angular sass
One of the first things you'll usually do in a project is to bring in Sass to make working with CSS easier.
通常,在项目中要做的第一件事就是引入Sass来简化 CSS的工作。
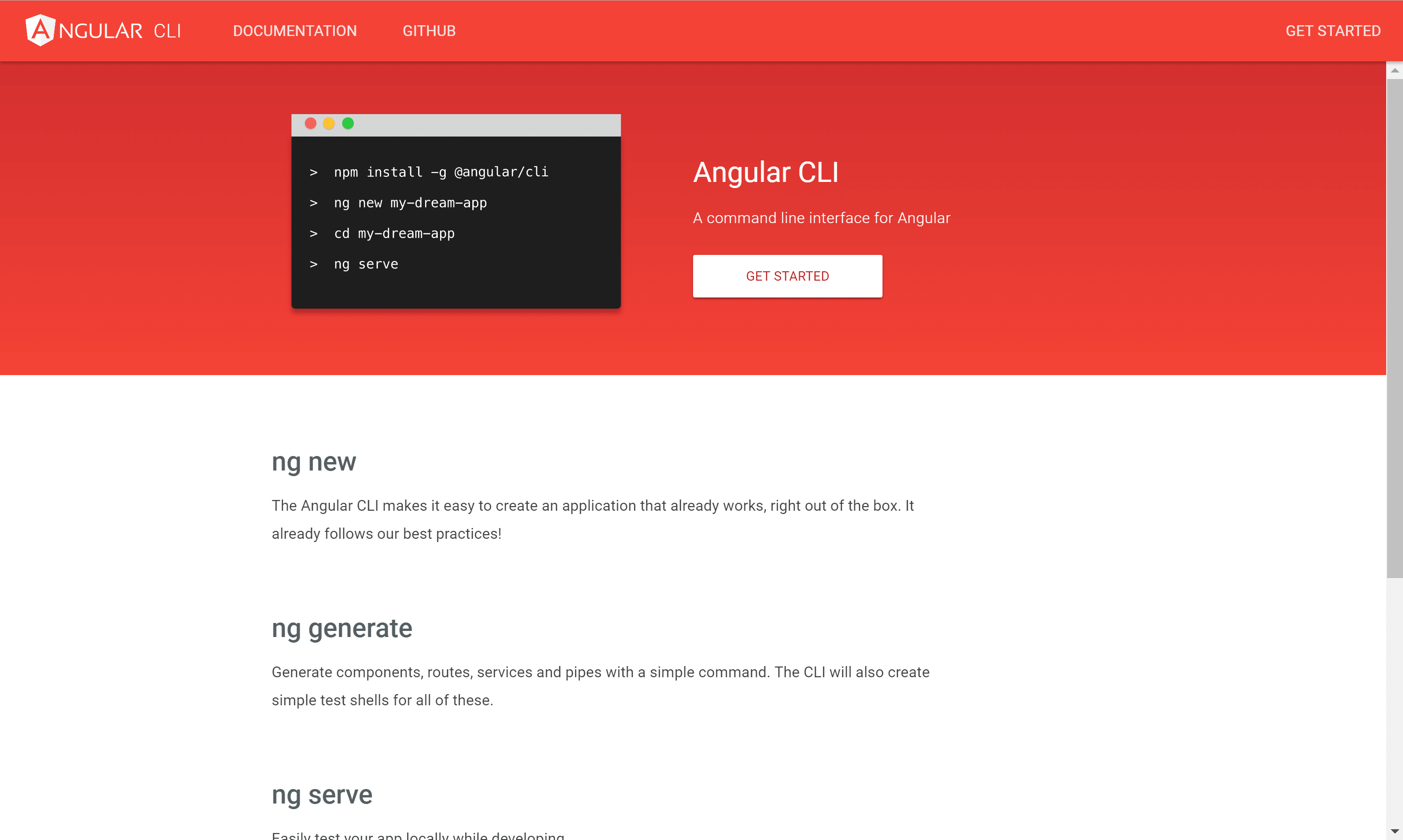
When working with the Angular CLI, the default stylesheets have the .css
extension.
