叶节点到根节点的路径
Problem statement:
问题陈述:
Given a Binary Tree of size N, write a program that prints all the possible paths from root node to the all the leaf node's of the binary tree.
给定大小为N的二叉树,编写一个程序, 打印从根节点到二叉树的所有叶节点的所有可能路径 。
Example:
例:
Let's the tree be like following:
让树如下所示:
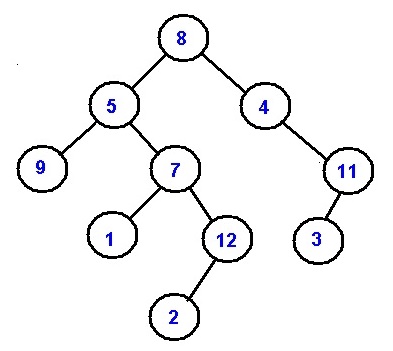
All possible root to leaf paths in this tree is:
此树中所有可能的从根到叶的路径是:
8->5->9
8->5->7->1
8->5->7->12->2
8->4->11->3
Solution:
解:
To print all the root to leaf paths we have used recursive approach.
为了将所有根打印到叶路径,我们使用了递归方法。
The idea is to maintain a list of nodes on the paths and to print the list while leaf node is reached.
想法是维护路径上的节点列表,并在到达叶节点时打印该列表。
Algorithm:
算法:
Pre-requisite:
先决条件:
Input binary tree root, list a
输入二叉树的根 ,列出一个
FUNCTION printpathrecursively(Node* current_node, list a){
1. base case
IF(current_node ==NULL)
return;
END IF
2. append current_node ->data to the list;
IFcurrent_nodeis leaf node //leaf node reached
Print the list; //printing the root to leaf path
END IF
3. printpathrecursively(current_node->left,a);//recur for left subtree
printpathrecursively(current_node->right,a);//recur for right subtree
END FUNCTION
In the main function create an empty list a, And call printpathrecursively(root, a);
在main函数中创建一个空列表a ,然后递归调用printpath(root,a);
Example with explanation:
带有说明的示例:
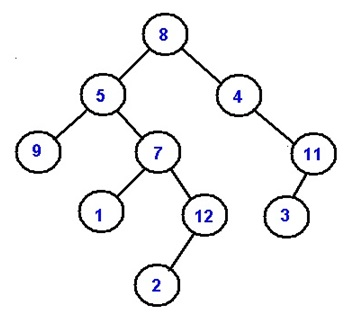
Nodes are represented by their respective values.
节点由它们各自的值表示。
In main we call printpathrecursively(root, a)
----------------------------------------------
printpathrecursively(8, a)
current node not NULL
append 8 to list a
list: 8
current node is not leaf node
call to printpathrecursively(8->left, a)
call to printpathrecursively(8->right, a)
----------------------------------------------
printpathrecursively(8->left, a):
=printpathrecursively(5, a)
current node not NULL
append 5 to list a
list: 8,5
current node is not leaf node
call to printpathrecursively(5->left, a)
call to printpathrecursively(5->right, a)
----------------------------------------------
printpathrecursively(5->left, a):
=printpathrecursively(9, a)
current node not NULL
append 9 to list a
list: 8,5, 9
current node is leaf node
Print the list
call to printpathrecursively(9->left, a)
call to printpathrecursively(9->right, a)
call to printpathrecursively(9->left, a)
----------------------------------------------
=printpathrecursively(NULL, a)
current nodeNULL
control return to FUNCTION printpathrecursively(9, a)
At printpathrecursively(9, a)
call to printpathrecursively(9->right, a)
----------------------------------------------
=printpathrecursively(NULL, a)
current node NULL
control return to FUNCTION printpathrecursively(9, a)
At printpathrecursively(9, a)
Control reaches end of the void function
Thus control returns to the calleefunction printpathrecursively(5, a)
printpathrecursively(5->right, a):
----------------------------------------------
=printpathrecursively(7, a)
current node not NULL
append 7 to list a
list: 8, 5, 7 //Don’t think list will be 8, 5, 9, 7.
List at this function was 8, 5 not 8, 5, 9
//List has not been passed by referenced
current node is not leaf node
call to printpathrecursively(7->left, a)
call to printpathrecursively(7->right, a)
This can be carried out until control gets back to main
which called function printpathrecursively(root, a)
You can do rest by your own to have much more clear idea about how the program is actually working.
您可以自己休息,以更清楚地了解程序的实际工作方式。
C++ Implementation:
C ++实现:
#include <bits/stdc++.h>
using namespace std;
//tree node
class Node{
public:
int data;
Node *left;
Node *right;
};
void print(vector<int> a){
for(int i=0;i<a.size();i++){
if(i==a.size()-1)
cout<<a[i];
else
cout<<a[i]<<"->";
}
}
void printpathrecursively(Node* root,vector<int> a){
if(root==NULL) //base case
return ;
a.push_back(root->data);
if(!root->left && !root->right){ //leaf node reached
print(a);
cout<<endl;
}
printpathrecursively(root->left,a);//recur for left subtree
printpathrecursively(root->right,a);//recur for right subtree
}
void printPaths(Node* root)
{
vector<int> a;
printpathrecursively(root,a);
}
//creating new nodes
Node* newnode(int data){
Node* node = (Node*)malloc(sizeof(Node));
node->data = data;
node->left = NULL;
node->right = NULL;
return(node);
}
int main() {
//**same tree is builted as shown in example**
cout<<"tree in the example is build here"<<endl;
//building the tree like as in the example
Node *root=newnode(8);
root->left= newnode(5);
root->right= newnode(4);
root->right->right=newnode(11);
root->right->right->left=newnode(3);
root->left->left=newnode(9);
root->left->right=newnode(7);
root->left->right->left=newnode(1);
root->left->right->right=newnode(12);
root->left->right->right->left=newnode(2);
cout<<"Printing all root to leaf paths......"<<endl;
printPaths(root);
return 0;
}
Output
输出量
tree in the example is build here
Printing all root to leaf paths......
8->5->9
8->5->7->1
8->5->7->12->2
8->4->11->3
翻译自: https://www.includehelp.com/icp/all-root-to-leaf-paths.aspx
叶节点到根节点的路径