javascript
Sometimes we get so wrapped up in new frameworks, coding styles, and tools we forget to stop and get some of the basics back into our head. This article is going to be about that, we are going to look at some of the available array methods and how we can use these methods to sort, filter and reduce an array to get the outcome we want to use.
有时,我们被新的框架,编码样式和工具所笼罩,因此我们忘记停下来,将一些基础知识重新带入我们的头脑。 本文将对此进行探讨,我们将研究一些可用的数组方法,以及如何使用这些方法对数组进行排序,过滤和归约以获得想要使用的结果。
To get more into the basics of JavaScript, we also have our Getting Started with JS for Web Development course you can check out!
为了进一步了解JavaScript的基础知识,我们还提供了JS Web开发入门指南,您可以查看!
介绍 ( Introduction )
Before we start looking at the methods, we need a deep understanding of what arrays are in JavaScript.
在开始研究这些方法之前,我们需要深入了解JavaScript中的数组。
Well, arrays are a list-like object that include methods for us to perform mutations on them, these methods are in the prototype of any array if you console log any array and open its prototype you will see a huge list like this:
好吧, 数组是一个类似于列表的对象,其中包含供我们在其上执行变异的方法,如果您控制台登录任何数组并打开其原型,则这些方法位于任何数组的原型中,您将看到一个庞大的列表,例如:
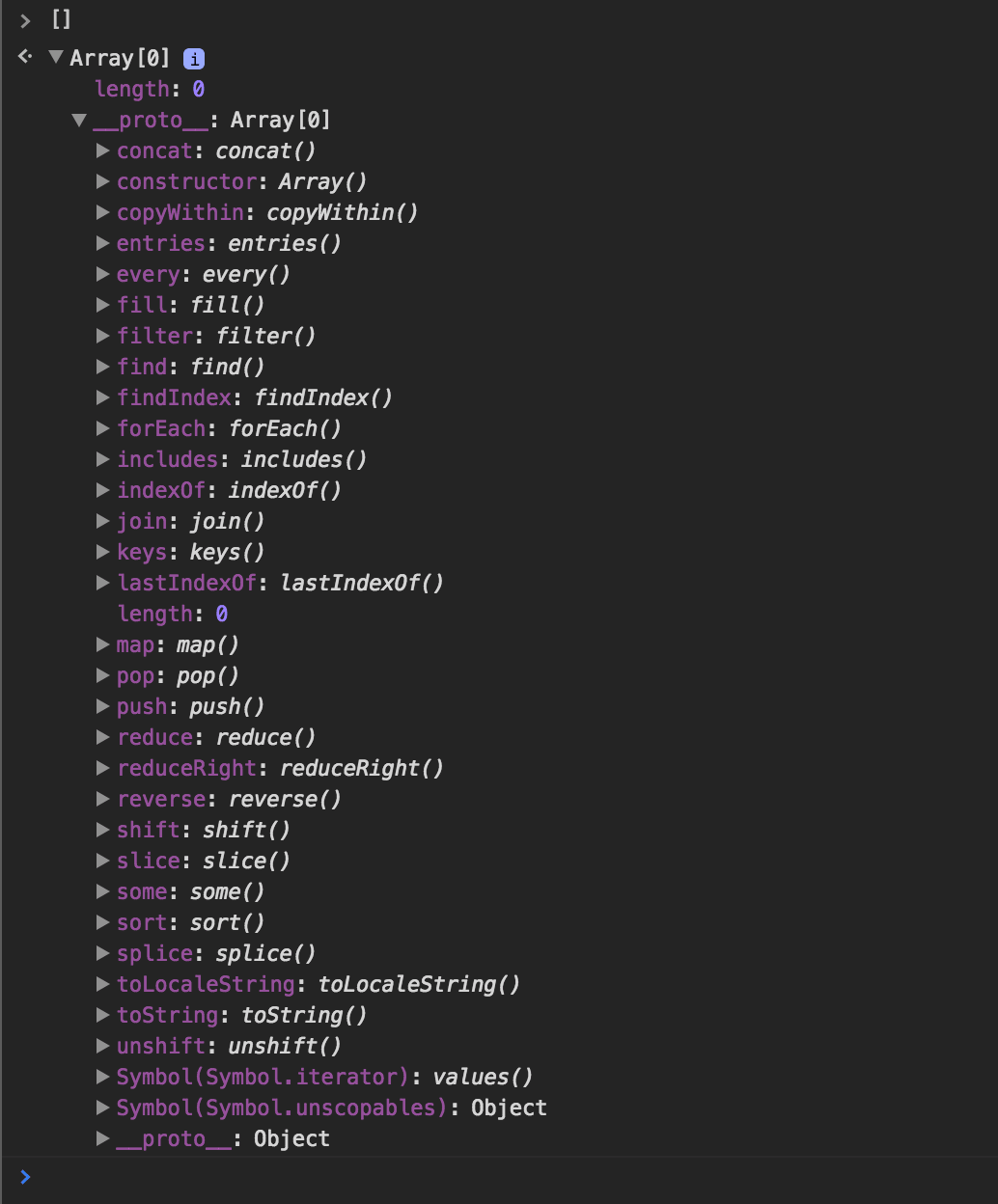
This lists is all the methods you can use in any array to transform it or get values from it. You also need to know that nothing in an array is fixed and can be changed at any time and for this arrays are by definition not immutable, of course, you could choose to make them immutable, but by definition, all arrays can change in contents and length.
