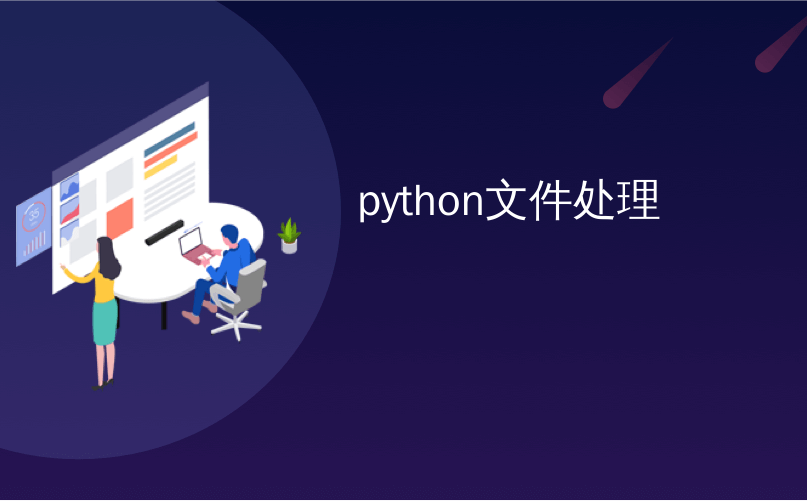
python文件处理
In this tutorial we will discuss about Python file handling concepts. The tutorial will include opening file, closing file, reading from and writing to file.
在本教程中,我们将讨论Python文件处理的概念。 本教程将包括打开文件,关闭文件,读取文件和写入文件。
Text files are a great way to store information. The normal text files stores data in the least possible memory size, generally in Kilobytes. Text Files are extremely easy to create and use. Moreover, it has cross-platform feature. Whether you create a text file in Windows or Linux, it can be transferred to another Operating System without any issue with the extension. Morever, every OS provides a basic tool to work around with basic text files.
文本文件是存储信息的好方法。 普通文本文件以最小的内存大小(通常以千字节为单位)存储数据。 文本文件非常易于创建和使用。 而且,它具有跨平台功能。 无论您是在Windows还是Linux中创建文本文件,都可以将其传输到另一个操作系统,而扩展名没有任何问题。 此外,每个操作系统都提供了一个基本工具来处理基本文本文件。
File reading is an important concept in programming. Here, we are going to give you more information about Python file handling concepts.
文件读取是编程中的重要概念。 在这里,我们将为您提供有关Python文件处理概念的更多信息。
Python文件处理 (Python File Handling)
打开和关闭文件 (Opening and Closing a File)
Syntax
句法
variable=open("File Name","Access Mode")
variable.close()
To access a file, we need to open it into the system. The open() method is used to open the file into the system for reading or writing or both. The close() method is used to close the opened file.
要访问文件,我们需要将其打开到系统中。 open()方法用于将文件打开到系统中以进行读取或写入,或同时进行这两者。 close()方法用于关闭打开的文件。
The open() function takes in two parameters: 1. File Name 2. Access Mode (Reading/Writing/Both)
open()函数采用两个参数: 1.文件名 2.访问模式(读/写/两者)
Example
例
var1=open("textset.txt","r")
var1.close()
Here, we have created a variable var1 which is assigned a value. The open() method is assigned to var1. We have taken a file named textset.txt that has a .txt extension. The mode of opening is read mode. As soon as we’re done with the file, we must close it to save the memory consumed by it.
在这里,我们创建了一个变量var1,并为其分配了一个值。 open()方法已分配给var1。 我们采用了一个扩展名为.txt的名为textset.txt的文件。 打开模式为读取模式。 处理完文件后,必须立即将其关闭以节省文件消耗的内存。
存取模式 (Access Modes)
“r” : This mode is used to read from a file. Python would display an error if the file doesn’t exist.
