c程序排序算法
堆排序 (Heap sort)
Heap sort was invented by John Williams. Heap sort is a sorting technique of data structure which uses the approach just opposite to selection sort. The selection sort finds the smallest element among n elements then the smallest element among n-1 elements and so on. Until the end of the array heap sort finds the largest element and put it at end of the array, the second largest element is found and this process is repeated for all other elements
堆排序是约翰·威廉姆斯(John Williams)发明的。 堆排序是一种数据结构的排序技术,它使用与选择排序相反的方法。 选择排序是在n个元素中找到最小的元素,然后在n-1个元素中找到最小的元素,依此类推。 直到数组堆排序的末尾找到最大的元素并将其放在数组的末尾,才找到第二个最大的元素,并对所有其他元素重复此过程
什么是堆? (What is Heap?)
Heap is a complete binary tree in which every parent node be either greater or lesser than its child nodes. Heap can be of two types that are:
堆是一个完整的二叉树,其中每个父节点大于或小于其子节点。 堆可以有两种类型:
1) Max heap
1)最大堆
A max heap is a tree in which value of each node is greater than or equal to the value of its children node.
最大堆是一棵树,其中每个节点的值都大于或等于其子节点的值。
Example of max heap:
最大堆的示例:
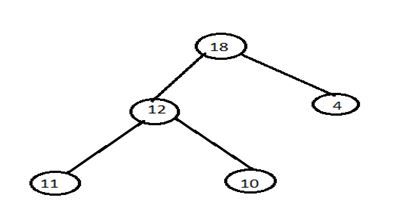
2) Min heap
2)最小堆
A min heap is a tree in which value of each node is less than or equal to the value of its children node.
最小堆是一棵树,其中每个节点的值小于或等于其子节点的值。
Example of min heap:
最小堆的示例:
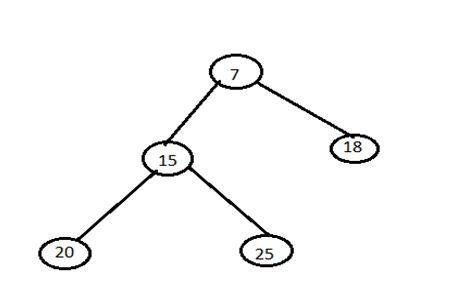
堆排序的工作 (Working of Heap Sort)
The heap sort begins by building a heap out of the data set and then removing the largest item and placing it at the end of the sorted array.
堆排序首先从数据集构建一个堆,然后删除最大的项并将其放在已排序数组的末尾。
After removing the largest item, it reconstructs the heap and removes the largest remaining item and places it in the next open position from the open position from the end of the sorted array.
删除最大项目后,它会重建堆并删除剩余的最大项目,并将其放置在已排序数组末尾的打开位置中的下一个打开位置。
This is repeated until there is no item left in the heap and the sorted array is full.
重复此操作,直到堆中没有剩余的项目并且已排序的数组已满。
Elementary implementation requires two arrays one to hold the heap and the other to hold the sorted elements.
基本实现需要两个数组,一个数组用于保存堆,另一个数组用于保存已排序的元素。
堆排序算法 (Algorithm for heap sort)
Procedure 1:
程序1:
INSHEAP(TREE, N, HEAP):
A heap H with N elements is stored in the array TREE, and VALUE information is given.
具有N个元素的堆H存储在数组TREE中,并给出VALUE信息。
This procedure inserts ITEM as a new element of H.
此过程将ITEM作为H的新元素插入。
PTR gives the location of ITEM as it rises in the tree, and PAR denotes the location of the parent of VALUE.
PTR给出了ITEM在树中上升时的位置,而PAR表示VALUE的父级的位置。
[Add new node to H and initialize PTR]
[向H添加新节点并初始化PTR]
Set N: N+1 and PTR: =N
设置N:N + 1和PTR:= N
[Find location to insert VALUE]
[查找要插入值的位置]
[Find location to insert VALUE]
[查找要插入值的位置]
Set PAR:=[PTR/2] {Location of parent node}
设置PAR:= [PTR / 2] {父节点的位置}
If VALUE <=TREE[PAR],Then:
如果VALUE <= TREE [PAR],则:
Set TREE[PTR]:=VALUE ,and return
设置TREE [PTR]:= VALUE,然后返回
Set TREE[PTR]:=TREE[PAR]
设置TREE [PTR]:= TREE [PAR]
Set PTR:=PAR
设置PTR:= PAR
[End of step 2 loop]
[第2步循环结束]
[Assign VALUE as the root of H]
[将值指定为H的根]
Set TREE[1]:=VALUE
设置TREE [1]:= VALUE
Return
返回
Procedure 2:
步骤2:
DELHEAP(TREE,N,VALUE):
A Heap H with N elements is stored in the array TREE.
