django前后端项目整合
为成功做与不做 (Dos and Don’ts For Success)
If you’ve been developing web applications for your company or a client for a few years, it’s possible you now find yourself with several individual Django projects that you’d like to consolidate. Each project might have one or a few apps with its own set of URL patterns, models, views, and more. This might be dizzying to think about, but with a few tips you can be well on your way to the lean, well-organized project you always dreamed of.
如果您几年来一直在为公司或客户开发Web应用程序,现在您可能会发现自己想合并几个Django项目。 每个项目可能都有一个或几个带有自己的URL模式,模型,视图等的应用程序。 考虑到这可能令人头晕,但是通过一些技巧,您可以顺利实现自己梦always以求的精益,组织良好的项目。
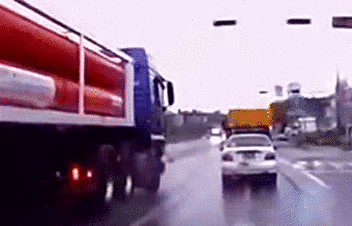
How not to do it
怎么不做
一般的做法 (General Approach)
The idea here will be to move your other projects’ applications into the consolidated project. This will be followed by merging and reducing project-level files, like settings.py
and requirements.txt
, while maintaining any application-level separation you want with modules like your views.py
and models.py
. This will “trim the fat” so there’s no duplicated code from the parent projects while making sure the resulting project is still manageably organized. Let’s get started!
这里的想法是将您其他项目的应用程序移到合并项目中。 接下来,将合并和减少项目级别的文件(例如settings.py
和requirements.txt
,同时保持与requirements.txt
应用程序级别的分离(例如, views.py
和models.py
。 这将“减轻负担”,因此在确保最终项目仍可管理地组织的同时,父项目不会有重复的代码。 让我们开始吧!
移动应用 (Moving an Application)
The first step for these migrations will be to move an existing application into your consolidated project. Assuming you have a Django project with an app called polls
, you might have a directory structure that looks like the following:
这些迁移的第一步将是将现有应用程序移至您的合并项目中。 假设您有一个包含名为polls
的应用程序的Django项目,则目录结构可能如下所示:
manage.py
manage.py
requirements.txt
requirements.txt
my_standalone_project/
my_standalone_project/
settings.py
settings.py
urls.py
urls.py
wsgi.py
wsgi.py
polls/
polls/
models.py
models.py
urls.py
urls.py
views.py
views.py
migrations/
migrations/
...
...
templates/
templates/
polls/
polls/
poll_detail.html
poll_detail.html
...
...
Everything under the polls/
directory is what you’ll move into the consolidated project. You can drag the folder into the project in your favorite GUI or IDE, or run something like:
polls/
目录下的所有内容都是您要移入合并项目的内容。 您可以将文件夹拖动到您喜欢的GUI或IDE中的项目中,或运行类似以下内容的文件:
$ mv my_standalone_project/polls/ /path/to/consolidated/project/
With the goal being that you move the entire polls/
folder into the project and not just its contents.
目的是将整个polls/
文件夹而不是仅将其内容移动到项目中。
Now that you’ve got the bulk of the application moved, the next step is to merge the supporting infrastructure from the parent project into the consolidated one.
现在您已经移动了大部分应用程序,下一步是将来自父项目的支持基础结构合并到合并的基础结构中。
settings.py
(settings.py
)
Each Django project ultimately needs just a single settings
module. Coming from n projects you’ll have n of these modules to contend with, so you’ll need to start by merging these. Depending on the complexity of your projects’ setups, this may be the most time-consuming piece. The goal here will be to end with a single module that contains all the settings from the parent applications’ settings modules.
每个Django项目最终都只需要一个settings
模块。 来自n个项目,您将要处理其中的n个模块,因此需要从合并这些模块开始。 根据项目设置的复杂程度,这可能是最耗时的工作。 这里的目标是以一个模块结束,该模块包含父应用程序的设置模块中的所有设置。
In many cases, settings will simply be duplicated across projects and can be pared down. Settings like USE_I18N
or TIME_ZONE
are typically identical. Simply mark these off in your parent projects and feel comfortable knowing they’re taken care of.
在许多情况下,设置只会在项目之间重复,并且可以简化。 USE_I18N
或TIME_ZONE
设置通常是相同的。 只需在您的父项目中将其标记出来,并知道它们已得到照顾就感到很自在。
More involved settings like DATABASES
and TEMPLATES
will need to be examined carefully. If you have multiple database instances you may need to re-instrument some of your code as outlined in Multiple Databases. You may want to consider migrating your data into a single database instance to avoid this altogether. With TEMPLATES
, you’ll want to make sure all template directories are accounted for from the parent projects. You’ll also want to verify that your templates are properly namespaced to the app they’re located in so that there aren’t any confusing name collisions when you try to {% include %}
them down the road!
诸如DATABASES
和TEMPLATES
类的更多涉及设置将需要仔细检查。 如果您有多个数据库实例,则可能需要重新插入一些代码,如“ 多个数据库”中所述 。 您可能要考虑将数据迁移到单个数据库实例中,以完全避免这种情况。 使用TEMPLATES
,您将要确保所有模板目录都来自父项目。 您还需要验证模板是否正确地命名了它们所在的应用程序的名称空间,以便在尝试{% include %}
时不会出现任何令人困惑的名称冲突!
