gatsby
Gatsby is a tool for creating static websites with React. It allows you to pull your data from virtually anywhere: content management systems (CMSs), Markdown files, APIs, and databases. Gatsby leverages GraphQL and webpack to combine your data and React code to generate static files for your website.
Gatsby是使用React创建静态网站的工具。 它使您几乎可以从任何地方提取数据:内容管理系统(CMS),Markdown文件,API和数据库。 Gatsby利用GraphQL和webpack来组合您的数据和React代码以为您的网站生成静态文件。
JAM - JavaScript, APIs, and Markup - apps are delivered by pre-rendering files and serving them directly from a CDN, removing the requirement to manage or run web servers. You may have heard of JAM apps as the JAMstack.
JAM-JavaScript,API和Markup-应用通过预渲染文件并直接从CDN提供服务来交付,从而消除了管理或运行Web服务器的要求。 您可能听说过JAM应用称为JAMstack。
Netlify is a hosting company for static sites that offers continuous integration, HTML forms, AWS Lambda functions, and even content management.
Netlify是一家托管静态网站的托管公司,提供持续集成,HTML表单,AWS Lambda函数甚至内容管理。
In this tutorial, I'll show you how to use Gatsby to create a blog that integrates with Netlify CMS for content. The app you build will support authoring posts in Markdown and adding/editing posts from your browser or via Git! Finally, I'll show you how to secure a section of your app with Okta.
在本教程中,我将向您展示如何使用Gatsby创建与Netlify CMS集成内容的博客。 您构建的应用将支持在Markdown中创作帖子以及通过浏览器或通过Git添加/编辑帖子! 最后,我将向您展示如何使用Okta保护应用程序的一部分。
Before you begin, here's a few things you'll need:
在开始之前,需要满足以下条件:
安装Gatsby CLI ( Install Gatsby CLI )
To create a Gatsby site, you'll need to install the Gatsby CLI. This tool gets you up and running with a Gatsby app in no time. It also runs a development server and builds your Gatsby application for production.
要创建Gatsby网站,您需要安装Gatsby CLI。 该工具可让您立即使用Gatsby应用启动并运行。 它还运行开发服务器并构建Gatsby应用程序进行生产。
npm install -g gatsby-cli
用盖茨比创建一个新项目 ( Create a New Project with Gatsby )
Run gatsby new
to create an app using Gatsby's Hello World starter:
运行gatsby new
以使用Gatsby的Hello World入门程序创建应用程序:
gatsby new gatsby-netlify-okta gatsbyjs/gatsby-starter-hello-world
If prompted to choose between yarn and npm, choose npm. This process creates a directory layout, adds a package.json
with dependencies, and prints out instructions to continue.
如果提示您在yarn和npm之间进行选择,请选择npm。 此过程将创建目录布局,添加具有依赖项的package.json
,并打印出说明以继续。
Your new Gatsby site has been successfully bootstrapped. Start developing it by running:cd gatsby-netlify-okta
gatsby develop
NOTE: You can also use npm start
as an alias for gatsby develop
. I use npm start
to do the default tasks on most of my Node projects. I love this attention to detail from the Gatsby developers! ❤️
注意:您也可以将npm start
用作gatsby develop
的别名。 我使用npm start
在大多数Node项目上执行默认任务。 我喜欢Gatsby开发人员对细节的关注! ❤️
You can use the tree
command to view your new project's directory structure.
您可以使用tree
命令查看新项目的目录结构。
$cd gatsby-netlify-okta
$ tree -I node_modules
.
├── LICENSE
├── README.md
├── gatsby-config.js
├── package-lock.json
├── package.json
├── public
│ ├── favicon.ico
│ ├── index.html
│ ├── page-data