“ r”:此模式用于读取文件。 如果该文件不存在,Python将显示错误。
“w”: This mode is used to write to a file. The file contents are over-written by the new contents if the file exists, otherwise it will be newly created.
“ w”:此模式用于写入文件。 如果文件存在,则文件内容会被新内容覆盖,否则它将被重新创建。
“a”: This mode is used to append to a file. The data is appended or added into the file if it exists otherwise its newly created.
“ a”:此模式用于附加到文件。 如果数据存在,则将数据追加或添加到文件中;否则,将新创建数据。
“r+”: This mode is used to read from and write to a file. Python would display an error if the file doesn’t exist.
“ r +”:此模式用于读取和写入文件。 如果该文件不存在,Python将显示错误。
“w+”: This mode is used to write to and read from a file. The contents are overwritten if the file exists, if the file doesn’t exist, it is created.
“ w +”:此模式用于写入和读取文件。 如果文件存在,则内容将被覆盖;如果文件不存在,则将创建它。
“a+”: This mode is used to append first and then read from a file. The New data is appended to it if the file is already created. If the file doesn’t exist, it is created.
“ a +”:此模式用于首先附加,然后从文件读取。 如果已创建文件,则将新数据附加到该文件。 如果文件不存在,则创建它。
从文件读取 (Reading from File)
Example
例
# -*- coding: utf-8 -*-
var=open("/home/tusharsoni/Desktop/textset.txt","r")
a=var.read()
print(a)
var.close()
Output
输出量
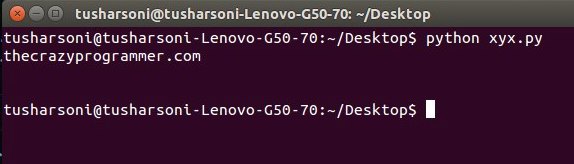
Note: The first line plays a very important role without which your code sometimes won’t work. You must use this statement if you are trying to use UTF-8 Characters.
注意:第一行起着非常重要的作用,没有它,您的代码有时将无法工作。 如果尝试使用UTF-8字符,则必须使用此语句。
The second line opens the file into the System. The open() method takes in two parameters. The first is the File Name or the complete location of the file to be accessed which is optional. Here, the file that we are reading data from is textset.txt. The next is the mode. Then, we use the read() function which actually takes in all the text written into the text file. After taking the value read by read() function in a, we print the variable a onto the console. After we are done with the file, we need to close the file using the close() method as if we don’t do it, it will remain in the memory consuming important space.
第二行将文件打开到系统中。 open()方法采用两个参数。 第一个是文件名或要访问的文件的完整位置,这是可选的。 在这里,我们从中读取数据的文件是textset.txt。 接下来是模式。 然后,我们使用read()函数,该函数实际上接收所有写入文本文件的文本。 在使用a中的read()函数读取的值之后,我们将变量a打印到控制台上。 处理完文件后,我们需要使用close()方法关闭文件,就好像我们不这样做一样,它将保留在占用内存的重要空间中。
从文件读取字符 (Read a Character from File)
Syntax
句法
print textset.readline(1)
The above function readline() is used to read a specific character at a specific location and then print it onto the console. The readline() function takes in a single parameter that specifies the number of characters that are to be read.
上面的函数readline()用于读取特定位置的特定字符,然后将其打印到控制台上。 readline()函数采用单个参数,该参数指定要读取的字符数。
Example
例
# -*- coding: utf-8 -*-
var=open("/home/tusharsoni/Desktop/textset.txt","r")
print var.readline(1)
var.close()
Output
输出量
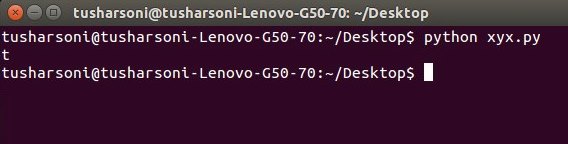
从文件中读取一行 (Read a Single Line from File)
Syntax
句法
print textset.readline()
Example
例
# -*- coding: utf-8 -*-
var=open("/home/tusharsoni/Desktop/textset.txt","r")
print var.readline()
var.close()
The above program reads a text file line by line. So executing the above program will give you the output of the first line on the console.
