aws lambda使用
This tutorial details how AWS Lambda and API Gateway can be used to develop a simple code evaluation API, where an end user submits code, via an AJAX form submission, which is then executed securely by a Lambda function.
本教程详细介绍了如何使用AWS Lambda和API Gateway开发简单的代码评估API,最终用户可以通过AJAX表单提交来提交代码,然后由Lambda函数安全地执行该代码。
Check out the live demo of what you’ll be building in action here.
在此处查看您将要实际操作的实时演示。
WARNING: The code found in this tutorial is used to build a toy app to prototype a proof of concept and is not meant for production use.
警告:本教程中找到的代码用于构建玩具应用程序以对概念验证进行原型设计,并不用于生产。
This tutorial assumes that you already have an account set up with AWS. Also, we will use the US East (N. Virginia)
/ us-east-1
region. Feel free to use the region of your choice. For more info, review the Regions and Availability Zones guide.
本教程假定您已经使用AWS设置了帐户。 另外,我们将使用US East (N. Virginia)
us-east-1
US East (N. Virginia)
/ us-east-1
地区。 随意使用您选择的区域。 有关更多信息,请查看“ 区域和可用区”指南。
什么是AWS Lambda? (What is AWS Lambda?)
Amazon Web Services (AWS) Lambda is an on-demand compute service that lets you run code in response to events or HTTP requests.
Amazon Web Services(AWS)Lambda是一种按需计算服务,可让您运行代码以响应事件或HTTP请求。
Use cases:
用例:
Event | 事件 | Action | 行动 | ||
---|---|---|---|---|---|
Image added to S3 | 图片已添加到S3 | Image is processed | 图像已处理 | ||
HTTP Request via API Gateway | 通过API网关的HTTP请求 | HTTP Response | HTTP响应 | ||
Log file added to Cloudwatch | 日志文件已添加到Cloudwatch | Analyze the log | 分析日志 | ||
Scheduled event | 预定活动 | Back up files | 备份文件 | ||
Scheduled event | 预定活动 | Synchronization of files | 文件同步 |
For more examples, review the Examples of How to Use AWS Lambda guide from AWS.
有关更多示例,请参阅AWS的“如何使用AWS Lambda示例”指南。
You can run scripts and apps without having to provision or manage servers in a seemingly infinitely-scalable environment where you pay only for usage. This is “serverless” computing in a nut shell. For our purposes, AWS Lambda is a perfect solution for running user-supplied code quickly, securely, and cheaply.
您可以运行脚本和应用程序,而不必在看似无限扩展的环境中(仅需为使用付费)配置或管理服务器。 简而言之,这就是“ 无服务器 ”计算。 出于我们的目的,AWS Lambda是快速,安全且廉价地运行用户提供的代码的理想解决方案。
As of writing, Lambda supports code written in JavaScript (Node.js), Python, Java, and C#.
在撰写本文时,Lambda 支持以JavaScript(Node.js),Python,Java和C#编写的代码。
项目设置 (Project Setup)
Start by cloning down the base project:
首先克隆基础项目:
|
Then, check out the v1 tag to the master branch:
然后,将v1标签签出到master分支:
|
Open the index.html file in your browser of choice:
在您选择的浏览器中打开index.html文件:
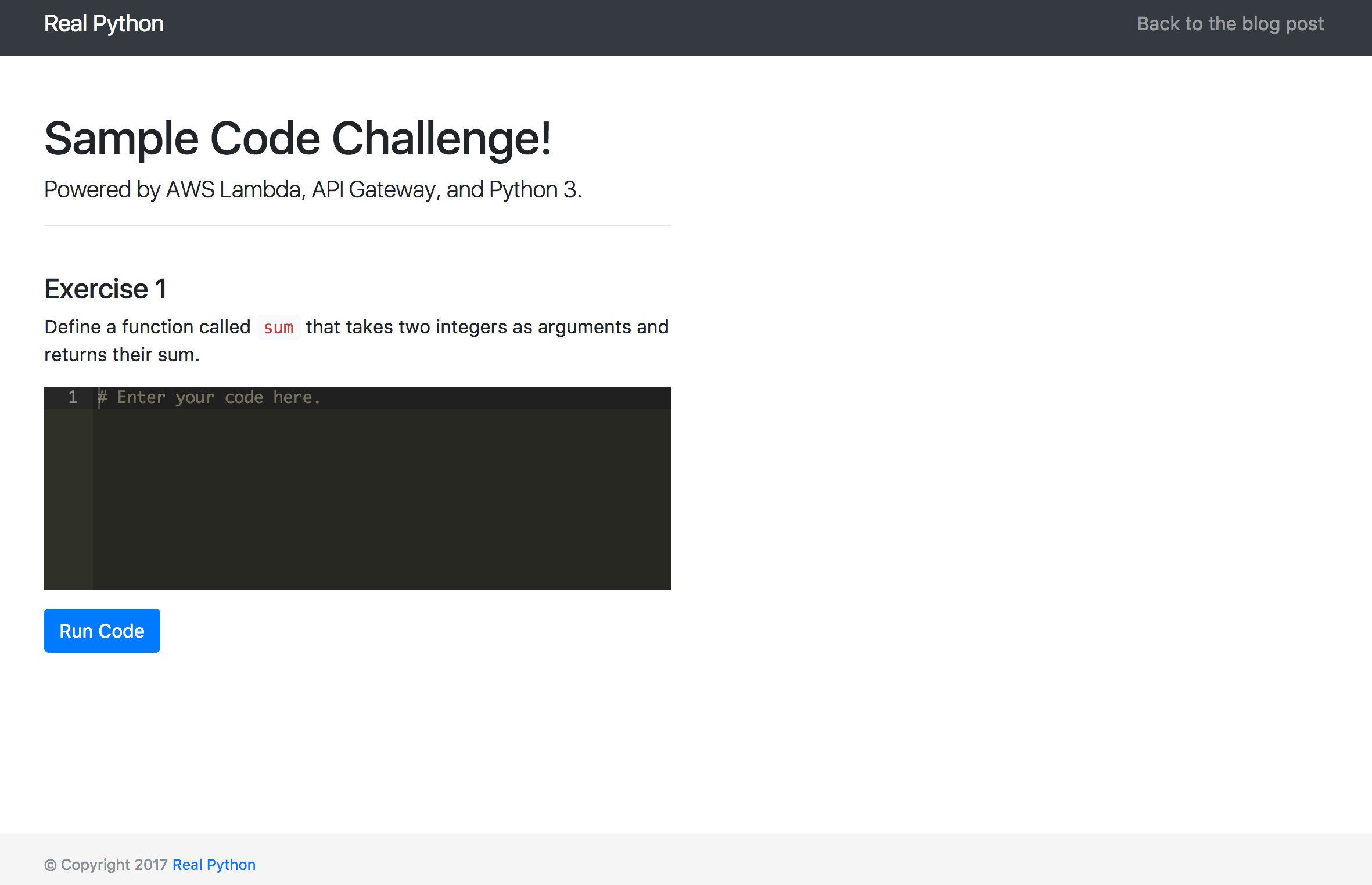
Then, open the project in your code editor:
然后,在代码编辑器中打开项目:
|
Let’s quickly review the code. Essentially, we just have a simple HTML form styled with Bootstrap. The input field is replaced with Ace, an embeddable code editor, which provides basic syntax highlighting. Finally, within assets/main.js, a jQuery event handler is wired up to grab the code from the Ace editor, when the form is submitted, and send the data somewhere (eventually to API Gateway) via an AJAX request.
