Here you will get android SharedPreferences example.
在这里,您将获得android SharedPreferences示例。
SharedPreferences in android is used to save data which is available across entire application and the data persists even after the application is closed. It is widely used for session management in android applications. SharedPreferences uses key-value pair to save and retrieve data.
android中的SharedPreferences用于保存可在整个应用程序中使用的数据,即使关闭应用程序,该数据也会保留。 它被广泛用于android应用程序中的会话管理。 SharedPreferences使用键值对保存和检索数据。
保存数据 (Save Data)
First of all we have to get SharedPreferences instance by calling getSharedPreferences() method. It can be done in following way.
首先,我们必须通过调用getSharedPreferences()方法来获取SharedPreferences实例。 可以通过以下方式完成。
SharedPreferences sp = getSharedPreferences(YourPreferencesKey,Mode);
Here first parameter is the user define key in string format and second parameter is the mode. The various modes that we can use are:
这里的第一个参数是字符串格式的用户定义键,第二个参数是模式。 我们可以使用的各种模式是:
MODE_APPEND
MODE_APPEND
MODE_ENABLE_WRITE_AHEAD_LOGGING
MODE_ENABLE_WRITE_AHEAD_LOGGING
MODE_MULTI_PROCESS
MODE_MULTI_PROCESS
MODE_PRIVATE
MODE_PRIVATE
MODE_WORLD_READABLE
MODE_WORLD_READABLE
MODE_WORLD_WRITEABLE
MODE_WORLD_WRITEABLE
Next step is to get SharedPreferences.Editor class object by calling edit() method using SharedPreferences object. It can be done in following way.
下一步是通过使用SharedPreferences对象调用edit()方法来获取SharedPreferences.Editor类对象。 可以通过以下方式完成。
SharedPreferences.Editor editor = sp.edit();
After that call putString() method using Editor object.
之后,使用Editor对象调用putString()方法。
editor.putString(key,value);
Here both key and value are in string format. There are some other methods like putBoolean(), putInt(), putLong() and putFloat() to save boolean, integer, long and float values respectively.
这里的key和value都是字符串格式。 有分别一些其他方法,如putBoolean(),putInt(),putLong()和putFloat()以保存布尔,整数,长和浮点值。
Finally call commit() method to save the changes.
最后,调用commit()方法以保存更改。
editor.commit();
检索数据 (Retrieve Data)
First get instance of SharedPreferences by calling getSharedPreferences() method. After that use getString() method to fetch the data. It can be done in following way.
首先通过调用getSharedPreferences()方法获取SharedPreferences实例。 之后,使用getString()方法获取数据。 可以通过以下方式完成。
SharedPreferences sp = getSharedPreferences(YourPreferencesKey,Mode);
sp.getString(key,default_value);
When there is no data associated with given key then the default value is returned. There are some other methods to fetch data, like getBoolean(), getInt(), getFloat() and getLong().
如果没有与给定键关联的数据,则返回默认值。 还有其他一些获取数据的方法,例如getBoolean() , getInt() , getFloat()和getLong() 。
We can use contains() method to check whether SharedPreferences contain some value or not.
我们可以使用contains()方法来检查SharedPreferences是否包含某些值。
if(sp.contains(key)) {
//perform some task here
}
修改资料 (Modify Data)
To modify any data, save the data using the same key that you used earlier. It will overwrite new data on previous data.
要修改任何数据,请使用先前使用的相同密钥保存数据。 它将在以前的数据上覆盖新数据。
删除资料 (Delete Data)
Use remove() method to delete a data associated with particular key. You can use clear() method to delete all the data present in SharedPreferences. It can be done in following way.
使用remove()方法删除与特定键关联的数据。 您可以使用clear()方法删除SharedPreferences中存在的所有数据。 可以通过以下方式完成。
editor.remove(key); //remove single data
editor.clear(); //remove all data
editor.commit();
You must always call commit() method after doing any changes in data.
对数据进行任何更改后,必须始终调用commit()方法。
Below I have shared one simple example that will show how SharedPreferences is used in android.
