java 应用程序 gui
A GUI -- Graphical User Interface -- of an application built using Java is made up of layers of containers. The first layer is the window used to move the application around the screen of your computer. It is a top-level container that gives all other containers and graphical components a place to work in. For a desktop application, this top-level container is usually made using the JFrame class.
使用Java构建的应用程序的GUI( 图形用户界面 )由多个容器层组成。 第一层是用于在计算机屏幕上移动应用程序的窗口。 它是一个顶级容器,为所有其他容器和图形组件提供了工作的场所。对于桌面应用程序,通常使用JFrame类来制作此顶级容器。
背景 ( Background )
How many layers a GUI has depends on your design. You can place graphical components such as text boxes, labels, and buttons directly into the JFrame, or they can be grouped in other containers depending on how complex the application GUI needs to be.
GUI的层数取决于您的设计。 您可以将诸如文本框,标签和按钮之类的图形组件直接放置到JFrame中 ,也可以将它们组合在其他容器中,具体取决于应用程序GUI的复杂程度。
This sample code below shows how to build an application out of a JFrame, two JPanels and a JButton, which determines the visibility of the components held in the two JPanels. Follow along with what is happening in the code by reading the implementation comments, indicated by two slashes at the beginning of each comment line.
下面的示例代码显示了如何使用JFrame,两个JPanel和一个JButton构建应用程序,该应用程序确定两个JPanel中包含的组件的可见性。 通过阅读实现注释 ,跟踪代码中发生的一切,在每个注释行的开头用两个斜杠表示。
This code goes with the Coding a Simple Graphical User Interface - Part I step-by-step guide. It shows how to build an application out of a JFrame
, two JPanels
and JButton
. The button determines the visibility of the components held within the two JPanels
.
该代码与“ 编码简单图形用户界面-第一部分”逐步指南一起使用。 它显示了如何使用JFrame
,两个JPanels
和JButton
来构建应用程序。 该按钮确定两个JPanels
包含的组件的可见性。
Java代码 ( Java Code )
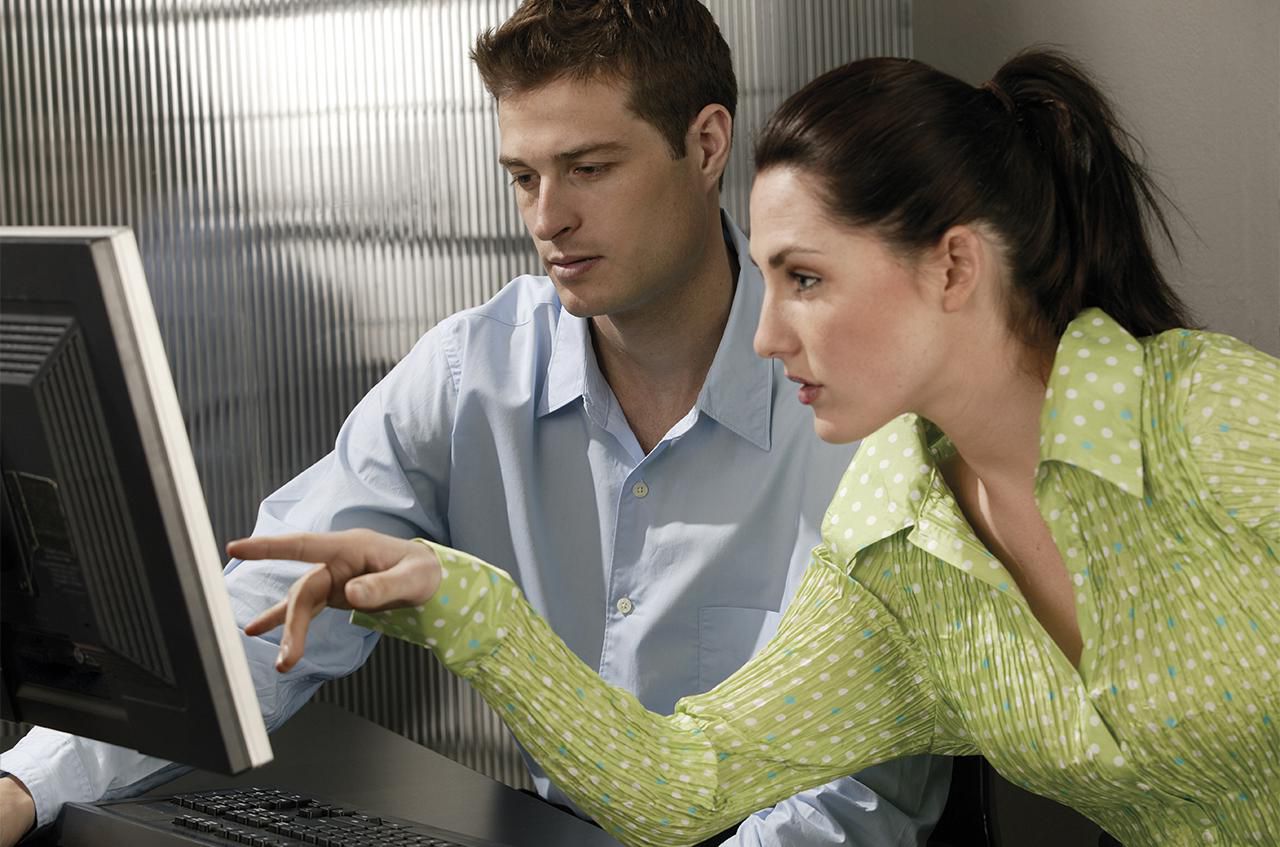
Compare this Java code with program listing generated from the Coding a Simple Graphical User Interface - Part II which uses the NetBeans GUI Builder to create the same GUI application.