此列表列出了您可以在任何数组中用来对其进行转换或从中获取值的所有方法。 您还需要知道数组中的任何内容都是固定的,并且可以随时更改,并且根据定义,该数组不是不可变的,您当然可以选择使其变为不可变的,但是根据定义,所有数组的内容都可以更改和长度。
排序和反向 ( Sort and Reverse )
I'm going to start with these two because, in my opinion, they are the easiest ones to get to know and use in our daily life, I can not tell you how many times I have used any of these two function in an array.
我将从这两个开始,因为我认为它们是我们日常生活中最容易了解和使用的函数,我无法告诉您我在数组中使用这两个函数有多少次了。 。
For these methods we are going to use a simple number array like so:
对于这些方法,我们将使用一个简单的数字数组,如下所示:
const array = [1, 8, 3, 5, 9, 7];
Imagine you were handed this array, but you wanted to show it starting from the lowest number to the highest one. What sort does is sort any array given ascending so in this case, if you did this:
想象一下,您已经收到了这个数组,但是您想要显示从最小的数字到最高的数字。 排序是对给定升序的任何数组进行排序,因此,在这种情况下,如果您这样做:
let sortedArray = array.sort();
It would return a new array with the values:
它将返回一个具有以下值的新数组:
[1, 3, 5, 7, 8, 9]
This sort method also works with names and string characters so if you would like to alphabetically order an array of names or anything involving strings it would do that without any parameters.
这种排序方法也适用于名称和字符串字符,因此,如果您想按字母顺序排列名称数组或任何涉及字符串的东西,它将在没有任何参数的情况下做到这一点。
You can also pass one parameter to it, and that parameter is the sorting function, by default what javascript does is this:
您还可以向其传递一个参数,该参数是排序功能,默认情况下,javascript会执行以下操作:
array.sort((a, b) => {
// a = current item in array
// b = next item in array
return a < b;
});
What this function does in concrete is that it goes through every element in the array and sees if the current one is smaller than the next one and in that way organizes your new array. So if you wanted to this backward all you would have to do is see if the current item is bigger than the next one, like so:
此函数具体作用是遍历数组中的每个元素,并查看当前元素是否小于下一个元素,并以此方式组织新数组。 因此,如果您想向后退,则只需查看当前项目是否大于下一项,如下所示:
array.sort((a, b) => {
return a > b;
});
// [9, 8, 7, 5, 3, 1]
There is also another way to reverse an array, and this is where the reverse function comes in, all this function does is reverse the order of an array, so instead, we can do this:
还有另一种反转数组的方法,这是反转函数的用处,此函数所做的全部就是反转数组的顺序,因此,我们可以这样做:
array.sort().reverse();
// [9, 8, 7, 5, 3, 1]
And it is the same as what we did above in the function we passed inside the sort. This function doesn't take any parameters and what you see is what you get.