让我们快速回顾一下代码。 本质上,我们只有一个简单HTML表单,样式为Bootstrap 。 输入字段被Ace (可嵌入的代码编辑器)替换,该编辑器提供了基本的语法突出显示功能。 最后,在资产/main.js中,连接了一个jQuery事件处理程序,以在提交表单后从Ace编辑器中获取代码,并通过AJAX请求将数据发送到某个地方(最终发送到API Gateway)。
Lambda设置 (Lambda Setup)
Within the AWS Console, navigate to the main Lambda page and click “Create a function”:
在AWS控制台内 ,导航到Lambda主页 ,然后单击“创建函数”:
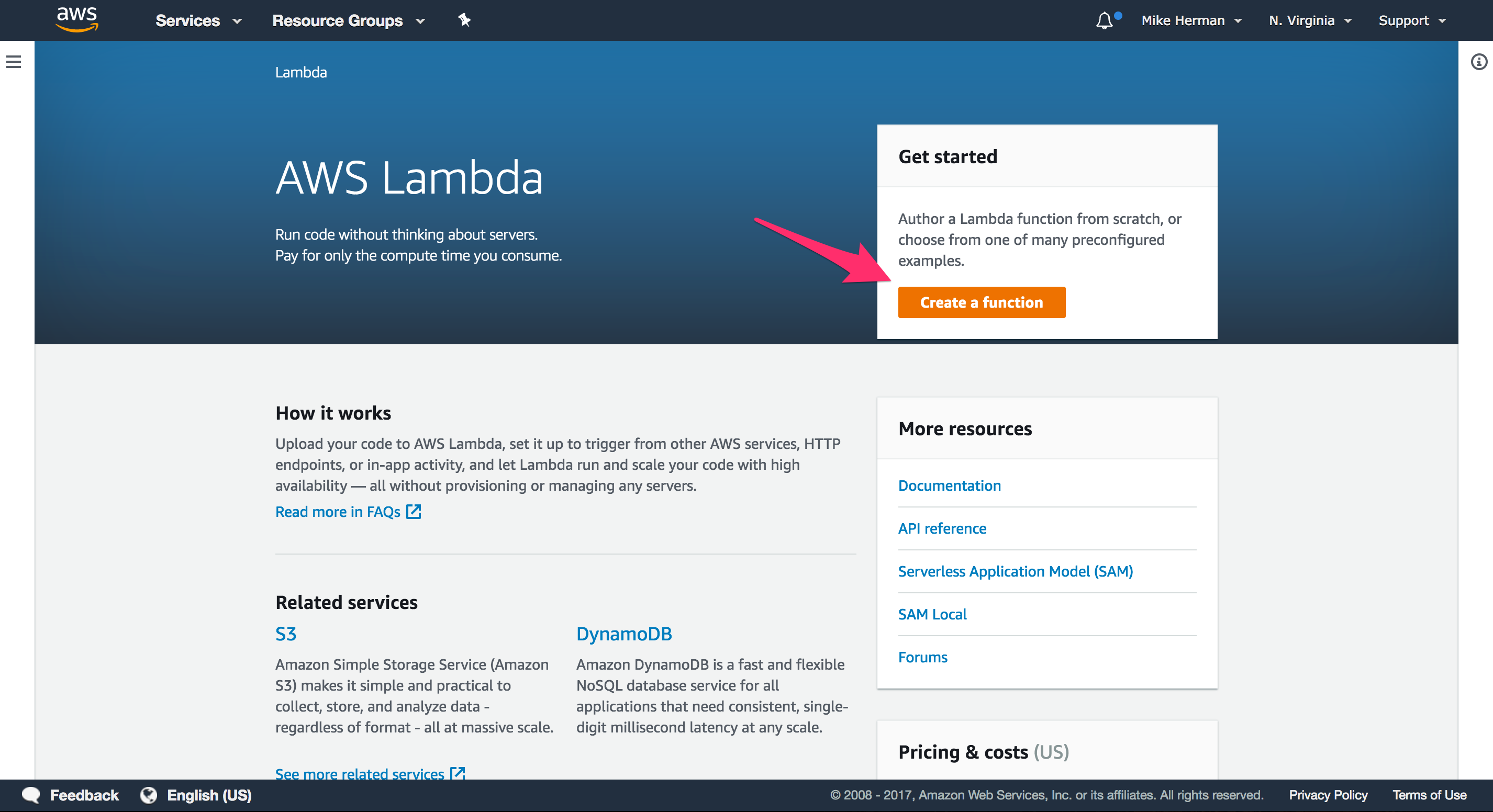
创建功能 (Create function)
Steps…
脚步…
-
Select blueprint: Click “Author from scratch” to start with a blank function:
-
Configure Triggers: We’ll set up the API Gateway integration later, so simply click “Next” to skip this part.
-
Configure function: Name the function
execute_python_code
, and add a basic description –Execute user-supplied Python code
. Select “Python 3.6” in the “Runtime” drop-down. -
Within the inline code editor, update the
lambda_handler
function definition with:1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
Here, within
lambda_handler
, which is the default entry point for Lambda, we parse the JSON request body, passing the supplied code along with some test code –sum(1,1)
– to the exec function – which executes the string as Python code. Then, we simply ensure the actual results are the same as what’s expected – e.g., 2 – and return the appropriate response.Under “Lambda function handler and role”, leave the default handler and then select “Create a new Role from template(s)” from the drop-down. Enter a “Role name”, like
