In this Python GUI programming tutorial you will learn about how to make GUI programs using Python Tkinter toolkit.
在此Python GUI编程教程中,您将学习如何使用Python Tkinter工具包制作GUI程序。
Graphical User Interface, also known as GUI is one of the best features in programming that makes a program look visually more attractive than the normal text based programs covered with a black or any other static color. GUI makes user interactions much easier and consistent.
图形用户界面(也称为GUI)是编程中的最佳功能之一,它使程序在视觉上比用黑色或任何其他静态颜色覆盖的常规文本程序更具吸引力。 GUI使用户交互变得更加轻松和一致。
Python GUI编程 (Python GUI Programming)
To create a GUI program in Python, you will need to use a GUI toolkit. One of the widely used toolkit available in Python is Tkinter. It is one of the most stable and popular GUI toolkit that Python developers use.
要使用Python创建GUI程序,您将需要使用GUI工具箱。 Tkinter是Python中广泛使用的工具包之一。 它是Python开发人员使用的最稳定和最受欢迎的GUI工具箱之一。
安装Tkinter模块 (Install Tkinter Module)
Tkinter module is by default available in Windows operating system. However, if you’re using any other operating system, you will need to download the module separately.
默认情况下,Tkinter模块在Windows操作系统中可用。 但是,如果使用任何其他操作系统,则需要单独下载该模块。
For Windows OS https://www.python.org/downloads/
对于Windows操作系统 https://www.python.org/downloads/
For Linux OS
对于Linux OS
sudo apt-get install python-tk (For Python 2.x) sudo apt-get install python3-tk (For Python 3.x)
sudo apt-get install python-tk(对于Python 2.x) sudo apt-get install python3-tk(对于Python 3.x)
You can create GUI elements by instantiating objects from classes pre-defined in Tkinter module, included in the Tkinter Toolkit.
您可以通过从Tkinter工具包中包含的Tkinter模块中预定义的类实例化对象来创建GUI元素。
Some of the GUI Elements in Tkinter module are listed below.
下面列出了Tkinter模块中的一些GUI元素。
Frame: It holds other GUI elements such as Label, Button, TextBox ,etc.
框架:它包含其他GUI元素,例如Label,Button,TextBox等。
Label: It displays an uneditable text or icons on the screen layout.
标签:它在屏幕布局上显示不可编辑的文本或图标。
Button: It performs an action when the user activates it or presses it using mouse.
按钮:当用户激活它或使用鼠标按下它时,它将执行一个动作。
Text Entry: It accepts one line of text and displays it.
文字输入:它接受一行文字并显示。
Text Box: This GUI element accepts multiple lines of text and displays it.
文本框:此GUI元素接受多行文本并将其显示。
Check Button: It allows the user to select or unselect an option.
Check Button:它允许用户选择或取消选择一个选项。
Radio Button: This button provides the user to select one option from several options listed.
单选按钮:此按钮使用户可以从列出的多个选项中选择一个选项。
GUI programs are traditionally event driven. Event driven means that the Buttons, Icons or any other graphical object on the screen respond to actions regardless of the order in which they occur. They respond to the actions performed by the user and not the logical flow as we saw in text based programming before.
GUI程序传统上是事件驱动的。 事件驱动意味着按钮,图标或屏幕上的任何其他图形对象对操作做出响应,而与操作发生的顺序无关。 它们响应用户执行的操作,而不是我们之前在基于文本的编程中看到的逻辑流程。
Python GUI编程示例 (Python GUI Programming Example)
from Tkinter import *
root= Tk()
root.title("My First GUI")
root.geometry("500x500")
root.mainloop()
Output
输出量
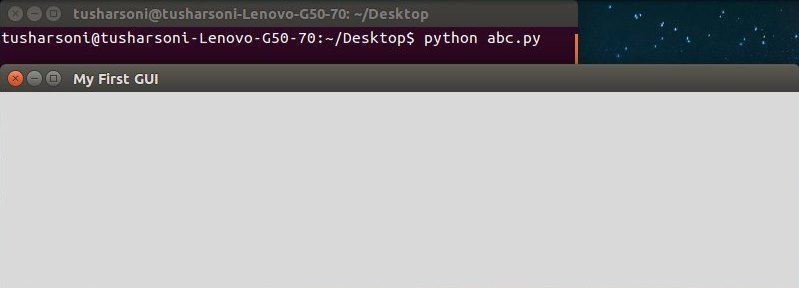
The first line of the program code imports the Tkinter module from the Python library in the namesapce of the current program.