│ │ ├── dev-404-page
│ │ │ └── page-data.json
│ │ └── index
│ │ └── page-data.json
│ └── static
├── src
│ └── pages
│ └── index.js
└── static
└── favicon.ico
Run npm start
and check out your "Hello World" app at http://localhost:8000
.

运行npm start
并在http://localhost:8000
签出您的“ Hello World”应用程序。
Now let's move on to adding a neat feature, sourcing content from Netlify CMS!
现在,让我们继续添加一个简洁的功能, 从Netlify CMS采购内容 !
添加用于内容管理的Netlify CMS ( Add Netlify CMS for Content Management )
Netlify CMS is a single-page React app too! Its features include custom-styled previews, UI widgets, editor plugins, and backends to support different Git platform APIs.
Netlify CMS也是单页React应用程序! 它的功能包括自定义样式的预览,UI小部件,编辑器插件和支持不同Git平台API的后端。
You can install Netlify CMS and the Gatsby plugin for it using npm
:
您可以使用npm
为它安装Netlify CMS和Gatsby插件:
npm i netlify-cms-app@2.10.0 gatsby-plugin-netlify-cms@4.1.33
In gatsby-config.js
, register the Netlify CMS plugin:
在gatsby-config.js
,注册Netlify CMS插件:
module.exports = {
plugins: [`gatsby-plugin-netlify-cms`],
}
Then create a static/admin
directory and a config.yml
file in it.
然后在其中创建一个static/admin
目录和一个config.yml
文件。
.static/admin/config.yml
.static/admin/config.yml
backend:
name: test-repo
media_folder: static/assets
public_folder: assets
collections:
- name: blog
label: Blog
folder: blog
create: true
fields:
- { name: path, label: Path }
- { name: date, label: Date, widget: datetime }
- { name: title, label: Title }
- { name: body, label: Body, widget: markdown }
Restart your app using Ctrl+C
and npm start
.
使用Ctrl+C
和npm start
重新启动您的应用npm start
。
You'll now be able to edit content at http://localhost:8000/admin/
.
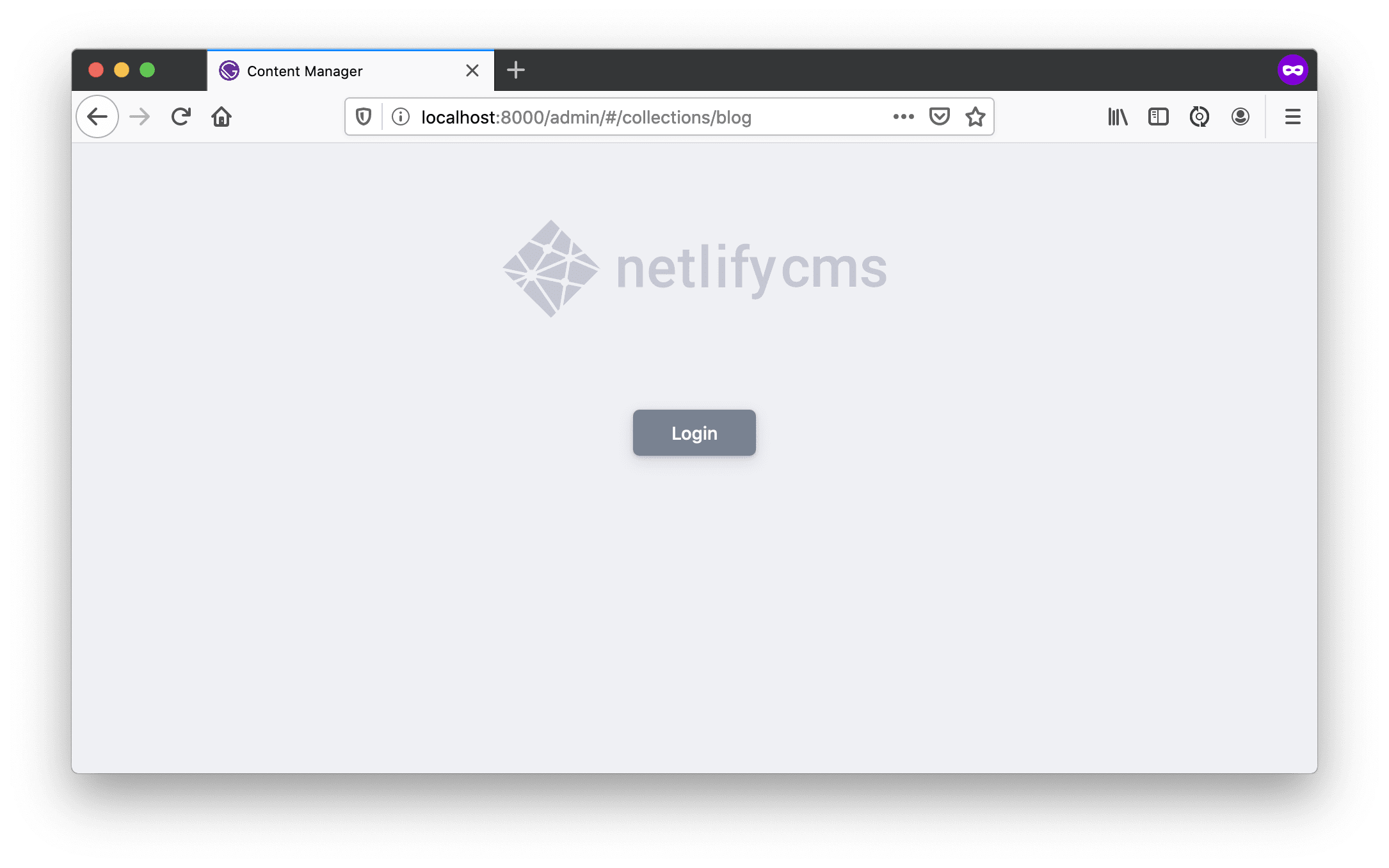
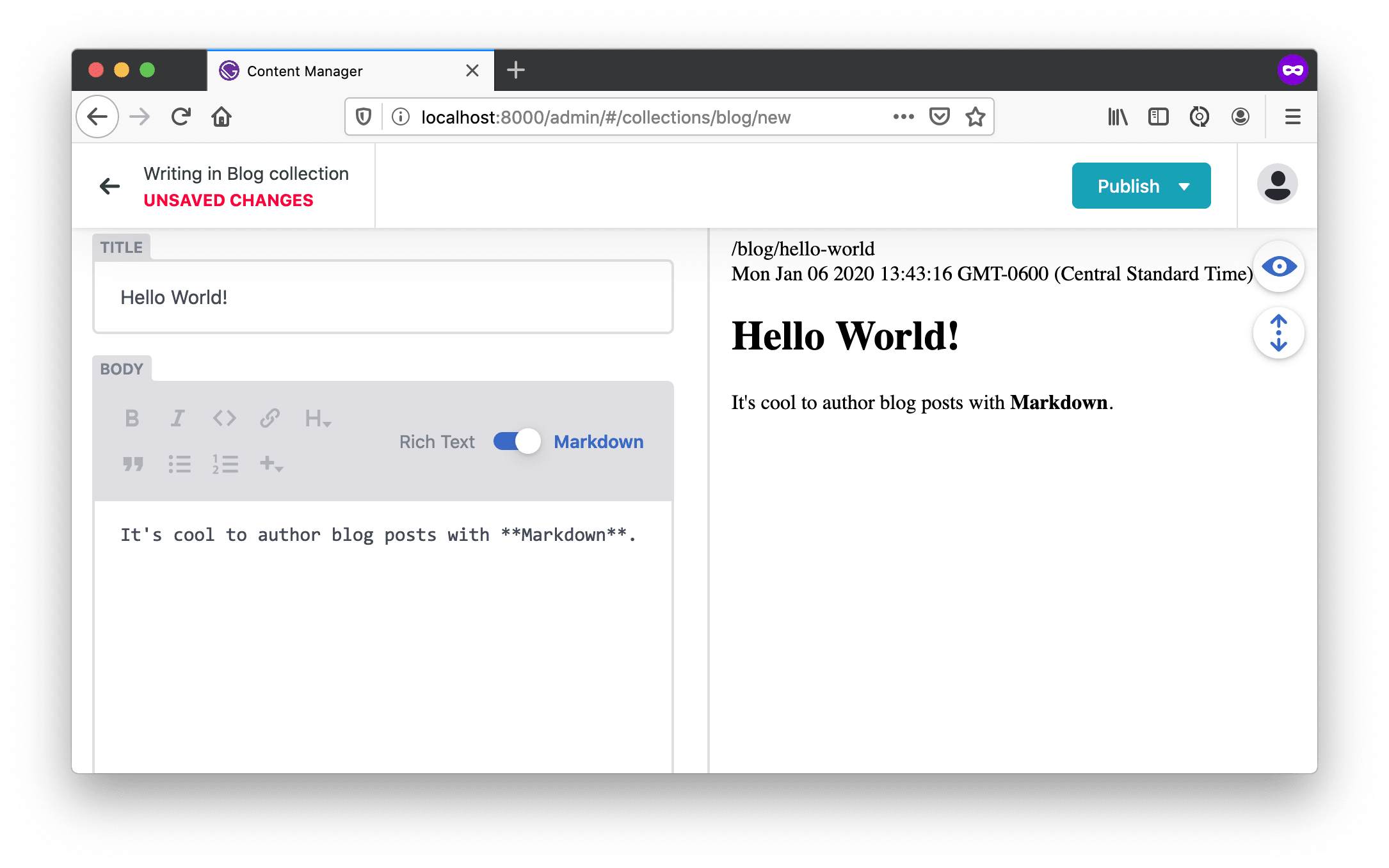
现在,您可以在http://localhost:8000/admin/
上编辑内容。 登录按钮,您将看到下面的屏幕。

Click Publish and you're in business!

点击发布即可开展业务!
Unfortunately, you'll lose your post as soon as you restart your development server. However, you can update Netlify CMS to store files in Git instead!
不幸的是,重新启动开发服务器后,您将失去职位。 但是,您可以更新Netlify CMS来将文件存储在Git中!
将Netlify CMS与GitHub集成以进行持续部署 ( Integrate Netlify CMS with GitHub for Continuous Deployment )
To save to a Git repository, you can create a repo on GitHub, for example, I created one at oktadeveloper/gatsby-netlify-okta-example
.
要保存到Git存储库,您可以在GitHub上创建一个存储库,例如,我在oktadeveloper/gatsby-netlify-okta-example
创建了一个存储库。
You can add Git to your Gatsby project using the following commands:
您可以使用以下命令将Git添加到Gatsby项目中:
git init
git add .
git commit -m "Add project to Git"
git remote add origin git@github.com:${user}/${repo}.git
git push origin master
Now you can publish your Gatsby site straight from GitHub using Netlify's create a new site page.

现在,您可以使用Netlify的create new site page直接从GitHub发布您的Gatsby网站。
You'll be prompted for a Git hosting provider. Click on GitHub.

系统将提示您选择一个Git托管服务提供商。 点击GitHub 。
Find the repository you deployed to.
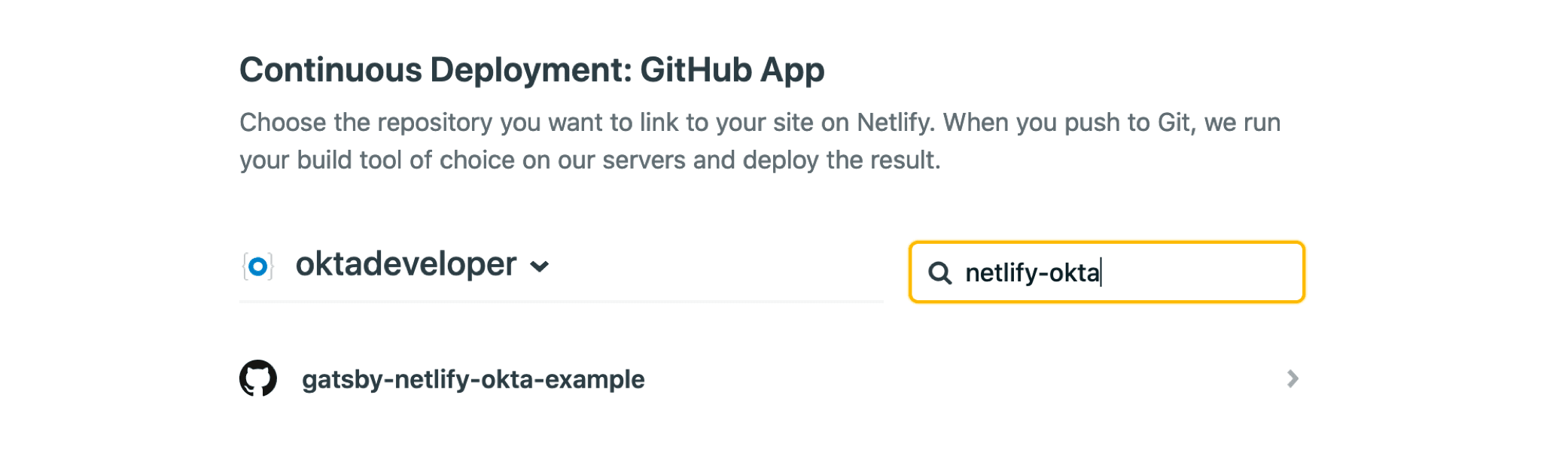
查找您部署到的存储库。
Accept all the default deploy settings and click Deploy site.
