ssh keep-live
为什么要为持续集成而烦恼 ( Why Bother About Continuous Integration )
Continuous Integration (CI) is a development practice that enables teams to rapidly build, test and deploy while at the same time detecting problems as early as possible.
持续集成(CI)是一种开发实践,使团队能够快速构建,测试和部署,同时尽早发现问题。
Some of the most popular CI tools are
一些最受欢迎的CI工具是
The final decision on what CI tool to use entirely depends on your team's requirements. Most of these tools provide support to:
关于完全使用哪种CI工具的最终决定取决于您团队的要求。 这些工具大多数都为以下方面提供支持:
- Quickly build code from Github using hooks. 使用钩子从Github快速构建代码。
- Create an isolated environment, install all the dependencies and trigger other services such as tests through a
circle.yml
file. 创建一个隔离的环境,安装所有依赖项,并通过circle.yml
文件触发其他服务,例如测试。 - Run your tests. 运行测试。
- Secure remote access to you application to quickly debug without making unnecessary commits to your application repository. 保护对应用程序的远程访问,以快速调试,而无需对应用程序存储库进行不必要的提交。
- Quickly deploy to hosting services such as Heroku, Amazon AWS and Google Cloud Platform 快速部署到托管服务,例如Heroku,Amazon AWS和Google Cloud Platform
SSH访问调试简介 ( Introduction To Debugging With SSH Access )
More often than not, your code will fail, and it is bound to fail. With your CI tool of choice, you may quickly identify and fix such bugs before pushing your code into production.
通常,您的代码会失败,并且注定会失败。 使用您选择的CI工具,您可以在将代码投入生产之前快速识别并修复此类错误。
But once it fails, how do you fix it?
但是一旦失败,您如何解决?
Now there are a number of ways to go about fixing your errors depending on the bug's complexity and urgency. We will work under the assumption that your team lead just assigned the error to you as a Github issue.
现在,有很多方法可以根据错误的复杂性和紧急程度来修复错误。 我们将在假设您的团队领导刚刚将错误分配给您作为Github问题的前提下进行工作。
Do you create 5 to 10 new commits, push the code again and pray that the issue has been fixed, Circle CI passes and everyone goes home happy? Or do you find the cause of the error and make one commit that is sure to pass?
您是否创建5到10个新的提交,再次推送代码,并祈祷问题已解决,Circle CI通过并且每个人都回家感到高兴? 还是您找到错误的原因并确定要提交一次提交?
In this tutorial, we will be going through how to debug remotely in a CircleCI container build with SSH.
在本教程中,我们将介绍如何在使用SSH的CircleCI容器构建中进行远程调试。
为失败做好自己的准备 ( Setting Ourselves Up For Failure )
To understand how debugging via SSH works. We will have to cause havoc on a project. Not to worry, the project we will be breaking in this case will not crash any financial markets or cause an increase in the temperature of the earth's atmosphere.
了解如何通过SSH调试。 我们将不得不对一个项目造成破坏。 不用担心,在这种情况下,我们将要中断的项目不会破坏任何金融市场或导致地球大气温度升高。
该项目 (The Project)
We will be working with a simple node.js application that has one route that returns a 500 status code when a GET request is made to /
. Let's call it project-abc.
我们将使用一个简单的node.js应用程序,该应用程序具有一个路由,当对/
发出GET请求时,该路由返回500状态代码 。 我们称之为project-abc 。
/index.js
/index.js
'use strict';
var express = require('express'),
app = express();
app.get('/', function (req, res) {
res.status(500)
.send('Success is going from failure to failure without losing enthusiasm.');
});
app.set('port', process.env.PORT || 8080);
app.listen(app.get('port'), function () {
console.log('Magic happens on port', app.get('port'));
});
module.exports.app = app;
project-abc will also contain a single test that asserts that when a GET request is made to /
, a 200 status code is returned.
project-abc也将包含一个测试,该测试断言当对/
进行GET请求时,将返回200状态代码 。
/test/test.js
/test/test.js
'use strict';
var request = require('supertest'),
app = require('../index').app;
describe('GET /', function () {
it('Should return HTTP response with status code 200', function (done) {
request(app).get('/').expect(200, done);
});
});
While this is meant to fail, we will inspect the issue via CircleCI's secure SSH access, identify the bug, fix it remotely and run tests to ensure that it passes.