这与我们在排序内部传递的函数中所做的相同。 该函数不带任何参数,您所看到的就是所得到的。
.filter()数组 ( .filter() the Array )
After we saw sort and reverse it's time to learn how to filter an array using filter and for this exercise and the ones after this one we are going to use the following array of people:
在看到排序和反向之后,是时候学习如何使用filter过滤数组了,对于本练习以及之后的练习,我们将使用以下人员数组 :
const people = [
{
name: 'Sara',
age: 25,
gender: 'f',
us: true
},
{
name: 'Mike',
age: 18,
gender: 'm',
us: false,
},
{
name: 'Peter',
age: 17,
gender: 'm',
us: false,
},
{
name: 'Ricky',
age: 27,
gender: 'm',
us: false,
},
{
name: 'Martha',
age: 20,
gender: 'f',
us: true
}
];
Having this array imagine you only wanted to get the women in here. Basically what you wanted to do is filter this array and only get the people that have the gender key equal to f.
有这样的想象,你只想把女人带到这里。 基本上,您想要做的是过滤此数组,仅获取性别键等于f的人员。
As you can see I used the word filter a lot because that is exacly the function we are going to use in order to get this new array of elements. The filter function takes one argument and that argument is the function we want to run for each element in the array.
如您所见,我经常使用单词filter,因为这恰好是我们将用来获得此新元素数组的函数。 filter函数接受一个参数,该参数是我们要为数组中的每个元素运行的函数 。
This function we pass into the filter also takes one argument and that one is the current element of the array the filter is in.
我们传递给过滤器的此函数还接受一个参数,其中一个是过滤器所在的数组的当前元素。
得到女人 (Get the Women)
To use this to get the women we would do something like this:
为了使用这个来吸引女性,我们会做这样的事情:
// Filter every element of this array
let women = people.filter(function(person) {
// only return the objects that have the key gender equal to f
return person.gender === 'f';
});
We can actually improve this a lot using ES6 and arrow functions:
实际上,我们可以使用ES6和箭头功能对此进行很多改进:
let women = people.filter((person) => person.gender === 'f');
We are missing the return because when using arrow functions in one line javascript will assume you want to return whatever is in that line.
我们错过了返回值,因为在一行中使用箭头功能时,javascript会假定您要返回该行中的任何内容。
Cool tip: In cases like this you can use
console.table
instead ofconsole.log
to get a table as the output instead of getting the object references.温馨提示:在这种情况下,可以使用
console.table
而不是console.log
来获取表作为输出,而不是获取对象引用。
So if you head over to the console and console.table this we will get the following:
因此,如果您转到console和console.table,我们将获得以下信息:

让人们喝足了 (Getting People Old Enough to Drink)
Now to get into something a little more tricky using filter, imagine you only want to return the people who are old enough to drink, and this defers whether you are from the US or not, for simplicity sake let's assume everyone outside the US has a legal drinking age of 18, in this case we first need to check if the person is in the US or not and then either return people who are older then 21 or if they are not from the US return anyone older than 18.
现在,使用过滤器来解决一些棘手的问题,想象一下,您只想返回那些年龄足够大的可以喝酒的人,这会推迟您是否来自美国,为简单起见,让我们假设美国以外的每个人都有法定饮酒年龄为18岁,在这种情况下,我们首先需要检查此人是否在美国,然后返回21岁以上的人,或者如果他们不是来自美国,则返回18岁以上的人 。
For this we need a small if statement that will check if the person is in the US or not and return it according to it, like so:
为此,我们需要一个小的if语句,该语句将检查该人是否在美国,并根据该人将其返回,如下所示:
let legal = people.filter((person) => {
// If the person in the US only return it if they are older or 21
// If not return the person if the are 18 or above
return person.us ? person.age >= 21 : person.age >= 18;
});
If you now console.table the legal variable you will see this:
如果现在使用console.table合法变量,则会看到以下内容:

As you can see by these two small examples the filter function is really handy for filtering arrays only to get the results you actually want the user to see, this can be very handy when handling website filters.
正如您从这两个小示例可以看到的那样,过滤器功能非常有用,它仅用于过滤数组即可获得您真正希望用户看到的结果,这在处理网站过滤器时非常方便。
.map()数组 ( .map() the Array )
We already created an array that only has the people who have the legal age to drink but wouldn't it be better to add legal as a key of every object and set it to true or false?
