二叉树右视图
Problem statement:
问题陈述:
Given a binary tree, print the bottom view from left to right.
给定一棵二叉树,从左到右打印底视图。
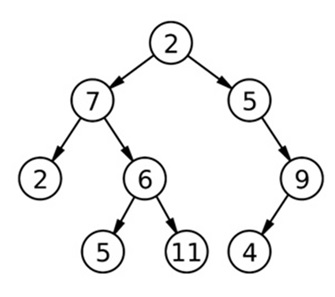
Example:
例:
In the above example the bottom view is:
2 5 6 11 4 9 (left to right)
Solution:
解:
What is bottom view?
什么是底视图?
Bottom view is not only the leaf nodes. We can consider the tree like below: (same as the problem of finding vertical sum)
底视图不仅是叶节点。 我们可以像下面这样考虑树:(与求垂直和的问题相同)
For the above tree, let's check what the vertical sum is for the tree,
对于上面的树,让我们检查一下树的垂直和,
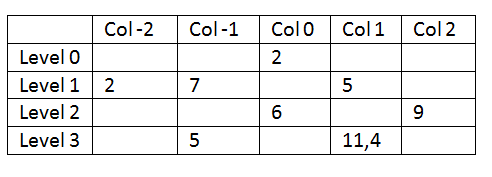
Just consider, we have partitioned the tree nodes into column & rows where rows are the level no starting from 0. Then the above tree can be converted into the above table easily.
考虑一下,我们将树节点划分为列和行,其中行是从0开始的级别。然后,可以将上面的树轻松转换为上面的表。
Start from root. Root column is 0.
从根开始。 根列为0。
If next node is at left of current node
如果下一个节点在当前节点的左侧
Then column of
然后的
next node = column of current node-1
下一个节点= 当前节点1的 列
Else
其他
Column of
栏目
next node = column of current node+1
下一个节点= 当前节点的 列 +1
Using the above steps the tree can be easily partitioned to the table. It is to observe that there may be several entries at a specific (row, column) position. Like here, at Level3, column no 1 has two entry 11 & 4.
使用上述步骤,可以轻松地将树分区到表中。 可以观察到在特定位置(行,列)可能存在多个条目。 像这里一样,在Level3,第1列有两个条目11和4。
Rest is about printing the last entry (bottommost) in each column. If there are multiple entries in the bottommost (in this case 11 & 4 for col 1) the last one entry is taken only.
剩下的就是在每列中打印最后一个条目(最底部)。 如果最底部有多个条目(本例中第1列为11和4),则仅取最后一个条目。
Thus the output should be 2, 5, 6, 4, 9 (from col -2 to col 2 direction).
因此,输出应为2、5、6、4、9(从col -2到col 2方向)。
Though the visual description seems to be very easy to solve this problem, in programming view it's not that easy.
尽管视觉描述似乎很容易解决此问题,但在编程视图中并不是那么容易。
Algorithm to find vertical sum
求垂直和的算法
The basic concept is to do pre-order traversal & while traversing we will keep track for each column (hashing) & make the entry.
基本概念是进行预遍历,遍历时我们将跟踪每个列(散列)并进行输入。
Thus the column no is used as key & we need a map to process our algorithm.
因此,列号用作键,我们需要一个映射来处理我们的算法。
Initialize map<int, int>hash. //(ordered map)
初始化map <int,int> hash 。 //(有序地图)
Initialize map<int, int>hash. //(ordered map)
初始化map <int,int> hash 。 //(有序地图)
Call
呼叫
findbottom(root, 0, hash);
findbottom(root,0,hash);
Recursive function
递归函数
Function findbottom( tree node, column, reference of map hash) a. If (node== NULL) Return; //hash[column].push_back(node->data) b. Add node value in the column list; // findbottom(node->left, column-1, reference of map hash) c. Recursive do for the left subtree; We are passing col-1 since it's on the left // findbottom(node->right, column+1, reference of map hash) d. Recursive do for the right subtree; We are passing col+1 since it's on the right End Function
Print the last entry only for each column to output the bottom view.
仅打印每列的最后一个条目以输出底视图。
C++ implementation
C ++实现
#include <bits/stdc++.h>
using namespace std;
class tree{
public:
int data;
tree *left;
tree *right;
};
void findbottom(tree* root,map<int,vector<int>>& m, int col){
if(!root)
return;
m[col].push_back(root->data);
findbottom(root->left,m,col-1);
findbottom(root->right,m,col+1);
}
void bottomView(tree *root)
{
// Your Code Here
map<int,vector<int>> m;
//m[0].push_back(root->data);
findbottom(root,m,0);
for(auto it=m.begin();it!=m.end();it++){
cout<<(it->second)[(it->second).size()-1]<<" ";
}
}
// creating new node
tree* newnode(int data)
{
tree* node = (tree*)malloc(sizeof(tree));
node->data = data;
node->left = NULL;
node->right = NULL;
return(node);
}
int main()
{
//**same tree is builted as shown in example**
cout<<"Tree is built like the example aforesaid"<<endl;
//building the tree like as in the example
tree *root=newnode(2);
root->left= newnode(7);
root->right= newnode(5);
root->right->right=newnode(9);
root->right->right->left=newnode(4);
root->left->left=newnode(2);
root->left->right=newnode(6);
root->left->right->left=newnode(5);
root->left->right->right=newnode(11);
cout<<"printing bottom view......"<<endl;
bottomView(root);
return 0;
}
Output
输出量
Tree is built like the example aforesaid
printing bottom view......
2 5 6 4 9
翻译自: https://www.includehelp.com/icp/bottom-view-of-binary-tree.aspx
二叉树右视图