尽管这将失败,但我们将通过CircleCI的安全SSH访问检查问题,识别错误,进行远程修复并运行测试以确保通过。
This tutorial works under the assumption that you are comfortable intergrating a project on Github with CircleCI, running tests using mocha and testing HTTP requests using supertest. The test will still be easy to understand without prior knowledge of testing.
本教程的前提是您可以轻松地将Github上的项目与CircleCI集成在一起,使用mocha运行测试,并使用supertest测试HTTP请求。 如果没有测试的先验知识,则该测试仍将易于理解。
A simple circle.yml
file is also included in the repository to trigger the tests on CircleCI.
存储库中还包含一个简单的circle.yml
文件,以触发CircleCI上的测试。
test:
override:
- mocha
获取CircleCI构建容器信息 ( Obtaining The CircleCI Build Container Information )
If everything worked out correctly (and by correctly I mean everything failed) with the above set up, Our CircleCI build should have failed with the following error:
如果通过以上设置正确完成所有工作(正确地说,我的意思是全部失败),那么我们的CircleCI构建应该已失败,并出现以下错误:

Perfect! Things are failing!
完善! 事情失败了!
In the next step we will go straight ahead to find the bug directly on the Isolated container that Circle CI Spins up for our project. On your CircleCI dashboard, go to the Debug via SSH section and click on Retry this build with SSH enabled
在下一步中,我们将直接在Circle CI为我们的项目旋转的Isolated容器中直接找到错误。 在CircleCI仪表板上,转到“ 通过SSH调试”部分,然后单击“在启用SSH的情况下重试此版本”

The rebuild works in the same way that a normal build would run with the exception that no deployment will take place. This is essential for us because more often than not, the reason for us remotely accessing the build via SSH is because something went wrong, and we would not like to push our failing tests to Heroku or Amazon AWS.
重建的运行方式与正常构建的运行方式相同,不同之处在于不会进行任何部署。 这对我们至关重要,因为我们经常通过SSH远程访问内部版本的原因是因为出现了问题,并且我们不希望将失败的测试推送到Heroku或Amazon AWS。
The build VM will remain available for 30 minutes after the build finishes running and then automatically shut down. (Or you can cancel it.)
构建完成运行后,构建VM将保持可用状态30分钟,然后自动关闭。 (或者您可以取消它。)
A succesfull rebuild will present you with a load of information that will enable us to remotely SSH into our Ubuntu container.
一次成功的重建将为您提供大量信息,这些信息将使我们能够远程SSH到我们的Ubuntu容器中。
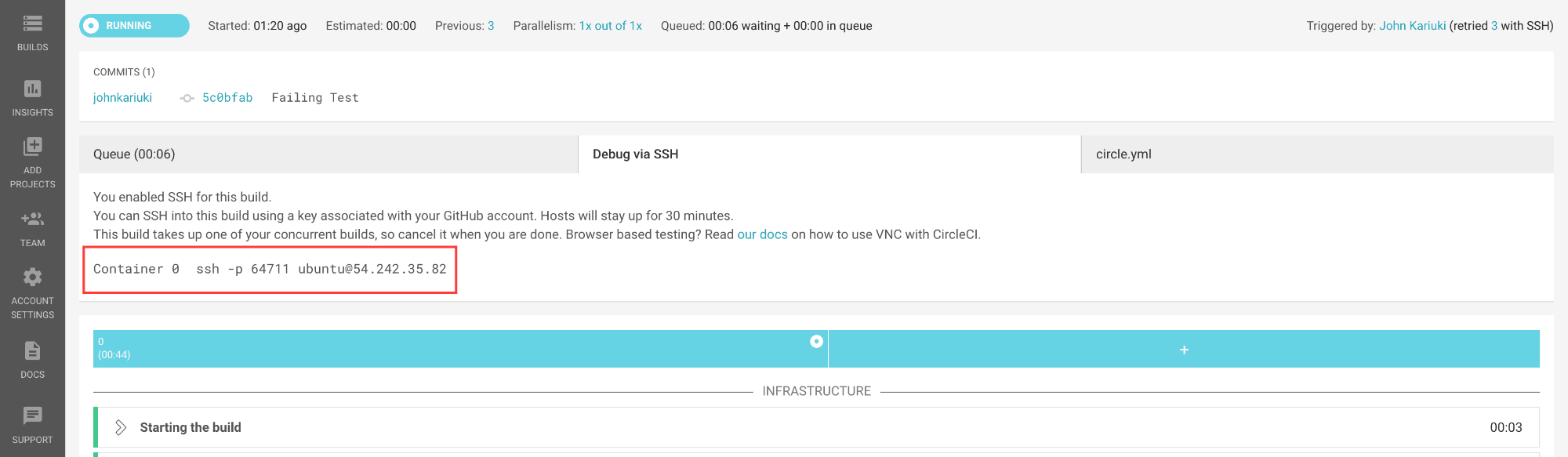
在终端上访问CircleCI构建容器 ( Accessing The CircleCI Build Container On The Terminal )
集装箱信息 (The Container Information)
With the Container Information details ready and good to go, you can simply copy and paste the container details on your terminal, In my case this would be ssh -p 64711 ubuntu@54.242.35.82
.
