Description:
描述:
In this article we are going to see how to compute minimum number of squares need to sum a number N? This article has been featured in interview rounds of Amazon, Wipro.
在本文中,我们将看到如何计算需要求和N的最小平方数? 亚马逊,Wipro的采访回合中都刊登了这篇文章。
Problem statement:
问题陈述:
Given a number N, write a program to print the minimum number of squares that sums to N.
给定数字N ,编写一个程序来打印求和为N的最小平方数。
Input:
N = 41
Output:
Minimum number of squares needed: 2
Explanation with example
举例说明
We can think similar way as the coin change problem (refer to my article on coin change). We can start with the greedy approach and can see whether it works or not.
我们可以用与硬币找零问题类似的方式思考(请参阅我有关硬币找零的文章)。 我们可以从贪婪的方法开始,看看它是否有效。
So, a greedy approach can be
因此,贪婪的方法可以是
First thing is to find whether we will be able to find answers for any number N. Is it possible to find squares that will sum up to N. Yes, we will be able to find for any number N because 1 is itself a square and we can get any number by summing 1?
第一件事是确定我们是否能够找到任意数量N的答案。 是否有可能找到总和为N的正方形。 是的,我们将能够找到任意数量的N,因为1本身就是一个正方形,并且通过求和1可以获得任意数量的数字吗?
Since we are to find the minimum number of squares it's apparent that we should pick the square with the maximum value closest to N and keep trying with that until the remaining sum is less the square. Then on course, we keep choosing the next best one. So, the algorithm would be like.
由于我们要找到最小的平方数,因此很明显,我们应该选择最大值最接近N的平方,并继续尝试直到剩余的总和小于平方。 然后,当然,我们会继续选择次佳的。 因此,该算法将是这样。
1) Let, count=0 to count minimum number of squares to sum
2) Pick up square x closest to N
3) While N≥x
N=N-x
count=count+1
4) if N=0
Go to Step 7
5) Pick up the next best square value <x & closest to N .assign it to x
6) Go to Step 2
7) End. count is the minimum number of squares needed to sum up.
Let's see whether the above greedy algorithm leads to the desired result or not.
让我们看看上面的贪婪算法是否导致了期望的结果。
N = 41
The square value closest to 41 is 36
count = 1, N = 5
Next best square value is 4
count = 2, N = 1
Next best square value is 1
count = 3, N = 0
So, minimum number of squares needed is 3
But is it really minimum?
If we choose 25 and 16, that sums up to 41 and we need only two squares.
That's the minimum, not 3.
So, greedy does not work as the local optimum choices doesn't make
global optimum choice. Hence, we need dynamic programing.
Problem Solution Approach
问题解决方法
In the coin change problem (refer to my article of coin change) we had an amount M and n number of coins, { C1, C2, ..., Cn} to make change. We can think this minimum square problem as we did in coin change problem. We can frame this problem lie the coin change problem, where, Amount M = Number N
在硬币找零问题(请参阅我的硬币找零文章)中,我们有M个和n个硬币数量{C 1 ,C 2 ,...,C n }进行找零。 我们可以像在硬币找零问题中那样思考这个最小平方问题。 我们可以将这个问题归结为硬币更换问题,其中, 金额M =数字N
Coin denominations { C1, C2, ..., Cn} = {x1, x2, ..., xn } where xi is square and xi<N
硬币面额{C 1 ,C 2 ,...,C n } = {x 1 ,x 2 ,...,x n }其中x i是正方形,x i <N
Now,
现在,
We have two choice for any xi,
对于x i ,我们有两种选择。
Use xi and recur for N-xi
使用x i并递归Nx i
Don't use xi and recur for N with other squares
不要使用x i并与其他平方重复使用N
f(n)= minimum number of squares needed to sum n
f(n)=总和n所需的最小平方数
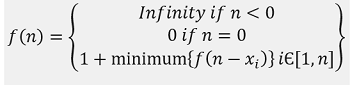
We can formulate the above recursion using DP.
我们可以使用DP来制定上述递归。
1) Initialize DP[N+1] with index value
[for any number 0 to N, minimum number of squares needed
is initially same as index no, i.e., minimum number squares
needed for number i=i( i times 1) ]
2) DP[0]=0 //base case of above recursion
3) Create X[n]with squares<N
4) for i=1 to N //iterate numbers
for squares xi = X[0] to X[n]
If i>=xi && DP[i-xi]+1<DP[i])
DP[i]=DP[i-xi]+1; //update value
End for
End for
The result is DP[N]
C++ implementation:
C ++实现:
#include <bits/stdc++.h>
using namespace std;
int min_square(int m)
{
int DP[m + 1];
//base value
for (int i = 1; i <= m; i++)
DP[i] = i;
DP[0] = 0;
//create the array of squares to be used to sum
vector<int> a;
for (int i = 1;; i++) {
if (i * i <= m)
a.push_back(i * i);
else
break;
}
int n = a.size();
for (int i = 1; i <= m; i++) { //iterate for the numbers
for (int j = 0; j < n; j++) { //iterate on the squares
if (i >= a[j] && DP[i - a[j]] + 1 < DP[i])
DP[i] = DP[i - a[j]] + 1;
}
}
//result
return DP[m];
}
int main()
{
int n, item, m;
cout << "Enter the number\n";
cin >> n;
int ans = min_square(n);
cout << "Minimum number of squares needed: " << ans << endl;
return 0;
}
Output
输出量
Enter the number
41
Minimum number of squares needed: 2
翻译自: https://www.includehelp.com/icp/get-minimum-squares.aspx