vc++ 6.0 堆栈
To implement a stack using a linked list, basically we need to implement the push() and pop() operations of a stack using linked list.
要使用链接列表实现堆栈 ,基本上,我们需要使用链接列表实现堆栈的push()和pop()操作。
Example:
例:
Input numbers: 1,3,5,8,4,0
输入数字:1,3,5,8,4,0
We push the numbers into the stack and whenever it executes a pop() operation, the number is popped out from the stack.
我们将数字压入堆栈,每执行一次pop()操作,数字就会从堆栈中弹出。
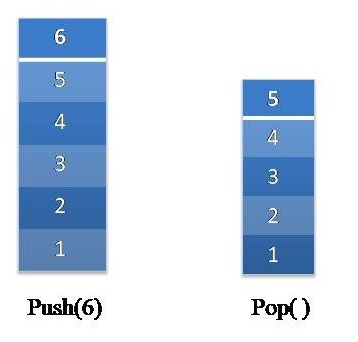
Algorithm:
算法:
To implement the push() operation:
要实现push()操作 :
If the Linked list is empty then create a node and point it as head of that Linked List.
如果“链接列表”为空,则创建一个节点并将其指向该“链接列表”的头。
If the Linked List is not empty then create a node with the input number to be pushed and make it head of the Linked List.
如果“链接列表”不为空,则创建一个带有要推送的输入编号的节点,并使它成为“链接列表”的头。
To implement The pop() operation
实现pop()操作
If the Linked List is already empty then do nothing. Output that empty stack.
如果“链接列表”已经为空,则什么也不做。 输出该空堆栈。
If the Linked List is not empty then delete the node from head.
如果“链接列表”不为空,则从头删除该节点。
C++ implementation:
C ++实现:
#include<bits/stdc++.h>
using namespace std;
struct node{
int data;
node* next;
};
//Create a new node
struct node* create_node(int x){
struct node* temp= new node;
temp->data=x;
temp->next=NULL;
return temp;
}
//Enter the node into the linked list
void push(node** head,int x){
struct node* store=create_node(x);
if(*head==NULL){
*head =store;
return;
}
struct node* temp=*head;
//add the number in the front of the linked list
store->next=temp;
*head=store;
}
//pop from the stack
void pop(node** head){
if(*head==NULL)
return;
struct node* temp=(*head)->next;
*head=temp; //delete from the front
}
void print(node* head){
struct node* temp=head;
while(temp){
cout<<temp->data<<" ";
temp=temp->next;
}
}
int main()
{
struct node* l=NULL;
push(&l,1);
push(&l,2);
push(&l,3);
push(&l,4);
push(&l,5);
push(&l,6);
cout<<"Before the pop operation"<<endl;
print(l);
pop(&l);
pop(&l);
cout<<"\nAfter the pop operation"<<endl;
print(l);
return 0;
}
Output
输出量
Before the pop operation
6 5 4 3 2 1
After the pop operation
4 3 2 1
翻译自: https://www.includehelp.com/cpp-programs/implement-stack-using-linked-list-in-cpp.aspx
vc++ 6.0 堆栈