api_gateway_access
, and select “ Simple Microservice permissions” for the “Policy templates”, which provides access to API Gateway.Click “Next”.
-
Review: Create the function after a quick review.
-
选择蓝图:单击“从头开始”以启动空白功能:
配置触发器:我们稍后将设置API网关集成,因此只需单击“下一步”即可跳过这一部分。
-
配置函数:将函数命名为
execute_python_code
,并添加基本描述-Execute user-supplied Python code
。 在“运行时”下拉列表中选择“ Python 3.6”。 -
lambda_handler
联代码编辑器中,使用以下命令更新lambda_handler
函数定义:1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
在这里,在
lambda_handler
(这是Lambda的默认入口点)中,我们解析JSON请求主体,并将提供的代码以及一些测试代码(sum(1,1)
传递给exec函数(该函数将字符串作为Python代码执行) 。 然后,我们只需确保实际结果与预期的结果相同(例如2),然后返回适当的响应即可。在“ Lambda函数处理程序和角色”下,保留默认处理程序,然后从下拉列表中选择“从模板创建新角色”。 输入一个“角色名称”,例如
api_gateway_access
,然后为“策略模板”选择“简单微服务权限”,从而可以访问API网关。点击下一步”。
审阅:快速审阅后创建功能。
测试 (Test)
Next click on the “Test” button to execute the newly created Lambda:
接下来,单击“测试”按钮以执行新创建的Lambda:
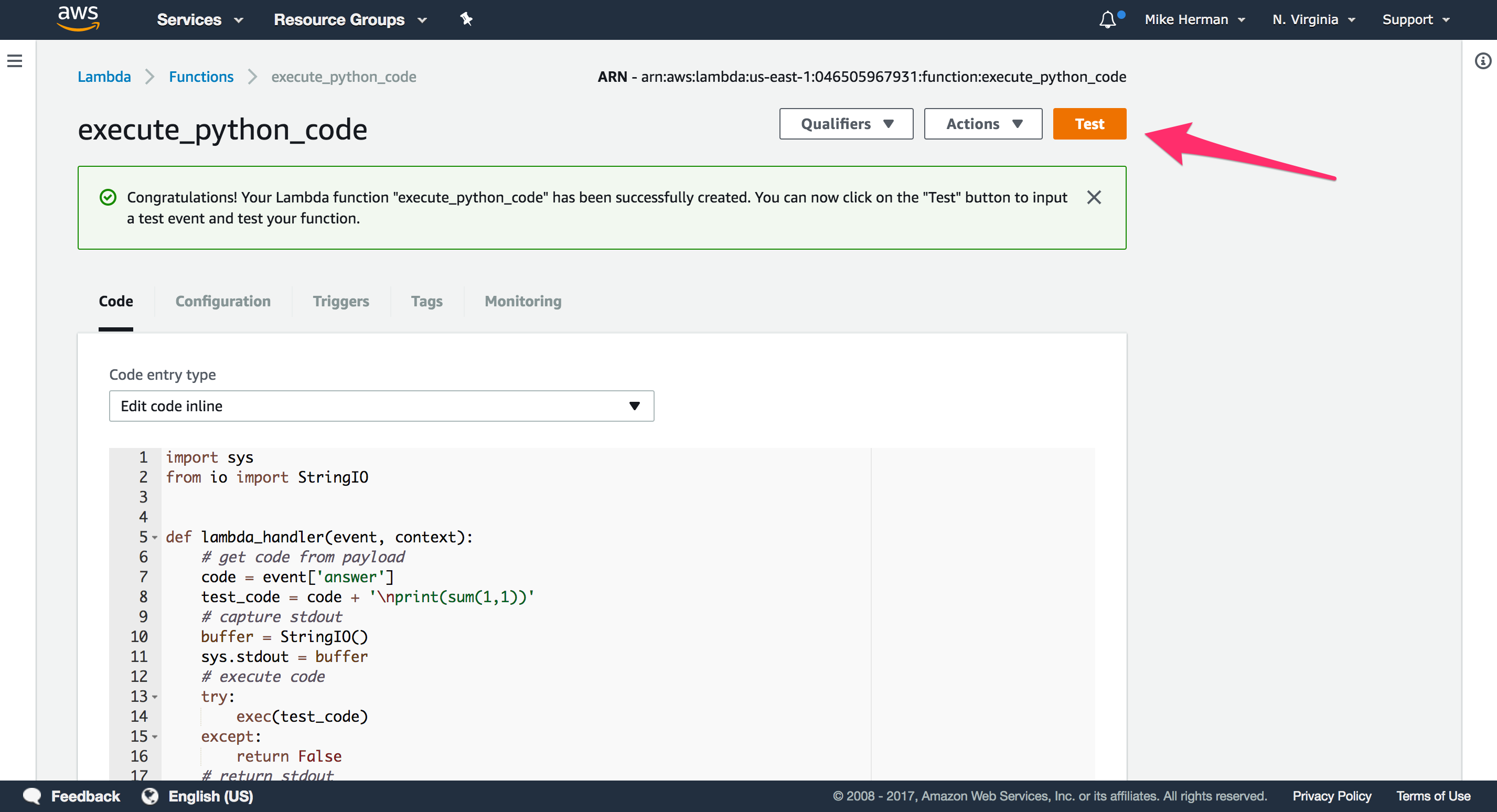
Using the “Hello World” event template, replace the sample with:
使用“ Hello World”事件模板,将示例替换为:
|
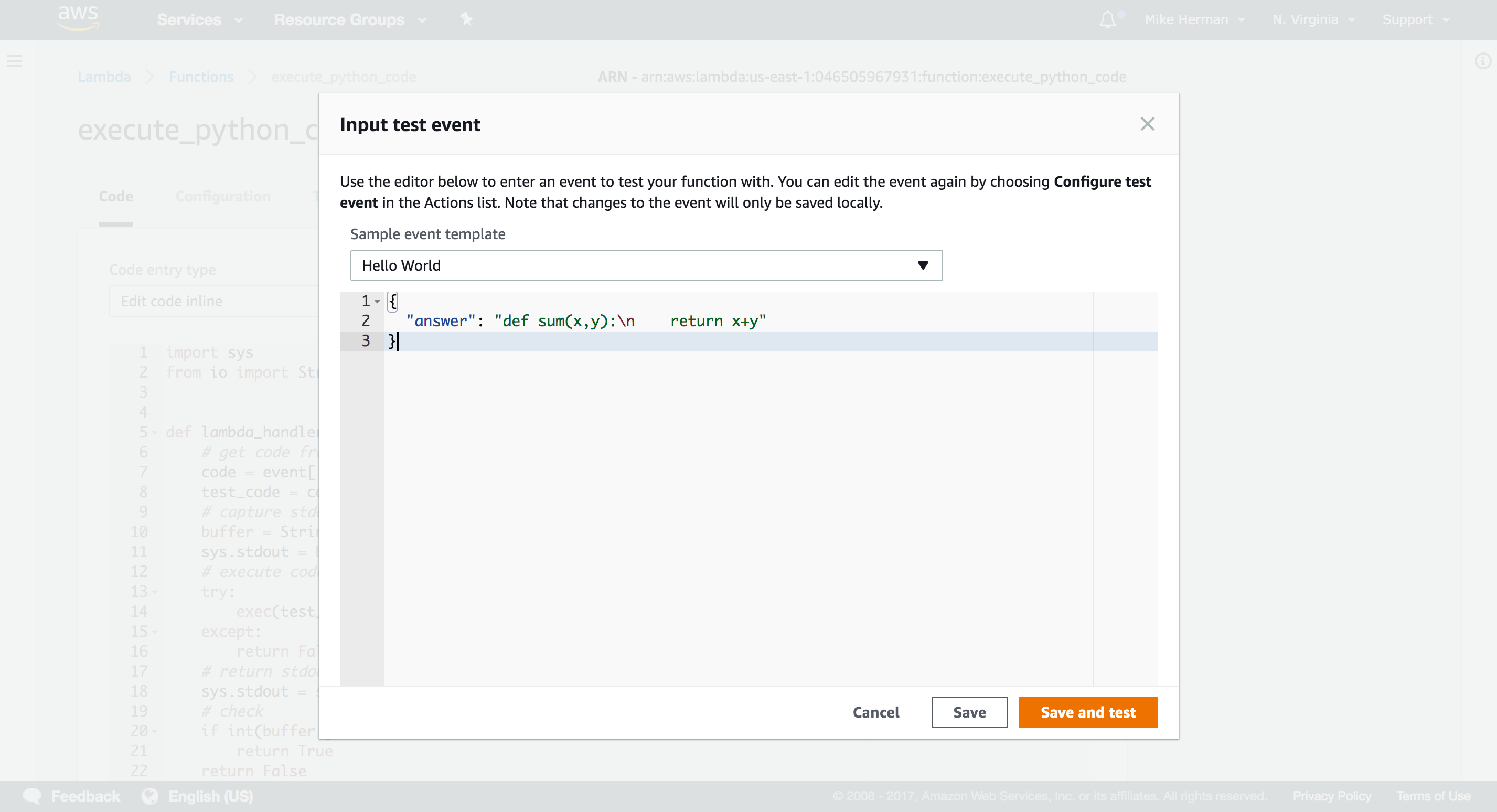
Click the “Save and test” button at the bottom of the modal to run the test. Once done, you should see something similar to:
单击模式底部的“保存并测试”按钮以运行测试。 完成后,您应该看到类似以下内容的内容:
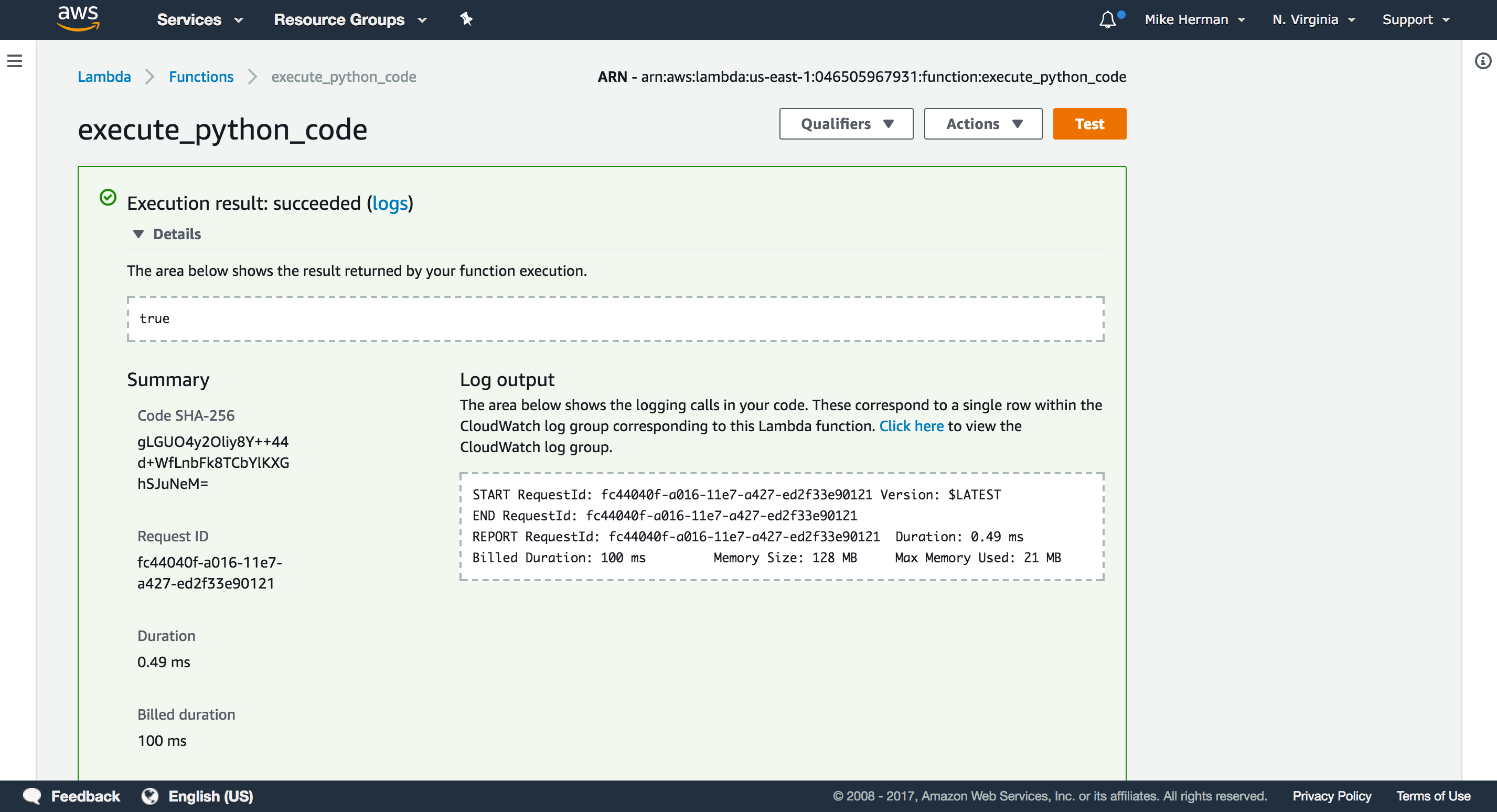
With that, we can move on to configuring the API Gateway to trigger the Lambda from user-submitted POST requests…
这样,我们可以继续配置API网关,以从用户提交的POST请求中触发Lambda。
API网关设置 (API Gateway Setup)
API Gateway is used to define and host APIs. In our example, we’ll create a single HTTP POST endpoint that triggers the Lambda function when an HTTP request is received and then responds with the results of the Lambda function, either true
or false
.
API网关用于定义和托管API。 在我们的示例中,我们将创建一个HTTP POST端点,该端点在接收到HTTP请求时触发Lambda函数,然后使用Lambda函数的结果( true
或false
进行响应。
Steps:
脚步:
- Create the API
- Test it manually
- Enable CORS
- Deploy the API
- Test via cURL
- 创建API
- 手动测试
- 启用CORS
- 部署API
- 通过cURL测试
创建API (Create the API)
-
To start, from the API Gateway page, click the “Get Started” button to create a new API:
-
Select “New API”, and then provide a descriptive name, like
code_execute_api
:Then, create the API.
-
Select “Create Resource” from the “Actions” drop-down.
-
Name the resource
execute
, and then click “Create Resource”. -
With the resource highlighted, select “Create Method” from the “Actions” drop-down.
-
Choose “POST” from the method drop-down. Click the checkmark next to it.
-
In the “Setup” step, select “Lambda Function” as the “Integration type”, select the “us-east-1” region in the drop-down, and enter the name of the Lambda function that you just created.