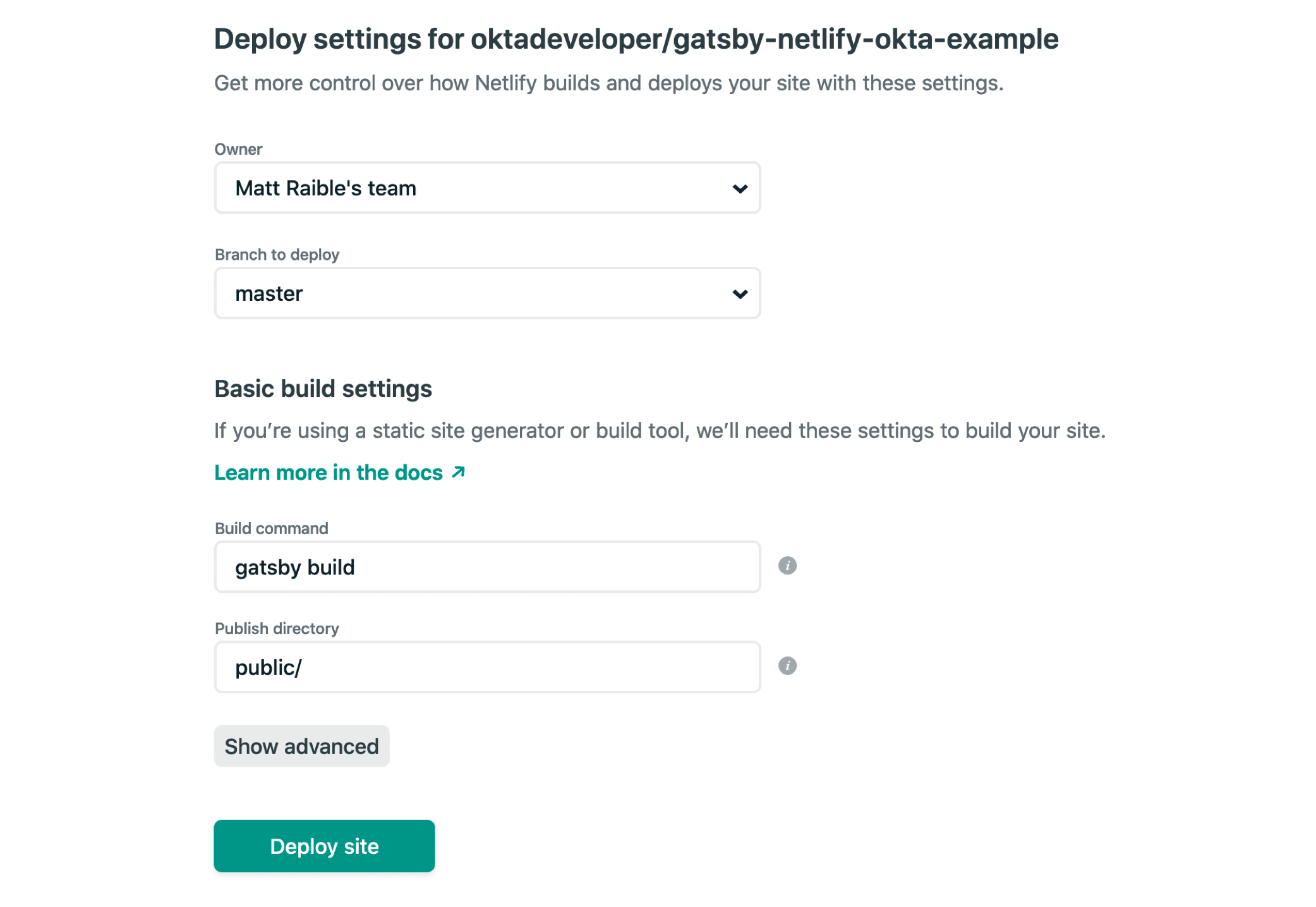
接受所有默认的部署设置,然后单击“ 部署站点” 。
You'll return to your site's dashboard, and the build will be in progress.
您将返回到站点的仪表板,并且构建正在进行中。

In a couple of minutes, your site will be live!

几分钟后,您的网站就会上线!
If you scroll down to the Production deploys section, you can click on the build and see what happened.
如果向下滚动到“ 生产部署”部分,则可以单击生成并查看发生了什么。
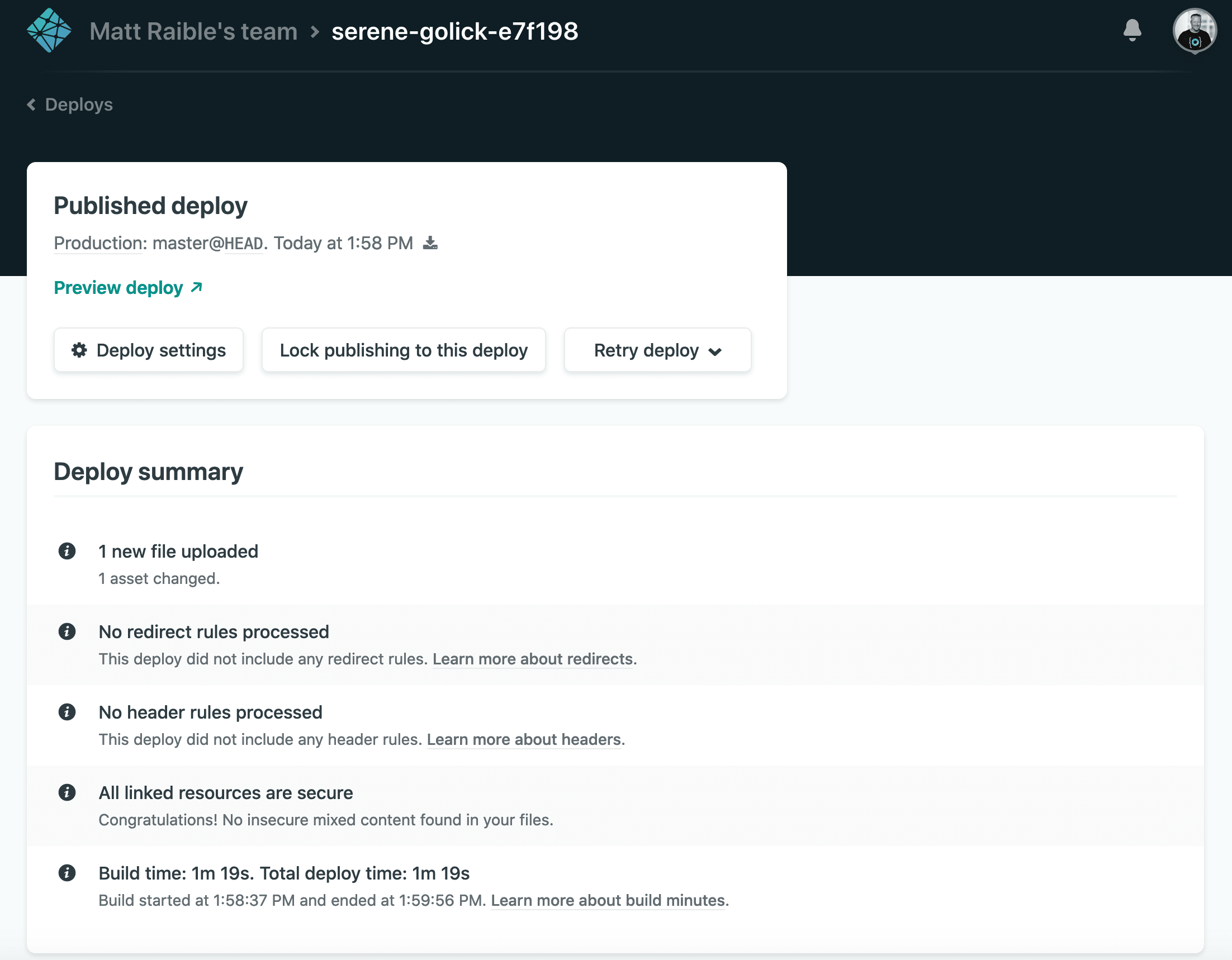
You've built a React app, checked it into source control, and published it to production - that’s pretty cool!
