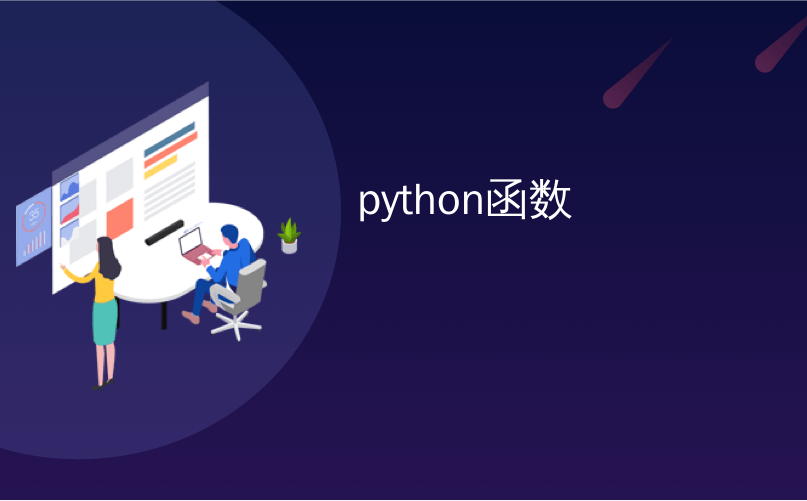
python函数
In this tutorial you will learn about Python function.
在本教程中,您将学习Python函数。
Functions are a great way to support programs that consists of many lines of code. They go off and perform a task and then return control to your program.
函数是支持包含多行代码的程序的一种好方法。 他们开始执行任务,然后将控制权返回给您的程序。
Creating your own functions offers you many advantages and features. It allows you to break up your code into manageable and small chunks. Some python programs that are long series of instructions with no logical breaks are hard to maintain understand and write. Functions, if included in a program can help the programmers to easily develop it and maintain it properly.
创建自己的功能可为您提供许多优点和功能。 它使您可以将代码分解为可管理的小块。 有些python程序的指令很长,没有逻辑中断,很难维护和理解。 函数(如果包含在程序中)可以帮助程序员轻松地开发并正确维护它。
Python provides built-in functions such as print(), range(), len() and many more. However, if you need to develop your own functions, it is quite possible to create it in Python like in other programming environments, also known as user-defined functions.
Python提供了内置函数,例如print(),range(),len()等。 但是,如果您需要开发自己的函数,则很有可能像在其他编程环境中一样在Python中创建它,也称为用户定义函数。
Python函数定义 (Python Function Definition)
Syntax
句法
def function_name():
statement 1
statement 2
statement n
Example
例
def addition():
This statement would tell the python interpreter that a function named addition is being defined at the prompt and following this would be the actual function block definition.
该语句将告诉python解释器,正在提示符处定义了一个名为additioning的函数,其后将是实际的功能块定义。
Note: It is important to note that the indentations are required to be proper because if it is not then the Python interpreter would not be able to identify the scope or body of the function and hence it will raise an error.
注意:需要注意的是,缩进必须正确,因为如果不是这样,则Python解释器将无法识别函数的范围或主体,因此将引发错误。
Python函数调用 ( Python Function Calling)
没有参数 (Without Parameters)
Syntax
句法
Function_Name()
Example
例
addition()
In case you don’t want to pass any value to a user defined function, you can directly write the name of the function and the control will go to the function definition, execute the statements within the function and the moment all the statements have been executed by the interpreter, the control will come out the function and will return to the next line where the calling of function was done.
如果您不想将任何值传递给用户定义的函数,则可以直接编写函数的名称,控件将转到函数定义,在函数中执行语句,并在所有语句被执行后立即执行由解释器执行后,控件将出现该函数,并返回到完成该函数调用的下一行。
带参数 (With Parameters)
Syntax
句法
Function_Name(Parameter 1.., Parameter n)
Example
例
addition(var1,var2)
Suppose you want to pass a value to the function, you can directly write it in the function name during the calling of a function and it will work for you. For multiple values passing, you can separate it by including a Comma (,).