-
Click “Save”, and then click “OK” to give permission to the API Gateway to run your Lambda function.
-
首先,从“ API网关”页面 ,单击“入门”按钮以创建新的API:
-
选择“ New API”,然后提供一个描述性名称,例如
code_execute_api
:然后,创建API。
-
从“操作”下拉列表中选择“创建资源”。
-
将资源命名为
execute
,然后单击“创建资源”。 -
在资源突出显示的情况下,从“操作”下拉列表中选择“创建方法”。
-
从方法下拉列表中选择“ POST”。 单击它旁边的复选标记。
-
在“设置”步骤中,选择“ Lambda函数”作为“集成类型”,在下拉列表中选择“ us-east-1”区域,然后输入刚创建的Lambda函数的名称。
单击“保存”,然后单击“确定”,以授予API网关运行Lambda函数的权限。
手动测试 (Test it manually)
To test, click on the lightning bolt that says “Test”.
要进行测试,请单击显示“测试”的闪电。
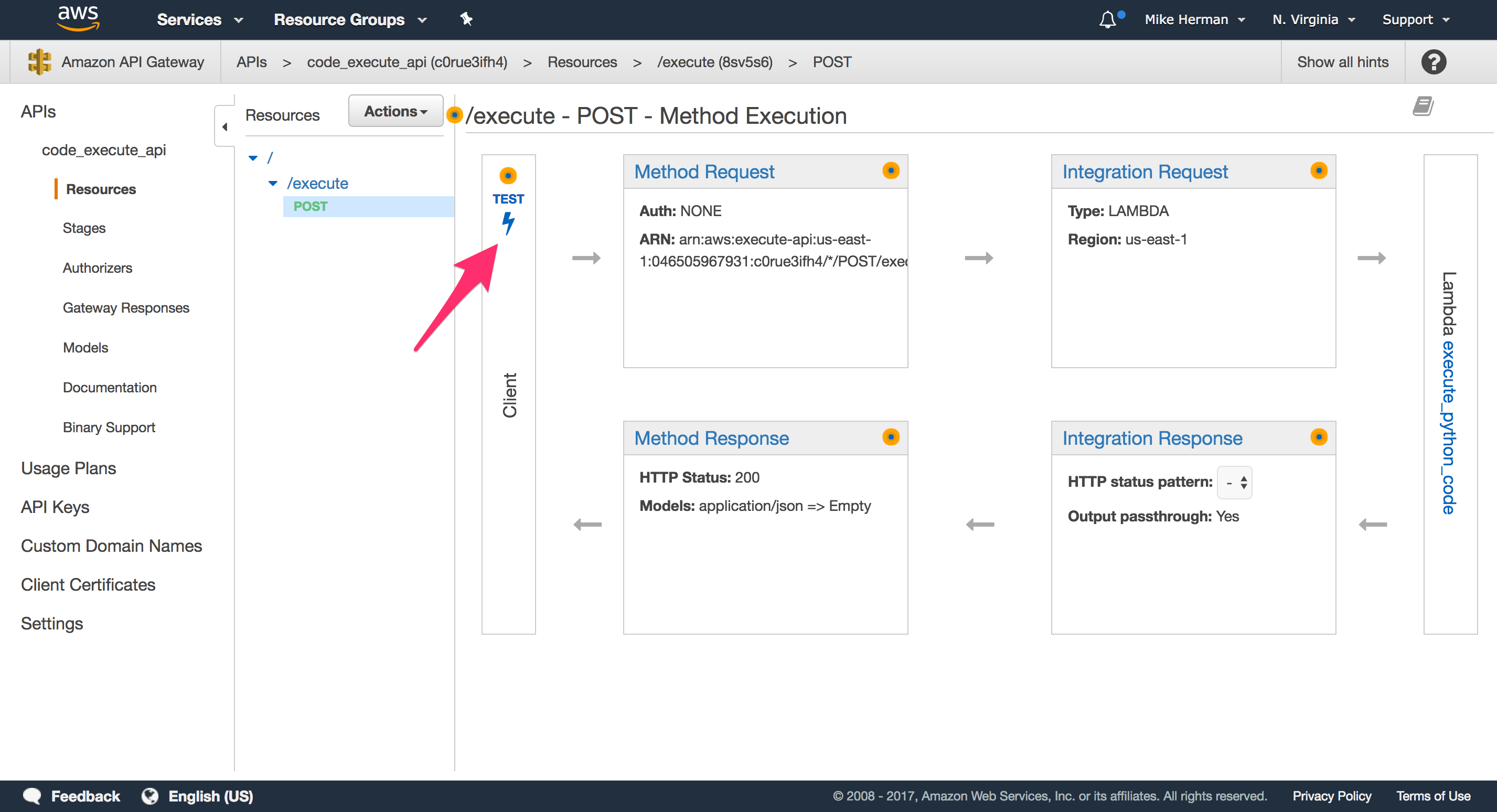
Scroll down to the “Request Body” input and add the same JSON code we used with the Lambda function:
向下滚动到“请求正文”输入,并添加与Lambda函数一起使用的JSON代码:
|
Click “Test”. You should see something similar to:
点击“测试”。 您应该看到类似以下内容:
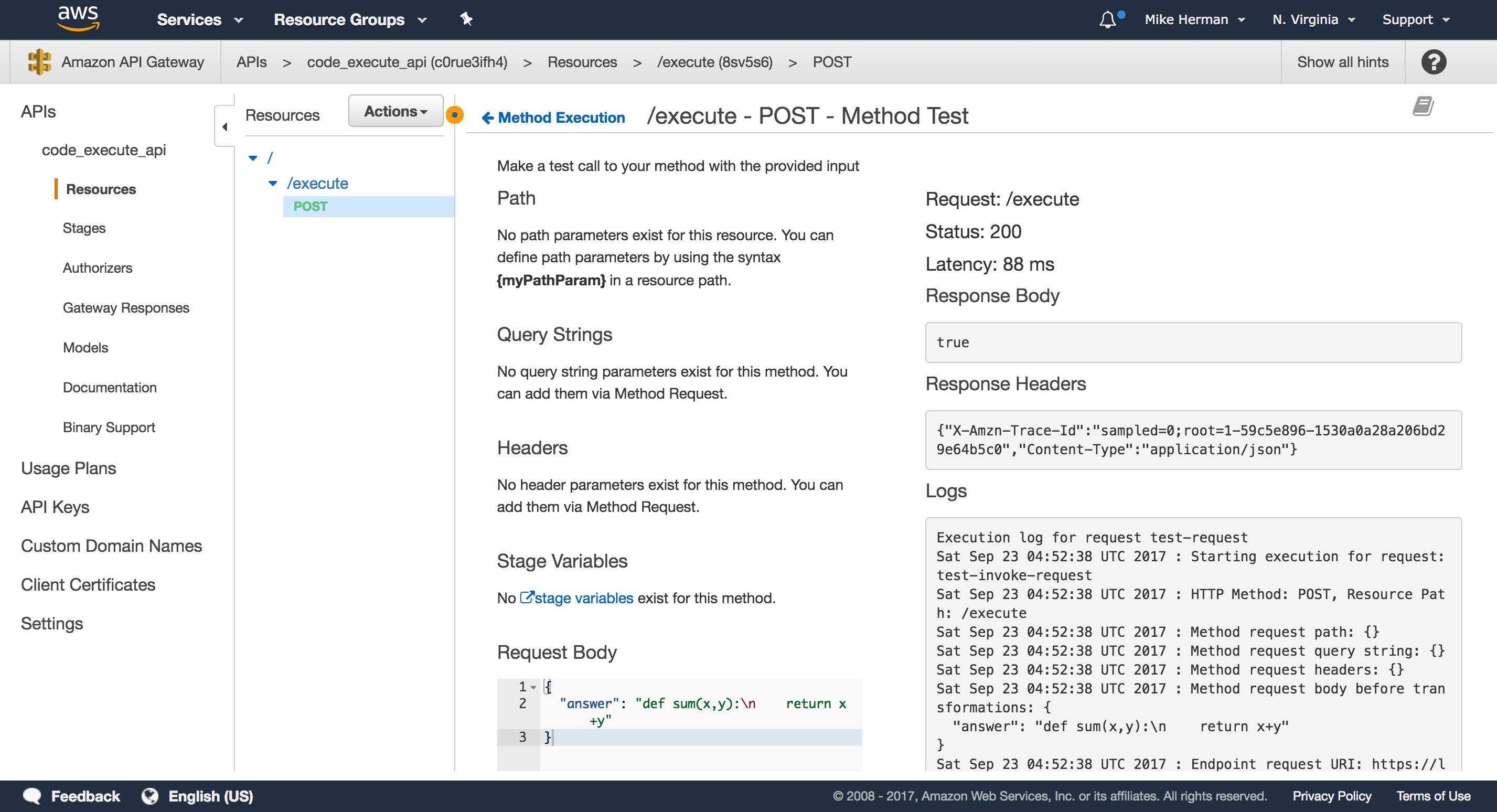
启用CORS (Enable CORS)
Next, we need to enable CORS so that we can POST to the API endpoint from another domain.
接下来,我们需要启用CORS,以便我们可以从另一个域POST到API端点。
With the resource highlighted, select “Enable CORS” from the “Actions” drop-down:
在资源突出显示的情况下,从“操作”下拉列表中选择“启用CORS”:
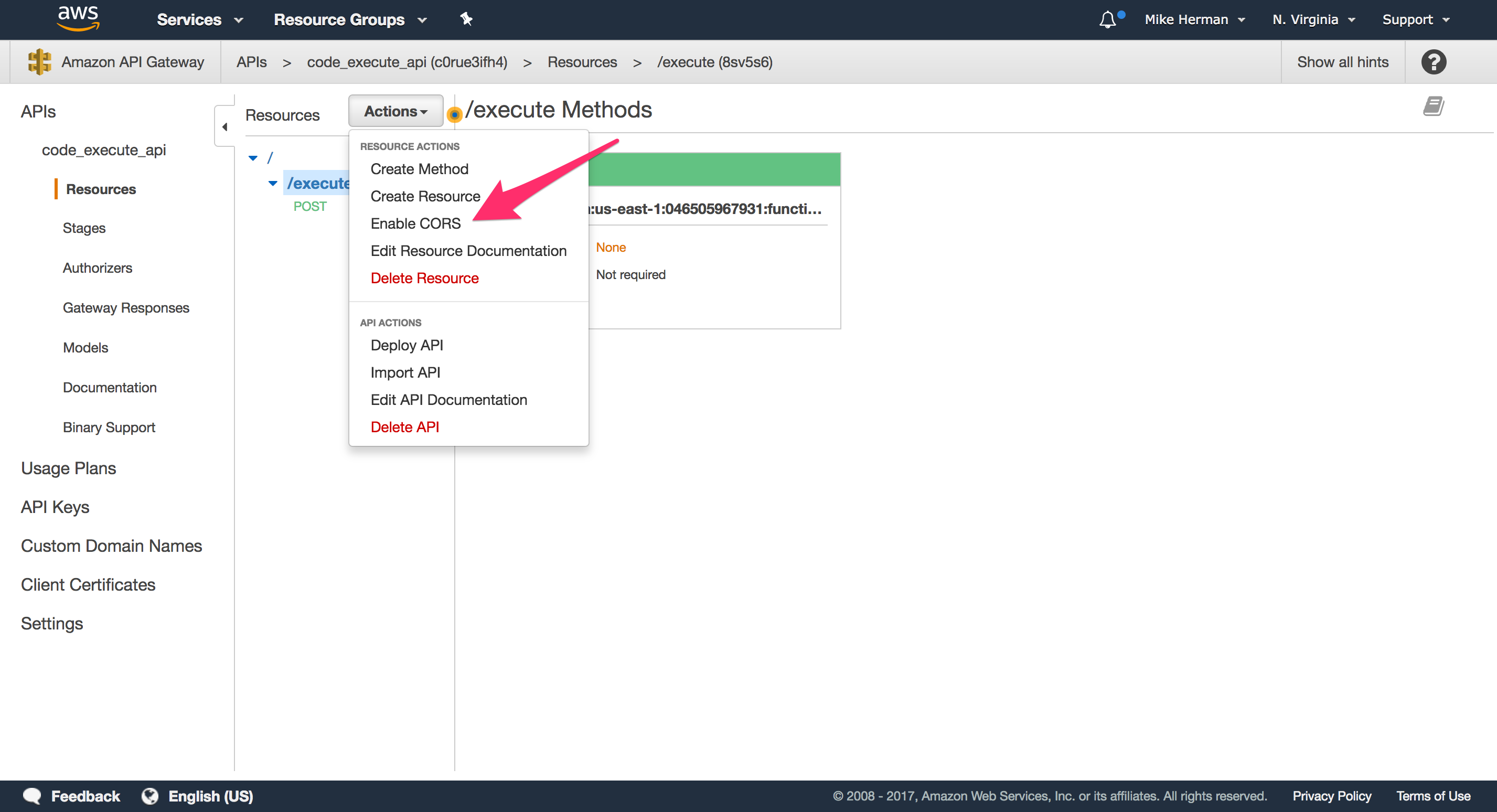
Just keep the defaults for now since we’re still testing the API. Click the “Enable CORS and replace existing CORS headers” button.