您已经构建了一个React应用程序,将其检入到源代码管理中,并将其发布到生产环境中-太酷了!
Not only that, but you automated the deployment process. Netlify will automatically deploy any changes you push to your GitHub repo. 😎
不仅如此,还使部署过程自动化。 Netlify将自动部署您推送到GitHub存储库的所有更改。 😎
将您的GitHub存储库添加为Netlify CMS后端 ( Add Your GitHub Repo as a Netlify CMS Backend )
Netlify CMS will need to authenticate with GitHub to save your content changes to your repo.
Netlify CMS将需要通过GitHub进行身份验证才能将您的内容更改保存到存储库中。
Modify static/admin/config.yml
to use your GitHub repo:
修改static/admin/config.yml
以使用您的GitHub存储库:
backend:
name: github
repo: your-username/your-repo-name
In my case, I used:
就我而言,我使用了:
backend:
name: github
repo: oktadeveloper/gatsby-netlify-okta-example
Save config.yml
, commit the change, and push it to your GitHub repo.
保存config.yml
,提交更改,并将其推送到您的GitHub存储库中。
git add .
git commit -m "Add GitHub Backend"
git push origin master
When your changes finish deploying on Netlify (it should take around 30 seconds), navigate to your site's /admin/
endpoint. You'll be prompted to log in with GitHub.
当您的更改完成在Netlify上的部署时(大约需要30秒),导航到站点的/admin/
端点。 系统将提示您使用GitHub登录。
Click Login with GitHub, and you'll see a page that says "No Auth Provider Found".
单击“ 使用GitHub登录” ,您将看到一个页面,显示“未找到身份验证提供程序”。
Go to your site's dashboard and navigate to Access control > (scroll down) OAuth.
转到网站的信息中心,然后导航至访问控制 >(向下滚动) OAuth 。
Click Install provider. It will prompt you for a client ID and secret. To get this, navigate to GitHub Developer settings > OAuth Apps > New OAuth App.
单击安装提供程序 。 它将提示您输入客户端ID和密码。 为此,请导航至GitHub Developer设置 > OAuth应用 > 新的OAuth应用 。
Register a new application with the following settings:
使用以下设置注册新的应用程序:
- Application name:
My Gatsby Blog
应用名称:My Gatsby Blog
- Homepage URL:
<copy URL from Netlify>
主页URL:<copy URL from Netlify>
- Authorization callback URL:
https://api.netlify.com/auth/done
授权回调网址:https://api.netlify.com/auth/done
:https://api.netlify.com/auth/done

Click Register application, and you'll be provided with the client ID and secret you were looking for.
点击注册应用程序 ,您将获得所需的客户端ID和密码。
Copy and paste these values into your Netlify OAuth provider dialog and click Install.
将这些值复制并粘贴到您的Netlify OAuth提供程序对话框中,然后单击安装 。
Now, if you go to your site's /admin/
endpoint and log in with GitHub, you'll be prompted for authorization. Click the green Authorize button at the bottom to continue.
现在,如果您转到站点的/admin/
端点并使用GitHub登录,则会提示您进行授权。 单击底部的绿色“ 授权”按钮继续。
If you see an error the first time it loads, you can ignore it. It happens because no blogs exist. Add a new one, and it'll go away. For the path, use something like /blog/first-post
.
如果您在首次加载时看到错误,则可以忽略它。 这是因为不存在博客。 添加一个新的,它将消失。 对于路径,请使用类似/blog/first-post
。
In a terminal window, run git pull origin master
, and you'll see your project is updated with the post you created.
在终端窗口中,运行git pull origin master
,您将看到您的项目已使用您创建的帖子进行更新。
git pull origin master
remote: Enumerating objects: 5, done.
remote: Counting objects: 100% (5/5), done.
remote: Compressing objects: 100% (3/3), done.
remote: Total 4 (delta 1), reused 0 (delta 0), pack-reused 0
Unpacking objects: 100% (4/4), done.
From github.com:oktadeveloper/gatsby-netlify-okta-example
* branch master -> FETCH_HEAD
c1b8722..421a113 master -> origin/master
Updating c1b8722..421a113
Fast-forward
blog/1st-post.md | 6 ++++++
1 file changed, 6 insertions(+)
create mode 100644 blog/1st-post.md
Run npm start
locally to see the blog at http://localhost/admin/
. But how can others (without admin access) read it?
在本地运行npm start
以查看位于http://localhost/admin/
的博客。 但是其他人(没有管理员访问权限)如何阅读呢?
使用新的BlogRoll React组件渲染博客 ( Render Blogs with a New BlogRoll React Component )
Create a src/components/BlogRoll.js
file. This file will contain a React component that queries for blog posts using GraphQL.
创建一个src/components/BlogRoll.js
文件。 该文件将包含一个React组件,该组件使用GraphQL查询博客文章。
import React from 'react'
import PropTypes from 'prop-types'
import { Link, graphql, StaticQuery } from 'gatsby'
class BlogRoll extends React.Component {
render() {
const { data } = this.props;
const { edges: posts } = data.allMarkdownRemark;
return (
<div className="columns is-multiline">
{posts &&
posts.map(({ node: post }) => (
<div className="is-parent column is-6" key={post.id}>
<article
className={`blog-list-item tile is-child box notification ${
post.frontmatter.featuredpost ? 'is-featured' : ''
}`}
>
<header>
<p className="post-meta">
<Link
className="title has-text-primary is-size-4"
to={post.frontmatter.path}
>
{post.frontmatter.title}
</Link>
<span> • </span>
<span className="subtitle is-size-5 is-block">
{post.frontmatter.date}
</span>
</p>
</header>
<p>
{post.excerpt}
<br />
<br />
<Link className="button" to={post.frontmatter.path}>
Keep Reading →
</Link>
</p>
</article>
</div>
))}
</div>
)
}
}
BlogRoll.propTypes = {
data: PropTypes.shape({
allMarkdownRemark: PropTypes.shape({
edges: PropTypes.array,
}),
}),
};
export default () => (
<StaticQuery
query={graphql`query BlogRollQuery {
allMarkdownRemark(
sort: { order: DESC, fields: [frontmatter___date] }
) {
edges {
node {
excerpt(pruneLength: 400)
id
frontmatter {
path
title
date(formatString: "MMMM DD, YYYY")
}
}
}
}
}`}
render={(data, count) => <BlogRoll data={data} count={count} />}
/>
)
Create a new page at src/pages/blog.js
to serve as the index page for blogs.
在src/pages/blog.js
创建一个新页面,以用作博客的索引页面。
import React from 'react'
import BlogRoll from '../components/BlogRoll'
export default class BlogIndexPage extends React.Component {
render() {
return (
<React.Fragment>
<h1>Latest Posts</h1>
<section>
<div className="content">
<BlogRoll />
</div>
</section>
</React.Fragment>
)
}
}
Then add a link to it in src/pages/index.js
:
然后在src/pages/index.js
添加指向它的链接:
import React from 'react'
import { Link } from 'gatsby'
export default () => {
return (
<>
Hello world!
<p><Link to="/blog">View Blog</Link></p>
</>)
}
Restart your Gatsby app using npm start
and navigate to http://localhost:8000
.
使用npm start
重新启动Gatsby应用,并导航到http://localhost:8000
。
You'll receive an error because your project doesn't have Markdown support.
您会收到一条错误消息,因为您的项目不支持Markdown。
Generating development JavaScript bundle failed
/Users/mraible/blog/gatsby-netlify-okta/src/components/BlogRoll.js
62:9 error Cannot query field"allMarkdownRemark" on type "Query" graphql/template-strings
✖ 1 problem (1 error, 0 warnings)
File: src/components/BlogRoll.js
为Gatsby添加Markdown支持 ( Add Markdown Support to Gatsby )
Gatsby's Add Markdown Pages docs show the process that it uses to create pages from Markdown files:
Gatsby的“ 添加Markdown页面”文档显示了其用于从Markdown文件创建页面的过程:
- Read files into Gatsby from the filesystem 从文件系统将文件读入Gatsby
- Transform Markdown to HTML and frontmatter to data 将Markdown转换为HTML,并将其转换为数据
- Add a Markdown file 添加Markdown文件
- Create a page component for the Markdown files 为Markdown文件创建页面组件
- Create static pages using Gatsby's Node.js
createPage()
API 使用Gatsby的Node.jscreatePage()
API创建静态页面
Install a couple of Gatsby plugins to make this happen.
