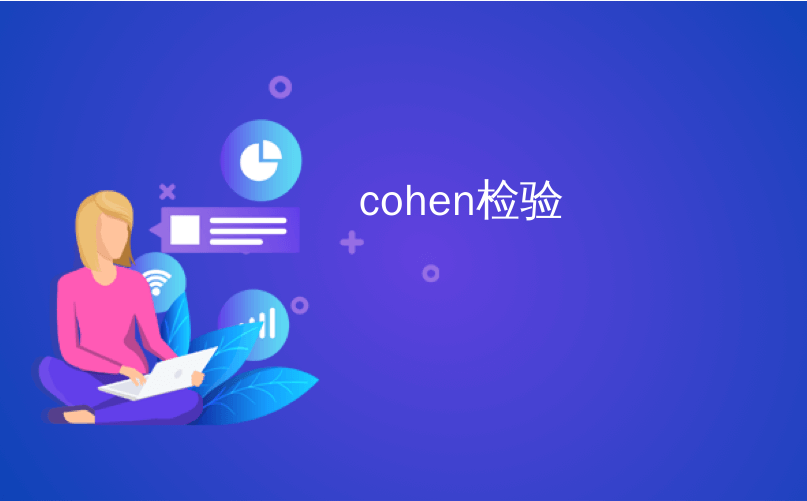
cohen检验
Here you will learn about cohen sutherland line clipping algorithm in C and C++.
在这里,您将了解C和C ++中的科恩·萨瑟兰行裁剪算法。
This is one of the oldest and most popular line clipping algorithm. To speed up the process this algorithm performs initial tests that reduce number of intersections that must be calculated. It does so by using a 4 bit code called as region code or outcodes. These codes identify location of the end point of line.
这是最古老和最受欢迎的换行算法之一。 为了加快处理速度,该算法执行了初始测试,以减少必须计算的交叉点数量。 它通过使用称为区域代码或外码的4位代码来实现。 这些代码标识直线终点的位置。
Each bit position indicates a direction, starting from the rightmost position of each bit indicates left, right, bottom, top respectively.
每个比特位置指示一个方向,从每个比特的最右位置开始分别指示左,右,下,上。
Once we establish region codes for both the endpoints of a line we determine whether the endpoint is visible, partially visible or invisible with the help of ANDing of the region codes.
一旦我们为一条线的两个端点建立了区域代码,就可以借助区域代码的AND运算来确定该端点是可见的,部分可见的还是不可见的。
There arises 3 cases which are explained in the algorithm below in step 4.
出现3种情况,以下步骤4中的算法对此进行了说明。
Also Read: Liang Barsky Line Clipping Algorithm in C and C++
另请阅读: C和C ++中的Liang Barsky线剪切算法
算法 (Algorithm)
1. Read 2 end points of line as p1(x1,y1) and p2(x2,y2)
1.将线的2个端点读取为p1(x1,y1)和p2(x2,y2)
2. Read 2 corner points of the clipping window (left-top and right-bottom) as (wx1,wy1) and (wx2,wy2)
2.读取裁剪窗口的两个角点(左上和右下)为(wx1,wy1)和(wx2,wy2)
3. Assign the region codes for 2 endpoints p1 and p2 using following steps:-
3.使用以下步骤为2个端点p1和p2分配区域代码:
initialize code with 0000
用0000初始化代码
Set bit 1 if x<wx1
如果x <wx1则设置位1
Set bit 2 if x>wx2
如果x> wx2则设置位2
Set bit 3 if y<wy2
如果y <wy2,则设置位3
Set bit 4 if y>wy1
如果y> wy1,则设置位4
4. Check for visibility of line
4.检查线的可见性
- If region codes for both endpoints are zero then line is completely visible. Draw the line go to step 9. 如果两个端点的区域代码均为零,则该线完全可见。 画线,请转到步骤9。
- If region codes for endpoints are not zero and logical ANDing of them is also nonzero then line is invisible. Discard the line and move to step 9. 如果端点的区域代码不为零,并且它们的逻辑与也不为零,则该行不可见。 丢弃该行,然后转到步骤9。
- If it does not satisfy 4.a and 4.b then line is partially visible. 如果不满足4.a和4.b,则该线是部分可见的。
5. Determine the intersecting edge of clipping window as follows:-
5.确定裁剪窗口的相交边缘,如下所示:
- If region codes for both endpoints are nonzero find intersection points p1’ and p2’ with boundary edges. 如果两个端点的区域代码都不为零,则找到具有边界边的交点p1'和p2'。
- If region codes for any one end point is non zero then find intersection point p1’ or p2’. 如果任一端点的区域代码不为零,则找到交点p1'或p2'。
