mootools
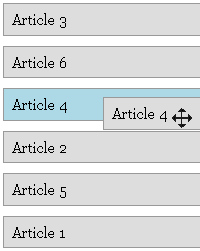
One of my most popular posts has been Using MooTools 1.2 for Drag, Drop, Sort, Save. My post detailed how you can create a drag'n'drop, AJAX-ified system to allow the user to drag and drop elements and quickly save them with PHP and MySQL on the server side. I've chosen to update the post with a faster, more efficient set of MooTools and PHP code. I've also provided a jQuery equivalent. Enjoy!
我最受欢迎的文章之一是使用MooTools 1.2进行拖放,排序,保存 。 我的帖子详细介绍了如何创建AJAX拖放式系统,以允许用户拖放元素并在服务器端使用PHP和MySQL快速保存它们。 我选择用一组更快,更有效的MooTools和PHP代码来更新帖子。 我还提供了jQuery等效项。 请享用!
MySQL表 (The MySQL Table)
id | title | sort_order |
---|---|---|
1 | Article 1 | 1 |
2 | Article 2 | 2 |
3 | Article 3 | 3 |
4 | Article 4 | 4 |
5 | Article 5 | 5 |
6 | Article 6 | 6 |
ID | 标题 | 排序 |
---|---|---|
1个 | 第1条 | 1个 |
2 | 第二条 | 2 |
3 | 第三条 | 3 |
4 | 第4条 | 4 |
5 | 第5条 | 5 |
6 | 第6条 | 6 |
This table shows only the important fields per this functionality: ID, Title, and Sort Order. Your table will likely have many more columns.
下表仅显示此功能的重要字段:ID,标题和排序顺序。 您的表可能会有更多的列。
PHP / HTML列表构建 (The PHP / HTML List Build)
<?php
$query = 'SELECT id, title FROM test_table ORDER BY sort_order ASC';
$result = mysql_query($query,$connection) or die(mysql_error().': '.$query);
if(mysql_num_rows($result)) {
?>
<p>Drag and drop the elements below. The database gets updated on every drop.</p>
<div id="message-box"><?php echo $message; ?> Waiting for sortation submission...</div>
<form id="dd-form" action="<?php echo $_SERVER['REQUEST_URI']; ?>" method="post">
<p>
<input type="checkbox" value="1" name="autoSubmit" id="autoSubmit" <?php if($_POST['autoSubmit']) { echo 'checked="checked"'; } ?> />
<label for="autoSubmit">Automatically submit on drop event</label>
</p>
<ul id="sortable-list">
<?php
$order = array();
while($item = mysql_fetch_assoc($result)) {
echo '<li title="',$item['id'],'">',$item['title'],'</li>';
$order[] = $item['id'];
}
?>
</ul>
<br />
<input type="hidden" name="sort_order" id="sort_order" value="<?php echo implode(',',$order); ?>" />
<input type="submit" name="do_submit" value="Submit Sortation" class="button" />
</form>
<?php } else { ?>
<p>Sorry! There are no items in the system.</p>
<?php } ?>
We'll start out by querying the database to retrieve all records from the table. If there are no records available, we simply show a message saying so. Once we have established that records are available, we:
我们将从查询数据库开始以检索表中的所有记录开始。 如果没有可用的记录,我们仅显示一条消息。 建立记录可用后,我们:
- create a message box DIV that will notify users of the status of AJAX request actions. 创建一个消息框DIV,该消息框将通知用户AJAX请求操作的状态。
- create a form element. 创建一个表单元素。
- create an "auto-save" option checkbox that directs whether or not the sort order should be saved on every drag/drop. 创建一个“自动保存”选项复选框,该复选框指示是否应在每次拖放时保存排序顺序。
create a UL element that outputs the list of records in their current sort order. Each LI element has its ID temporarily stored in its HTML attribute. (For those of you who don't mind using custom element attributes, feel free to create a custom attribute to store the record ID).
创建一个UL元素,以其当前排序顺序输出记录列表。 每个LI元素的ID都临时存储在其HTML属性中。 (对于那些不介意使用自定义元素属性的人,可以随意创建一个自定义属性来存储记录ID) 。
- create a hidden INPUT element to dynamically store the current sort order. 创建一个隐藏的INPUT元素以动态存储当前的排序顺序。
- create a submit button that will work via AJAX or typical form submission. 创建一个可以通过AJAX或典型表单提交工作的提交按钮。
There's a lot of stuff going on here but it's all necessary to ensure positive user experience, maximal functionality, and reliability.
这里有很多事情要做,但是必须确保积极的用户体验,最大的功能和可靠性。
CSS (The CSS)
#sortable-list { padding:0; }
#sortable-list li { padding:4px 8px; color:#000; cursor:move; list-style:none; width:500px; background:#ddd; margin:10px 0; border:1px solid #999; }
#message-box { background:#fffea1; border:2px solid #fc0; padding:4px 8px; margin:0 0 14px 0; width:500px; }
None of the code here is required by, as always, you need to style your elements to fit your website. Since the drag and drop effect looks so cool, you're going to want to make your elements look cool too.