安装几个Gatsby插件以实现此目的。
npm i gatsby-source-filesystem gatsby-transformer-remark
Then configure them in gatsby-config.js
:
然后在gatsby-config.js
配置它们:
module.exports = {
plugins: [
`gatsby-plugin-netlify-cms`,
{
resolve: `gatsby-source-filesystem`,
options: {
path: `${__dirname}/blog`,
name: `markdown-pages`,
},
},
`gatsby-transformer-remark`
]
}
Restart everything and you'll be able to see your blog posts at /blogs
.

重新启动所有内容,您将可以在/blogs
上看到您的博客文章。
However, if you try to navigate into a blog, it doesn't work because you didn't tell Gatsby to generate pages for each one.
但是,如果您尝试进入博客,则该博客不起作用,因为您没有告诉Gatsby为每个博客生成页面。
使用Gatsby的Node API生成静态博客页面 ( Use Gatsby's Node API to Generate Static Blog Pages )
Create a gatsby-node.js
in the root directory of your project and add code to create a static page for each blog.
在项目的根目录中创建一个gatsby-node.js
并添加代码以为每个博客创建一个静态页面。
const path = require(`path`);
exports.createPages = async ({actions, graphql, reporter}) => {
const {createPage} = actions;
const blogPostTemplate = path.resolve(`src/templates/blog.js`);
const result = await graphql(`{
allMarkdownRemark(
sort: { order: DESC, fields: [frontmatter___date] }
limit: 1000
) {
edges {
node {
frontmatter {
path
}
}
}
}
}`);
// Handle errors
if (result.errors) {
reporter.panicOnBuild(`Error while running GraphQL query.`);
return
}
result.data.allMarkdownRemark.edges.forEach(({node}) => {
createPage({
path: node.frontmatter.path,
component: blogPostTemplate,
context: {}, // additional data can be passed via context
})
})
};
You might notice this JavaScript code uses a template at src/templates/blog.js
. Create this file with the following code in it.
您可能会注意到此JavaScript代码在src/templates/blog.js
使用了模板。 使用以下代码创建该文件。
import React from "react"
import { graphql } from "gatsby"
export default function Template({
data, // this prop will be injected by the GraphQL query below.
}) {
const { markdownRemark } = data // data.markdownRemark holds your post data
const { frontmatter, html } = markdownRemark
return (
<div className="blog-post-container">
<div className="blog-post">
<h1>{frontmatter.title}</h1>
<h2>{frontmatter.date}</h2>
<div
className="blog-post-content"
dangerouslySetInnerHTML={{ __html: html }}
/>
</div>
</div>
)
}
export const pageQuery = graphql`query($path: String!) {
markdownRemark(frontmatter: { path: { eq: $path } }) {
html
frontmatter {
date(formatString: "MMMM DD, YYYY")
path
title
}
}
}`
Restart your app to see Markdown rendering properly!

重新启动您的应用程序以查看Markdown渲染正确!
Commit your changes and verify everything works in production.
提交您的更改,并验证生产中一切正常。
git add .
git commit -m "Add /blog and Markdown support"
git push origin master
添加帐户部分 ( Add an Account Section )
Add an Account section for your site by creating a file at src/pages/account.js
.
通过在src/pages/account.js
创建文件,为您的站点添加一个Account部分。
import React from 'react'
import { Router } from '@reach/router'
import { Link } from 'gatsby'
const Home = () => <p>Home</p>;
const Settings = () => <p>Settings</p>;
const Account = () => {
return (
<>
<nav>
<Link to="/">Home</Link>{' '}
<Link to="/account">My Account</Link>{' '}
<Link to="/account/settings">Settings</Link>{' '}
</nav>
<h1>My Account</h1>
<Router>
<Home path="/account"/>
<Settings path="/account/settings"/>
</Router>
</>
)
};
export default Account
Add a link to the account page in src/pages/index.js
:
在src/pages/index.js
添加指向帐户页面的链接:
import React from 'react'
import { Link } from 'gatsby'
export default () => {
return (
<>
Hello world!
<p><Link to="/blog">View Blog</Link></p>
<p><Link to="/account">My Account</Link></p>
</>)
}
Since this section will have dynamic content that shouldn't be rendered statically, you need to exclude it from the build. Add the following JavaScript to the bottom of gatsby-node.js
to indicate that /account
is a client-only route.
由于本节将包含不应静态呈现的动态内容,因此您需要将其从构建中排除。 在gatsby-node.js
的底部添加以下JavaScript,以表明/account
是仅客户端的路由。
exports.onCreatePage = async ({ page, actions }) => {
const { createPage } = actions;
if (page.path.match(/^\/account/)) {
page.matchPath = "/account/*";
createPage(page)
}
};
Restart with npm start
and you should be able to navigate to this new section.
通过npm start
重新npm start
,您应该能够导航到这个新部分。
向Okta注册您的应用 ( Register Your App with Okta )
To begin with Okta, you'll need to register your app, just like you did with GitHub. Log in to your Okta developer account and navigate to Applications > Add Application.
要开始使用Okta,您需要注册您的应用程序,就像使用GitHub一样。 登录到您的Okta开发人员帐户 ,然后导航至“ 应用程序” >“ 添加应用程序” 。
- Choose Single-Page App and Next 选择单 页应用程序 , 然后单击下一步
- Enter a name like
Gatsby Account
输入类似Gatsby Account
的名称 - Specify the following Login redirect URIs:
http://localhost:8000/account
http://localhost:9000/account
https://<your-site>.netlify.com/account
-
http://localhost:8000/account
-
http://localhost:9000/account
-
https://<your-site>.netlify.com/account
- Specify the following Logout redirect URIs:
http://localhost:8000
http://localhost:9000
https://<your-site>.netlify.com
-
http://localhost:8000
-
http://localhost:9000
-
https://<your-site>.netlify.com
- Click Done 点击完成
Your Okta application settings should resemble the screenshot below.
您的Okta应用程序设置应类似于以下屏幕截图。

为盖茨比网站添加可信来源 (Add Trusted Origins for Your Gatsby Sites)
Gatsby can run on two different ports (8000 and 9000) locally. One is for development and one is for production (invoked with gatsby build
and gatsby serve
). You also have your production Netlify site. Add all of these as Trusted Origins in API > Trusted Origins.
Gatsby可以在本地两个不同的端口(8000和9000)上运行。 一种是用于开发,另一种是用于生产(使用gatsby build
和gatsby serve
调用)。 您也有您的生产Netlify网站。 将所有这些都添加为API > Trusted Origins中的Trusted Origins 。
Click Add Origin, select CORS and Redirect for Type, and add each of the following:
单击“ 添加来源” ,选择“ CORS”和“ 重定向”作为“类型”,然后添加以下各项:
http://localhost:8000
http://localhost:8000
http://localhost:9000
http://localhost:9000
https://<your-site>.netlify.com
https://<your-site>.netlify.com
When you're finished, your screen should resemble the following.