将此Java代码与“ 编码简单图形用户界面-第二部分”生成的程序清单进行比较,该部分使用NetBeans GUI Builder创建相同的GUI应用程序。
//Imports are listed in full to show what's being used
//could just import javax.swing.* and java.awt.* etc..
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JComboBox;
import javax.swing.JButton;
import javax.swing.JLabel;
import javax.swing.JList;
import java.awt.BorderLayout;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
public class GuiApp1 {
//Note: Typically the main method will be in a
//separate class. As this is a simple one class
//example it's all in the one class.
public static void main(String[] args) {
new GuiApp1();
}
public GuiApp1()
{
JFrame guiFrame = new JFrame();
//make sure the program exits when the frame closes
guiFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
guiFrame.setTitle("Example GUI");
guiFrame.setSize(300,250);
//This will center the JFrame in the middle of the screen
guiFrame.setLocationRelativeTo(null);
//Options for the JComboBox
String[] fruitOptions = {"Apple", "Apricot", "Banana"
,"Cherry", "Date", "Kiwi", "Orange", "Pear", "Strawberry"};
//Options for the JList
String[] vegOptions = {"Asparagus", "Beans", "Broccoli", "Cabbage"
, "Carrot", "Celery", "Cucumber", "Leek", "Mushroom"
, "Pepper", "Radish", "Shallot", "Spinach", "Swede"
, "Turnip"};
//The first JPanel contains a JLabel and JCombobox
final JPanel comboPanel = new JPanel();
JLabel comboLbl = new JLabel("Fruits:");
JComboBox fruits = new JComboBox(fruitOptions);
comboPanel.add(comboLbl);
comboPanel.add(fruits);
//Create the second JPanel. Add a JLabel and JList and
//make use the JPanel is not visible.
final JPanel listPanel = new JPanel();
listPanel.setVisible(false);
JLabel listLbl = new JLabel("Vegetables:");
JList vegs = new JList(vegOptions);
vegs.setLayoutOrientation(JList.HORIZONTAL_WRAP);
listPanel.add(listLbl);
listPanel.add(vegs);
JButton vegFruitBut = new JButton( "Fruit or Veg");
//The ActionListener class is used to handle the
//event that happens when the user clicks the button.
//As there is not a lot that needs to happen we can
//define an anonymous inner class to make the code simpler.
vegFruitBut.addActionListener(new ActionListener()
{
@Override
public void actionPerformed(ActionEvent event)
{
//When the fruit of veg button is pressed
//the setVisible value of the listPanel and
//comboPanel is switched from true to
//value or vice versa.
listPanel.setVisible(!listPanel.isVisible());
comboPanel.setVisible(!comboPanel.isVisible());
}
});
//The JFrame uses the BorderLayout layout manager.
//Put the two JPanels and JButton in different areas.
guiFrame.add(comboPanel, BorderLayout.NORTH);
guiFrame.add(listPanel, BorderLayout.CENTER);
guiFrame.add(vegFruitBut,BorderLayout.SOUTH);
//make sure the JFrame is visible
guiFrame.setVisible(true);
}
}
翻译自: https://www.thoughtco.com/example-java-code-for-building-a-simple-gui-application-2034066
java 应用程序 gui