reactjs使用百度地图
React is enabling frontend developers to build apps like never before. It's benefits are many: one-way data flow, easy component lifecycle methods, declarative components and more.
React使前端开发人员能够构建前所未有的应用程序。 它的好处很多:单向数据流,简单的组件生命周期方法,声明性组件等等。
Reapp was recently released on React. It's a mobile app platform designed for performance and productivity. Think of it as a well-optimized UI kit, along with a build system and a bunch of helpers that let you build apps easily.
Reapp最近在React上发布了。 这是一个旨在提高性能和生产力的移动应用程序平台。 可以将其视为经过优化的UI套件,以及构建系统和大量帮助程序,可让您轻松构建应用程序。
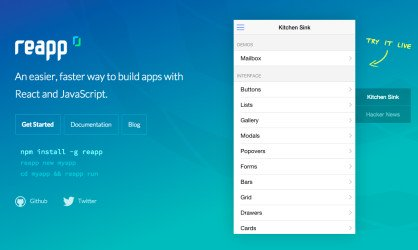
Reapp gives us some nice things out of the box:
Reapp提供了一些开箱即用的好东西:
- A complete UI kit for mobile 完整的手机版UI套件
- "reapp new" to generate a working app “重新应用”以生成可运行的应用
- "reapp run" to serve our app with ES6 and hot reloading “重新运行”通过ES6和热重载为我们的应用提供服务
- Themes and animations 主题和动画
- Routing and requests packages 路由和请求包
- Building our app to Cordova 将我们的应用程序构建到Cordova
我们将要建设的 (What we'll be building)
To explore using Reapp we're going to build an app that lets you search with the Flickr API and view the results in a photo gallery. This tutorial should take you less than half an hour to follow along with!
为了使用Reapp进行探索,我们将构建一个应用程序,让您使用Flickr API进行搜索并在图库中查看结果。 本教程将花费您不到半小时的时间!

起步 (Starting out)
With node installed, lets run sudo npm install -g reapp
to install the Reapp CLI. Once that installs, run reapp new flickrapp
. Finally, cd flickrapp
and reapp run
.
安装了节点后,让我们运行sudo npm install -g reapp
来安装Reapp CLI。 安装完成后,运行reapp new flickrapp
。 最后, cd flickrapp
并reapp run
。
You should see this:
您应该看到以下内容:

Browse to localhost:3010 and you can see the default Reapp app:
浏览到localhost:3010 ,您可以看到默认的Reapp应用程序:

Tip: With Chrome's Developer Tools, enable mobile device emulation to view your app as a mobile app
提示:使用Chrome的开发人员工具, 启用移动设备仿真可将您的应用视为移动应用

Alright! Now we're fully set up with a React stack using Reapp components. Lets check the file structure:
好的! 现在,我们已经使用Reapp组件完全设置了React堆栈。 让我们检查文件结构:
/app
components/
home/
Sub.jsx
App.jsx
Home.jsx
app.js
routes.js
/assets
Reapp scaffolded us some demonstration stuff here, which is what you see in . app/components
. The rest is just setting up our app. ./app/app.js
is the entry to our app, it loads Reapp and runs our routes, which are found in ./app/routes.js
.
Reapp在这里为我们提供了一些示范性的东西,这就是您所看到的. app/components
. app/components
。 剩下的只是设置我们的应用程序。 ./app/app.js
是我们应用程序的入口,它加载Reapp并运行我们的路线,这些路线位于./app/routes.js
。
开始我们的看法 (Start Our View)
We have our app generated, but Reapp generates us a full demo app showing nested views, and we won't need much more than a single page. Lets simplify things. In routes.js
we can swap it out to just look like this:
我们已经生成了我们的应用程序,但是Reapp生成了一个完整的演示应用程序,它显示了嵌套视图,我们只需要一个页面就可以了。 让我们简化一下。 在routes.js
我们可以将其换成如下所示:
module.exports = ({ routes, route }) =>
routes(require,
route('app', '/', { dir: '' })
);
This wires up the base route (at http://localhost:3010) to the name app
, which Reapp's router will automatically look for in ./components/App.jsx
.
这会将基本路由(位于http:// localhost:3010 )连接到名称app
,Reapp的路由器将自动在./components/App.jsx
查找该./components/App.jsx
。
Now we can delete the Home.jsx
and home/Sub.jsx
files, since we don't need multiple views. You can leave them be as well if you'd like to explore using them later.