有了容器信息详细信息并且可以使用之后,您可以简单地将容器详细信息复制并粘贴到终端上,对于我来说,这是ssh -p 64711 ubuntu@54.242.35.82
。
This contains:
其中包含:
- The ssh command. ssh命令。
- The port to connect to. 要连接的端口。
- The unique IP to the Ubuntu container. Ubuntu容器的唯一IP。
Note that at this point, you need to have set up your SSH keys . You can confirm by running:
请注意,此时,您需要设置SSH密钥 。 您可以通过运行以下命令进行确认:
ssh git@github.com
深入了解容器环境 (Getting Inside The Container Environment)
Once you have setup the permissions and run the ssh
command from Github, you will be presented with remote access to your container.
一旦设置了权限并从Github运行ssh
命令,系统将为您提供对容器的远程访问。
This means that we can run commands such as listing directory details, changing directories and even opening files (with vim
of course).
这意味着我们可以运行命令,例如列出目录详细信息,更改目录甚至打开文件(当然使用vim
)。
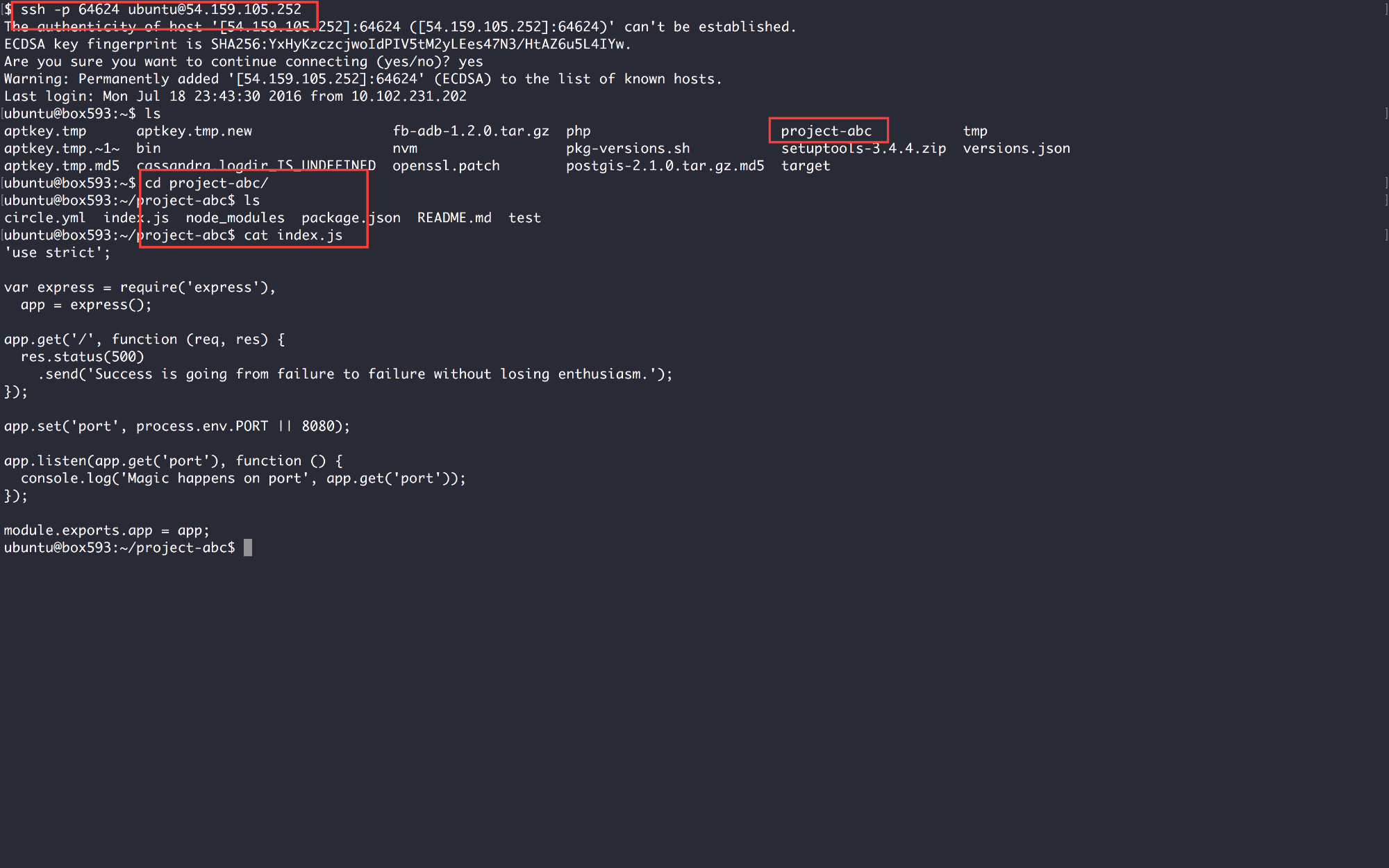
As you can see, I am able to see the project-abc directory, move into it and see the file contents. How cool is that?
