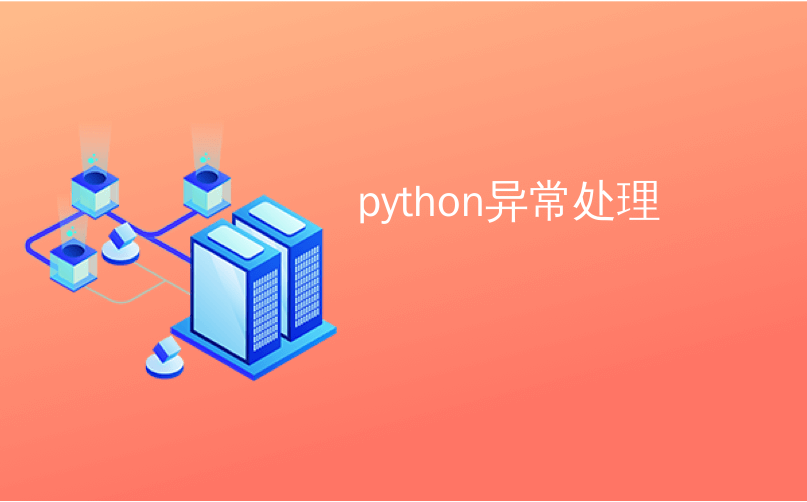
python异常处理
In this tutorial you will learn about Python exception handling, try, catch and finally block.
在本教程中,您将学习有关Python异常处理,尝试,捕获和最终阻止的知识。
Program code is developed by humans and therefore it is about to be wrong sometimes. It may happen that our code consists of errors such as run-time error, syntax error, semantic errors and many others. It is important for Python to block the execution of a program and raise error messages. This is what is known as exceptions.
程序代码是人类开发的,因此有时会出错。 我们的代码可能包含错误,例如运行时错误,语法错误,语义错误和许多其他错误。 对于Python来说,阻止程序执行并引发错误消息很重要。 这就是所谓的异常。
Python异常处理 (Python Exception Handling)
An exception is an undesirable event which arises during the execution of a program that disrupts the normal flow of the program’s instructions.
异常是在程序执行期间发生的不希望发生的事件,它破坏了程序指令的正常流程。
To handle these errors or exceptions, we use the technique of Exception Handling.
为了处理这些错误或异常,我们使用异常处理技术。
Exceptions are normally caused due to the following events:
异常通常是由于以下事件引起的:
1. A File that is to be opened but is not found in the memory. 2. Invalid entry of data by the user.
1.一个要打开但在内存中找不到的文件。 2.用户无效的数据输入。
Example
例
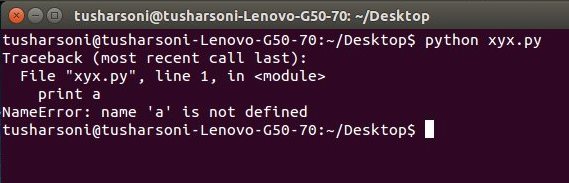
This is an example of Python error. The benefit with Python is that it provides us well details error messages.
这是Python错误的示例。 Python的好处在于,它可以为我们提供详细的错误消息。
In the above program, it tells me the error line number and the error line code. Morever, it also tells me if I have forgotten anything. Like, in the above program I have missed to declare variable a and directly printing variable onto the console.
在上面的程序中,它告诉我错误行号和错误行代码。 而且,它还告诉我是否忘记了什么。 就像在上面的程序中,我错过了声明变量a并直接将变量打印到控制台上的过程。
With the help of Python exception handling technique, we can avoid abrupt termination of a program and handle interruptions and errors and prevent the program from closing abruptly.
借助Python异常处理技术,我们可以避免程序突然终止,并处理中断和错误,并防止程序突然关闭。
While making your program code, if you think that a certain part of your program code may not work properly, then on execution it may terminate abruptly and your system may crash. To prevent all of this, you may add an exception block in your code so that if an error occurs, Python Interpreter will catch that exception and prevent your program from crashing.
在编写程序代码时,如果您认为程序代码的某些部分可能无法正常工作,则在执行时它可能会突然终止,并且系统可能会崩溃。 为了防止所有这些,您可以在代码中添加一个异常块,以便在发生错误时,Python Interpreter会捕获该异常并防止程序崩溃。
Python标准异常 (Python Standard Exceptions)
There are some pre-defined or standard exceptions in the Python library. So, you can use one of them if your needs are sufficed. These exceptions are as follow:
Python库中有一些预定义或标准例外。 因此,如果您的需求足够,您可以使用其中之一。 这些例外如下:
IOError: It is raised when an I/O operation fails to execute such as when an attempt is made to open a file in read mode that does not exist.
IOError:当I / O操作执行失败(例如,尝试以不存在的读取模式打开文件)时,引发此错误。
IndexError: This error is raised when a sequence is indexed with a number of an element that does not exist.
IndexError:使用不存在的多个元素索引序列时,会引发此错误。
KeyError: This error is raised when a dictionary key is not found.
KeyError:当找不到字典键时会引发此错误。
NameError: It is raised when a name of an identifier such as a variable or a function is not found.
NameError:当未找到标识符(例如变量或函数)的名称时引发。
SyntaxError: It is raised when a syntax error occurs.
SyntaxError:发生语法错误时引发。
TypeError: It is raised when a built-in operation or function is applied to an object of inappropriate datatype.