完成后,您的屏幕应类似于以下内容。
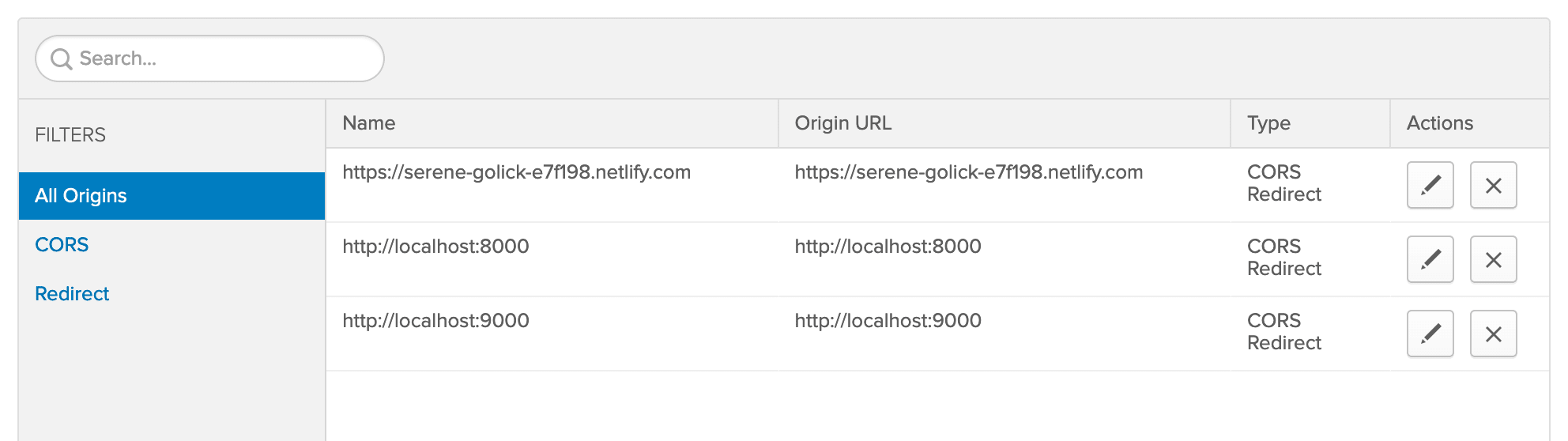
使用Okta保护您的盖茨比帐户部分 ( Protect Your Gatsby Account Section with Okta )
Install Okta's Sign-In Widget:
安装Okta的登录小部件:
npm i @okta/okta-signin-widget@3.6.0
Create a Login
component in src/components/Login.js
:
在src/components/Login.js
创建一个Login
组件:
import OktaSignIn from '@okta/okta-signin-widget';
import '@okta/okta-signin-widget/dist/css/okta-sign-in.min.css';
import React from 'react';
const config = {
baseUrl: '<okta-org-url>',
clientId: '<okta-client-id>',
logo: '//logo.clearbit.com/gatsbyjs.org',
redirectUri: typeof window !== 'undefined' && window.location.origin + '/account',
el: '#signIn',
authParams: {
pkce: true,
responseType: ['token', 'id_token']
}
};
export const signIn = typeof window !== 'undefined' && new OktaSignIn(config);
export default class Login extends React.Component {
constructor(props) {
super(props);
this.state = {
user: false
};
this.signIn = signIn;
}
async componentDidMount() {
const authClient = this.signIn.authClient;
const session = await authClient.session.get();
console.log('session.status', session.status);
// Session exists, show logged in state.
if (session.status === 'ACTIVE') {
// clear parameters from browser window
window.location.hash = '';
// set username in state
this.setState({user: session.login});
localStorage.setItem('isAuthenticated', 'true');
// get access and ID tokens
authClient.token.getWithoutPrompt({
scopes: ['openid', 'email', 'profile'],
}).then((tokens) => {
tokens.forEach(token => {
if (token.idToken) {
authClient.tokenManager.add('idToken', token);
}
if (token.accessToken) {
authClient.tokenManager.add('accessToken', token);
}
});
// Say hello to the person who just signed in
authClient.tokenManager.get('idToken').then(idToken => {
console.log(`Hello, ${idToken.claims.name} (${idToken.claims.email})`);
window.location.reload();
});
}).catch(error => console.error(error));
return;
} else {
this.signIn.remove();
}
this.signIn.renderEl({el: '#signIn'})
}
render() {
return (
<div id="signIn"/>
)
}
}
Replace the placeholders near the top of this file with your Okta app settings.
用Okta应用设置替换该文件顶部附近的占位符。
const config = {
baseUrl: '<okta-org-url>',
clientId: '<okta-client-id>',
...
};
For example:
例如:
const config = {
baseUrl: 'https://dev-133320.okta.com',
clientId: '0oa2ee3nvkHIe8vzX357',
...
};
Modify src/pages/account.js
to include an Account
component that uses Login
to get ID tokens and logout.
修改src/pages/account.js
以包括一个使用Login
来获取ID令牌和注销的Account
组件。
import React from 'react'
import { navigate, Router } from '@reach/router'
import { Link } from 'gatsby'
import Login, { signIn } from '../components/Login'
const Home = () => <p>Account Information</p>;
const Settings = () => <p>Settings</p>;
const isAuthenticated = () => {
if (typeof window !== 'undefined') {
return localStorage.getItem('isAuthenticated') === 'true';
} else {
return false;
}
};
class Account extends React.Component {
constructor(props) {
super(props);
this.state = {user: false};
this.logout = this.logout.bind(this);
}
async componentDidMount() {
const token = await signIn.authClient.tokenManager.get('idToken');
if (token) {
this.setState({user: token.claims.name});
} else {
// Token has expired
this.setState({user: false});
localStorage.setItem('isAuthenticated', 'false');
}
}
logout() {
signIn.authClient.signOut().catch((error) => {
console.error('Sign out error: ' + error)
}).then(() => {
localStorage.setItem('isAuthenticated', 'false');
this.setState({user: false});
navigate('/');
});
}
render() {
if (!isAuthenticated()) {
return (
<Login/>
);
}
return (
<>
<nav>
<Link to="/">Home</Link>{' '}
<Link to="/account">My Account</Link>{' '}
<Link to="/account/settings">Settings</Link>{' '}
</nav>
<h1>My Account</h1>
<React.Fragment>
<p>Welcome, {this.state.user}. <button onClick={this.logout}>Logout</button></p>
</React.Fragment>
<Router>
<Home path="/account"/>
<Settings path="/account/settings"/>
</Router>
</>
)
}
}
export default Account
Restart your app with npm start
, open http://localhost:8000
in a private window, and click on My Account. You'll be prompted to log in.
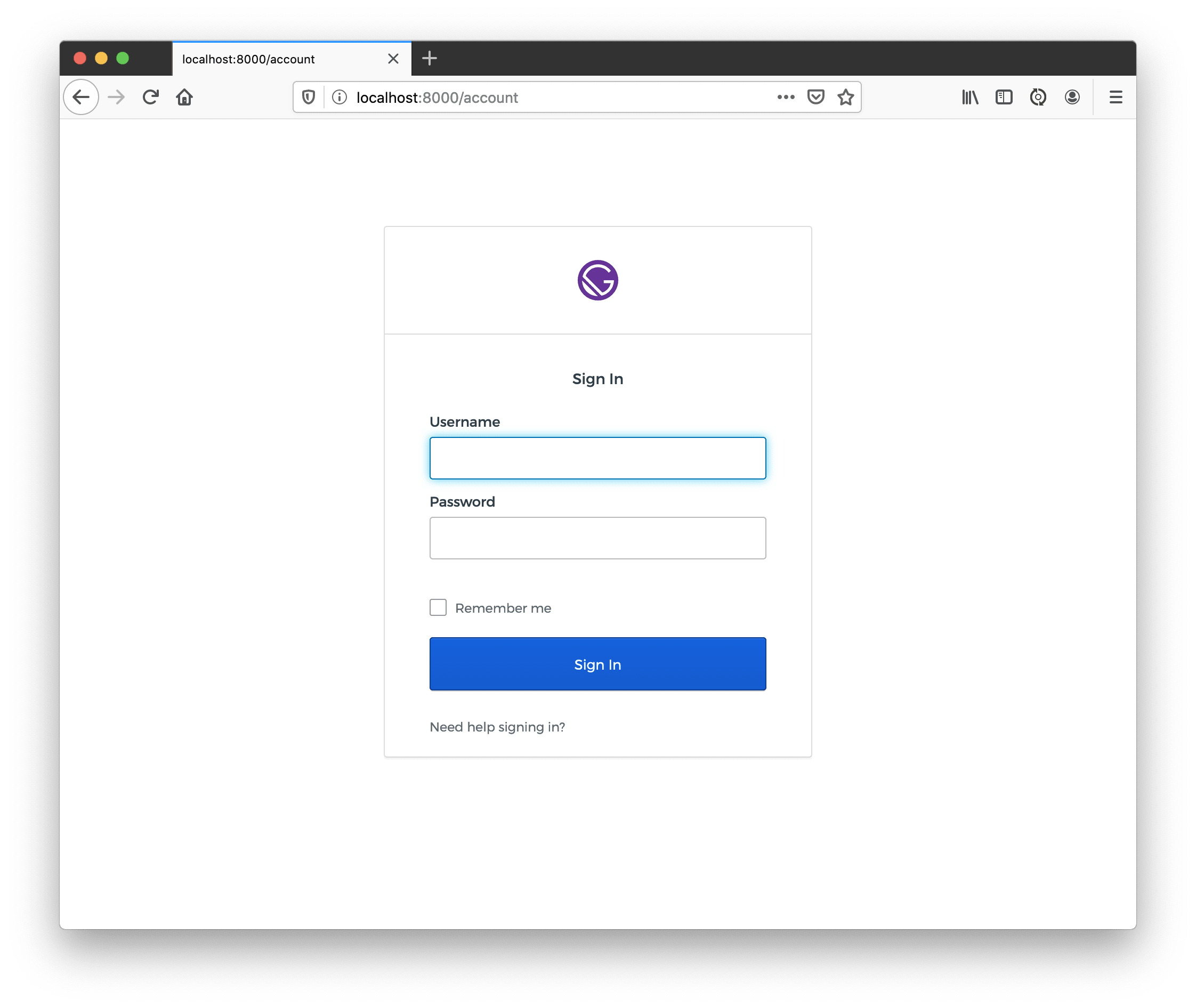
使用npm start
重新启动您的应用npm start
,在一个私有窗口中打开http://localhost:8000
,然后单击我的帐户 。 系统将提示您登录。
Enter your credentials and click Sign In to browse the account section. You should also see your name and be able to logout.
