jquery-css
In the previous articles, we have talked about JQuery selectors and how to add content to the HTML DOM using JQuery. In this article, we'll learn how to use CSS with JQuery to style elements on the DOM dynamically? We'll practice this on the mini pokedex we were building last time so make sure you have this code with you,
在之前的文章中,我们讨论了JQuery选择器以及如何使用JQuery向HTML DOM添加内容。 在本文中,我们将学习如何结合使用CSS和JQuery在DOM上动态设置元素样式? 我们将在上次构建的微型pokedex上进行练习,因此请确保您拥有此代码,
index.html:
index.html:
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<!-- Compiled and minified CSS -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/materialize/1.0.0/css/materialize.min.css">
<!-- Compiled and minified JavaScript -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/materialize/1.0.0/js/materialize.min.js"></script>
<title>Pokedex</title>
<style>
body {
background: whitesmoke;
}
.card-image img {
height: 200px;
width: 200px;
}
</style>
</head>
<script>
var id = 0;
</script>
<body>
<div class="container">
<h1 class="center">My Pokedex</h1>
<div class="row cards">
<div class="col l3">
<div class="card">
<div class="card-image">
<img src="https://assets.pokemon.com/assets/cms2/img/pokedex/full/007.png">
</div>
<div class="card-content" id="1">
<p class="center name">Squirtle</p>
</div>
</div>
</div>
<div class="col l3">
<div class="card">
<div class="card-image">
<img src="https://giantbomb1.cbsistatic.com/uploads/scale_small/13/135472/1891761-004charmander.png">
</div>
<div class="card-content" id="2">
<p class="center name">Charmander</p>
</div>
</div>
</div>
<div class="col l3">
<div class="card">
<div class="card-image">
<img src="https://d2skuhm0vrry40.cloudfront.net/2019/articles/2019-10-31-15-44/pokemon_sword_shield_starters_grookey.jpg/EG11/resize/300x-1/quality/75/format/jpg">
</div>
<div class="card-content" id="3">
<p class="center name">Grookey</p>
</div>
</div>
</div>
<div class="col l3">
<div class="card">
<div class="card-image">
<img src="https://pokemonletsgo.pokemon.com/assets/img/common/char-eevee.png">
</div>
<div class="card-content" id="4">
<p class="center name" id="4">Eevee</p>
</div>
</div>
</div>
</div>
<div class="row cards">
<div class="col l3">
<div class="card">
<div class="card-image">
<img src="https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcQIuCM0zB4HkGx85Z8D6EdRVrlGxAUWT1dbwZcZFNzonHle__6Uhg&s">
</div>
<div class="card-content" id="5">
<p class="center name">Wigglytuff</p>
</div>
</div>
</div>
<div class="col l3">
<div class="card">
<div class="card-image">
<img src="https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcQcvGrOH5M64dkU9a-XC-P5rDkPLABag47e88zGybJtFbWPjsUh&s">
</div>
<div class="card-content" id="6">
