javascript运算符
JavaScript按位运算符 (JavaScript Bitwise Operators)
A lot of times you come across some strange operators where you're knocking your head to understand what is going on in the code. Almost all the programming languages have bitwise operators. It is essential for a language to have these since they work at the binary level. Let's first understand what bitwise operators are and why they are the way they are for any general language or framework then we can dive into how to use them in JavaScript.
很多时候,您遇到了一些奇怪的运算符,您在这里敲了一下头,以了解代码中发生了什么。 几乎所有的编程语言都具有按位运算符 。 语言对这些语言至关重要,因为它们在二进制级别起作用。 首先让我们了解按位运算符是什么以及为什么它们对于任何通用语言或框架都是如此,然后我们将深入研究如何在JavaScript中使用它们。
In the number system, we have more than one way of representing numbers. Most of the time we are using the decimal numbers which have a base-10 but there are other types of numbers such as hexadecimal, binary, octal, etc. Bitwise operators interact with the numbers at the binary level. Since any machine understands only binary (a mere combination of the 0's and 1's), the way a machine understands what we're doing in a program is by converting into its own language of the 0's and 1's. That's why the most fundamental way of playing with numbers is by breaking them down at the binary level. When you store a variable or carry out even basic arithmetic operations, in reality, these operations are being done by first converting the base -10 numbers to binary numbers. Even variables stored in memory are referenced through binary numbers and memory addresses are themselves a hexadecimal value. So understanding Bitwise is a great way to understand how a machine is interpreting things and anything has done using them is always faster than what you'd achieve through some other operation.
在数字系统中,我们有多种表示数字的方法。 大多数时候,我们使用十进制数(以10为底),但还有其他类型的数字,例如十六进制,二进制,八进制等。 按位运算符在二进制级别与数字交互。 由于任何机器都只能理解二进制(0和1的组合),因此机器理解我们在程序中所做的工作的方式是将其转换为自己的0和1语言。 这就是为什么使用数字的最基本方法是在二进制级别分解数字。 实际上,当您存储变量或执行基本算术运算时,这些运算是通过首先将以-10为底的数字转换为二进制数来完成的。 甚至存储在内存中的变量也通过二进制数来引用,而内存地址本身就是一个十六进制值。 因此,了解Bitwise是了解机器如何解释事物以及使用这些事物所做的任何事情的好方法,它总是比您通过其他操作获得的结果更快。
Bitwise operators help us to carry out bit masking operations and play with the numbers at the binary level. We will look at the following most common bitwise operators in JavaScript.
按位运算符可帮助我们执行位屏蔽操作,并在二进制级别处理数字。 我们将研究以下JavaScript中最常见的按位运算符 。
JavaScript中按位运算符的列表 (List of Bitwise Operators in JavaScript )
Operator | Description |
---|---|
& | Bitwise AND |
| | Bitwise OR |
^ | Bitwise XOR |
~ | Bitwise NOT |
<< | Bitwise Left Shift |
>> | Bitwise Right Shift |
操作员 | 描述 |
---|---|
和 | 按位与 |
| | 按位或 |
^ | 按位异或 |
〜 | 按位非 |
<< | 按位左移 |
>> | 按位右移 |
Note:
注意:
JavaScript stores numbers as 64 bits floating-point numbers, but all bitwise operations are performed on 32 bits binary numbers.
JavaScript将数字存储为64位浮点数,但是所有按位运算都是对32位二进制数执行的。
Before a bitwise operation is performed, JavaScript converts numbers to 32 bits signed integers.
在执行按位运算之前,JavaScript将数字转换为32位带符号整数。
After the bitwise operation is performed, the result is converted back to 64 bits of JavaScript numbers.
执行按位运算后,结果将转换回64位JavaScript数字。
Let's first see what they mean by carrying out some operations. The bitwise AND first converts the two numbers into their binary and then does the AND operation (logical multiplication) from the end bit by bit.
首先,通过执行一些操作来了解它们的含义。 按位与首先将两个数字转换为二进制,然后从末尾开始逐位执行与运算(逻辑乘法)。
Let's say we have two numbers; 5 and 1.
Binary of 5: 1001
Binary of 1: 0001
If we take AND of every digit from the right side we get 0001 which is 1 in decimal notation. We can simply use & to get the same result, ie, the Bitwise AND between two numbers.
如果我们从右边取每个数字的AND ,我们将得到0001 ,即十进制表示法中的1 。 我们可以简单地使用&来获得相同的结果,即两个数字之间的按位与 。
To tryout, this code follow the following rules,
要进行试用,此代码应遵循以下规则,
Open the chrome dev console to try out the examples by right clicking on the browser → selecting inspect → selecting console or simply pree f12.
打开chrome开发人员控制台,通过右键单击浏览器→选择检查 →选择控制台或简单地按f12来尝试示例。
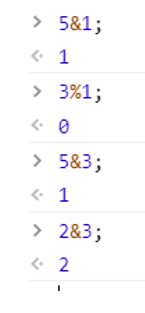
You can carry out these operations to verify the results. You simply need to change the decimal numbers to their binary equivalent and perform bit by bit operation and convert the binary so obtained back to decimal.
您可以执行这些操作来验证结果。 您只需要将十进制数更改为等效的二进制数,然后逐位执行操作,然后将如此获得的二进制数转换回十进制数即可。
The Bitwise OR does logical addition of two binary numbers.
按位或对两个二进制数进行逻辑加法运算。
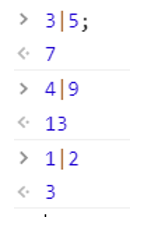
The Bitwise XOR gives 1 if the bits are different and 0 otherwise.