6. Divide the line segments considering intersection points.
6.考虑相交点划分线段。
7. Reject line segment if any end point of line appears outside of any boundary.
7.如果线的任何端点出现在任何边界之外,则拒绝线段。
8. Draw the clipped line segment.
8.绘制剪切的线段。
9. Stop.
9.停下来。
C和C ++中的Cohen Sutherland裁线算法程序 (Program for Cohen Sutherland Line Clipping Algorithm in C and C++)
C程序 (C Program)
#include<stdio.h>
#include<stdlib.h>
#include<math.h>
#include<graphics.h>
#include<dos.h>
typedef struct coordinate
{
int x,y;
char code[4];
}PT;
void drawwindow();
void drawline(PT p1,PT p2);
PT setcode(PT p);
int visibility(PT p1,PT p2);
PT resetendpt(PT p1,PT p2);
void main()
{
int gd=DETECT,v,gm;
PT p1,p2,p3,p4,ptemp;
printf("\nEnter x1 and y1\n");
scanf("%d %d",&p1.x,&p1.y);
printf("\nEnter x2 and y2\n");
scanf("%d %d",&p2.x,&p2.y);
initgraph(&gd,&gm,"c:\\turboc3\\bgi");
drawwindow();
delay(500);
drawline(p1,p2);
delay(500);
cleardevice();
delay(500);
p1=setcode(p1);
p2=setcode(p2);
v=visibility(p1,p2);
delay(500);
switch(v)
{
case 0: drawwindow();
delay(500);
drawline(p1,p2);
break;
case 1: drawwindow();
delay(500);
break;
case 2: p3=resetendpt(p1,p2);
p4=resetendpt(p2,p1);
drawwindow();
delay(500);
drawline(p3,p4);
break;
}
delay(5000);
closegraph();
}
void drawwindow()
{
line(150,100,450,100);
line(450,100,450,350);
line(450,350,150,350);
line(150,350,150,100);
}
void drawline(PT p1,PT p2)
{
line(p1.x,p1.y,p2.x,p2.y);
}
PT setcode(PT p) //for setting the 4 bit code
{
PT ptemp;
if(p.y<100)
ptemp.code[0]='1'; //Top
else
ptemp.code[0]='0';
if(p.y>350)
ptemp.code[1]='1'; //Bottom
else
ptemp.code[1]='0';
if(p.x>450)
ptemp.code[2]='1'; //Right
else
ptemp.code[2]='0';
if(p.x<150)
ptemp.code[3]='1'; //Left
else
ptemp.code[3]='0';
ptemp.x=p.x;
ptemp.y=p.y;
return(ptemp);
}
int visibility(PT p1,PT p2)
{
int i,flag=0;
for(i=0;i<4;i++)
{
if((p1.code[i]!='0') || (p2.code[i]!='0'))
flag=1;
}
if(flag==0)
return(0);
for(i=0;i<4;i++)
{
if((p1.code[i]==p2.code[i]) && (p1.code[i]=='1'))
flag='0';
}
if(flag==0)
return(1);
return(2);
}
PT resetendpt(PT p1,PT p2)
{
PT temp;
int x,y,i;
float m,k;
if(p1.code[3]=='1')
x=150;
if(p1.code[2]=='1')
x=450;
if((p1.code[3]=='1') || (p1.code[2]=='1'))
{
m=(float)(p2.y-p1.y)/(p2.x-p1.x);
k=(p1.y+(m*(x-p1.x)));
temp.y=k;
temp.x=x;
for(i=0;i<4;i++)
temp.code[i]=p1.code[i];
if(temp.y<=350 && temp.y>=100)
return (temp);
}
if(p1.code[0]=='1')
y=100;
if(p1.code[1]=='1')
y=350;
if((p1.code[0]=='1') || (p1.code[1]=='1'))
{
m=(float)(p2.y-p1.y)/(p2.x-p1.x);
k=(float)p1.x+(float)(y-p1.y)/m;
temp.x=k;
temp.y=y;
for(i=0;i<4;i++)
temp.code[i]=p1.code[i];
return(temp);
}
else
return(p1);
}
C ++程序 (C++ Program)
#include<iostream.h>
#include<stdlib.h>
#include<math.h>
#include<graphics.h>
#include<dos.h>