<p class="center name">Galarian Ponyta</p>
</div>
</div>
</div>
<div class="col l3">
<div class="card">
<div class="card-image">
<img src="https://pokemonletsgo.pokemon.com/assets/img/common/char-pikachu.png">
</div>
<div class="card-content" id="7">
<p class="center name">Pikachu</p>
</div>
</div>
</div>
<div class="col l3">
<div class="card">
<div class="card-image">
<img src="https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcRiOrI-gcZubLIku3Ogxl6SLC2_ZmDgNjrPpk095qBxuxKX8lxmvA&s">
</div>
<div class="card-content" id="8">
<p class="center name">Mew</p>
</div>
</div>
</div>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<script src="index.js"></script>
</body>
</html>
Output
输出量
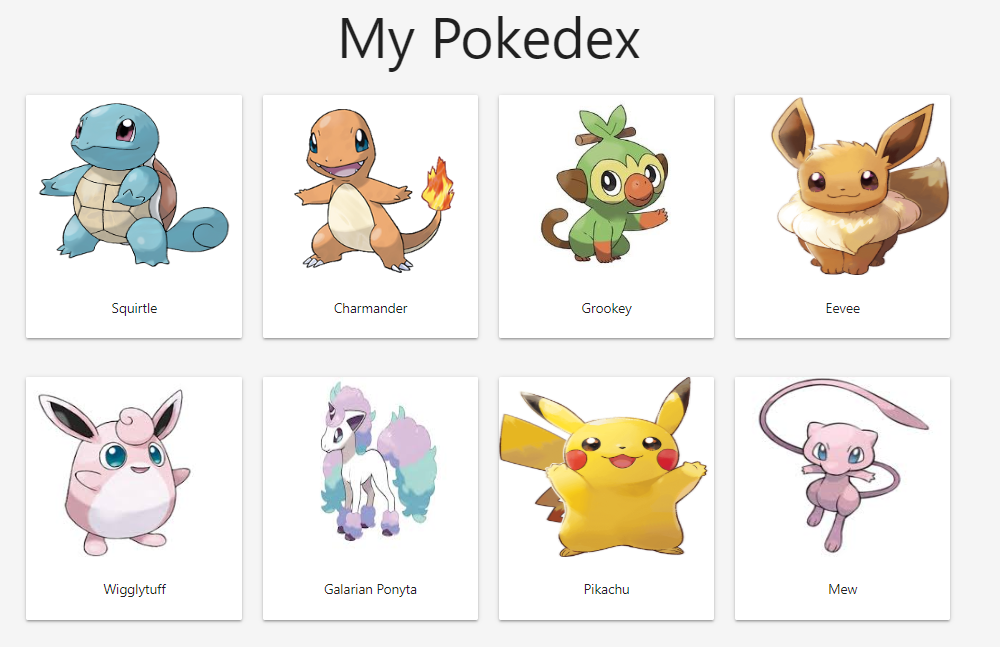
I have named all the pokemons (hopefully they're right). Make sure you have cleared the index.js from the previous article. The first thing I want to show is how to hide and unhide elements. If we were doing it with JavaScript we'd simply grab a hold of that element and onto its style property set the display to none. Let's first get a reference to these cards using JQuery,
我已经命名了所有的宠物小精灵(希望它们是正确的)。 确保已清除上一篇文章中的index.js。 我要展示的第一件事是如何隐藏和取消隐藏元素。 如果我们使用JavaScript进行操作,我们将简单地抓住该元素并将其style属性设置为none。 首先让我们使用JQuery来参考这些卡,
const cards=$('.card');
cards[0].style.display="none";
Output: The first card is removed from the DOM.
输出:第一张卡已从DOM中删除。
This is how we'd normally do using vanilla JS. Let's do this using the JQuery hide() method which hides a certain element from the DOM. Before that, notice that I have all the cards under a class name ‘cards' that I gave myself. We can use this class to get a reference to all the cards on the DOM. This would be a great workaround for the exercise for the previous article. Let's target this first,
这就是我们通常使用香草JS的方式。 让我们使用JQuery hide()方法执行此操作,该方法从DOM中隐藏某个元素。 在此之前,请注意,我所有的卡都属于我给自己的类名“卡”。 我们可以使用此类来获取对DOM上所有卡的引用。 对于上一篇文章的练习,这将是一个很好的解决方法。 让我们首先瞄准
const cards=$('.cards');
console.log(cards);
Output:
输出:
k.fn.init(2) [div.row.cards, div.row.cards, prevObject: k.fn.init(1)]
0: div.row.cards
1: div.row.cards
length: 2
prevObject: k.fn.init [document]
__proto__: Object(0)
You can see the 0th element gives us the first row of pokemons and 1st element gives us the second row. We can all the elements in a single index by putting both the rows inside a div with the class 'cards'. For the time being let's stick to what we have already done.
您可以看到第0个元素给了我们口袋妖怪的第一行,第一个元素给了我们第二行。 通过将两行都放在带有“ cards”类的div中,我们可以将所有元素包含在一个索引中。 暂时让我们坚持已经完成的工作。