由于我们仍在测试API,因此请暂时保留默认设置。 单击“启用CORS并替换现有的CORS标头”按钮。
部署API (Deploy the API)
Finally, to deploy, select “Deploy API” from the “Actions” drop-down:
最后,要进行部署,请从“操作”下拉列表中选择“部署API”:
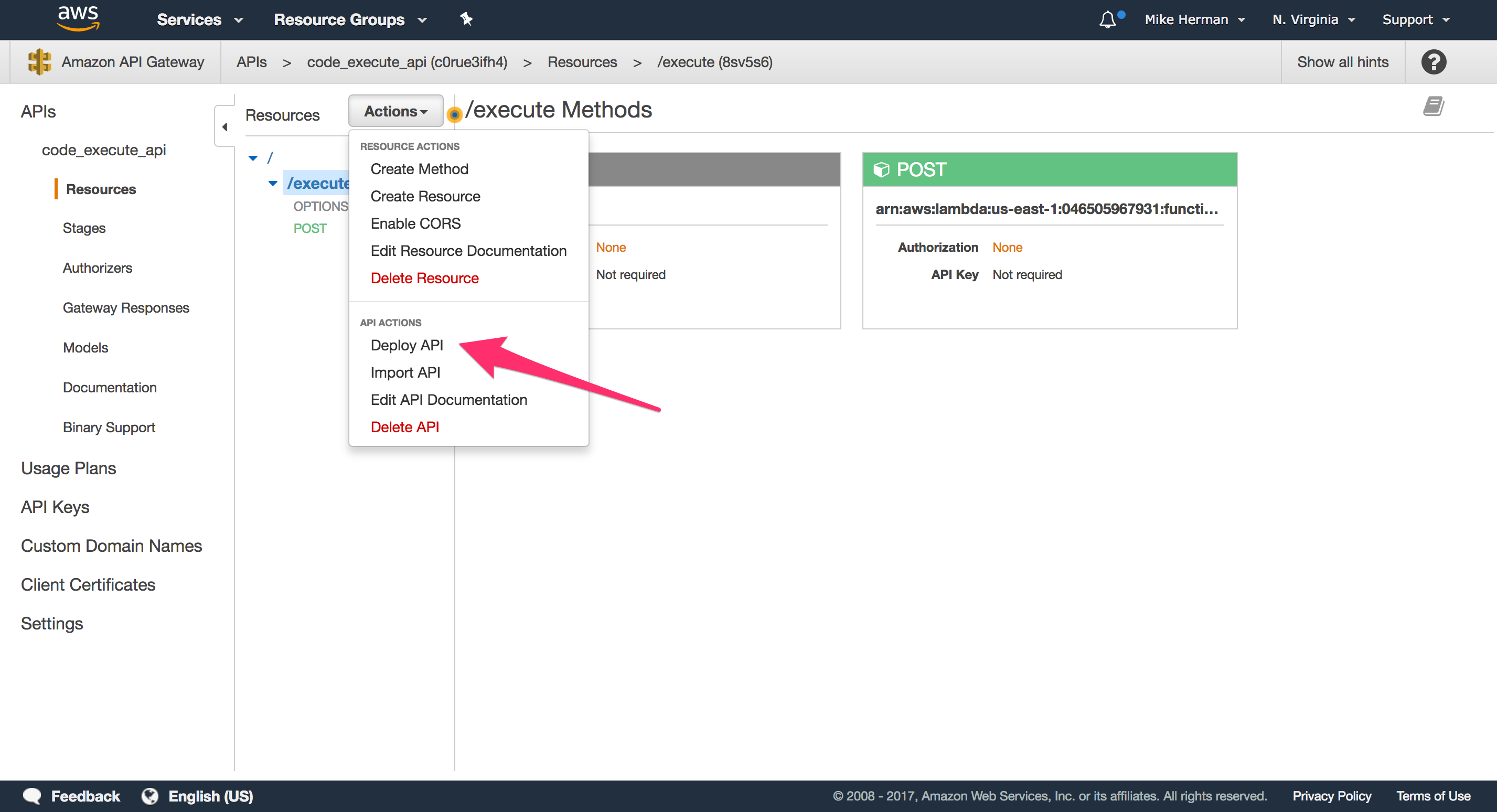
Create a new “Deployment stage” called ‘v1’:
创建一个名为“ v1”的新“部署阶段”:
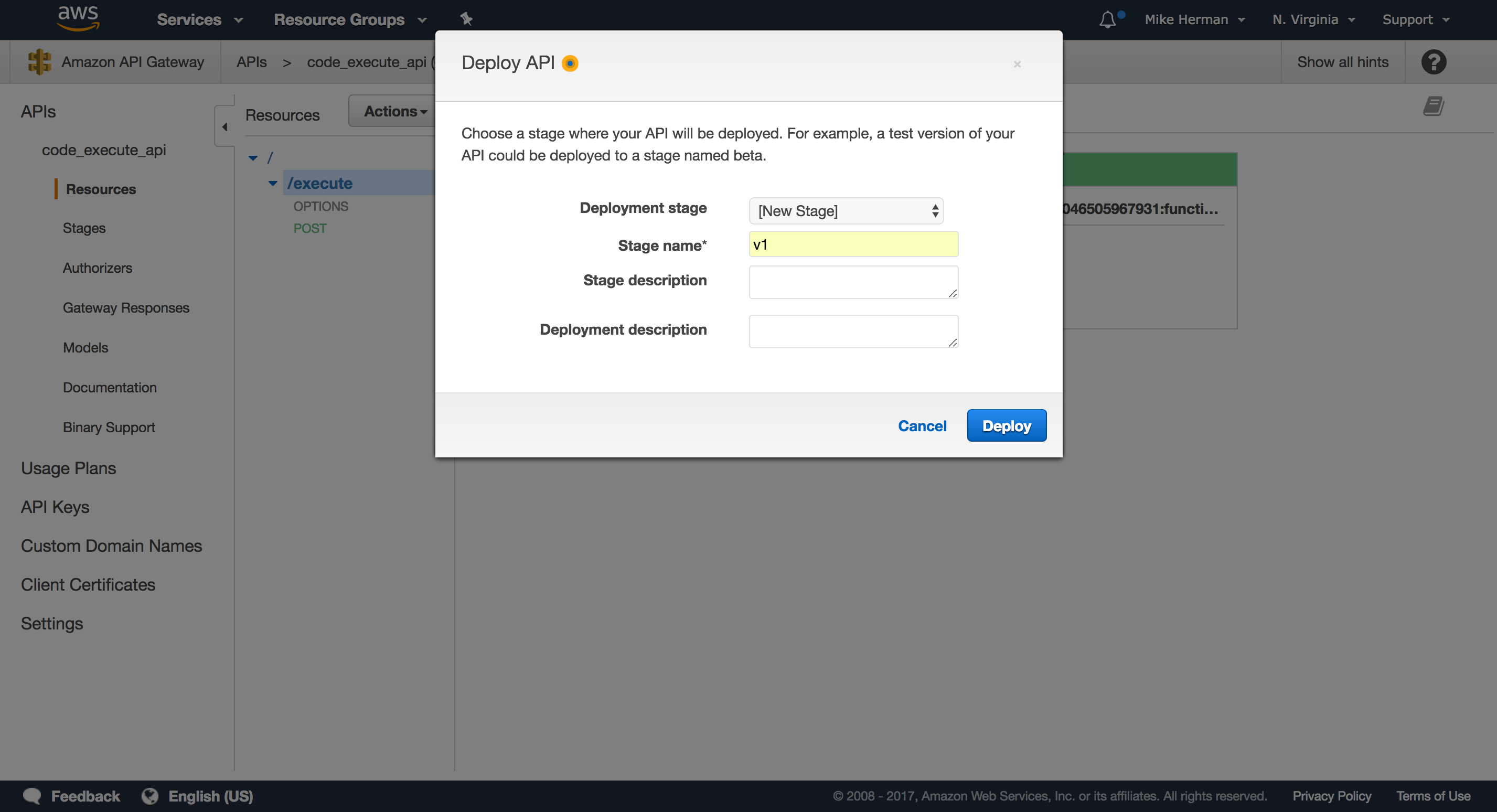
API gateway will generate a random subdomain for the API endpoint URL, and the stage name will be added to the end of the URL. You should now be able to make POST requests to a similar URL:
API网关将为API端点URL生成一个随机子域,并将阶段名称添加到URL的末尾。 现在,您应该可以向类似的URL发出POST请求:
|
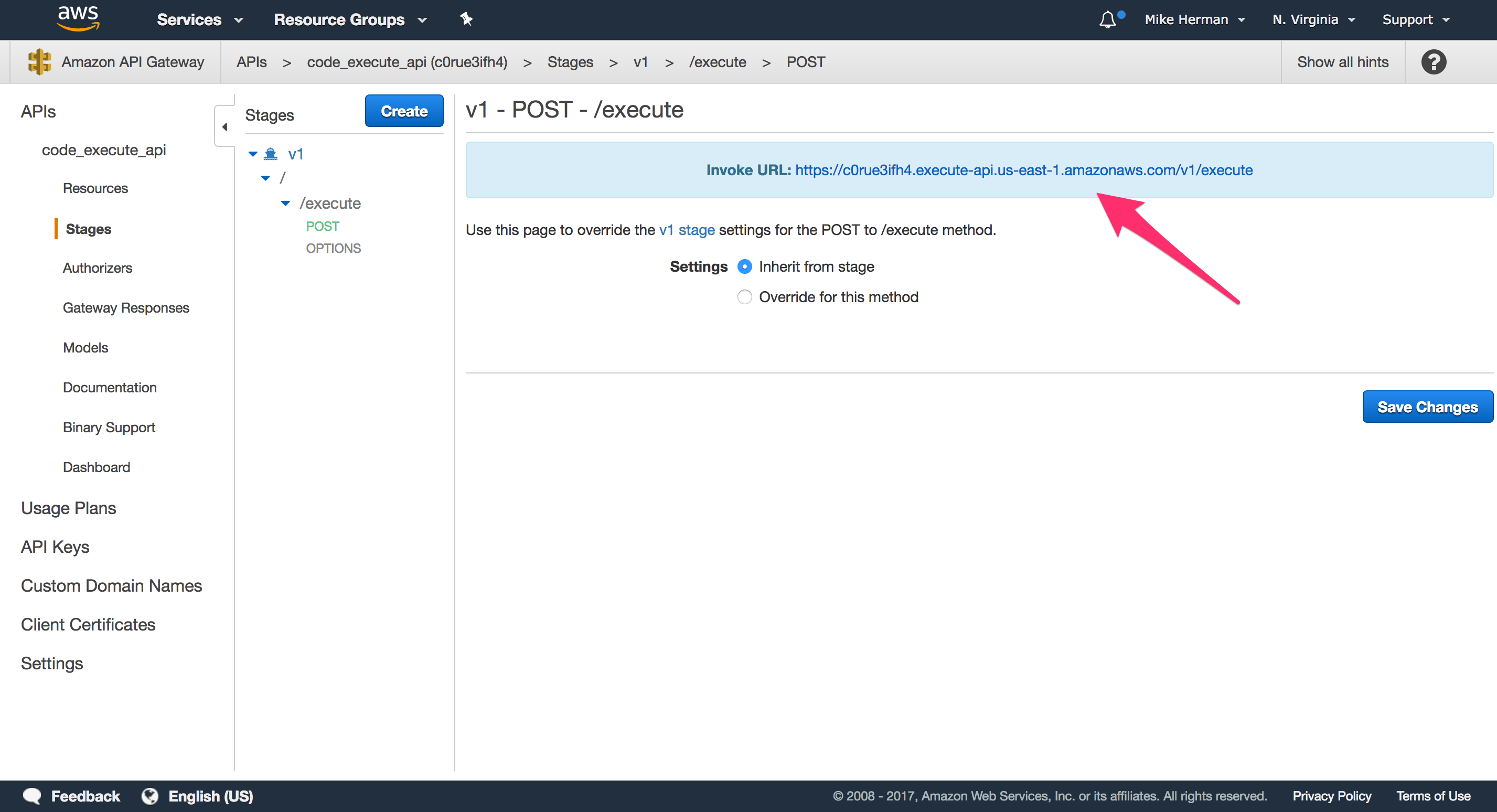
通过cURL测试: (Test via cURL:)
|
更新表格 (Update the Form)
Now, to update the form so that it sends the POST request to the API Gateway endpoint, first add the URL to the grade
function in assets/main.js:
现在,要更新表单,以便将POST请求发送到API网关端点,请首先将URL添加到asset / main.js中的grade
函数:
|
Then, update the .done
and .catch()
functions, like so:
然后,更新.done
和.catch()
函数,如下所示:
|
Now, if the request is a success, the appropriate message will be added – via the jQuery html method – to an HTML element with a class of answer
. Add this element, just below the HTML form, within index.html:
现在,如果请求成功,则将通过jQuery html方法将适当的消息添加到具有answer
类HTML元素中。 在index.html中HTML表单下方添加此元素:
|
Let’s add a bit of style as well to the assets/main.css file:
让我们在assets / main.css文件中添加一些样式:
|
Test it out!
测试一下!