程序代码的第一行从当前程序的名称空间中的Python库导入Tkinter模块。
The second statement is used to instantiate an object of the Tkinter class Tk and it is assigned to a user defined variable root.
第二条语句用于实例化Tkinter类Tk的对象,并将其分配给用户定义的变量root。
The third statement uses the title() method that describes the title to be displayed on to the window title bar. It takes in a string argument.
第三条语句使用title()方法,该方法描述要显示在窗口标题栏上的标题。 它接受一个字符串参数。
The third statement uses a geometry() method which is used to set the dimensions of the window. It takes in a string (and not integers) as arguments that represents the window’s width and height, separated by the “x” character.
第三条语句使用geometry()方法设置窗口的尺寸。 它接受一个字符串(而不是整数)作为参数, 该 参数 表示窗口的宽度和高度,以“ x”字符分隔。
Next statement finally starts up the GUI window application and waits in for the user to command in.
下一条语句最终启动GUI窗口应用程序,并等待用户输入。
Note: You can only have one root window in a Tkinter program. If you try to create multiple windows in one single program, you program is bound to crash.
注意: Tkinter程序中只能有一个根窗口。 如果您尝试在一个程序中创建多个窗口,则程序肯定会崩溃。
Python Tkinter框架 (Python Tkinter Frame)
A Frame is a widget or a base widget which is used to place in other widget such as labels, text boxes and others. This are an important first step as nothing can be done unless frames are developed. It basically holds other widgets.
框架是用于放置在其他小部件(例如标签,文本框等)中的小部件或基本小部件。 这是重要的第一步,因为除非开发框架,否则什么也做不了。 它基本上包含其他小部件。
app=Frame(root)
app =框架(根)
Here, we have passed root to the Frame constructor. As a result, the new frame is placed inside the root window.
在这里,我们已将root传递给Frame构造函数。 结果,新框架被放置在根窗口内。
app.grid()
app.grid()
grid() method is the one that all widgets have. It’s associated with a layout manager, which lets you arrange widgets in a Frame.
grid()方法是所有小部件都具有的方法。 它与布局管理器关联,该管理器使您可以在框架中排列小部件。
Python Tkinter标签 (Python Tkinter Label)
GUI elements are called widgets. Label is one of the simplest widget. It consists of uneditable text or icons (or both). It’s often used to label other widgets. Labels aren’t interactive. You won’t generate any command by clicking on a label. But, labels are important for naming other widgets so that there is no confusion for the end user.
GUI元素称为小部件。 标签是最简单的小部件之一。 它由不可编辑的文本或图标(或两者)组成。 它通常用于标记 其他小部件。 标签不是交互式的。 单击标签将不会生成任何命令。 但是,标签对于命名其他小部件很重要,因此最终用户不会感到困惑。
Example
例
from Tkinter import *
root= Tk()
root.title("My First GUI")
root.geometry("800x200")
frame1=Frame(root)
frame1.grid()
label1 = Label(frame1, text = "Here is a label!")
label1.grid()
root.mainloop()
Output
输出量
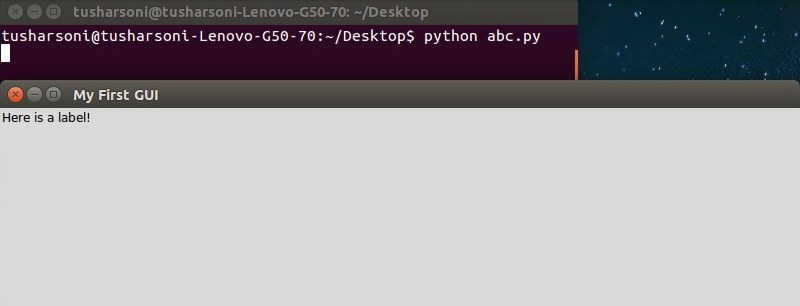
Here, we have first created a Frame which is assigned to variable frame1. We have then passed frame1 to the label1 object’s constructor, and thereby the frame that app refers to the master of the label widget. As a result, the label is placed in the frame.
在这里,我们首先创建了一个分配给变量frame1的框架。 然后,我们将frame1传递给了label1对象的构造函数,从而使应用程序引用标签小部件的母版的框架。 结果,标签被放置在框架中。
In this next Python GUI Programming tutorial we will discuss about more GUI elements or widgets.
在下一个Python GUI编程教程中,我们将讨论更多的GUI元素或小部件。
翻译自: https://www.thecrazyprogrammer.com/2015/08/python-gui-programming-tkinter-part-i.html