Let's say your poor squirtle lost a hefty battle. You can't have it on your team anymore as it needs rest and time to heal. We can remove the squirtle from the pokedex by using a JQuery filter. Filters are nothing but pseudo-classes in CSS like :first, :nth-child, :first-child etc. They are preceded by a colon. Inside the cards, variable the first card is that of the squirtle so we can easily remove it by using the JQuery selector and adding the JQuery CSS hide method to it. Let's do this,
假设您的可怜的松鼠输掉了一场艰苦的战斗。 您再也无法在团队中使用它了,因为它需要休息和时间才能恢复。 我们可以通过使用JQuery过滤器从pokedex中删除蠕虫。 过滤器只不过是CSS中的伪类,如:first , :nth-child , :first-child等。它们以冒号开头。 在卡片内部,变量的第一张卡片是松鼠的卡片,因此我们可以通过使用JQuery选择器并向其中添加JQuery CSS hide方法轻松地将其删除。 我们开工吧,
$('.cards :first').hide();
Output
输出量
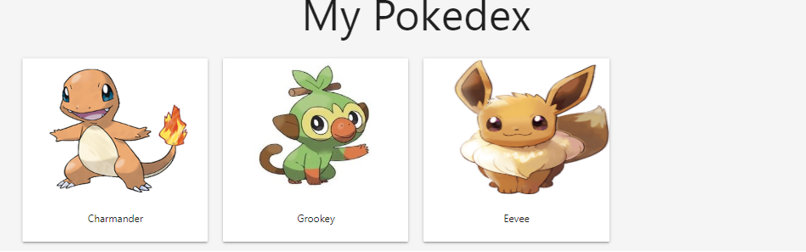
Voila! The squirtle is no longer part of the pokedex. Let's put it back using the show() method,
瞧! 蠕虫不再是pokedex的一部分。 让我们使用show()方法将其放回去,
$('.cards :first').show();
Output
输出量
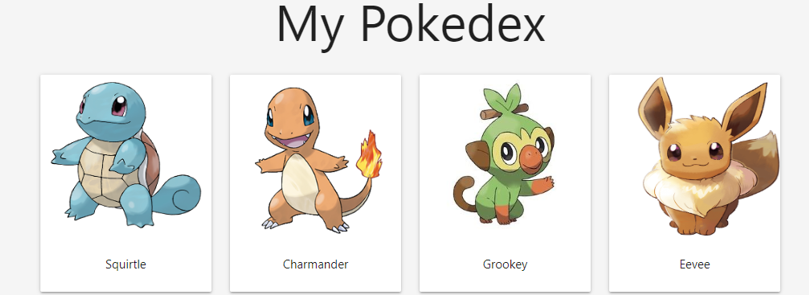
Great. We can now hide and show elements using the hide() and show() methods in JQuery. Let's style our elements using JQuery CSS now.
大。 现在,我们可以使用JQuery中的hide()和show()方法来隐藏和显示元素。 现在让我们使用JQuery CSS设置元素的样式。
I want the title to be a bit smaller. So let's give it a font size of 20px.
我希望标题要小一些。 因此,让我们给它一个20px的字体大小。
$('h1').css('font-size','20px');
Output
输出量
My Pokedex
The next thing I want is to give all these cards some background color. Typically the background color represents the type for a particular Pokemon for ex green for a grass type, blue for water type etc. So let's do this,
我想做的下一件事是给所有这些卡提供一些背景颜色。 通常,背景色代表特定口袋妖怪的类型,例如草绿色代表绿色,水代表蓝色,等等。
$('.cards :first').css('background','#90caf9');
Output
输出量
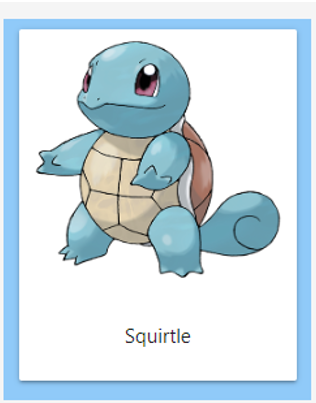
Our squirtle now has a background of blue because it's water type! Cool. Notice how the card inside remains uncolored. Can you figure out why?
现在我们的乌龟是蓝色的,因为它是水! 凉。 请注意里面的卡如何保持不变色。 你能弄清楚为什么吗?
This is because of the exact element we're setting the background color of. We're actually setting the background color of the element wrapping the card because of our selector. Let's use some filters to target the exact card now and give an electric yellow background color to our pikachu.
这是因为我们要为其设置背景色的确切元素。 实际上,由于选择器的原因,我们实际上是在设置包装卡片的元素的背景色。 现在让我们使用一些滤镜来定位确切的卡片,并为我们的皮卡丘提供一个黄色的背景色。
$('body > div > div:nth-child(3) > div:nth-child(3) > div').css('background-color','#ffea00');
Output
输出量
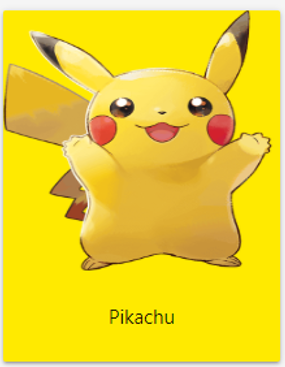
You can now see the entire card having a background color. The element we're targeting actually decides how the CSS properties are set. As an exercise, try to find out the types of all the remaining Pokemon and give them a background color for the same.
现在,您可以看到整个卡具有背景色。 我们要定位的元素实际上决定了CSS属性的设置方式。 作为练习,请尝试找出所有剩余的神奇宝贝的类型,并为其指定背景色。
翻译自: https://www.includehelp.com/code-snippets/jquery-css.aspx
jquery-css