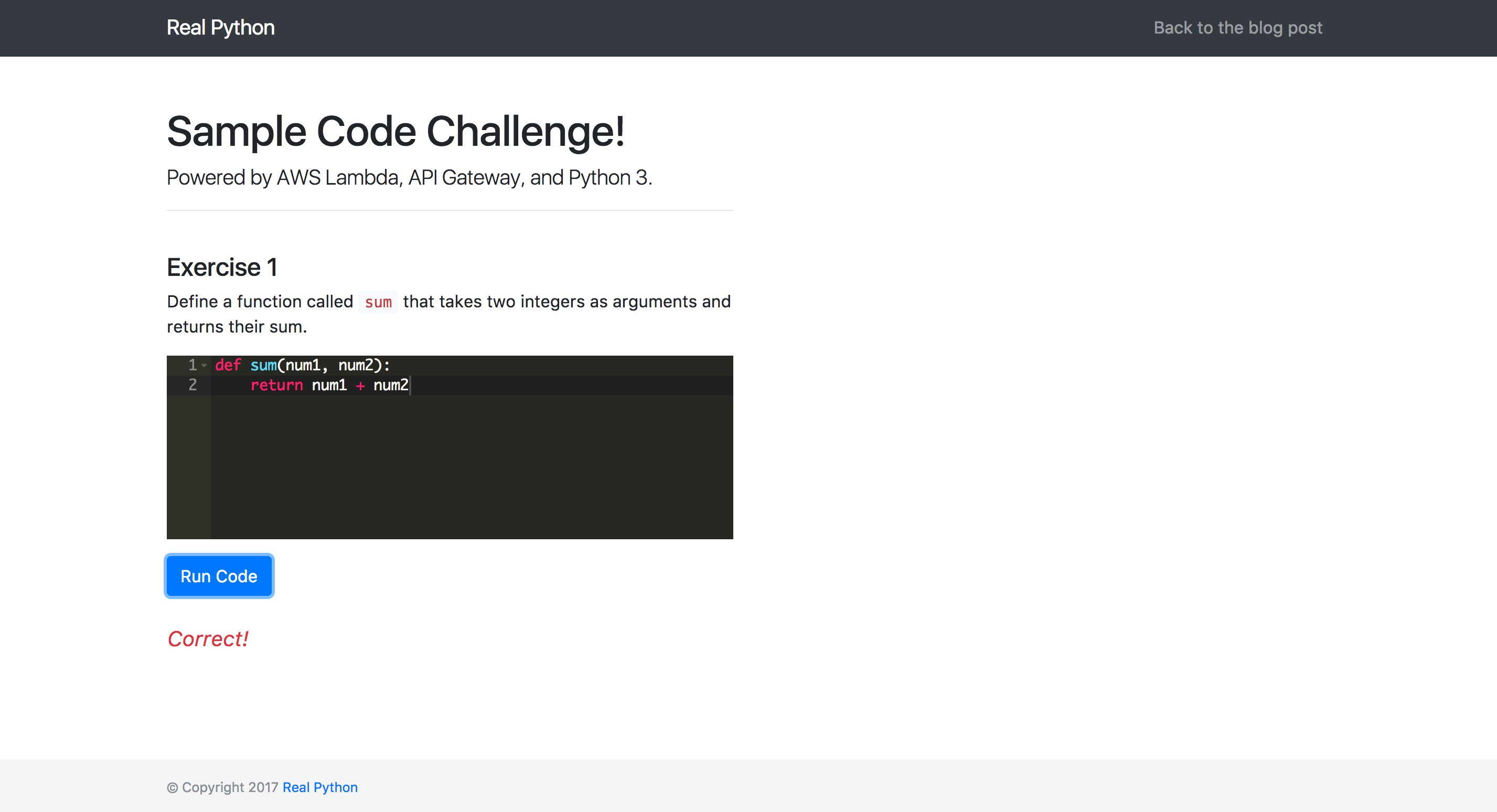
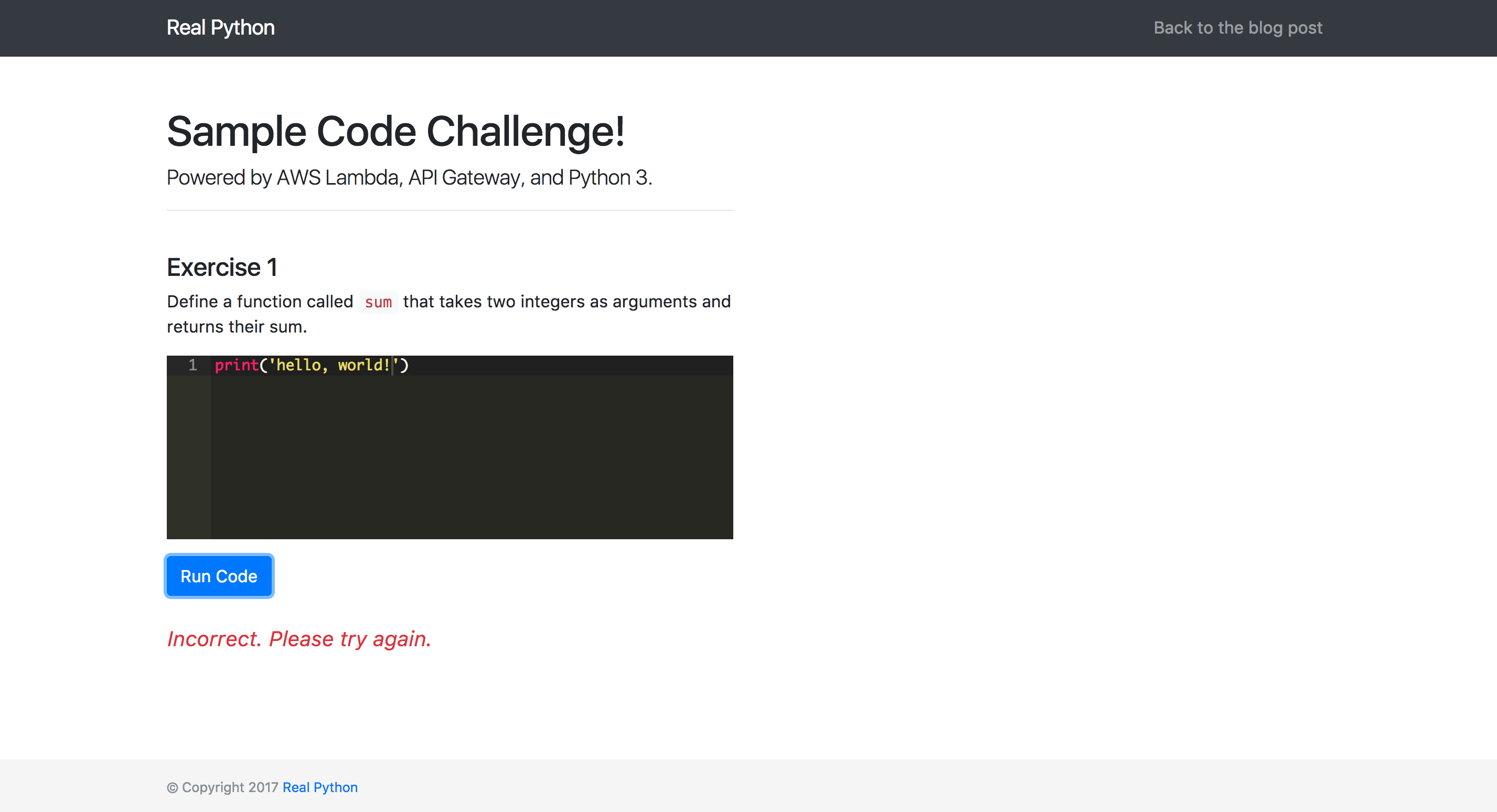
下一步 (Next Steps)
- Production: Think about what’s required for a more robust, production-ready application – HTTPS, authentication, possibly a data store. How would you implement these within AWS? Which AWS services can/would you use?
- Dynamic: Right now the Lambda function can only be used to test the
sum
function. How could you make this (more) dynamic, so that it can be used to test any code challenge (maybe even in any language)? Try adding a data attribute to the DOM, so that when a user submits an exercise the test code along with solution is sent along with the POST request – i.e.,<some-html-element data-test="nprint(sum(1,1))" data-results"2" </some-html-element>
. - Stack trace: Instead of just responding with
true
orfalse
, send back the entire stack trace and add it to the DOM when the answer is incorrect.
- 生产:考虑一个更健壮,可用于生产的应用程序需要什么-HTTPS,身份验证,可能还有数据存储。 您将如何在AWS中实施这些? 您可以/将使用哪些AWS服务?
- 动态:现在,Lambda函数只能用于测试
sum
函数。 您如何才能使它(更)动态,以便可以用来测试任何代码挑战(甚至可以使用任何语言)? 尝试将数据属性添加到DOM,以便当用户提交练习时,测试代码以及解决方案与POST请求一起发送-即,<some-html-element data-test="nprint(sum(1,1))" data-results"2" </some-html-element>
。 - 堆栈跟踪:答案不正确时,请发回整个堆栈跟踪并将其添加到DOM,而不仅仅是用
true
或false
响应。
翻译自: https://www.pybloggers.com/2017/09/code-evaluation-with-aws-lambda-and-api-gateway/
aws lambda使用