typedef struct coordinate
{
int x,y;
char code[4];
}PT;
void drawwindow();
void drawline(PT p1,PT p2);
PT setcode(PT p);
int visibility(PT p1,PT p2);
PT resetendpt(PT p1,PT p2);
void main()
{
int gd=DETECT,v,gm;
PT p1,p2,p3,p4,ptemp;
cout<<"\nEnter x1 and y1\n";
cin>>p1.x>>p1.y;
cout<<"\nEnter x2 and y2\n";
cin>>p2.x>>p2.y;
initgraph(&gd,&gm,"c:\\turboc3\\bgi");
drawwindow();
delay(500);
drawline(p1,p2);
delay(500);
cleardevice();
delay(500);
p1=setcode(p1);
p2=setcode(p2);
v=visibility(p1,p2);
delay(500);
switch(v)
{
case 0: drawwindow();
delay(500);
drawline(p1,p2);
break;
case 1: drawwindow();
delay(500);
break;
case 2: p3=resetendpt(p1,p2);
p4=resetendpt(p2,p1);
drawwindow();
delay(500);
drawline(p3,p4);
break;
}
delay(5000);
closegraph();
}
void drawwindow()
{
line(150,100,450,100);
line(450,100,450,350);
line(450,350,150,350);
line(150,350,150,100);
}
void drawline(PT p1,PT p2)
{
line(p1.x,p1.y,p2.x,p2.y);
}
PT setcode(PT p) //for setting the 4 bit code
{
PT ptemp;
if(p.y<100)
ptemp.code[0]='1'; //Top
else
ptemp.code[0]='0';
if(p.y>350)
ptemp.code[1]='1'; //Bottom
else
ptemp.code[1]='0';
if(p.x>450)
ptemp.code[2]='1'; //Right
else
ptemp.code[2]='0';
if(p.x<150)
ptemp.code[3]='1'; //Left
else
ptemp.code[3]='0';
ptemp.x=p.x;
ptemp.y=p.y;
return(ptemp);
}
int visibility(PT p1,PT p2)
{
int i,flag=0;
for(i=0;i<4;i++)
{
if((p1.code[i]!='0') || (p2.code[i]!='0'))
flag=1;
}
if(flag==0)
return(0);
for(i=0;i<4;i++)
{
if((p1.code[i]==p2.code[i]) && (p1.code[i]=='1'))
flag='0';
}
if(flag==0)
return(1);
return(2);
}
PT resetendpt(PT p1,PT p2)
{
PT temp;
int x,y,i;
float m,k;
if(p1.code[3]=='1')
x=150;
if(p1.code[2]=='1')
x=450;
if((p1.code[3]=='1') || (p1.code[2]=='1'))
{
m=(float)(p2.y-p1.y)/(p2.x-p1.x);
k=(p1.y+(m*(x-p1.x)));
temp.y=k;
temp.x=x;
for(i=0;i<4;i++)
temp.code[i]=p1.code[i];
if(temp.y<=350 && temp.y>=100)
return (temp);
}
if(p1.code[0]=='1')
y=100;
if(p1.code[1]=='1')
y=350;
if((p1.code[0]=='1') || (p1.code[1]=='1'))
{
m=(float)(p2.y-p1.y)/(p2.x-p1.x);
k=(float)p1.x+(float)(y-p1.y)/m;
temp.x=k;
temp.y=y;
for(i=0;i<4;i++)
temp.code[i]=p1.code[i];
return(temp);
}
else
return(p1);
}
Output
输出量
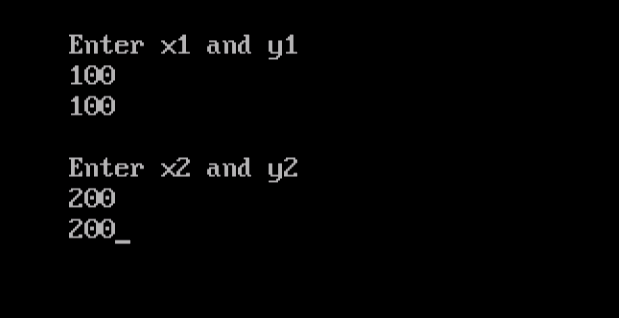
Before Clipping
剪裁前
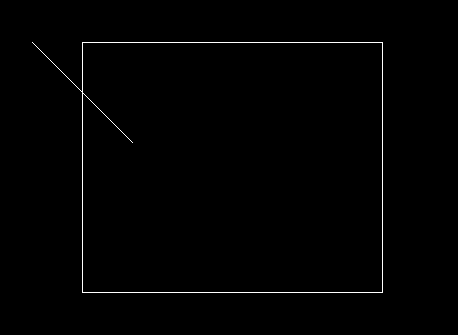
After Clipping
剪裁后
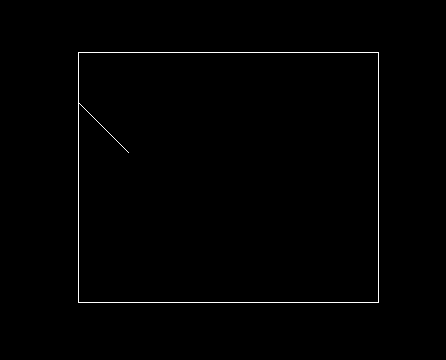
This article is submitted by Rahul Maheshwari. You can connect with him on facebook.
本文由Rahul Maheshwari提交。 您可以在Facebook上与他建立联系。
Comment below if you have doubts or found any information incorrect in above cohen sutherland line clipping algorithm in C and C++.
如果您对上面的C和C ++中的cohen sutherland行剪切算法有疑问或发现任何不正确的信息,请在下面评论。
翻译自: https://www.thecrazyprogrammer.com/2017/02/cohen-sutherland-line-clipping-algorithm.html
cohen检验