类的初始化结合实例的初始化
实例化和初始化方法 ( Instantiation and the Initialize Method )
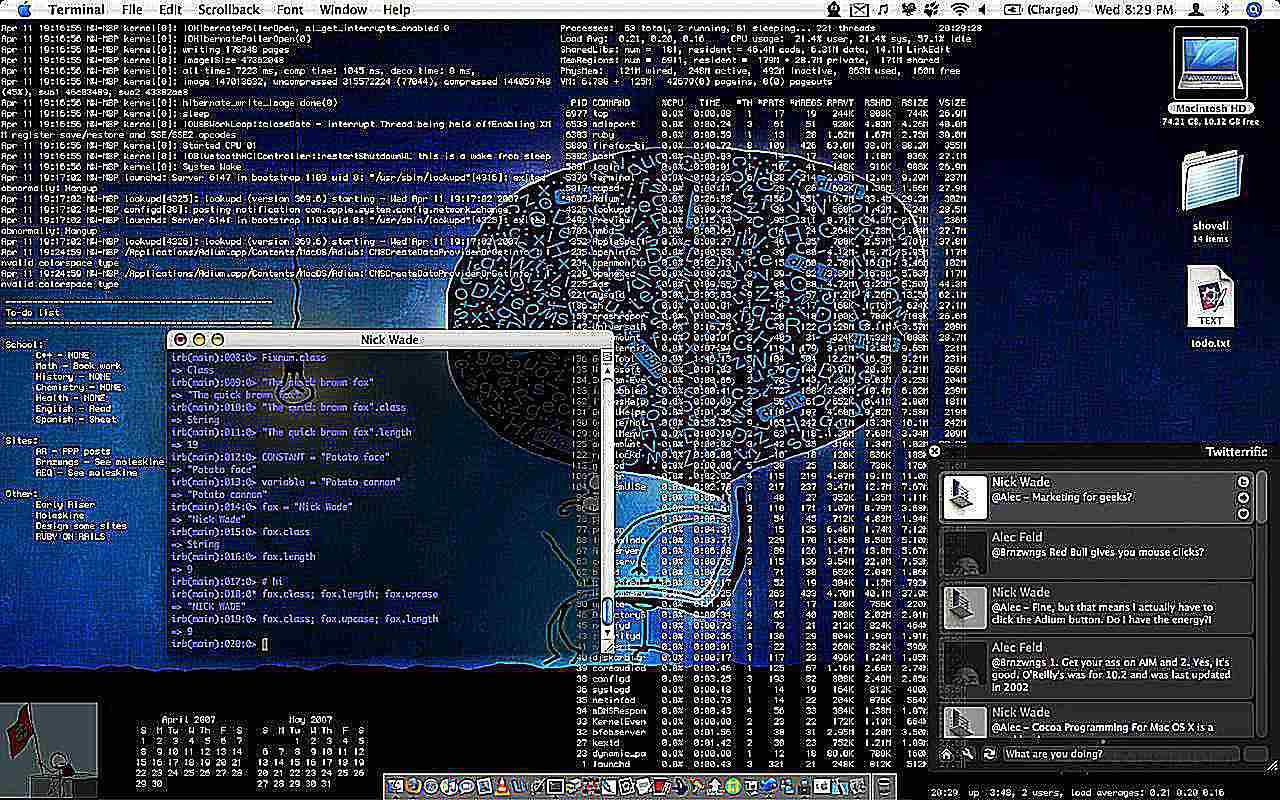
When you define a class in Ruby, Ruby will assign a new class object to the class name constant. For example, if you were to say class Person; end, this is roughly equivalent to Person = Class.new. This class object is of the type Class, and holds a number of methods useful for making instances of copies of those instances.
当您在Ruby中定义一个类时,Ruby会为该类名constant分配一个新的类对象。 例如,如果您要说人类。 最后 ,这大致相当于Person = Class.new 。 该类对象的类型为Class ,并包含许多用于制作这些实例副本的实例的方法。
Making Instances
To make a new instance of a class, call that class's new method. By default, this will allocate the required memory for the class and return a reference to the new object. So, if you were to make a new instance of the Person class, you would call Person.new.
While at first this seems a bit backwards, there is no new keyword in Ruby or any special syntax. New objects are created through a normal method that, all said and done, does relatively simple things.
