给定序列二叉排序树的构建
Description: Solution to check the given Binary Search tree is balanced or not.
说明:检查给定的二叉搜索树是否平衡的解决方案。
Problem Statement: Write a program that accepts input from user to form a binary search tree and check whether the formed tree is balanced or not.
问题陈述:编写一个程序,接受用户的输入以形成二叉搜索树,并检查形成的树是否平衡 。
Example 1:
范例1:
Input: 2 1 3 4 5 -1
输入:2 1 3 4 5 -1
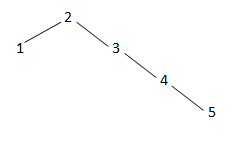
Output: Given BST is Balanced : False
输出:给定BST是平衡的:False
Example 2:
范例2:
Input: 2 1 3 4 -1
输入:2 1 3 4 -1
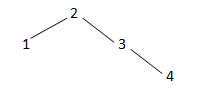
Output: Given BST is Balanced : True
输出:鉴于BST已平衡:True
Explanation...
说明...
What do you mean by height of a tree?
一棵树的高度是什么意思?
A height of a tree is the largest number of edges in a path from node to a leaf node. If a tree has only one node: i.e. root node itself then the height of tree is 0.
树的高度是从节点到叶节点的路径中最大的边数。 如果一棵树只有一个节点:即根节点本身,则树的高度为0。
Example:
例:
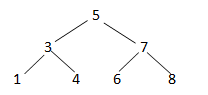
Height of tree: 2 ( maximum 2 edges from root to leaf)
树的高度:2 (从根到叶最多2个边缘)
Check when tree is balanced:
检查树何时平衡:
A non-empty binary search tree is said to be balanced if:
非空二进制搜索树在以下情况下被认为是平衡的:
Its left subtree is balanced.
它的左子树是平衡的。
Its Right subtree is balanced.
它的Right子树是平衡的。
The difference between heights of left and right subtree is less than or equal to 1.
左右子树的高度之差小于或等于1。
Example:
例:
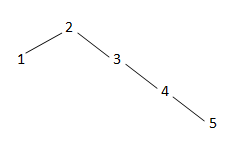
At root height of left subtree is 1 , whereas height of right subtree is 3
左子树的根高度为1,而右子树的高度为3
Difference = 3-1=2 (which is greater than 1) i.e. Tree is not balanced.
差异= 3-1 = 2(大于1),即树不平衡。
Algorithm:
算法:
Set root=root of given binary search tree.
设置root =给定二进制搜索树的根 。
Set leftHt= height of left subtree.
设置leftHt =左子树的高度 。
Set rightHt= height of right subtree.
设置rightHt =右子树的高度 。
if abs(leftHt – rightHt ) > 1
如果abs(leftHt – rightHt)> 1
return false;
返回false;
else isHeightBalanced(root->left) &&isHeightBalanced(root->right)
else isHeightBalanced(root-> left)&& isHeightBalanced(root-> right)
C++ Code
C ++代码
#include <iostream>
#include <cmath>
using namespace std;
class TreeNode {
public:
int data;
TreeNode* left;
TreeNode* right;
TreeNode(int d) {
data = d;
left = NULL;
right = NULL;
}
};
TreeNode* insertInBST(TreeNode* root, int x) {
if (root == NULL) {
TreeNode* tmp = new TreeNode(x);
return tmp;
}
if (x < root->data) {
root->left = insertInBST(root->left , x);
return root;
} else {
root->right = insertInBST(root->right, x);
return root;
}
}
TreeNode* createBST() {
TreeNode* root = NULL;
int x;
cin >> x;
//Take input until user inputs -1
while (true) {
if (x == -1) break;
root = insertInBST(root, x);
cin >> x;
}
return root;
}
//Calculate height of tree with given root
int height(TreeNode* root) {
if (root == NULL) return 0;
int leftHt = height(root->left);
int rightHt = height(root->right);
int curHt = max(leftHt, rightHt) + 1;
return curHt;
}
//Check whether tree is balanced or not
bool isHeightBalanced(TreeNode* root) {
if (!root) {
return true;
}
int leftHt = height(root->left);
int rightHt = height(root->right);
if (abs(leftHt - rightHt) > 1)
return false;
return isHeightBalanced(root->left) && isHeightBalanced(root->right);
}
int main() {
//Input BST
cout<<"Input Tree elements : ";
TreeNode* root = createBST();
cout << "Given BST is Balanced : ";
if (isHeightBalanced(root)) {
cout << "True";
}
else {
cout << "False";
}
return 0;
}
Output
输出量
First run:
Input Tree elements(stop taking input when -1 encountered) : 2 1 3 4 5 -1
Given BST is Balanced : False
Second run:
Input Tree elements(stop taking input when -1 encountered) : 2 1 3 4 -1
Given BST is Balanced : True
翻译自: https://www.includehelp.com/cpp-programs/given-binary-search-tree-is-balanced.aspx
给定序列二叉排序树的构建