与往常一样,这里不需要任何代码,您需要样式化元素以适合您的网站。 由于拖放效果看起来很酷,因此您将要使元素看起来也很酷。
MooTools JavaScript (The MooTools JavaScript)
/* when the DOM is ready */
window.addEvent('domready', function() {
/* grab important elements */
var sortInput = document.id('sort_order');
var submit = document.id('autoSubmit');
var messageBox = document.id('message-box');
var list = document.id('sortable-list');
/* get the request object ready; re-use the same Request */
var request = new Request({
url: '<?php echo $_SERVER["REQUEST_URI"]; ?>',
link: 'cancel',
method: 'post',
onRequest: function() {
messageBox.set('text','Updating the sort order in the database.');
},
onSuccess: function() {
messageBox.set('text','Database has been updated.');
}
});
/* worker function */
var fnSubmit = function(save) {
var sortOrder = [];
list.getElements('li').each(function(li) {
sortOrder.push(li.retrieve('id'));
});
sortInput.value = sortOrder.join(',');
if(save) {
request.send('sort_order=' + sortInput.value + '&ajax=' + submit.checked + '&do_submit=1&byajax=1');
}
};
/* store values */
list.getElements('li').each(function(li) {
li.store('id',li.get('title')).set('title','');
});
/* sortables that also *may* */
new Sortables(list,{
constrain: true,
clone: true,
revert: true,
onComplete: function(el,clone) {
fnSubmit(submit.checked);
}
});
/* ajax form submission */
document.id('dd-form').addEvent('submit',function(e) {
if(e) e.stop();
fnSubmit(true);
});
});
The first step in the process is rounding up the list of key elements in the page. Then we create our Request instance which will be used for every AJAX request. Next we create fnSubmit the function that will round up the LI elements (records) and their sort order. Lastly, we create our Sortables instance and connect submission event to the form's submit button. When you split the pieces apart, the system is actually quite simple.
该过程的第一步是四舍五入页面中的关键元素列表。 然后,我们创建我们的Request实例,该实例将用于每个AJAX请求。 接下来,我们创建fnSubmit函数,该函数将对LI元素(记录)及其排序顺序进行取整。 最后,我们创建Sortables实例并将提交事件连接到表单的“提交”按钮。 当您将各个部分分开时,该系统实际上非常简单。
jQuery JavaScript (The jQuery JavaScript)
/* when the DOM is ready */
jQuery(document).ready(function() {
/* grab important elements */
var sortInput = jQuery('#sort_order');
var submit = jQuery('#autoSubmit');
var messageBox = jQuery('#message-box');
var list = jQuery('#sortable-list');
/* create requesting function to avoid duplicate code */
var request = function() {
jQuery.ajax({
beforeSend: function() {
messageBox.text('Updating the sort order in the database.');
},
complete: function() {
messageBox.text('Database has been updated.');
},
data: 'sort_order=' + sortInput[0].value + '&ajax=' + submit[0].checked + '&do_submit=1&byajax=1', //need [0]?
type: 'post',
url: '<?php echo $_SERVER["REQUEST_URI"]; ?>'
});
};
/* worker function */
var fnSubmit = function(save) {
var sortOrder = [];
list.children('li').each(function(){
sortOrder.push(jQuery(this).data('id'));
});
sortInput.val(sortOrder.join(','));
console.log(sortInput.val());
if(save) {
request();
}
};
/* store values */
list.children('li').each(function() {
var li = jQuery(this);
li.data('id',li.attr('title')).attr('title','');
});
/* sortables */
list.sortable({
opacity: 0.7,
update: function() {
fnSubmit(submit[0].checked);
}
});
list.disableSelection();
/* ajax form submission */
jQuery('#dd-form').bind('submit',function(e) {
if(e) e.preventDefault();
fnSubmit(true);
});
});
The jQuery code is surprisingly similar to the MooTools version. Please note that duplicating the jQuery functionality will require that you also download the jQuery UI library. It's a good chunk of extra code but the functionality works great.
jQuery代码惊人地类似于MooTools版本。 请注意,复制jQuery功能将需要您还下载jQuery UI库。 这是很多额外的代码,但是功能很好。
“标题” PHP / MySQL (The "Header" PHP/MySQL)
/* on form submission */
if(isset($_POST['do_submit'])) {
/* split the value of the sortation */
$ids = explode(',',$_POST['sort_order']);
/* run the update query for each id */
foreach($ids as $index=>$id) {
$id = (int) $id;
if($id != '') {
$query = 'UPDATE test_table SET sort_order = '.($index + 1).' WHERE id = '.$id;
$result = mysql_query($query,$connection) or die(mysql_error().': '.$query);
}
}
/* now what? */
if($_POST['byajax']) { die(); } else { $message = 'Sortation has been saved.'; }
}
The "header" or processing PHP file receives the sort order, splits the string apart by the comma delimiter, and executes queries to update the sort order. Since PHP's mysql_query function wont allow for more than one query at a time, queries need to be executed separately. If you use another MySQL/PHP library (PDO, etc.) you may want to append the queries to a single string and execute them all at once. Depending on the method by which the user submitted the update (AJAX or normal post), the PHP will either die out or reload the page per usual.
“标头”或处理中PHP文件接收排序顺序,用逗号定界符将字符串分开,并执行查询以更新排序顺序。 由于PHP的mysql_query函数一次不允许进行多个查询,因此查询需要单独执行。 如果您使用另一个MySQL / PHP库(PDO等),则可能需要将查询附加到单个字符串并立即执行所有查询。 根据用户提交更新的方法(AJAX或普通帖子),PHP将照常消失或重新加载页面。
My clients have always loved this feature within their CMS. Sorting records can be hugely important and allowing for an easy method by which to do so can make you look like a miracle worker.
我的客户一直喜欢在CMS中使用此功能。 对记录进行排序可能非常重要,并且允许使用简便的方法可以使您看起来像个奇迹般的工作者。
mootools