Here you will get program for hamming code in C and C++.
在这里,您将获得用于在C和C ++中编写汉明代码的程序。
Hamming code is a popular error detection and error correction method in data communication. Hamming code can only detect 2 bit error and correct a single bit error which means it is unable to correct burst errors if may occur while transmission of data.
汉明码是数据通信中流行的错误检测和纠错方法。 汉明码只能检测2位错误并纠正单个位错误,这意味着如果在传输数据时可能发生突发错误,则汉明码将无法纠正。
Also Read: Checksum Program in C and C++
另请参阅: C和C ++中的Checksum程序
Hamming code uses redundant bits (extra bits) which are calculated according to the below formula:-
汉明码使用根据以下公式计算的冗余位(额外位):
2r ≥ m+r+1
2r≥m + r + 1
Where r is the number of redundant bits required and m is the number of data bits.
其中r是所需的冗余位数,而m是数据位数。
R is calculated by putting r = 1, 2, 3 … until the above equation becomes true.
通过将r = 1、2、3…直到上述方程式变为真,可以计算出R。
R1 bit is appended at position 20
R1位附加在位置20
R2 bit is appended at position 21
R2位附加在位置21
R3 bit is appended at position 22 and so on.
R3位附加在位置22上 ,依此类推。
These redundant bits are then added to the original data for the calculation of error at receiver’s end.
然后将这些冗余位添加到原始数据,以在接收器端计算错误。
At receiver’s end with the help of even parity (generally) the erroneous bit position is identified and since data is in binary we take complement of the erroneous bit position to correct received data.
在接收器端,借助偶数奇偶校验(通常),可以识别错误的位位置,并且由于数据是二进制的,因此我们对错误的位位置进行了补充以校正接收到的数据。
Respective index parity is calculated for r1, r2, r3, r4 and so on.
计算r1,r2,r3,r4等的相应索引奇偶校验。
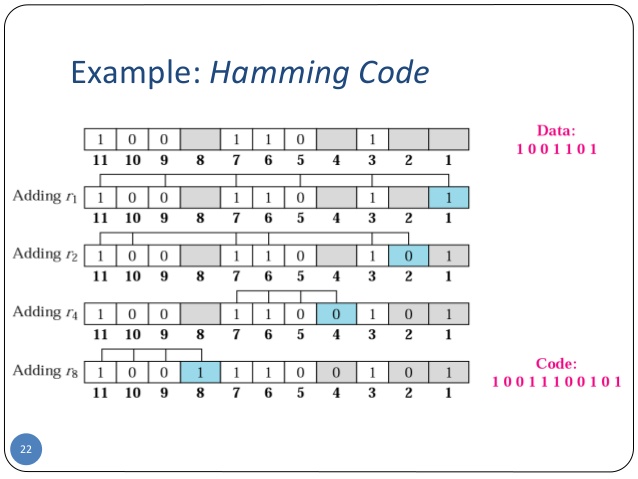
海明码的优点 (Advantages of Hamming Code)
- Easy to encode and decode data at both sender and receiver end. 易于在发送器和接收器端对数据进行编码和解码。
- Easy to implement. 易于实现。
海明码的缺点 (Disadvantages of Hamming Code)
- Cannot correct burst errors. 无法纠正突发错误。
- Redundant bits are also sent with the data therefore it requires more bandwidth to send the data. 冗余位也会与数据一起发送,因此发送数据需要更多带宽。
C语言汉明码程序 (Program for Hamming Code in C)
#include<stdio.h>
void main() {
int data[10];
int dataatrec[10],c,c1,c2,c3,i;
printf("Enter 4 bits of data one by one\n");
scanf("%d",&data[0]);
scanf("%d",&data[1]);
scanf("%d",&data[2]);
scanf("%d",&data[4]);
//Calculation of even parity
data[6]=data[0]^data[2]^data[4];
data[5]=data[0]^data[1]^data[4];
data[3]=data[0]^data[1]^data[2];
printf("\nEncoded data is\n");
for(i=0;i<7;i++)
printf("%d",data[i]);
printf("\n\nEnter received data bits one by one\n");
for(i=0;i<7;i++)
scanf("%d",&dataatrec[i]);