现在我们可以删除Home.jsx
和home/Sub.jsx
文件,因为我们不需要多个视图。 如果您以后想使用它们,也可以保留它们。
In the App.jsx
file, we can simplify it to:
在App.jsx
文件中,我们可以将其简化为:
import React from 'react';
import View from 'reapp-ui/views/View';
export default React.createClass({
render() {
var { photos } = this.state;
return (
<View title="Flickr Search" styles={{ inner: { padding: 20 } }}>
<p>Hello World</p>
</View>
);
}
});
If you refresh, you should see an empty view with your new title "Flickr Search" at top.
如果您刷新,应该会看到一个空白视图,顶部带有新标题“ Flickr搜索”。
从Flickr获取数据 (Fetch Data from Flickr)
Now we have an interface with no logic. Before we can link together the Button to the display of photos, we need to grab the photos from Flickr using React conventions. First, get yourself a Flickr account and API key using their quick sign up form.
现在我们有了一个没有逻辑的接口。 在将Button链接到照片显示之前,我们需要使用React约定从Flickr中获取照片。 首先,使用快速注册表格为其获取Flickr帐户和API密钥。
After filling it out (and signing up if necessary) copy the Public Key they give you and add it as a constant to App.jsx
. You'll also need the URL that's used for searching for photos, which I found by using their [API explorer](https://www.flickr.com/services/api explore/flickr.photos.search).
填写完(并在必要时签名)后,复制他们给您的公钥并将其作为常量添加到App.jsx
。 您还需要用于搜索照片的URL,我通过使用其[API资源管理器](https://www.flickr.com/services/apiexplor/flickr.photos.search)找到了该URL。
It should look like this:
它看起来应该像这样:
const key = '__YOUR_KEY_HERE__';
const base = 'https://api.flickr.com/services/rest/?api_key=${key}&format=rest&format=json&nojsoncallback=1';
Be sure to put your key instead of "YOUR_KEY_HERE".
确保输入密钥而不是“ YOUR_KEY_HERE ”。
Note: const
is a new feature in the next version of JavaScript, called ES6. It's just like a variable, but one that can never be changed once it's set. How can we use this in our app now? Reapp has a Webpack build system built in that gives you all sorts of features, including ES6 support!
注意: const
是JavaScript下一版本ES6中的一项新功能。 它就像一个变量,但是一旦设置便永远无法更改。 我们现在如何在我们的应用程序中使用它? Reapp具有内置的Webpack构建系统,可为您提供各种功能,包括ES6支持!
Next, define getInitialState()
on our React class, so our component can track the photos we'll be fetching. We add this as the first property after React.createClass
. Because we're storing photos in a list, add an array:
接下来,在我们的React类上定义getInitialState()
,以便我们的组件可以跟踪将要获取的照片。 我们将此添加为React.createClass
之后的第一个属性。 由于我们将照片存储在列表中,因此添加一个数组:
getInitialState() {
return {
photos: []
}
},
This will give us access to this.state.photos
in our render function. In the UI we'll need a Button and Input to use for searching:
这将使我们能够访问渲染函数中的this.state.photos
。 在UI中,我们需要一个Button和Input来进行搜索:
import Button from 'reapp-ui/components/Button';
import Input from 'reapp-ui/components/Input';
And then change the render()
function:
然后更改render()
函数:
render() {
var { photos } = this.state;
return (
<View title="Flickr Search">
<Input ref="search img-responsive" />
<Button onTap={this.handleSearch}>Search Images</Button>
<div className="verticalCenter">
{!photos.length &&
<p>No photos!</p>
}
</div>
</View>
);
}
And we get this:
我们得到这个:

Pretty easy! There's a few things to note here. First, notice the ref property on the Input? Ref is short for reference, and lets us track DOM elements in our class. We'll use that in the future for getting the value of the field.
挺容易! 这里有几件事要注意。 首先,注意Input上的ref属性吗? Ref是参考的简称,它使我们可以跟踪类中的DOM元素。 将来我们将使用它来获取字段的价值。
Also, note className="verticalCenter"
on the div. Two things: Because we're using JSX that compiles to JS objects ([more reading here](http://facebook.github.io react/docs/jsx-in-depth.html)), we can't use the normal class
attribute, so instead use use the JavaScript convention of className
to set the class. The verticalCenter
property is given to us by Reapp, that will align things centered on our page.
