In this article, we'll see how to change the class of an element using JavaScript? Through HTML classes we can group certain elements and style them collectively. Dynamically changing classes through JavaScript offers a great user experience on the client-side.
在本文中,我们将看到如何使用JavaScript更改元素的类? 通过HTML类,我们可以对某些元素进行分组并共同设置样式。 通过JavaScript动态更改类可在客户端上提供出色的用户体验。
Let's learn how to do it through a practical example,
让我们通过一个实际的例子来学习如何做到这一点,
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<!-- Compiled and minified CSS -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/materialize/1.0.0/css/materialize.min.css">
<!-- Compiled and minified JavaScript -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/materialize/1.0.0/js/materialize.min.js"></script>
</head>
<style>
body {
background: black;
}
span {
font-weight: bolder;
}
.rule-yellow {
position: relative;
left: 200px;
}
ul {
font-size: 24px;
}
.to-do {
color: tomato;
}
</style>
<body>
<div class="container">
<h2 class="center white">Tasks</h2>
<h5 class="center white-text">Let's mark our tasks in progress, completed and yet to be touched</h5>
<br>
<br>
<br>
<p class="white-text">Remeber to follow the rules:</p>
<span style="color: tomato" class="left rule-red">Red for not yet started</span>
<span style="color:yellow" class="center rule-yellow">Yellow for under progress</span>
<span style="color: green" class="right rule-green">Green for completed</span>
<br>
<br>
<br>
<br>
<ul class="collection tasks white center">
<li class="collection-item to-do">Study for end semester</li>
<li class="collection-item to-do">Learn JavaScript on includehelp.com</li>
<li class="collection-item to-do">Take cooper for a walk</li>
<li class="collection-item to-do">Solve sudoku</li>
</ul>
</div>
</body>
<script>
</script>
</html>
Output
输出量
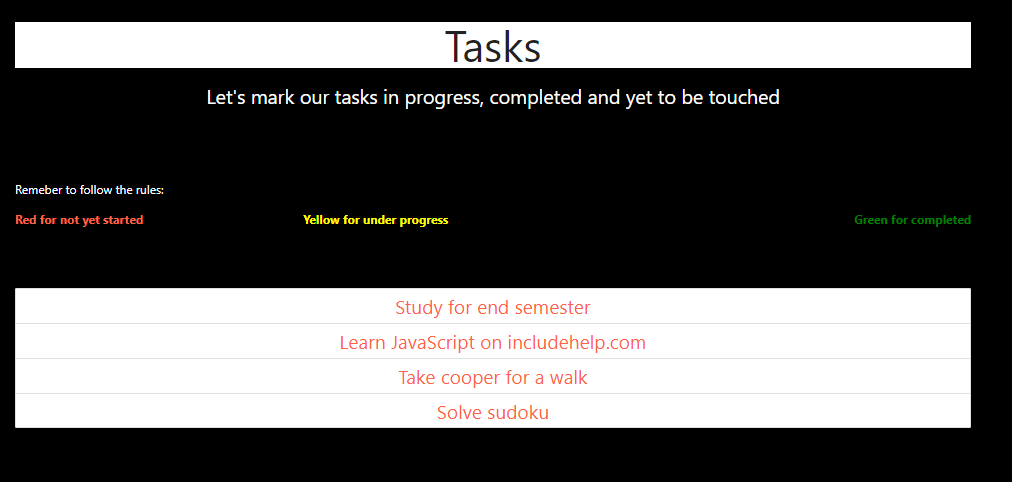
We have created a very naive task manager where we can mark tasks as done, not yet started or under progress by changing the font color of that task. Before we get started I want you to quickly take a look at the styles I wrote for this,
我们创建了一个非常幼稚的任务管理器,在其中可以通过更改任务的字体颜色将任务标记为已完成,尚未启动或正在进行中。 在我们开始之前,我希望您快速了解一下我为此编写的样式,
.to-do{
color: tomato;
}
You can see that this class is responsible for setting the font color of our tasks as red. This also indicates that we have not started on the task yet. Let's add two more classes that will set the font color to yellow and green for marking them as under progress and completed respectively.
您可以看到此类负责将我们的任务的字体颜色设置为红色。 这也表明我们尚未开始该任务。 我们再添加两个类,将字体颜色设置为黄色和绿色,分别将它们标记为进行中和完成。
.in-progress {
color: yellow;
}
.completed {
color: green;
}
Great. Now we're done. Next thing we need is a reference to our list,
大。 现在我们完成了。 接下来我们需要参考列表
<script>
const tasks=document.querySelectorAll('.tasks li');
console.log(tasks);
</script>
Output
输出量
NodeList(4) [li.collection-item.to-do, li.collection-item.to-do, li.collection-item.to-do, li.collection-item.to-do]
0: li.collection-item.to-do
1: li.collection-item.to-do
2: li.collection-item.to-do
3: li.collection-item.to-do
length: 4
We can now access the individual li's through their index. Let's say you're done studying for your end sems. We need to get our first li and change its name from to-do to completed.
现在,我们可以通过其索引访问单个li。 假设您已经完成了针对结束语的学习。 我们需要获得我们的第一个li,并将其名称从“待办事项”更改为“完成”。
tasks[0].classList.add('completed').remove('to-do');
Output
输出量

Our first task is marked completed! Cool. Since you're always learning JS let's mark the second task as under progress.
我们的第一个任务已标记为完成! 凉。 由于您一直在学习JS,因此让我们将第二项任务标记为正在进行中。
tasks[1].classList.add('in-progress').remove('to-do');
Output
输出量

What we're doing is adding the new class and removing the old class and in essence we're changing the class!
我们正在做的是添加新类并删除旧类,从本质上讲,我们正在更改类!
We can also use the toggle() method to add or remove that class if it does not exist. Let's say you solved the sudoku so mark it completed,
我们也可以使用toggle()方法添加或删除该类(如果不存在)。 假设您解决了数独问题,因此将其标记为完成,
<li class="collection-item completed">Solve sudoku</li>
Output
输出量

Oops, you solved it wrong! We can add it back to the task list by toggling the completed class,
糟糕,您解决错了! 我们可以通过切换完成的类将其添加回任务列表,
tasks[3].classList.toggle('completed');
Output
输出量

Thus we have implemented an easy functionality by just changing css classes through JavaScript. You can extend this functionality by adding event listeners which when triggered change these classes for the elements they were clicked on. Sounds like an idea for a great JavaScript project!
因此,我们仅通过JavaScript更改了CSS类就实现了简单的功能。 您可以通过添加事件侦听器来扩展此功能,该事件侦听器在触发时会更改这些类,以使其单击时会更改其元素。 听起来像是一个很棒JavaScript项目的主意!
翻译自: https://www.includehelp.com/code-snippets/how-to-change-the-class-of-an-element-in-javascript.aspx