Initializing Instances
A blank object is not very exciting. In order to start using your object, it must first be initialized (assuming it has any instance variables that needs initializing). This is done via the initialize method. Ruby will pass any arguments you pass to SomeClass.new on to initialize on the new object. You can then use normal variable assignments and methods to initialize the state of the object. In this example, a Person class is presented whose initialize method will take a name and age argument, and assign them to instance variables.
class Person def initialize(name, age) @name, @age = name, age end end bob = Person.new('Bob', 34)
You can also use this opportunity to acquire any resources you may need. Open network sockets, open files, read in any data you need, etc. The only caveat is that people generally don't expect initialize methods to fail. Be sure to document any possibly failing initialize methods thoroughly.
Destorying Objects
In general, you don't destroy objects in Ruby. If you're coming from C++ or another language without a garbage collector, this might seem strange. But in Ruby (and most other garbage collected languages), you don't destroy objects, you simply stop referring to it. On the next garbage collection cycle, any object without anything referring to it will be destroyed automatically. There are some bugs with circular references, but in general this works flawlessly and you don't even need a "destructor."
If you're wondering about resources, don't worry about it. When the object holding the resource is destroyed, the resource will be freed. Open files and network connections will be closed, memory deallocated etc. Only if you allocate any resources in a C extension will you really need to worry about deallocating resources. Though there is no guarantee when the garbage collector will be run. In order to deallocate resources in a timely manner, try to free them manually.
Making Copies of Objects
Ruby is pass by reference. If you pass a reference to an object to a method, and that method calls a method that modifies the state of that object, unintended consequences can occur. Further, methods can then save the reference to the object to modify at a much later time, causing a delayed effect for the bug. To avoid this, Ruby provides some methods to duplicate objects.
To duplicate any object, simply call the some_object.dup method. A new object will be allocated and all of the object's instance variables will be copied over. However, copying instance variables is what this was supposed to avoid: this is what's called a "shallow copy." If you were to hold a file in an instance variable, both of the duplicated objects would now be referring to the same file.
Just be aware that the copies are shallow copies before using the dup method. See the article Making Deep Copies in Ruby for more information.
制作实例
要创建类的新实例,请调用该类的new方法。 默认情况下,这将为该类分配所需的内存,并返回对新对象的引用。 因此,如果要创建Person类的新实例,则应调用Person.new 。
虽然起初看起来有些倒退,但是Ruby或任何特殊语法中都没有new关键字。 新对象是通过通常的方法创建的,该方法完成了所有相对简单的事情。
初始化实例
一个空白的对象不是很令人兴奋。 为了开始使用您的对象,必须首先对其进行初始化(假设它具有任何需要初始化的实例变量 )。 这是通过initialize方法完成的。 Ruby会将传递给SomeClass.new的所有参数传递给新对象进行初始化 。 然后,您可以使用常规变量分配和方法来初始化对象的状态。 在此示例中,显示了一个Person类,该类的initialize方法将使用name和age参数,并将它们分配给实例变量。
您还可以利用此机会获取您可能需要的任何资源。 打开网络套接字 ,打开文件,读入您需要的任何数据,等等。唯一的警告是,人们通常不希望初始化方法失败。 确保彻底记录任何可能失败的初始化方法。
目的地对象
通常,您不会破坏Ruby中的对象。 如果您来自C ++或其他没有垃圾收集器的语言,这似乎很奇怪。 但是在Ruby(以及大多数其他垃圾收集的语言)中,您不会破坏对象,而只是停止引用它。 在下一个垃圾回收周期中,任何没有任何引用的对象将被自动销毁。 有一些带有循环引用的错误,但是总的来说,它可以完美地工作,甚至不需要“析构函数”。
如果您想了解资源,请不必担心。 当持有资源的对象被破坏时,资源将被释放。 打开的文件和网络连接将被关闭,内存被释放等。仅当您在C扩展中分配任何资源时,您才真正需要担心释放资源。 尽管不能保证何时运行垃圾收集器。 为了及时释放资源,请尝试手动释放它们。
复制对象
Ruby是通过引用传递的。 如果将对对象的引用传递给method ,并且该方法调用修改该对象状态的方法,则可能会发生意料之外的后果。 此外,方法随后可以保存对对象的引用以在以后的很多时间进行修改,从而导致对该bug的延迟影响。 为了避免这种情况,Ruby提供了一些方法来复制对象。
要复制任何对象,只需调用some_object.dup方法。 将分配一个新对象,并将复制该对象的所有实例变量。 但是,应该避免复制实例变量:这就是所谓的“浅复制”。 如果要将文件保存在实例变量中,则两个重复的对象现在都将引用同一文件。
翻译自: https://www.thoughtco.com/instantiation-and-the-initialize-method-2908097
类的初始化结合实例的初始化