另外,请注意div上的className="verticalCenter"
。 两件事:因为我们使用的是可编译为JS对象的JSX([更多阅读此处](http://facebook.github.io react / docs / js / in-depth.html)),所以我们不能使用普通的class
属性,因此请改用className
JavaScript约定来设置类。 Reapp提供了verticalCenter
属性,该属性将使页面中心的内容对齐。
Finally, the onTap
property on Button? It's pointing to this.handleSearch
. But, we don't have any handleSearch function. React will expect that function defined on the class, so lets wire it up. First, npm install --save superagent
which gives us the excellent Superagent request library. Then, import it:
最后,Button的onTap
属性? 它指向this.handleSearch
。 但是,我们没有任何handleSearch函数。 React将期望在类中定义该函数,因此让其连接起来。 首先, npm install --save superagent
,它为我们提供了出色的Superagent请求库。 然后,将其导入:
import Superagent from 'superagent';
Finally, define handleSearch:
最后,定义handleSearch:
handleSearch() {
let searchText = this.refs.search.getDOMNode().value;
Superagent
.get(`${base}&method=flickr.photos.search&text=${searchText}&per_page=10&page=1`, res => {
if (res.status === 200 && res.body.photos)
this.setState({
photos: res.body.photos.photo
});
});
},
A few notes:
一些注意事项:
this.refs.search.getDOMNode()
returns the input DOM node that we put the "search" ref on earlier.this.refs.search.getDOMNode()
返回输入的DOM节点,我们之前将其放置在“搜索”参考上。${base}
will grab the URL we put in the constant.${base}
将获取我们放入常量中的URL。this.setState
will take our response photos and put them into thethis.state.photos
array we defined earlier ingetInitialState
.this.setState
将获取我们的响应照片并将其放入我们之前在getInitialState
定义的this.state.photos
数组中。
显示Flickr照片 (Displaying Flickr Photos)
Now we've fetched our Flickr photos and put them into the state. The last step is to display them. You can add this to the first line of your render function to see what Flickr returns:
现在,我们已经获取了Flickr照片,并将其置于状态。 最后一步是显示它们。 您可以将其添加到渲染函数的第一行,以查看Flickr返回的内容:
render() {
console.log(this.state.photos);
// ... rest of render
}
In your console you'll see that Flickr returns an object with some properties. On this helpful page I found out how to render the URL's for flickr.
在控制台中,您将看到Flickr返回具有某些属性的对象。 在这个有用的页面上,我了解了如何为flickr呈现URL。
Here's how I landed on constructing the URL for a photo, which I put as a simple function on the class we're building:
这是我为照片构建URL的方式,将其作为简单函数放在要构建的类中:
getFlickrPhotoUrl(image) {
return `https://farm${image.farm}.staticflickr.com/${image.server}/${image.id}_${image.secret}.jpg`;
},
This function takes our Flickr object and turns them into the URL we need to display. Next, lets edit the handleSearch setState
call:
此函数获取Flickr对象,并将其转换为我们需要显示的URL。 接下来,让我们编辑handleSearch setState
调用:
this.setState({
photos: res.body.photos.photo.map(this.getFlickrPhotoUrl)
});
The map
function will loop over those photo objects and pass them to getFlickrPhotoUrl, which returns our URL. We're all ready to display them!
map
函数将遍历这些照片对象,并将它们传递给getFlickrPhotoUrl,后者将返回我们的URL。 我们已经准备好展示它们!
Lets import the Gallery component from reapp and use it:
让我们从reapp导入Gallery组件并使用它:
import Gallery from 'reapp-ui/components/Gallery';
In the render function, below the <p>No photos found!</p>
block:
在渲染功能的<p>No photos found!</p>
块下面:
{!!photos.length &&
<Gallery
images={photos}
width={window.innerWidth}
height={window.innerHeight - 44}
/>
}
The Gallery widget takes these three properties and outputs fullscreen images that you can swipe between. With this in place, we have completed the flow of our app. Check out your browser and see it in action.
Gallery小部件具有这三个属性,并输出可在其间滑动的全屏图像。 有了这个,我们已经完成了我们的应用程序流程。 签出您的浏览器并查看其运行情况。
Note: Why window.innerHeigth - 44
? We're adjusting for the TitleBar height in our app. There are better ways we could do this, but for now this is simple and works well
注意:为什么选择window.innerHeigth - 44
? 我们正在为应用程序中的TitleBar高度进行调整。 我们有更好的方法可以做到这一点,但是现在这很简单并且效果很好
最后的润色 (Final touches)
We're just about good, but there's a couple tweaks we can do. The gallery never lets us close it as it is now. If we add an onClose property to gallery though, it will let us. But, we'll also need to update the state to reflect the gallery being closed. It's actually pretty easy. Just add this to Gallery:
我们差不多了,但是我们可以做一些调整。 画廊从不让我们现在关闭它。 但是,如果我们向画廊添加一个onClose属性,它将允许我们。 但是,我们还需要更新状态以反映图库已关闭。 实际上很简单。 只需将其添加到图库:
onClose={() => this.setState({ photos: [] })}
Also, our Input looks a little plain as it is. Lets add a border, margin and placeholder:
同样,我们的输入看起来仍然很简单。 让我们添加边框,边距和占位符:
<Input ref="search" placeholder="Enter your search" styles={{
input: {
margin: '0 0 10px 0',
border: '1px solid #ddd'
}
}} />


Much better!