假设您要向函数传递值,则可以在调用函数期间直接在函数名称中写入它,它将对您有用。 对于多个值传递,可以通过包含逗号(,)来将其分隔。
Example
例
def addition(var1,var2): #Defining the Function
var3=var1+var2
print("You are in Addition Function")
print(var3)
var1=5
var2=4
addition(var1,var2) #Calling the Function
print("You are back to Main Function")
Output
输出量
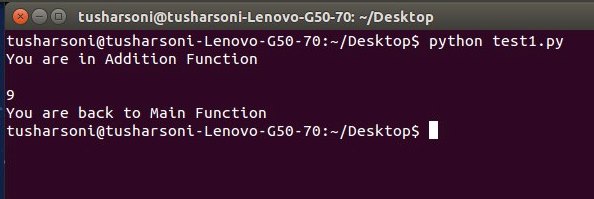
In this program, we have pre-declared the user-defined function i.e., before the actual main function. We have declared an addition() that takes in two parameters. A new variable is declared that stores in the addition of var1 and var2.
在此程序中,我们已经预先声明了用户定义的功能,即在实际的主要功能之前。 我们已经声明了一个带有两个参数的additional()。 声明了一个新变量,该变量存储了var1和var2的附加内容。
When the program is interpreted, it first checks in the value of var1 and var2. Then, it encounters the addition(var1,var2) statement which then takes the control of the program to def addition(var1,var2) and hence the following three statements are executed by the Python interpreter. After print(var3), control comes back to calling function and the remaining statement print(“You are back to Main function”) is executed.
解释程序后,它将首先检入var1和var2的值。 然后,它遇到加法(var1,var2)语句,然后该语句控制程序以定义加法(var1,var2),因此以下三个语句由Python解释器执行。 在print(var3)之后,控制权返回到调用函数,并执行剩余的语句print(“您返回主函数”)。
传递默认值 (Passing Default Values)
def addition(var1,var2): #Defining the Function
var3=var1+var2
print("You are in Addition Function")
print(var3)
var1=5
var2=4
addition(var1=7,var2=10)
print("You are back to Main Function")
Output
输出量
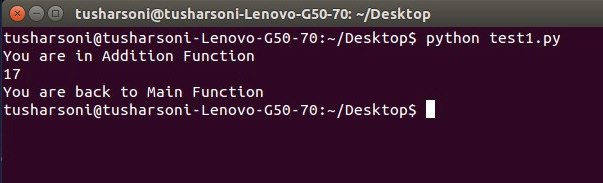
Here, we have used passing default values which override the previous values that have been assigned to variables var1 and var2. The values that var1 consist of previously will be overwritten by 7 and that of var2 by 10 and the same values will be passed into the user defined function.
在这里,我们使用了传递的默认值,这些默认值将覆盖已分配给变量var1和var2的先前值。 以前由var1组成的值将被7覆盖,而由var2组成的值将被10覆盖,并且相同的值将被传递到用户定义的函数中。
Python返回语句 ( Python return Statement)
The return statement helps to return a value from the function to the calling function.
return语句有助于将值从函数返回到调用函数。
Example
例
def addition(var1,var2): #Defining the Function
var3=var1+var2
print("You are in Addition Function")
return var3 #return statement
var1=5
var2=4
var3=addition(var1=7,var2=10)
print("This is return Function addition():",var3)
Output
输出量
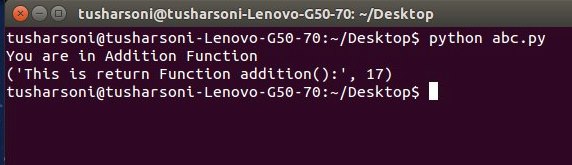
In the above example, we have created an addition function which after executing the statement var3=var1+var2 stores the value in the variable var3 and returns this value into whatever function has called it.
在上面的示例中,我们创建了一个加法函数,该函数在执行语句var3 = var1 + var2之后将值存储在变量var3中,并将该值返回到任何调用它的函数中。
翻译自: https://www.thecrazyprogrammer.com/2015/08/python-function.html
python函数