我们已经创建了一个数组,仅具有合法年龄的人可以喝酒,但是将Legal作为每个对象的键并将其设置为true或false会更好吗?
This type of transformation is what the map function does, it runs a function for every element in the array we give it and it returns our new array.
这种转换类型是map函数的工作, 它为我们提供的数组中的每个元素运行一个函数,并返回新数组。
In this case, half our work is already done, all this function needs to do is the same conditional statement we just ran in the filter function, the only change it needs to have is get a parameter that will be the object, so we need a function like this:
在这种情况下,我们的工作已经完成了一半,此功能需要做的就是与我们在filter函数中运行的条件语句相同,它唯一需要做的更改就是获取将成为对象的参数,因此我们需要像这样的功能:
let legalFunction = (person) => person.us ? person.age >= 21 : person.age >= 18;
This function either returns true or false depending on the location of the person. Let's move on to the map function and run this legalFunction
everytime map loops the array we give it:
此函数根据人的位置返回true或false。 让我们继续前进到map函数,并在每次map循环我们提供给它的数组时运行此legalFunction
:
let legalFunction = (person) => person.us ? person.age >= 21 : person.age >= 18;
let legalIncluded = people.map((person) => {
// set the new legal key equal to the return of the function we just set
person.legal = legalfunction(person);
// return this person but with the legal key added
return person;
});
Again if we console.table()
this we get:
再次,如果我们console.table()
我们得到:
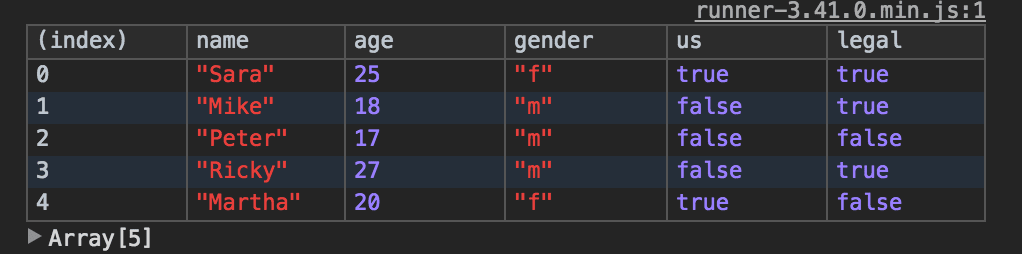
If you check, all the values are correct and our new array has all the information we need. This is of course a simple example of what the map function actually does, if you can run a function for every element in an array you can literally do anything, so if you wanted add 10 years to each person you could simply do:
如果您检查,所有值都是正确的,并且我们的新数组具有我们需要的所有信息。 当然,这是map函数实际作用的简单示例,如果您可以为数组中的每个元素运行一个函数,那么您实际上可以做任何事情,因此,如果您想给每个人加10年,您可以简单地做:
let increaseAge = people.map((person) => {
// set the age key equal it plus 10
person.age = person.age + 10;
return person;
});
In my opnion map is the most flexible transform function you have because of how it allows you to change every element and also add or remove keys with great ease.
在我的opnion贴图中,您拥有的最灵活的转换函数是因为它使您能够轻松更改每个元素以及轻松添加或删除键。
.reduce()数组 ( .reduce() the Array )
In all these cases we started and ended with the same array structure, we may have added or changed the elements inside it but the structure was still the same and sometimes this doesn't cut it.
在所有这些情况下,我们都以相同的数组结构开始和结束,我们可能已经添加或更改了其中的元素,但是结构仍然相同,有时这并没有削减。
Sometimes we need information from an array and transform it into a completly diffrent array, an object or even a number. Let's say we want to get all the combined ages of the people in the array, there is no way we could do this with how it's structured, this needs to be a number and not an array.
有时,我们需要来自数组的信息并将其转换为完全不同的数组,对象甚至是数字。 假设我们要获取数组中所有人员的总年龄,就无法通过其结构来做到这一点,它必须是数字而不是数组。
This is where reduce stands out, it allows you to return a whole new element, this function also takes two arguments, the first one is the function we want to run inside the reduce and the second argument is what we want to start with, bascially our starting structure.