使用Angular CLI时 ,默认样式表具有.css
扩展名。
Let's explore how we can easily bring in Sass to our Angular CLI projects.
让我们探讨如何轻松地将Sass引入我们的Angular CLI项目。

If you want a quick intro into the Angular CLI, check out the official docs and our Use the Angular CLI For Faster Angular v2+ Projects.
如果您想快速了解Angular CLI,请查看官方文档和我们的《 使用Angular CLI进行更快的Angular v2 +项目》 。
使用Sass启动Angular CLI项目 ( Starting an Angular CLI Project with Sass )
Normally, when we run ng new my-app
, our app will have .css
files. To get the CLI to generate .scss
files (or .sass
/.less
) is an easy matter.
通常,当我们运行ng new my-app
,我们的应用程序将包含.css
文件。 为了让CLI生成.scss
文件(或.sass
/ .less
)是一件容易的事。
Create a new project with Sass with the following:
使用以下命令用Sass创建一个新项目:
ng new my-sassy-app --style=scss
You can also set the --style
flag with the following:
您还可以使用以下设置--style
标志:
--style=scss
--style=scss
--style=sass
--style=sass
--style=less
--style=less
将当前应用程序转换为Sass ( Converting a Current App to Sass )
If you've already created your Angular CLI app with the default .css
files, it will take a bit more work to convert it over. You can tell Angular to start processing Sass files with the following command:
如果您已经使用默认的.css
文件创建了Angular CLI应用程序,则将需要更多的工作来进行转换。 您可以使用以下命令告诉Angular开始处理Sass文件:
ngset defaults.styleExt scss
This will go ahead and tell the Angular CLI to start processing .scss
files. If you want to peek under the hood at what this command did, check out the Angular CLI config file: .angular-cli.json
.
这将继续进行,并告诉Angular CLI开始处理.scss
文件。 如果您想.angular-cli.json
了解此命令的功能,请查看Angular CLI配置文件: .angular-cli.json
。
You'll find the new config line at the bottom of the file:
您将在文件底部找到新的配置行:
"defaults": {
"styleExt": "scss",
"component": {
}
}
将CSS文件更改为Sass (Changing the CSS Files to Sass)
The Angular CLI will start processing Sass files now. However, it doesn't go through the process of converting your already existing .css
files to .scss
files. You'll have to make the conversion manually.
Angular CLI现在将开始处理Sass文件。 但是,它不会经历将您已经存在的.css
文件转换为.scss
文件的过程。 您必须手动进行转换 。
使用Sass导入 ( Using Sass Imports )
I personally like creating Sass files for project variables and for project mixins. This way, we can bring in any variables/mixins we'll need quickly and easily.
我个人喜欢为项目变量和项目混合创建Sass文件。 这样,我们可以快速轻松地引入我们需要的任何变量/混合。
For instance, let's create a brand new CLI app:
例如,让我们创建一个全新的CLI应用程序:
ng new my-sassy-app --style=scss
Next, we'll create the following files:
接下来,我们将创建以下文件:
|- src/
|- sass/
|- _variables.scss
|- _mixins.scss
|- styles.scss
To start using these new Sass files, we'll import the _variables.scss
and _mixins.scss
into the main styles.scss
.
为了开始使用这些新的Sass文件,我们将_variables.scss
和_mixins.scss
导入到主要的styles.scss
。
// src/sass/styles.scss
@import './variables';
@import './mixins';
The last step is to update our .angular-cli.json
config to use this new src/sass/styles.scss
instead of the src/styles.scss
. In our .angular-cli.json
file, just change the following line to point to the right styles.scss
.
最后一步是更新我们的.angular-cli.json
配置,以使用此新的src/sass/styles.scss
而不是src/styles.scss
。 在我们的.angular-cli.json
文件中,只需.angular-cli.json
更改为指向正确的styles.scss
。
"styles": [
"sass/styles.scss"
],
I like separating out our Sass into its own folder because it allows us to create a more robust Sass foundation. I personally lean towards the Sass 7-1 Pattern.