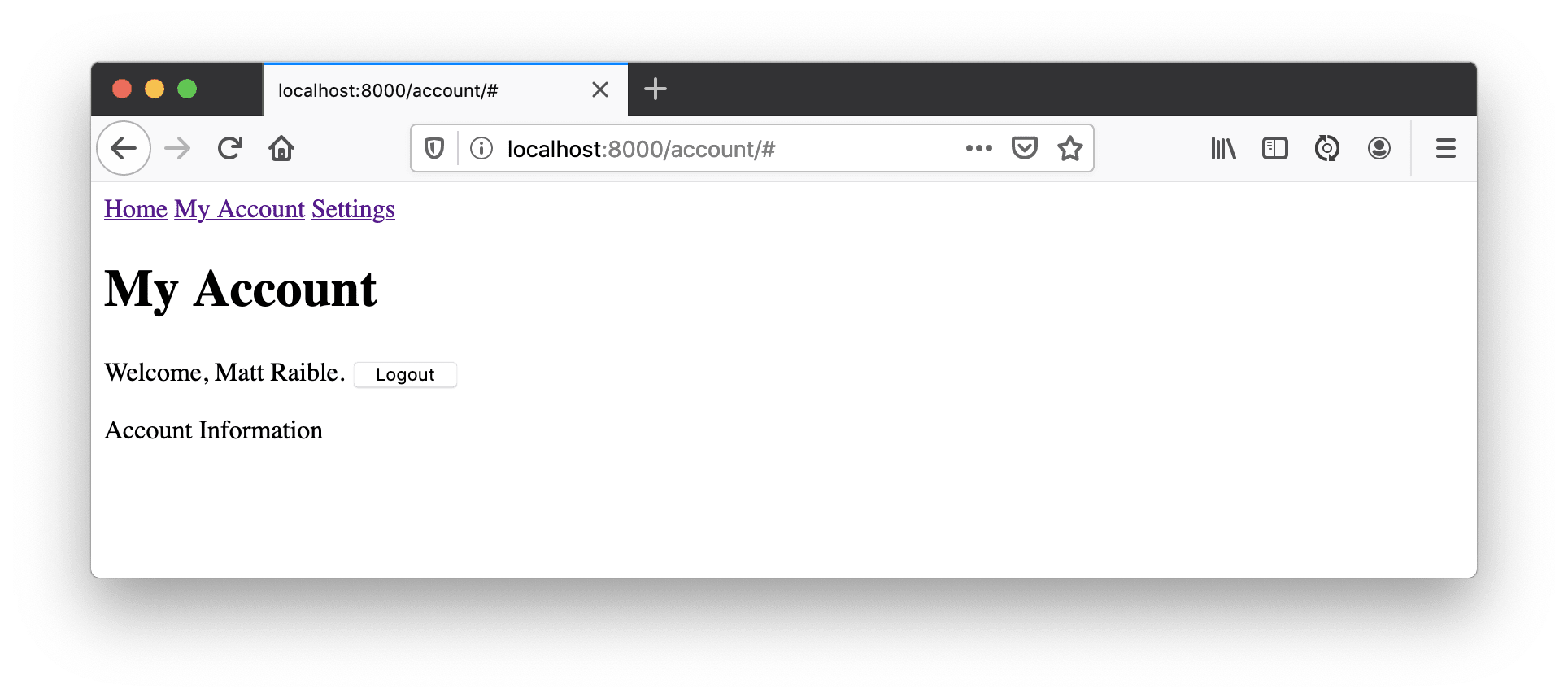
输入您的凭据,然后单击登录以浏览帐户部分。 您还应该看到您的名字并能够注销。
Fix Gatsby生产版本 ( Fix Gatsby Production Build )
To test building your app for production, run gatsby build
. You'll get an error because Okta's Sign-In Widget doesn't expect to be compiled for server-side rendering.
要测试构建用于生产的应用程序,请运行gatsby build
。 您会收到错误消息,因为Okta的Sign-In Widget不希望被编译用于服务器端渲染。
failed Building static HTMLfor pages - 1.730s
ERROR #95312
"window" is not available during server side rendering.
See our docs page for more info on this error: https://gatsby.dev/debug-html
12 |
13 | (function (){
> 14 | var isChrome = 'chrome' in window && window.navigator.userAgent.indexOf('Edge') < 0;
| ^
15 | if ('u2f' in window || !isChrome) {
16 | return;
17 | }
WebpackError: ReferenceError: window is not defined
- u2f-api-polyfill.js:14
node_modules/u2f-api-polyfill/u2f-api-polyfill.js:14:1
- u2f-api-polyfill.js:754 Object../node_modules/u2f-api-polyfill/u2f-api-polyfill.js
node_modules/u2f-api-polyfill/u2f-api-polyfill.js:754:2
- okta-sign-in.entry.js:3 webpackUniversalModuleDefinition
node_modules/@okta/okta-signin-widget/dist/js/okta-sign-in.entry.js:3:104
- okta-sign-in.entry.js:10 Object../node_modules/@okta/okta-signin-widget/dist/js/okta-sign-in.entry.js
node_modules/@okta/okta-signin-widget/dist/js/okta-sign-in.entry.js:10:2
- Login.js:1 Module../src/components/Login.js
src/components/Login.js:1:1
- account.js:1 Module../src/pages/account.js
src/pages/account.js:1:1
To fix this, you can exclude it from the compilation process. Modify the webpack build to exclude it from compilation by configuring webpack. Add the JavaScript below to the bottom of gatsby-node.js
.
要解决此问题,可以将其从编译过程中排除。 通过配置webpack来修改webpack构建以将其从编译中排除。 将以下JavaScript添加到gatsby-node.js
的底部。
exports.onCreateWebpackConfig = ({ stage, loaders, actions }) => {
if (stage === 'build-html') {
// Exclude Sign-In Widget from compilation path
actions.setWebpackConfig({
module: {
rules: [
{
test: /okta-sign-in/,
use: loaders.null(),
}
],
},
})
}
};
Try gatsby build
again and it should work this time. Run gatsby serve
to see if the production build works on http://localhost:9000
. Rejoice when it does!
再次尝试gatsby build
,这次应该可以使用了。 运行gatsby serve
来查看生产版本是否可以在http://localhost:9000
。 当它高兴时!
添加用户注册 ( Add User Registration )
To give people the ability to sign-up for accounts, go to your Okta dashboard > Users > Registration, and enable it.
要使人们能够注册帐户,请转到Okta信息中心> 用户 > 注册并启用它。
Modify src/components/Login.js
to add Okta's user registration feature.
修改src/components/Login.js
以添加Okta的用户注册功能。
const config = {
...
authParams: {
pkce: true,
responseType: ['token', 'id_token']
},
features: {
registration: true
}
};
Then build for production and serve it up again.
然后进行生产并再次使用。
gatsby build
gatsby serve
You will now see a Sign Up link at the bottom of the login form.
