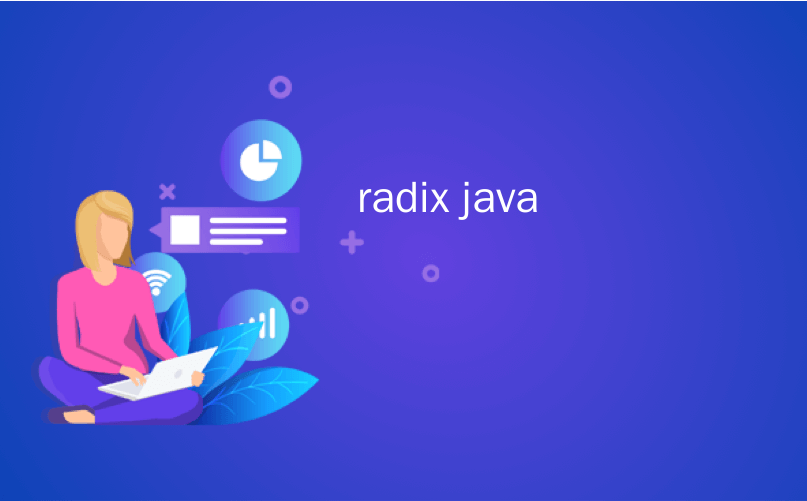
radix java
This tutorial is about radix sort java program and algorithm.
本教程是关于基数排序的Java程序和算法。
Here is a very basic and detailed description of algorithm that we follow to implement the radix sort in java. We mostly rely on the place value of digits to sort it out in the given list. So, our first basic task is to find out the number with maximum length and then iterate the whole loop over the length of the digit.
这是我们在Java中实现基数排序所遵循的算法的非常基本且详细的描述。 我们主要依靠数字的位置值在给定列表中进行排序。 因此,我们的第一个基本任务是找出最大长度的数字,然后在数字长度上迭代整个循环。
Next we arrange every number considering 1s place value, 10s place value, 100s place value and so on till the length of the maximum digit.
接下来,我们将每个数字考虑到1s,10s,100s等,直到最大位数的长度。
基数Java排序算法 (Radix Sort Java Algorithm)
1. Find the length of the number that has maximum number of digits. 2. Initialize i=0, Repeat the below procedure till the length equals i. 3. Fill the bucket with all the digits in ith position. 4. Sort out the digits according to the order. 5. If length=i, i=i*10, goto to step 3. Else go to step 5 6. Print the sorted array.
1.查找具有最大位数的数字的长度。 2.初始化i = 0,重复以下步骤,直到长度等于i。 3.将所有数字填入第i个位置的存储桶中。 4.根据顺序对数字进行排序。 5.如果length = i,i = i * 10,转到步骤3。否则转到步骤5。6.打印排序的数组。
复杂 (Complexity)
The complexity of Radix Sort is far better than that of bubble sort and some other sorting techniques. It is as shown below depends on d and b.
Radix Sort的复杂性远优于气泡排序和其他一些排序技术。 如下所示取决于d和b。
O (d*(n+b)) d is digits in input integers. b is the base for representing numbers.
O(d *(n + b))d是输入整数中的数字。 b是代表数字的基础。
说明 (Explanation)
Let us start the implementation of the program. First we define a class named RadixSort and obviously it has only one method named sort to sort the numbers given. This function is the central part of discussion and as always, we take the inputs for the user using the main function and pass it on to the sorting function. The below code snippet shows it.
让我们开始执行程序。 首先,我们定义一个名为RadixSort的类,并且显然只有一个名为sort的方法可以对给定的数字进行排序。 此功能是讨论的中心部分,并且一如既往,我们使用主要功能为用户提供输入并将其传递给排序功能。 下面的代码片段显示了它。
Scanner scan = new Scanner( System.in );
System.out.println("Radix Sort Test\n");
int n, i;
System.out.println("Enter number of integer elements");
n = scan.nextInt();
int arr[] = new int[ n ];
System.out.println("\nEnter "+ \n +" integer elements");
for (i = 0; i < n; i++)
arr[i] = scan.nextInt();
sort(arr);
You can observe that the function that we are going to implement doesn’t return anything as its return type is void. It takes in an unsorted array of numbers and sorts it. Now we will have a look at the algorithm to implement the sort function. The algorithm says we need to first find the number of digits in the longest number.
您可以观察到我们将要实现的函数不会返回任何内容,因为其返回类型为void。 它接受未排序的数字数组并将其排序。 现在我们来看看实现排序功能的算法。 该算法表示,我们需要首先找到最长的数字位数。
for (i = 1; i < n; i++)
if (a[i] > m)
m = a[i];
Using the above for loop we can find the maximum of all the numbers. Instead of finding its length we will iterate a while loop until the maximum number falls below zero by dividing the number by 10 for every iteration. Then sort the array using a temporary bucket and array b[ ] and place back the final contents.
使用上面的for循环,我们可以找到所有数字的最大值。 通过查找每次迭代,将其除以10,而不是找到其长度,我们将迭代while循环直到最大数降至零以下。 然后使用临时存储区对数组进行排序,并将数组b []放回原处。
while (m / exp > 0)
{
int[] bucket = new int[10];
for (i = 0; i < n; i++)
bucket[(a[i] / exp) % 10]++;
for (i = 1; i < 10; i++)
bucket[i] += bucket[i - 1];
for (i = n - 1; i >= 0; i--)
b[--bucket[(a[i] / exp) % 10]] = a[i];
for (i = 0; i < n; i++)
a[i] = b[i];
exp *= 10;
}
基数排序Java程序 (Radix Sort Java Program)
import java.util.Scanner;
class RadixSort
{
static void sort( int[] a)
{
int i, m = a[0], exp = 1, n = a.length;
int[] b = new int[10];
for (i = 1; i < n; i++)
if (a[i] > m)
m = a[i];
while (m / exp > 0)
{
int[] bucket = new int[10];
for (i = 0; i < n; i++)
bucket[(a[i] / exp) % 10]++;
for (i = 1; i < 10; i++)
bucket[i] += bucket[i - 1];
for (i = n - 1; i >= 0; i--)
b[--bucket[(a[i] / exp) % 10]] = a[i];
for (i = 0; i < n; i++)
a[i] = b[i];
exp *= 10;
}
}
public static void main(String[] args)
{
Scanner scan = new Scanner( System.in );
System.out.println("Radix Sort Testn");
int n, i;
System.out.println("Enter number of integer elements");
n = scan.nextInt();
int arr[] = new int[ n ];
System.out.println("\nEnter "+ n +" integer elements");
for (i = 0; i < n; i++)
arr[i] = scan.nextInt();
sort(arr);
System.out.println("\nElements after sorting ");
for (i = 0; i < n; i++)
System.out.print(arr[i]+" ");
System.out.println();
}
}
Output
输出量
Radix Sort Test
基数排序测试
Enter number of integer elements 6
输入整数元素的数量 6
Enter 6 integer elements 12 9 15 4 0 1
输入6个整数元素 12 9 15 4 0 1
Elements after sorting 0 1 4 9 12 15
排序后的元素 0 1 4 9 12 15
Code Reference: https://www.sanfoundry.com/java-program-implement-radix-sort/
代码参考: https : //www.sanfoundry.com/java-program-implement-radix-sort/
Comment below if you have queries or found anything incorrect in above radix sort java program and algorithm.
如果您有疑问或在上述基数排序Java程序和算法中发现任何不正确的内容,请在下面评论。
翻译自: https://www.thecrazyprogrammer.com/2015/06/radix-sort-java-program-and-algorithm.html
radix java