我喜欢将Sass分离到自己的文件夹中,因为它使我们可以创建更强大的Sass基础。 我个人倾向于Sass 7-1模式 。
Now when we start up our app, these new Sass files will be used!
现在,当我们启动我们的应用程序时,将使用这些新的Sass文件!
将Sass文件导入角组件 ( Importing Sass Files Into Angular Components )
We have new _variables.scss
and _mixins.scss
files that we will probably want to use in our components. In other projects, you may be used to having access to your Sass variables in all locations since your Sass is compiled by a task runner.
我们有新的_variables.scss
和_mixins.scss
文件,我们可能希望在组件中使用它们。 在其他项目中,由于Sass是由任务运行程序编译的,因此您可能习惯于在所有位置访问Sass变量。
In the Angular CLI, all components are self-contained and so are their Sass files. In order to use a variable from within a component's Sass file, you'll need to import the _variables.scss
file.
在Angular CLI中,所有组件都是独立的,其Sass文件也是如此。 为了在组件的Sass文件中使用变量,您需要导入_variables.scss
文件。
One way to do this is to @import
with a relative path from the component. This may not scale if you have many nested folders or eventually move files around.
一种方法是@import
带有组件的相对路径。 如果您有许多嵌套文件夹或最终移动文件,则可能无法缩放。
The CLI provides an easy way to import Sass files using the
~
.CLI提供了一种使用
~
导入Sass文件的简便方法。
No matter what component Sass file we're in, we can do an import like so:
无论我们位于哪个组件的Sass文件中,我们都可以像这样进行导入:
// src/app/app.component.scss
@import '~sass/variables';
// now we can use those variables!
The tilde (~
) will tell Sass to look in the src/
folder and is a quick shortcut to importing Sass files.
代字号( ~
)会告诉Sass在src/
文件夹中查找,并且是导入Sass文件的快速快捷方式。
Sass包含路径 ( Sass Include Paths )
In addition to using the ~
, we can specify the includePaths
configuration when working with the CLI. To tell Sass to look in certain folders, add the config lines to .angular-cli.json
like in the app
object next to the styles
setting.
除了使用~
,我们还可以在使用CLI时指定includePaths
配置。 要告诉Sass在某些文件夹中查找,请将配置行添加到.angular-cli.json
就像在styles
设置旁边的app
对象中一样。
"styles": [
"styles.scss"
],
"stylePreprocessorOptions": {
"includePaths": [
"../node_modules/bootstrap/scss"
]
},
使用Bootstrap Sass文件 ( Using Bootstrap Sass Files )
Another scenario we'll need to do often is to import third party libraries and their Sass files.
我们经常需要做的另一种情况是导入第三方库及其Sass文件。
We'll bring in Bootstrap and see how we can import the Sass files into our project. This is good since we can pick and choose what parts of Bootstrap we want to use. We can also import the Bootstrap mixins and use them in our own projects.
我们将引入Bootstrap ,看看如何将Sass文件导入到我们的项目中。 这很好,因为我们可以选择要使用的Bootstrap部分。 我们还可以导入Bootstrap mixin并在我们自己的项目中使用它们。
To get us started, install bootstrap:
要开始使用,请安装引导程序:
npm install --save bootstrap
Note: We're using the 4.0 beta because 4.0 is built with Sass and gives the proper .scss
files.
注意:我们使用的是4.0 beta版,因为4.0是使用Sass构建的,并提供了正确的.scss
文件。
添加Bootstrap CSS文件 (Adding Bootstrap CSS File)
Now that we have Bootstrap, let's look at how we can include the basic CSS file. This is an easy process by adding the bootstrap.css
file to our .angular-cli.json
config:
现在有了Bootstrap,让我们看一下如何包含基本CSS文件。 通过将bootstrap.css
文件添加到我们的.angular-cli.json
配置中,这是一个简单的过程:
"styles": [
"../node_modules/bootstrap/dist/css/bootstrap.css",
"sass/styles.scss"
],
Note: We're using the ..
because the CLI starts looking from within the src/
folder. We had to go up one folder to get to the node_modules
folder.