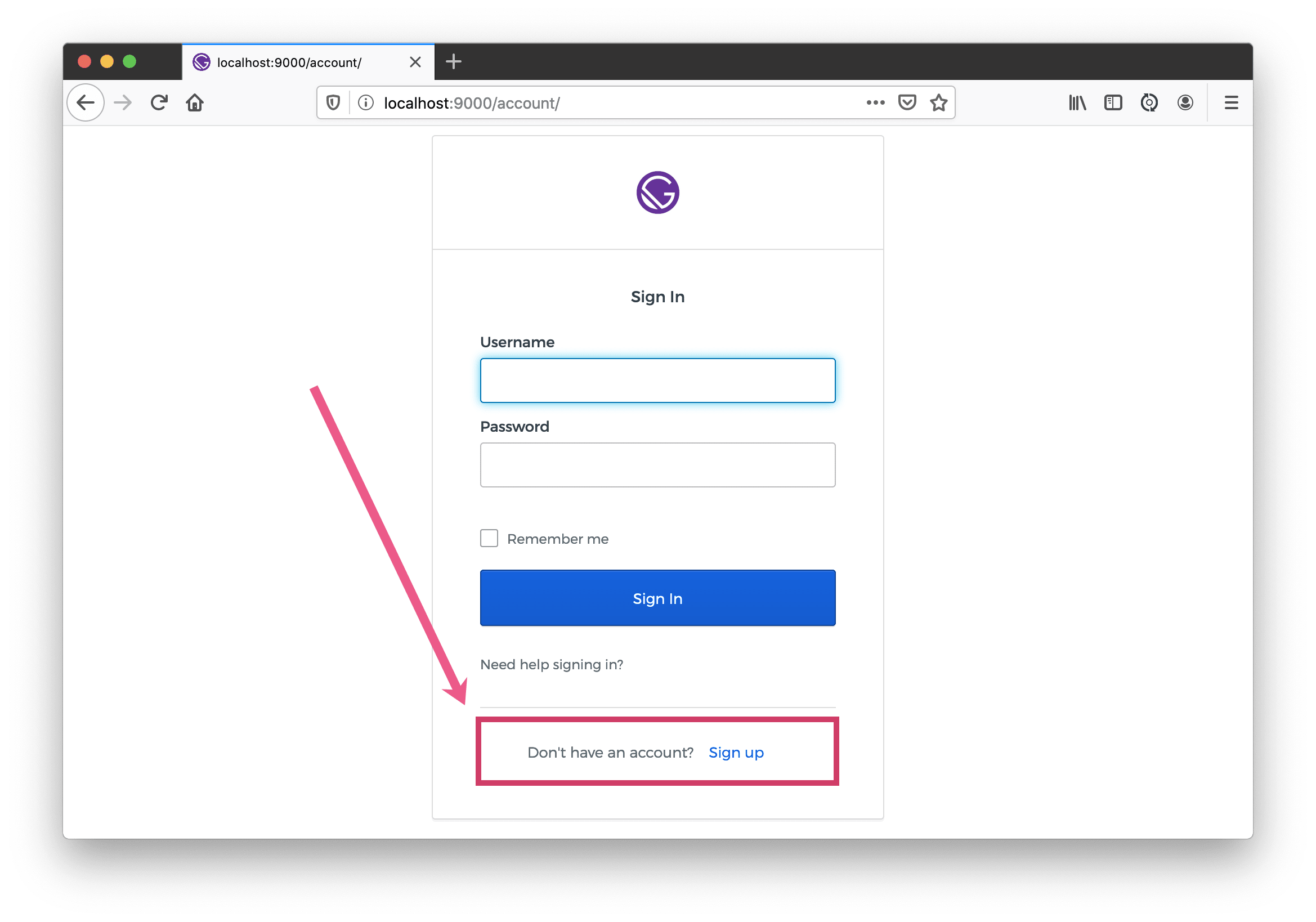
现在,您将在登录表单的底部看到一个“ 注册”链接。
Click the link to see the user registration form.

单击链接以查看用户注册表格。
Hooray - you did it! Check in your code and rejoice in your new-found knowledge. 🥳
万岁-您做到了! 签入您的代码,并以新发现的知识为乐。 🥳
扩展您的盖茨比帐户功能 (Extend Your Gatsby Account Functionality)
Armed with Okta for authentication, you could develop features in the account settings of your application. For example, a setting where people can sign up for a newsletter (e.g., with TinyLetter). You could store this setting in Okta by creating a Node app that uses the Okta Node SDK to update user attributes.
借助Okta进行身份验证,您可以在应用程序的帐户设置中开发功能。 例如,人们可以注册新闻通讯的设置(例如,使用TinyLetter )。 您可以通过创建使用Okta Node SDK更新用户属性的Node应用程序将该设置存储在Okta中。
In fact, you might even develop a Java or .NET backend to handle this and communicate to it from your Gatsby application using fetch()
and an OAuth 2.0 access token retrieved from the Sign-In Widget.
实际上,您甚至可以开发Java或.NET后端来处理此问题,并使用fetch()
和从“登录”小部件中检索到的OAuth 2.0访问令牌从Gatsby应用程序与其通信。
async componentDidMount() {
try {
const response = await fetch('http://<node-server-url>/user/settings', {
headers: {
Authorization: 'Bearer ' + await signIn.authClient.tokenManager.get('accessToken')
}
});
const data = await response.json();
this.setState({ settings: data.settings });
} catch (err) {
// handle error as needed
console.error(err);
}
}
了解有关Netlify,Gatsby,React和身份验证的更多信息 ( Learn More about Netlify, Gatsby, React, and Authentication )
Phew! This tutorial packed a punch! 💥👊
唷 ! 本教程是一拳! 💥👊
You learned how to build a new site with Gatsby, automate its deployment with Netlify, integrate Gatsby with Netlify CMS, process Markdown files, store your files in Git, and use Okta for authentication. Okta leverages OAuth 2.0 and OpenID Connect for its developer APIs. They're awesome!
您学习了如何使用Gatsby构建新站点,如何使用Netlify自动化其部署,如何将Gatsby与Netlify CMS集成,处理Markdown文件,将文件存储在Git中以及如何使用Okta进行身份验证。 Okta将OAuth 2.0和OpenID Connect用于其开发人员API。 他们很棒!
You can find the source code for this example on GitHub in the oktadeveloper/gatsby-netlify-okta-example repository.
您可以在oktadeveloper / gatsby-netlify-okta-example存储库中的GitHub上找到此示例的源代码。
If you want to make your Gatsby site even more secure, you can use the Gatsby Netlify plugin as it adds a bunch of basic security headers. After installing, configuring, and deploying, you can test your site's security with securityheaders.com.
如果您想使Gatsby网站更加安全,可以使用Gatsby Netlify插件,因为它添加了一堆基本的安全标头。 安装,配置和部署后,您可以使用securityheaders.com测试站点的安全性 。
I learned a bunch about Gatsby and authentication from Jason Lengstorf and Aaron Parecki in their Add authentication to your apps with Okta video.
我从Jason Lengstorf和Aaron Parecki的“通过Okta视频向您的应用程序添加身份验证”中学到了很多有关盖茨比和身份验证的知识 。
Gatsby's documentation was extremely helpful in writing this post, as was the Gatsby + Netlify CMS Starter.
Gatsby的文档和Gatsby + Netlify CMS Starter一样,对撰写本文非常有帮助。
To see how the Okta Sign-In Widget can be customized, check out developer.okta.com/live-widget.
要查看如何自定义Okta登录小部件,请访问developer.okta.com/live-widget 。
To learn more about Netlify, React, OAuth 2.0, and OIDC, I recommend some of our other blog posts:
要了解有关Netlify,React,OAuth 2.0和OIDC的更多信息,我推荐一些其他博客文章:
- How to Configure Better Web Site Security with Cloudflare and Netlify 如何使用Cloudflare和Netlify配置更好的网站安全性
- Simple User Authentication in React React中的简单用户身份验证
- Build a Basic CRUD App with Node and React 使用Node和React构建一个基本的CRUD应用
- Create a React Native App with Login in 10 Minutes 在10分钟内登录创建一个React Native应用
- An Illustrated Guide to OAuth and OpenID Connect OAuth和OpenID Connect图解指南
If you liked this tutorial, please follow @oktadev on Twitter. We also like to do screencasts and post them to our YouTube channel. If you have any questions, I'd be happy to answer them in the comments below. 🙂
如果您喜欢本教程,请在Twitter上关注@oktadev 。 我们还喜欢进行截屏视频并将其发布到我们的YouTube频道 。 如果您有任何疑问,我们很乐意在下面的评论中回答。 🙂
翻译自: https://scotch.io/tutorials/get-your-jam-on-with-gatsby-react-and-netlify
gatsby