好多了!
最终代码 (Final code)
As is, our entire codebase fits into the ./components/App.jsx
file. It's easy to read and understand and uses some nice new features of ES6. Here it is:
./components/App.jsx
,我们的整个代码库都适合于./components/App.jsx
文件。 它易于阅读和理解,并使用ES6的一些不错的新功能。 这里是:
import React from 'react';
import View from 'reapp-ui/views/View';
import Button from 'reapp-ui/components/Button';
import Input from 'reapp-ui/components/Input';
import Superagent from 'superagent';
import Gallery from 'reapp-ui/components/Gallery';
const MY_KEY = '__YOUR_KEY_HERE__';
const base = `https://api.flickr.com/services/rest/?api_key=${MY_KEY}&format=rest&format=json&nojsoncallback=1`;
export default React.createClass({
getInitialState() {
return {
photos: []
}
},
// see: https://www.flickr.com/services/api/misc.urls.html
getFlickrPhotoUrl(image) {
return `https://farm${image.farm}.staticflickr.com/${image.server}/${image.id}_${image.secret}.jpg`;
},
handleSearch() {
let searchText = this.refs.search.getDOMNode().value;
Superagent
.get(`${base}&method=flickr.photos.search&text=${searchText}&per_page=10&page=1`, res => {
if (res.status === 200 && res.body.photos)
this.setState({
photos: res.body.photos.photo.map(this.getFlickrPhotoUrl)
});
});
},
render() {
var { photos } = this.state;
return (
<View title="Flickr Search" styles={{ inner: { padding: 20 } }}>
<Input ref="search" placeholder="Enter your search" styles={{
input: {
margin: '0 0 10px 0',
border: '1px solid #ddd'
}
}} />
<Button onTap={this.handleSearch}>Search Images</Button>
<div className="verticalCenter">
{!photos.length &&
<p>No photos!</p>
}
{!!photos.length &&
<Gallery
onClose={() => this.setState({ photos: [] })}
images={photos}
width={window.innerWidth}
height={window.innerHeight - 44}
/>
}
</div>
</View>
);
}
});
下一步 (Next steps)
We could keep going from here. We could display a list of images before, and link them to the gallery. Reapp also has docs on it's components, so you can browse and add them as you need. Good examples of Reapp code include the Kitchen Sink demo and the Hacker News App they built.
我们可以继续从这里走。 我们可以在之前显示图像列表,并将其链接到图库。 Reapp 的组件上也有文档 ,因此您可以根据需要浏览并添加它们。 Reapp代码的好例子包括Kitchen Sink演示和他们构建的Hacker News App 。
签出代码 (Check out the code)
If you'd like to see this application's code you can clone this repo. It includes everything you need except a Flickr API key, which you'll want to sign up for and insert before testing it out.
如果您想查看此应用程序的代码,可以克隆此repo 。 它包含除Flickr API密钥以外的所有所需功能,您需要先注册并插入Flickr API密钥,然后再进行测试。
Steps to get the repo running:
使仓库运行的步骤:
- Install Node/npm, and Reapp:
sudo npm install -g reapp
安装Node / npm ,然后重新sudo npm install -g reapp
:sudo npm install -g reapp
- Clone the repo:
git clone git@github.com:reapp/flickr-demo
克隆git clone git@github.com:reapp/flickr-demo
:git clone git@github.com:reapp/flickr-demo
- Install dependencies:
npm install
安装依赖项:npm install
- Start server:
reapp run
启动服务器:reapp run
- View it in your browser at http://localhost:3010 在浏览器中的http:// localhost:3010上查看
You'll probably want to explore the Reapp getting started docs and the individual UI widgets docs to keep you going.
您可能需要探索Reapp入门文档和各个UI小部件文档,以使您继续前进。
Happy hacking!
骇客骇客!
翻译自: https://scotch.io/tutorials/make-a-mobile-app-with-reactjs-in-30-minutes
reactjs使用百度地图