具有N个元素的堆H存储在数组TREE中。
This procedure assigns the root TREE [1] of H to the variable VALUE and then reheaps the remaining elements.
此过程将H的根TREE [1]分配给变量VALUE,然后重堆其余元素。
The variable LAST saves the value of the original last node of H.
变量LAST保存H的原始最后一个节点的值。
The pointer PTR, LEFT and RIGHT gives the location of LAST and its left and right children as LAST sinks in the tree.
指针PTR,LEFT和RIGHT给出LAST的位置以及LAST在树中下沉时的左右子项。
Set VALUE:=TREE[1]
设置VALUE:= TREE [1]
Set LAST:=TREE[N] and N :=N-1
设置LAST:= TREE [N]和N:= N-1
Set PTR:=1,LEFT:=2 and RIGHT:=3
设置PTR:= 1,LEFT:= 2和RIGHT:= 3
Repeats steps 5 to 7 while RIGHT<=N:
在RIGHT <= N时重复步骤5至7:
If LAST >=TREE[LEFT] and LAST>=TREE [RIGHT], then:
如果LAST> = TREE [LEFT]而LAST> = TREE [RIGHT],则:
Set TREE[PTR]:=LAST and return
设置TREE [PTR]:= LAST并返回
If TREE [RIGHT]<=TREE[LEFT],then:
如果TREE [RIGHT] <= TREE [LEFT],则:
Set TREE[PTR]:=TREE[LEFT] and PTR:=LEFT
设置TREE [PTR]:= TREE [LEFT]和PTR:= LEFT
Else:
其他:
Set TREE[PTR]:=TREE[RIGHT] and PTR:=RIGHT
设置TREE [PTR]:= TREE [RIGHT]和PTR:= RIGHT
Set LEFT:=2*PTR and RIGHT:=LEFT+1
设置LEFT:= 2 * PTR和RIGHT:= LEFT + 1
If LEFT=N and if LAST<TREE[LEFT],then: set PTR :=LEFT
如果LEFT = N并且LAST <TREE [LEFT],则:设置PTR:= LEFT
Set TREE[PTR]:=LAST
设置TREE [PTR]:= LAST
Return
返回
Algorithm
算法
HEAPSORT (A, N):
An array A with N elements is given.
给出具有N个元素的数组A。
This algorithm sorts the element of A.
该算法对A的元素进行排序。
[Build a heap A ,using a procedure 1]
[使用过程1构建堆A]
Repeat for J=1 to N-1
重复J = 1至N-1
Call INSHEAP(A, J, A[J+1])
致电INSHEAP(A,J,A [J + 1])
[sort A by repeatedly deleting the root of H, using procedure 2]
[使用过程2通过重复删除H的根来对A进行排序]
Repeat while N>1:
当N> 1时重复:
Call DELHEAP(A , N,VALUE)
呼叫DELHEAP(A,N,VALUE)
Set A[n+1]:=value
设置A [n + 1]:=值
Exit
出口
堆排序的性能 (Performance of heap sort)
Heap Sort has O(nlogn) time complexities for all the cases (best case, average case and worst case).
对于所有情况(最佳情况,平均情况和最坏情况),堆排序都具有O(nlog n )时间复杂度。
用C语言进行堆排序的程序 (Program for heap sort in C language)
#include<stdio.h>
void create(int []);
void down_adjust(int [],int);
int main()
{
int heap[30],n,i,last,temp;
printf("Enter no. of elements:");
scanf("%d",&n);
printf("\nEnter elements:");
for(i=1;i<=n;i++)
scanf("%d",&heap[i]);
//create a heap
heap[0]=n;
create(heap);
//sorting
while(heap[0] > 1)
{
//swap heap[1] and heap[last]
last=heap[0];
temp=heap[1];
heap[1]=heap[last];
heap[last]=temp;
heap[0]--;
down_adjust(heap,1);
}
//print sorted data
printf("\nArray after sorting:\n");
for(i=1;i<=n;i++)
printf("%d ",heap[i]);
return 0;
}
void create(int heap[])
{
int i,n;
n=heap[0]; //no. of elements
for(i=n/2;i>=1;i--)
down_adjust(heap,i);
}
void down_adjust(int heap[],int i)
{
int j,temp,n,flag=1;
n=heap[0];
while(2*i<=n && flag==1)
{
j=2*i; //j points to left child
if(j+1<=n && heap[j+1] > heap[j])
j=j+1;
if(heap[i] > heap[j])
flag=0;
else
{
temp=heap[i];
heap[i]=heap[j];
heap[j]=temp;
i=j;
}
}
}
Output
输出量
Enter no. of elements:5
Enter elements:12 8 46 3 7
Array after sorting:
7 8 12 23 46
翻译自: https://www.includehelp.com/data-structure-tutorial/heap-sort-introducntion-algorithm-example.aspx
c程序排序算法