如您所见,我能够看到project-abc目录,将其移至其中并查看文件内容。 多么酷啊?
And it gets better.
而且会变得更好。
As we had said earlier, the CI tools provide you with an isolated environment that runs your application. The reason CircleCI is able to run tests is because it installs all the node packages from package.json
and runs the test command mocha
from the circle.yml file.
正如我们之前所说,CI工具为您提供了一个运行您的应用程序的隔离环境。 CircleCI之所以能够运行测试,是因为它从package.json
安装了所有节点程序包,并从circle.yml文件运行了测试命令mocha
。
This means that I have access to the npm command and can therefore install packages directly on the build. So let's go ahead and install mocha
globally and run our test.
这意味着我可以访问npm命令,因此可以直接在构建中安装软件包。 因此,让我们继续在全球范围内安装mocha
并进行测试。

There goes our good old failing test. Now let's fix it.
我们的旧测试失败了。 现在修复它。
调试容器中的错误 ( Debugging The Error In the Container )
Now that we can see the failing test, we can go and assess the situation on the necessary script and get to the root of this matter.
现在我们可以看到失败的测试,我们可以在必要的脚本上评估情况,并找到问题的根源。
As it turns out, the test expects a 200 status code but received a 500 status code. This means that we may be returning the wrong status in our index.js. Let's go fix that. This video provides a quick run through what we just discussed earlier as well as fixing the error to correct the test.
事实证明,测试期望状态码为200,但收到状态码为500。 这意味着我们可能在index.js中返回了错误的状态。 让我们去解决它。 该视频简要介绍了我们前面讨论的内容,并修复了错误以更正测试。
https://www.youtube.com/watch?v=CkUyMbS7PvA
https://www.youtube.com/watch?v=CkUyMbS7PvA
As you can see. We managed to do all the following without making a single commit to our Github repository.
如你看到的。 我们设法完成了所有以下操作,而没有对我们的Github存储库进行任何提交。
- Open the file using vim. 使用vim打开文件。
- Edit the status code from 500 to 200. 将状态码从500修改为200。
- Save the file and re-run the test 保存文件并重新运行测试
With this, It will now be easy for us to go directly to the issue in our project and make a foolproof bug fix and push code with an assured pass on all our tests.
这样,我们现在可以很容易地直接进入项目中的问题并进行可靠的错误修复,并通过所有测试的确定通过来推送代码。
结论 ( Conclusion )
While this was a small case study of how we can remotely debug and tinker with a build on CircleCI, this should set the pace of the amazing things you can do in your container.
尽管这是一个小案例研究,说明了我们如何在CircleCI上进行构建的远程调试和修补,但是这应该确定您可以在容器中完成的惊人工作的速度。
I have provided the link to the Github repo here if you would like to play around with the sample project. I have also found the CircleCI documentation quite helpful in getting my way around this topic and many more.
我所提供的链接到GitHub库在这里 ,如果你想玩弄示例项目。 我还发现CircleCI文档对于解决该主题以及其他问题很有帮助。
翻译自: https://scotch.io/tutorials/continuous-integration-debugging-live-circleci-builds-with-ssh-access
ssh keep-live