The other settings to look out for will be any that third-party applications such as django-debug-toolbar
depend on. Follow the same approach as above, copying and pasting any settings that are novel to a particular application into the project’s settings and merging or resolving any duplicated settings.
需要注意的其他设置是第三方应用程序(例如django-debug-toolbar
依赖的任何设置。 遵循与上述相同的方法,将对特定应用程序而言新颖的所有设置复制并粘贴到项目的设置中,并合并或解决所有重复的设置。
The final step is to make sure that your newly-migrated application is listed in the project’s INSTALLED_APPS
setting. This is one I always overlook that isn’t always well-described in the resulting error!
最后一步是确保在项目的INSTALLED_APPS
设置中列出了新迁移的应用程序。 我总是忽略这一点,在产生的错误中并不能很好地描述这一点!
requirements.txt (requirements.txt)
The requirements for each of your original projects might be vastly different if the applications do vastly different things. One might have dependencies for managing image uploads, while another might have dependencies for talking to third-party APIs. In general this will work like merging your settings—you’ll need to contend with conflicting dependency versions by making sure all applications work on a single one of the specified versions. Now might also be a good time to make sure your dependencies are up-to-date!
如果应用程序做的事情大不相同,那么每个原始项目的要求可能会大不相同。 一个可能具有管理图像上载的依赖性,而另一个可能具有与第三方API对话的依赖性。 通常,这将像合并您的设置一样工作—您需要通过确保所有应用程序都在单个指定版本上运行来应对冲突的依赖版本。 现在可能也是确保您的依赖关系是最新的好时机!
urls.py (urls.py)
In order to maintain existing URL pattern matching, you’ll need to add your application to the top-level urls.py
so the project knows about its patterns. I’ve seen some approaches that result in new URLs because of the way the patterns are consolidated, but this is not necessary. It’s easy enough to maintain existing patterns by doing the following:
为了维持现有的URL模式匹配,您需要将您的应用程序添加到顶级urls.py
以便项目了解其模式。 由于模式的合并方式,我已经看到了一些产生新URL的方法,但这不是必需的。 只需执行以下操作即可轻松维护现有模式:
import polls
If you didn’t have a namespace
on your application in the old project, now is a great time to add one per the code sample above. If you are adding a namespace now, you’ll need to update any references to named URL patterns in reverse
calls and {% url %}
blocks. Namespacing is a great way to avoid name collisions, especially in large projects where two applications might have need for similarly-named views.
如果您在旧项目中的应用程序上没有namespace
,那么现在是时候根据上面的代码示例添加一个namespace
了。 如果现在要添加名称空间,则需要在reverse
调用和{% url %}
块中更新对命名URL模式的所有引用。 名称间隔是避免名称冲突的好方法,尤其是在大型项目中,两个应用程序可能需要使用名称相似的视图。
报废的东西 (Things to Scrap)
If you’ve made it this far, you may just be at a point where you can scrap what remains of the original project. Your remaining files should look something like this:
如果您已经做到了这一点,则可能只是在某个时候可以废弃原始项目的剩余内容。 您剩余的文件应如下所示:
manage.py
manage.py
requirements.txt
requirements.txt
my_standalone_project/
my_standalone_project/
settings.py
settings.py
urls.py
urls.py
wsgi.py
wsgi.py
We’ve already handled requirements.txt
, settings.py
, and urls.py
. The remaining files are manage.py
and wsgi.py
. Unless you’ve created custom management commands or added custom instrumentation into your wsgi.py
, it’s likely these modules require no further work on your end. It’s time to say a fond goodbye to your old project and congratulate it on a job well done.
我们已经处理了requirements.txt
, settings.py
和urls.py
其余文件是manage.py
和wsgi.py
除非您已经创建了自定义管理命令或将自定义工具添加到wsgi.py
,否则这些模块最终可能不需要任何进一步的工作。 现在该向您的旧项目表示深深的告别,并祝贺它做得很好。
蛋糕上的糖衣 (The Icing on the Cake)
Now that everything is playing happily together in a single project, there are a few niceties you can take advantage of.
现在,所有事情都在一个项目中一起快乐地玩着,您可以利用其中的一些优点。
命名网址 (Named URLs)
If your old projects were supporting a single site before, chances are these projects had to link to pages served by each other at some point. Since Django only knows about URL patterns within a single project, these URLs were likely duplicated in each project, or worse, in each template where they were used.
如果以前的旧项目以前只支持一个站点,则这些项目有可能不得不链接到彼此服务的页面。 由于Django只知道单个项目中的URL模式,因此这些URL可能在每个项目中重复使用,或更糟的是在使用它们的每个模板中重复。
Now that all applications are running under a single project, these hard-coded URL patterns can be replaced with their named equivalents! Those ten references to /polls/38
you added in a hurry to release can all be simplified to {% url ‘polls:poll_detail’ 38 %}
so that when you move your polls to a new URL pattern down the line you don’t need to make a change in all those places.
现在,所有应用程序都在一个项目下运行,这些硬编码的URL模式可以替换为它们的等效名称! 您急忙发布的对/polls/38
十个引用都可以简化为{% url 'polls:poll_detail' 38 %}
这样,当您将民意调查移到新的URL模式时,就不必需要在所有这些地方进行更改。
讯号 (Signals)
翻译自: https://www.pybloggers.com/2017/10/how-to-consolidate-multiple-django-projects/
django前后端项目整合