上面的程序逐行读取一个文本文件。 因此,执行上述程序将为您提供控制台第一行的输出。
写入文件 (Writing to File)
We can write into a file using write mode. For this, we need to use the access mode as ‘w’ and also use the write() method. All the content of the file will be erased if it exists previously or else new file will be created.
我们可以使用写入模式写入文件。 为此,我们需要将访问模式用作“ w”,还需要使用write()方法。 如果该文件先前存在,则将删除该文件的所有内容,否则将创建新文件。
Example
例
# -*- coding: utf-8 -*-
var=open("/home/tusharsoni/Desktop/textset.txt","w")
var.write("This is thecrazyprogrammer.com")
var.close()
The write() method do not add new line character in the end of the string.
write()方法不会在字符串末尾添加换行符。
Tell()方法 (Tell() Method)
This method returns the current location or position of the read/write pointer within the file. This method doesn’t require any parameter to be passed in it.
此方法返回文件中读/写指针的当前位置或位置。 此方法不需要在其中传递任何参数。
Syntax
句法
FileVariableName.tell()
Example
例
# -*- coding: utf-8 -*-
var=open("/home/tusharsoni/Desktop/textset.txt","r")
print var.readline()
fp = var.tell()
print(fp)
var.close()
Output
输出量
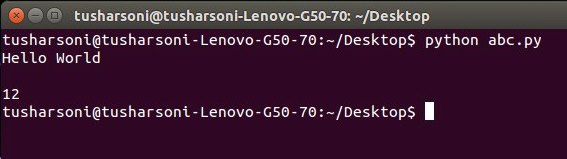
Seek()方法 (Seek() Method)
This method is used to set the file pointer to a particular position. Suppose, you want to goto the 5th character and start the printing after the 5th character, then seek() method is useful.
此方法用于将文件指针设置为特定位置。 假设您要转到第5个字符并在第5个字符之后开始打印,那么seek()方法很有用。
This method takes in two parameters.
此方法采用两个参数。
First is the position which takes in the position at where you need to set the file pointer.
首先是需要设置文件指针的位置。
The second parameter is the source parameter which is an optional one. It accepts only one of the three values mentioned below:
第二个参数是源参数,它是一个可选参数。 它仅接受以下三个值之一:
0: This is the default value and indicates absolute file position. 1: This value provides relative seeking to the current file pointer location. 2: This value indicates relative seeking to the File’s ending character.
0:这是默认值,表示文件的绝对位置。 1:此值提供相对于当前文件指针位置的相对查找。 2:该值表示相对于文件结尾字符的查找。
Syntax
句法
FileVariableName.seek(position,source)
Example
例
# -*- coding: utf-8 -*-
var=open("/home/tusharsoni/Desktop/textset.txt","r")
var.seek(5)
fp = var.readline()
print(fp)
var.close()
Output
输出量
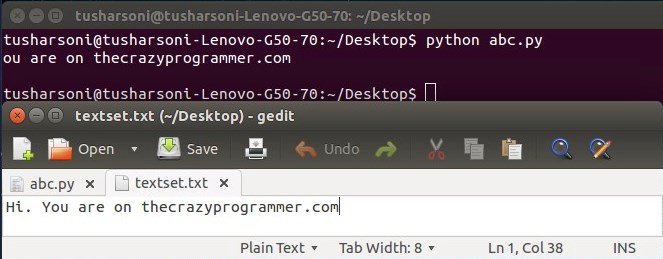
Comment below if you find anything incorrect or have doubts regarding above Python file handling tutorial.
如果您发现任何错误或对上面的Python文件处理教程有疑问,请在下面评论。
翻译自: https://www.thecrazyprogrammer.com/2015/08/python-file-handling.html
python文件处理