如果这些位不同, 则按位XOR给出1,否则返回0。
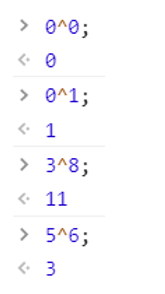
The Bitwise NOT ~ simply inverses all bits of the binary representation of the number. Wherever you have a 0, you'll have a 1 and vice versa.
按位NOT〜只是将数字的二进制表示形式的所有位求反。 无论您有0哪里,您都会有1,反之亦然。
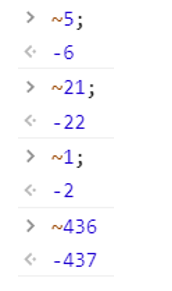
Notice, how NOT ~ of a number gives us the negative of that number incremented by 1? This can be logically verified concerning how the computer stores a negative number.
请注意,数字的非〜怎么给我们该数字的负数加1? 可以从逻辑上验证有关计算机如何存储负数的信息。
<< or the left shift operator pushes all the bits to the right and the leftmost one falls off.
<<或左移位运算符将所有位向右推,最左边的一位掉落。
Binary of 5: 0101 5<<1=> 1010 which is the binary representation of 10.
5的二进制: 0101 5 << 1 => 1010 ,它是10的二进制表示形式。
Similarly, the right shift >> operator pushes all the bits to the left and rightmost falls off.
类似地, 右移>>运算符将所有位推到最左边,最右边下降。
Binary of 5: 0101 5>>1=> 0010 which is the binary representation of 2.
5的二进制数: 0101 5 >> 1 => 0010 ,它是2的二进制表示形式。
Let's verify these,
让我们验证一下,
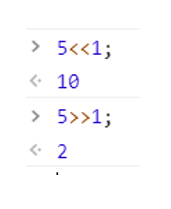
What if you left shift a certain number a b times? Or right shift a b times?
如果您左移了某个数字ab次该怎么办? 还是右移AB次?
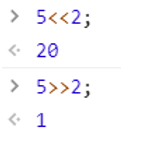
In general, we can say a<<b is a*(2^b). If a=5, b=2 then 5*(2^2) which gives 5*4=20.
通常,我们可以说a << b是a *(2 ^ b) 。 如果a = 5 , b = 2然后5 *(2 ^ 2)给出5 * 4 = 20 。
Similarly, we can say a>>b is a/(2^b). If a=5, b=2 then 5/(2^2) which gives 5/4=1.
类似地,我们可以说a >> b是a /(2 ^ b) 。 如果a = 5,b = 2然后5 /(2 ^ 2)给出5/4 = 1 。
Okay now let's write a simple function that accepts two numbers and performs all the bitwise operations we have discussed so far.
好吧,现在让我们编写一个简单的函数,该函数接受两个数字并执行到目前为止讨论的所有按位运算。
function bitwiseOperations(a=2,b=3){
console.log('${a} AND ${b} : ',a&b);
console.log('${a} OR ${b} : ',a|b);
console.log('${a} XOR ${b} : ',a^b);
console.log('NOT ${a} : ',~a);
console.log('NOT ${b} : ',~b);
console.log('Left Shift ${a} : ',a<<1);
console.log('${a} left shift ${b} : ',a>>b);
console.log('${a} right shift ${b} : ',a<<b);
}
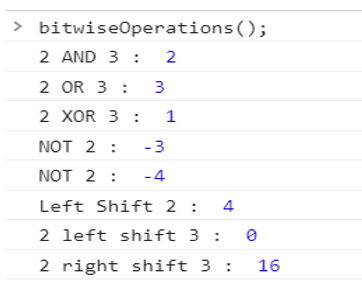
Let's look at the application of the bitwise operator. Let's say we have an array in which every number is repeated twice except for one number that occurs only once. We need to find that unique number. There can be different approaches to this problem but an efficient method would be to XOR all elements of the array. Let's see this with an example,
让我们看一下按位运算符的应用。 假设我们有一个数组,其中每个数字重复两次,但一个数字仅出现一次。 我们需要找到该唯一编号。 可以使用不同的方法来解决此问题,但有效的方法是对数组的所有元素进行XOR 。 让我们来看一个例子,
Say we have an array with the elements: 1,5,6,2,5,6,3,2,3
假设我们有一个包含以下元素的数组: 1,5,6,2,5,6,3,2,3
Clearly, the unique number is 1.
显然,唯一数字是1 。
If we XOR all these numbers together due to the same bits in the repeated numbers, they will give 0.
如果由于重复数字中的相同位而将所有这些数字异或 ,它们将给出0 。
5^5=6^6=3^3=2^2=0.
5 ^ 5 = 6 ^ 6 = 3 ^ 3 = 2 ^ 2 = 0 。
And, when we XOR 1 and 0, we'll get 1. This way we can easily find the unique number.
而且,当我们对1和0进行 XOR运算时,将得到1 。 这样,我们可以轻松找到唯一编号。
Let's implement this solution,
让我们实施这个解决方案,
function findUnique(arr){
var ans=0;
for(let i=0; i<arr.length; i++)
ans=ans^arr[i];
return ans;
}
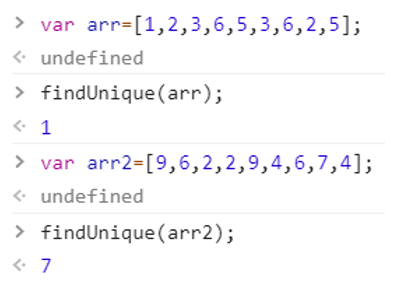
翻译自: https://www.includehelp.com/code-snippets/bitwise-operators-in-javascript.aspx
javascript运算符