory to compensate any loss in transmission or if the frames are received unordered.
在滑动窗口协议中,接收器必须具有一些内存,以补偿传输过程中的任何损失或无序接收帧。
Efficiency of Sliding Window Protocol
滑动窗口协议的效率
η = (W*tx)/(tx+2tp)
η=(W * t x )/(t x + 2t p )
W = Window Size
W =窗口大小
tx = Transmission time
t x =传输时间
tp = Propagation delay
t p =传播延迟
Sliding window works in full duplex mode
滑动窗口在全双工模式下工作
It is of two types:-
它有两种类型:
1. Selective Repeat: Sender transmits only that frame which is erroneous or is lost.
1.选择性重复:发送方仅发送错误或丢失的帧。
2. Go back n: Sender transmits all frames present in the window that occurs after the error bit including error bit also.
2.返回n:发送方发送窗口中存在的所有帧,该帧出现在错误位之后,包括错误位。
C语言的滑动窗口协议程序 (Sliding Window Protocol Program in C)
Below is the simulation of sliding window protocol in C.
下面是C语言中滑动窗口协议的仿真。
#include<stdio.h>
int main()
{
int w,i,f,frames[50];
printf("Enter window size: ");
scanf("%d",&w);
printf("\nEnter number of frames to transmit: ");
scanf("%d",&f);
printf("\nEnter %d frames: ",f);
for(i=1;i<=f;i++)
scanf("%d",&frames[i]);
printf("\nWith sliding window protocol the frames will be sent in the following manner (assuming no corruption of frames)\n\n");
printf("After sending %d frames at each stage sender waits for acknowledgement sent by the receiver\n\n",w);
for(i=1;i<=f;i++)
{
if(i%w==0)
{
printf("%d\n",frames[i]);
printf("Acknowledgement of above frames sent is received by sender\n\n");
}
else
printf("%d ",frames[i]);
}
if(f%w!=0)
printf("\nAcknowledgement of above frames sent is received by sender\n");
return 0;
}
Output
输出量
Enter window size: 3
输入窗口大小:3
Enter number of frames to transmit: 5
输入要传输的帧数:5
Enter 5 frames: 12 5 89 4 6
输入5帧:12 5 89 4 6
With sliding window protocol the frames will be sent in the following manner (assuming no corruption of frames)
使用滑动窗口协议时,将以以下方式发送帧(假设帧没有损坏)
After sending 3 frames at each stage sender waits for acknowledgement sent by the receiver
在每个阶段发送3帧后,发送方等待接收方发送的确认
12 5 89 Acknowledgement of above frames sent is received by sender
12 5 89 发送方已收到对以上帧的确认
4 6 Acknowledgement of above frames sent is received by sender
4 6 发送方收到上述帧的确认
C ++中的滑动窗口协议程序 (Sliding Window Protocol Program in C++)
Below is the simulation of sliding window protocol in C++.
下面是C ++中滑动窗口协议的仿真。
#include<iostream>
using namespace std;
int main()
{
int w,i,f,frames[50];
cout<<"Enter window size: ";
cin>>w;
cout<<"\nEnter number of frames to transmit: ";
cin>>f;
cout<<"\nEnter "<<f<<" frames: ";
for(i=1;i<=f;i++)
cin>>frames[i];
cout<<"\nWith sliding window protocol the frames will be sent in the following manner (assuming no corruption of frames)\n\n";
cout<<"After sending "<<w<<" frames at each stage sender waits for acknowledgement sent by the receiver\n\n";
for(i=1;i<=f;i++)
{
if(i%w==0)
{
cout<<frames[i]<<"\n";
cout<<"Acknowledgement of above frames sent is received by sender\n\n";
}
else
cout<<frames[i]<<" ";
}
if(f%w!=0)
cout<<"\nAcknowledgement of above frames sent is received by sender\n";
return 0;
}
Comment below if you have any queries regarding above program.
如果您对以上程序有任何疑问,请在下面评论。
翻译自: https://www.thecrazyprogrammer.com/2017/05/sliding-window-protocol-program-c.html
滑动窗口+滚动预测