注意:我们正在使用..
因为CLI从src/
文件夹开始查找。 我们必须上一个文件夹才能进入node_modules
文件夹。
While we can import the Bootstrap CSS this way, this doesn't let us import just sections of Bootstrap or use the Sass variables/mixins that Bootstrap provides.
尽管我们可以通过这种方式导入Bootstrap CSS,但这不能让我们仅导入Bootstrap的各个部分或使用Bootstrap提供的Sass变量/混合。
Let's look at how we can use the Bootstrap Sass files instead of the CSS file.
让我们看看如何使用Bootstrap Sass文件而不是CSS文件。
添加Bootstrap Sass文件 (Adding Bootstrap Sass Files)
Let's cut down the number of CSS rules that we use in our app. Let's look at all the Sass files that Bootstrap uses:
让我们减少在应用程序中使用CSS规则的数量。 让我们看一下Bootstrap使用的所有Sass文件 :
/*!
* Bootstrap v4.0.0-beta (https://getbootstrap.com)
* Copyright 2011-2017 The Bootstrap Authors
* Copyright 2011-2017 Twitter, Inc.
* Licensed under MIT (https://github.com/twbs/bootstrap/blob/master/LICENSE)
*/
@import "functions";
@import "variables";
@import "mixins";
@import "print";
@import "reboot";
@import "type";
@import "images";
@import "code";
@import "grid";
@import "tables";
@import "forms";
@import "buttons";
@import "transitions";
@import "dropdown";
@import "button-group";
@import "input-group";
@import "custom-forms";
@import "nav";
@import "navbar";
@import "card";
@import "breadcrumb";
@import "pagination";
@import "badge";
@import "jumbotron";
@import "alert";
@import "progress";
@import "media";
@import "list-group";
@import "close";
@import "modal";
@import "tooltip";
@import "popover";
@import "carousel";
@import "utilities";
That's a lot of tools that you may not use in your own project.
那是您自己的项目中可能不使用的许多工具。
Inside our src/sass/styles.scss
file, let's import only the Bootstrap files we'll need. Just like we imported Sass files from the src
folder using the tilde (~
), the tilde will also look into the node_modules
folder.
在我们的src/sass/styles.scss
文件中,让我们仅导入所需的Bootstrap文件。 就像我们使用波浪号( ~
)从src
文件夹中导入Sass文件一样,波浪号也将查找node_modules
文件夹。
While we could use the tilde, since we already added Bootstrap to our include_paths
in the stylePreprocessorOptions
section of our .angular-cli.json
:
尽管可以使用波浪号,但是由于我们已经在.angular-cli.json
的stylePreprocessorOptions
部分stylePreprocessorOptions
Bootstrap添加到了include_paths
中:
"styles": [
"styles.scss"
],
"stylePreprocessorOptions": {
"includePaths": [
"../node_modules/bootstrap/scss"
]
},
We can do the following to only get the Bootstrap base tools:
我们可以执行以下操作以仅获取Bootstrap基本工具:
// src/sass/styles.scss
@import
'functions',
'variables',
'mixins',
'print',
'reboot',
'type';
结论 ( Conclusion )
The tilde (~
) and the ability to add paths to stylePreprocessorOptions.includePaths
makes importing Sass files in the Angular CLI super easy! Hope this quick tip was helpful in your Angular journey. Thanks for reading.
波浪号( ~
)以及将路径添加到stylePreprocessorOptions.includePaths
使在Angular CLI中导入Sass文件非常容易! 希望这个快速提示对您的Angular旅程有所帮助。 谢谢阅读。
While it is easy to use the ~
in quick situations, I recommend using the includePaths
method to be explicit about the exact folders where your .scss
files live.
虽然在快速情况下易于使用~
,但我建议使用includePaths
方法来明确显示.scss
文件所在的确切文件夹。
翻译自: https://scotch.io/tutorials/using-sass-with-the-angular-cli
angular sass