下面我分享了一个简单的示例,该示例将展示如何在Android中使用SharedPreferences。
Android SharedPreferences示例 (Android SharedPreferences Example)
Create a project with package name thecrazyprogrammer.androidexample. Now paste following code in respective files.
创建一个程序包名称为thecrazyprogrammer.androidexample的项目。 现在,将以下代码粘贴到各个文件中。
activity_main.xml (activity_main.xml)
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent"
android:layout_height="match_parent" tools:context=".MainActivity"
android:orientation="vertical"
android:layout_marginLeft="15dp"
android:layout_marginRight="15dp"
android:layout_marginTop="15dp"
android:layout_marginBottom="15dp">
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/text"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Save"
android:id="@+id/saveButton"
android:onClick="buttonAction"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Remove"
android:id="@+id/removeButton"
android:onClick="buttonAction"/>
</LinearLayout>
MainActivity.java (MainActivity.java)
package thecrazyprogrammer.androidexample;
import android.app.Activity;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends Activity {
Button saveButton,removeButton;
EditText text;
SharedPreferences sp;
SharedPreferences.Editor editor;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
saveButton=(Button)findViewById(R.id.saveButton);
removeButton=(Button)findViewById(R.id.removeButton);
text=(EditText)findViewById(R.id.text);
sp=getSharedPreferences("TheCrazyProgrammer",MODE_PRIVATE);
editor=sp.edit();
if(sp.contains("name")){
text.setText(sp.getString("name","null"));
}
}
public void buttonAction(View view) {
if(view.getId()==R.id.saveButton){
editor.putString("name",text.getText().toString());
editor.commit();
Toast.makeText(getApplicationContext(),"Preferences Saved",Toast.LENGTH_LONG).show();
}
if(view.getId()==R.id.removeButton){
editor.clear();
editor.commit();
Toast.makeText(getApplicationContext(),"Preferences Removed",Toast.LENGTH_LONG).show();
}
}
}
Run project and save some data using textbox and save button. Now close the application and run it again. You will see that the textbox display the data you have saved earlier. This proves that data is not lost even after closing the application. You can use remove button to delete the data.
运行项目并使用文本框和保存按钮保存一些数据。 现在关闭应用程序,然后再次运行。 您将看到该文本框显示您之前保存的数据。 这证明即使关闭应用程序后数据也不会丢失。 您可以使用删除按钮删除数据。
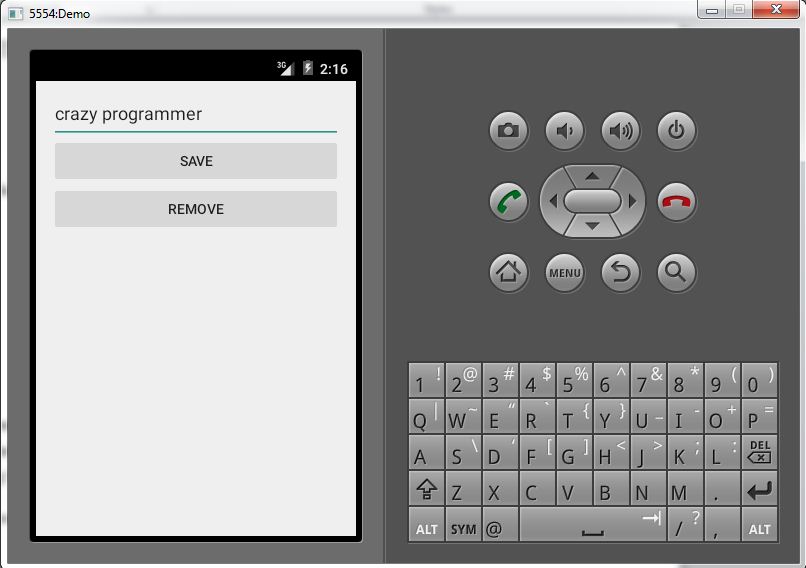
Comment below if you have any doubts regarding above android SharedPreferences example.
如果您对上述android SharedPreferences示例有任何疑问,请在下面评论。
Happy Coding!! 🙂 🙂
快乐编码! 🙂
翻译自: https://www.thecrazyprogrammer.com/2016/01/android-sharedpreferences-example.html