使用克隆的时侯报空指针异常
Problem statement:
问题陈述:
Given a linked list, there are two node pointers one point to the next node of the linked list and another is the random pointer which points to any random node of that linked list. Your task is to make a clone a linked list of that linked list.
给定一个链表,有两个节点指针,一个指向链表的下一个节点,另一个是随机指针,它指向该链表的任何随机节点。 您的任务是使克隆成为该链接列表的链接列表 。
Example:
例:
Input:
输入:
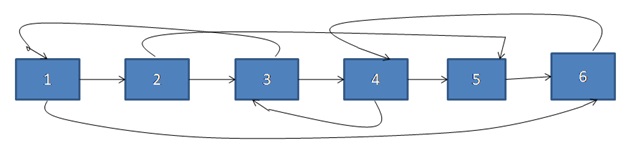
Output
输出量
Make a same copy of that linked list.
复制该链接列表。
Algorithm:
算法:
Node structure for random pointer:
随机指针的节点结构:
Node temp
{
Int data;
Node* next;
Node* random=NULL;
}
To solve that problem we follow this algorithm:
为了解决这个问题,我们遵循以下算法:
First, we copy the next pointers and make a linked list.
首先,我们复制下一个指针并创建一个链表。
In the second iteration, we copy the random pointers of that linked list.
在第二次迭代中,我们复制该链表的随机指针。
Using this algorithm the time complexity is O(n).
使用此算法, 时间复杂度为O(n) 。
C++ implementation:
C ++实现:
#include <bits/stdc++.h>
using namespace std;
struct node {
int data;
node* next;
node* random;
};
//Create a new node
struct node* create_node(int x)
{
struct node* temp = new node;
temp->data = x;
temp->next = NULL;
temp->random = NULL;
return temp;
}
void push_random(node* head, int x, int y)
{
struct node* temp1 = head;
while (temp1) {
if (temp1->data == x) {
break;
}
temp1 = temp1->next;
}
struct node* temp2 = head;
while (temp2) {
if (temp2->data == y) {
break;
}
temp2 = temp2->next;
}
temp1->random = temp2;
}
//Enter the node into the linked list
void push(node** head, int x)
{
struct node* store = create_node(x);
if (*head == NULL) {
*head = store;
return;
}
struct node* temp = *head;
while (temp->next) {
temp = temp->next;
}
temp->next = store;
}
//Reverse the linked list
struct node* copy(node* head)
{
struct node* temp = head;
struct node* store = create_node(0);
struct node* curr = store;
while (temp) {
curr->next = create_node(temp->data);
temp = temp->next;
curr = curr->next;
}
temp = head;
curr = store;
while (temp) {
curr->random = temp->random;
curr = curr->next;
temp = temp->next;
}
store = store->next;
return store;
}
//Print the list
void print(node* head)
{
struct node* temp = head;
while (temp) {
cout << temp->data << " ";
temp = temp->next;
}
}
int main()
{
struct node* l = NULL;
push(&l, 1);
push(&l, 2);
push(&l, 3);
push(&l, 4);
push(&l, 5);
push(&l, 6);
srand(time(0));
for (int i = 1; i <= 6; i++) {
int r = (rand() % 6) + 1;
push_random(l, i, r);
}
cout << "Original list" << endl;
print(l);
struct node* c = copy(l);
cout << "\nCopy list" << endl;
print(c);
return 0;
}
Output
输出量
Original list
1 2 3 4 5 6
Copy list
1 2 3 4 5 6
翻译自: https://www.includehelp.com/cpp-programs/clone-a-linked-list-with-next-and-random-pointer.aspx
使用克隆的时侯报空指针异常