c1=dataatrec[6]^dataatrec[4]^dataatrec[2]^dataatrec[0];
c2=dataatrec[5]^dataatrec[4]^dataatrec[1]^dataatrec[0];
c3=dataatrec[3]^dataatrec[2]^dataatrec[1]^dataatrec[0];
c=c3*4+c2*2+c1 ;
if(c==0) {
printf("\nNo error while transmission of data\n");
}
else {
printf("\nError on position %d",c);
printf("\nData sent : ");
for(i=0;i<7;i++)
printf("%d",data[i]);
printf("\nData received : ");
for(i=0;i<7;i++)
printf("%d",dataatrec[i]);
printf("\nCorrect message is\n");
//if errorneous bit is 0 we complement it else vice versa
if(dataatrec[7-c]==0)
dataatrec[7-c]=1;
else
dataatrec[7-c]=0;
for (i=0;i<7;i++) {
printf("%d",dataatrec[i]);
}
}
}
C ++中的汉明码程序 (Program for Hamming Code in C++)
#include<iostream>
using namespace std;
int main() {
int data[10];
int dataatrec[10],c,c1,c2,c3,i;
cout<<"Enter 4 bits of data one by one\n";
cin>>data[0];
cin>>data[1];
cin>>data[2];
cin>>data[4];
//Calculation of even parity
data[6]=data[0]^data[2]^data[4];
data[5]=data[0]^data[1]^data[4];
data[3]=data[0]^data[1]^data[2];
cout<<"\nEncoded data is\n";
for(i=0;i<7;i++)
cout<<data[i];
cout<<"\n\nEnter received data bits one by one\n";
for(i=0;i<7;i++)
cin>>dataatrec[i];
c1=dataatrec[6]^dataatrec[4]^dataatrec[2]^dataatrec[0];
c2=dataatrec[5]^dataatrec[4]^dataatrec[1]^dataatrec[0];
c3=dataatrec[3]^dataatrec[2]^dataatrec[1]^dataatrec[0];
c=c3*4+c2*2+c1 ;
if(c==0) {
cout<<"\nNo error while transmission of data\n";
}
else {
cout<<"\nError on position "<<c;
cout<<"\nData sent : ";
for(i=0;i<7;i++)
cout<<data[i];
cout<<"\nData received : ";
for(i=0;i<7;i++)
cout<<dataatrec[i];
cout<<"\nCorrect message is\n";
//if errorneous bit is 0 we complement it else vice versa
if(dataatrec[7-c]==0)
dataatrec[7-c]=1;
else
dataatrec[7-c]=0;
for (i=0;i<7;i++) {
cout<<dataatrec[i];
}
}
return 0;
}
Output
输出量
Enter 4 bits of data one by one 1 0 1 0
一对一输入4位数据 1 0 1 0
Encoded data is 1010010
编码数据为 1010010
Enter received data bits one by one 1 0 1 0 0 1 0
一对一输入接收到的数据位 1 0 1 0 0 1 0
No error while transmission of data
数据传输无误
Code Source: http://scanftree.com/programs/c/implementation-of-hamming-code/
代码源: http : //scanftree.com/programs/c/implementation-of-hamming-code/
This article is submitted by Rahul Maheshwari. You can connect with him on facebook.
本文由Rahul Maheshwari提交。 您可以在Facebook上与他建立联系。
Comment below if you have any queries related to above hamming code program in C and C++.
如果您对以上C和C ++中的汉明代码程序有任何疑问,请在下面评论。
翻译自: https://www.thecrazyprogrammer.com/2017/03/hamming-code-c.html