TypeError:将内置操作或函数应用于不合适的数据类型的对象时引发。
ValueError: It occurs when a built-in operation or a function receives an argument that has the right type but an inappropriate value.
ValueError:当内置操作或函数接收到类型正确但值不合适的参数时发生。
ZeroDivisionError: It is raised when the second argument of a division or modulo operation is zero.
ZeroDivisionError:当除法或模运算的第二个参数为零时引发。
Python尝试除块 (Python try except Block)
The standard way to handle exceptions is by including a try and except block in your program code. In the try block, you can write a section of code that could probably raise an error. The except block is then written so that if your exception comes true, the control of the program will be passed to the except block and you could thus prevent program from abnormal termination.
处理异常的标准方法是在程序代码中包含try和except块。 在try块中,您可以编写一段可能会引发错误的代码。 然后,将写入except块,以便如果您的异常实现,程序的控制权将传递给except块,从而可以防止程序异常终止。
Syntax
句法
try:
statement 1
statement 2
statement n
except:
statement 1
statement 2
statement n
Example
例
try:
var1=float(raw_input("Enter a Number:\n"))
print("\n")
except:
print("Erorr Executing")
Output
输出量
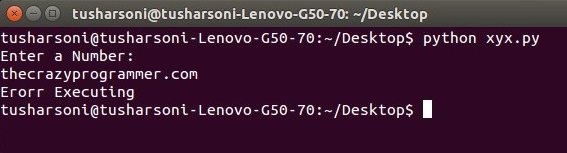
Here, I have declared a variable to take in float value through raw_input() function. I have included this code into try block so that if an error occurs I could transfer it into exception block and can then handle the program by giving an error message.
在这里,我声明了一个变量,以通过raw_input()函数获取浮点值。 我已经将此代码包含在try块中,以便如果发生错误,我可以将其转移到异常块中,然后可以通过给出错误消息来处理程序。
I have entered string value “thecrazyprogrammer.com” whereas the interpreter expects me to enter a float value, this arises an exception. On exception, the control is transferred to the except block and the print statement is executed.
我已经输入了字符串值“ thecrazyprogrammer.com”,而解释器希望我输入一个浮点值,这是一个例外。 在例外情况下,控制权转移到except块,并执行print语句。
多重异常块 (Multiple Exception Block)
You can also include multiple exception blocks with single try block which helps the Python interpreter to specify exactly what the error is.
您还可以在单个try块中包含多个异常块,这有助于Python解释器准确指定错误是什么。
Example
例
try:
var1=float(raw_input("Enter a Number:\n"))
print("\n")
except(SyntaxError):
print("Syntax Error Occured\n")
except(TypeError):
print("Invalid Datatype")
except(ValueError):
print("Invalid Value")
Output
输出量
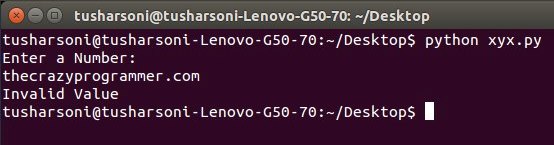
Here, I have entered an invalid value as “thecrazyprogrammer.com” which is wrong as the program expects a float value and hence the control is transferred to the correct exception block which is ValueError.
在这里,我输入了一个无效的值“ thecrazyprogrammer.com”,这是错误的,因为程序期望一个浮点值,因此控件被转移到了正确的异常块ValueError。
Python终于块了 (Python finally Block)
A finally block is very useful in Python exception handling. A finally clause always gets executed as soon the control completes the try block. It doesn’t matter whether an exception has occurred or not.
在Python异常处理中,finally块非常有用。 控件完成try块后,将始终执行finally子句。 是否发生异常都无关紧要。
Syntax
句法
finally():
statement 1
statement n
Example
例
try:
var1=float(raw_input("Enter a Number:\n"))
print("\n")
except:
print("Erorr Executing\n")
finally:
print("We are in finally block")
Output
输出量
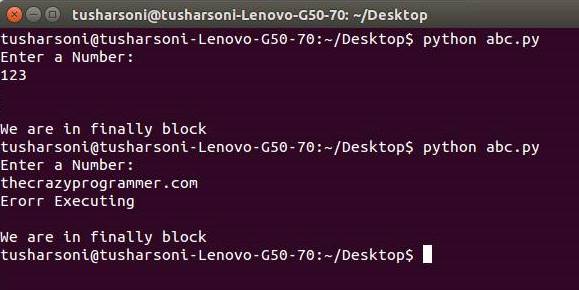
In the above example, the prompt asks the user to enter an integer. After an integer is entered, it checks in for exception and since the input is correct it directly goes to the finally block.
在上面的示例中,提示要求用户输入一个整数。 输入整数后,它会检查是否有异常,并且由于输入正确,因此直接进入finally块。
Again we try to run the same program and now enter a wrong input i.e., string. This time an exception has been occurred and now the control will go to the exception block and as soon as the exception block execution gets over, the control will move into finally block and then terminate or go to the next sequence of statements whichever occurs first.
再次,我们尝试运行相同的程序,现在输入错误的输入,即字符串。 这次发生了异常,现在控件将转到异常块,并且一旦异常块执行结束,该控件将移至finally块,然后终止或转到下一个语句序列,以先到者为准。
If you find anything incorrect or have any doubts regarding above Python exception handling tutorial then please comment below.
如果您对上述Python异常处理教程有任何不正确的地方或有疑问,请在下面发表评论。
翻译自: https://www.thecrazyprogrammer.com/2015/08/python-exception-handling.html
python异常处理