这是reduce突出的地方,它允许您返回一个全新的元素,该函数还带有两个参数,第一个是我们要在reduce中运行的函数,第二个参数是我们想开始的东西我们的起始结构。
The function inside the reduce also takes two parameters, the starter (in this case the number 0) and the current element we are looping through. If you see a reduce function it should look something like this:
reduce中的函数也有两个参数,启动器(在本例中为数字0)和我们正在循环通过的当前元素。 如果您看到一个reduce函数,它应该看起来像这样:
// the two parameters in the function are the 0 and the person we are in
let age = people.reduce((starter, person) => {
// return something to add to the starter
}, 0);
Having these in mind what we really want to do is add the age of the current person the starter of 0 everytime we loop through the array, so what we need is this.:
考虑到这些,我们真正想做的是每次循环遍历数组时,将当前人员的年龄添加为0的起始值,因此我们需要的是:
let age = people.reduce((starter, person) => {
// add the person age to the starter
// As it loops t
return starter + person.age;
}, 0);
// This will return 107
As simple as this example may be it shows us the power of the reduce, in here we got a huge array and changed in such a way that in the end all we had was the number 107. This means you can start with anything, let's use this in order to create an object like this:
就像这个示例一样简单,它向我们展示了reduce的强大功能,在这里我们得到了一个巨大的数组,并进行了更改,最终我们只剩下了数字107。这意味着您可以从任何东西开始,让我们使用它来创建这样的对象:
{men: 3, woman: 2}
So if we want to build this we need to start with an object that has two keys, one for woman and one for man that both have the value of 0 and from there we need to see what the gender of the person is and increase the correct key. That would look something like:
因此,如果要构建此对象,我们需要从一个具有两个键的对象开始,一个对象的值都为0,一个女人的值,一个男人的值,然后从那里查看该人的性别,并增加该值。正确的密钥。 看起来像:
let obj = people.reduce((starter, person) => {
// check if the person is male, if it is increment the value in the starter object with the men key
// if not do that for the woman key
person.gender === 'm' ? starter.men++ : starter.woman++;
// return the modified starter
return starter;
}, {men: 0, woman: 0}); // our starter structure and values
if we console.log this we will get: {men: 3, woman: 2}
as we would expect. In these cases where you want some data and you want to change the structure of it to return something completly diffrent from what you received in the beggining the reduce function is the way to go.
如果我们使用console.log,我们将得到: {men: 3, woman: 2}
。 在这些情况下,如果您需要一些数据并且想要更改其结构以返回与开始使用reduce函数时收到的数据完全不同的内容,则是必须的方法。
其他重要的阵列方法 ( Other Important Array Methods )
While .filter()
, .map()
, and .reduce()
are some of the more commonly used, there are many more:
虽然.filter()
.map()
和.reduce()
是一些比较常用的,还有更多:
.forEach()
: Do something for each item in the array.forEach()
:对数组中的每个项目执行操作.find()
: Like filter but only returns a single item.find()
:与过滤器类似,但仅返回单个项目.push()
: Add new elements to the end of the array.push()
:将新元素添加到数组的末尾.pop()
: Removes the last element of an array.pop()
:删除数组的最后一个元素.join()
: Join the elements of an array into a string.join()
:将数组的元素连接到字符串中.concat()
: Join two or more arrays (returns a copy).concat()
:连接两个或多个数组(返回一个副本)
To find all the methods, check out the reference on w3schools.
要找到所有方法,请查看w3schools上的参考 。
结论 ( Conclusion )
This all I have for you today when it comes to array manipulation and transformation. There is a lot more methods we can cover when it comes to arrays and changing them to fit our needs if you would like a second part here let me know in the comments. In the meantime I hoped this article got you back to the basics of learning the language or if you are just learning it now I hope you got a good grasp on how to modify arrays to fit your everyday needs.
今天,在数组操作和转换方面,我将为您提供所有这些。 如果需要第二部分,请在注释中让我知道,在涉及数组和更改数组以适应我们的需求时,我们可以介绍更多方法。 同时,我希望本文能使您重新学习该语言的基础知识,或者如果您现在只是在学习它,我希望您对如何修改数组以适应日常需要有很好的了解。
翻译自: https://scotch.io/tutorials/flex-those-javascript-array-muscles
javascript