c++枚举和c语言枚举
Hey, folks! Hope you all are doing well. In this article, we will be discussing Enumeration in C .
嘿伙计! 希望大家一切都好。 在本文中,我们将讨论C中的枚举 。
什么是枚举? (What is Enumeration?)
Enumeration in C is a user-defined data type. Thus, we can customize the data and store it into it according to the needs of the user/system.
C中的枚举是用户定义的数据类型。 因此,我们可以根据用户/系统的需求自定义数据并将其存储到其中。
Enumeration assigns string or character values i.e. enumeration tags to the integer data values. For every string literal or value, there is an integer index assigned to it as seen below.
枚举将字符串或字符值(即枚举标签)分配给整数数据值。 对于每个字符串文字或值,都有一个分配给它的整数索引,如下所示。
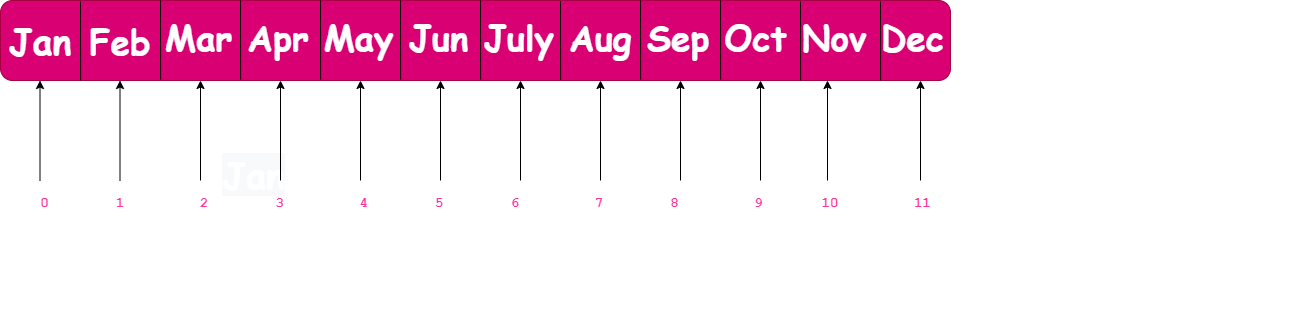
Like a C array, enum starts the index values from 0.
像C数组一样 ,枚举从0开始索引值。
Thus, enum helps us to simplify the code and increase the readability of the program.
因此,枚举可帮助我们简化代码并提高程序的可读性。
Now, let us understand the structure of Enumeration in C programming.
现在,让我们了解C编程中的枚举的结构。
C中的枚举语法 (Syntax of Enumeration in C)
So, now let us have a look at the declaration of Enum in C:
因此,现在让我们看一下C中的Enum声明:
enum enum-name {enumerators};
enum-name
: A valid name will be referred for all the enumeration related tasks.enum-name
:将为所有与枚举相关的任务引用一个有效的名称。enumerators
: The named integer literals.enumerators
:命名整数文字。
In order to fetch and display the data stored in enum, we need to associate an object to it using the below command:
为了获取并显示枚举中存储的数据,我们需要使用以下命令将一个对象与其关联:
enum enum-name object;
Further, to display any data value stored in the enum, we can associate the object to the specified string-literal as shown below:
此外,要显示存储在枚举中的任何数据值,我们可以将对象与指定的字符串文字相关联,如下所示:
object = enumerator;
Let us now implement the above syntax of enumeration through some examples.
现在让我们通过一些示例实现上述枚举语法。
示例C中枚举的用法 (Examples Usage of Enum in C)
In the below example, we have stored the names of the months in the enum and accessed the value of ‘Dec’ using object assigned to ‘year’.
在下面的示例中,我们将月份的名称存储在枚举中,并使用分配给“ year”的对象访问了“ Dec”的值。
#include<stdio.h>
enum year{Jan, Feb, Mar, Apr, May, Jun, July, Aug, Sep, Oct, Nov, Dec};
void main()
{
enum year obj;
obj = Dec;
printf("The value of Dec:\n%d",obj);
}
Output:
输出:
The value of Dec:
11
Now, we have created an enum of the week days and accessed every value assigned to it using a for loop.
现在,我们创建了一个工作日的枚举,并使用for循环访问分配给它的每个值。
#include<stdio.h>
enum Week_day{Mon, Tue, Wed, Thur, Fri, Sat, Sun};
int main()
{
for (int x=Mon; x<=Sun; x++)
printf("Day: %d\n", x);
return 0;
}
Output:
输出:
Day: 0
Day: 1
Day: 2
Day: 3
Day: 4
Day: 5
Day: 6
In enumeration, if we assign a particular integer value to some names or literals, all the rest of the un-assigned names would have a value which would be the value of the previous name plus 1.
在枚举中,如果我们为某些名称或文字分配特定的整数值,则所有其他未分配的名称将具有一个值,该值将是先前名称的值加1。
Have a look at the below example for the same.
看看下面的例子。
#include<stdio.h>
enum Week_day{Mon=10, Tue, Wed, Thur, Fri, Sat, Sun};
int main()
{
for (int x=Mon; x<=Sun; x++)
printf("Month: %d\n", x);
return 0;
}
We had assigned ‘Mon’ = 10, further to which all the rest of the enum literals got their values as the value of the previous name plus 1.
我们已将'Mon'= 10分配给所有枚举常量,它们的值等于前一个名称的值加1。
Output:
输出:
Month: 10
Month: 11
Month: 12
Month: 13
Month: 14
Month: 15
Month: 16
Multiple names/string literals in enumeration can have similar value or same values assigned to them.
枚举中的多个名称/字符串文字可以具有相似的值或分配给它们的相同值。
Let us have a look at the below example to validate the above statement.
让我们看下面的示例以验证上面的陈述。
#include <stdio.h>
enum switch_value {YES = 1, NO = 0, NEVER = 0};
int main()
{
printf("YES: %d\t, NO: %d\t, NEVER: %d", YES, NO, NEVER);
return 0;
}
Output:
输出:
YES: 1 , NO: 0 , NEVER: 0
结论 (Conclusion)
By this we have come to the end of this topic. Please feel free to comment below, in case you come across any doubt. Till then, Happy Learning!
至此,我们到了本主题的结尾。 如果您有任何疑问,请在下面发表评论。 到那时,祝您学习愉快!
参考资料 (References)
c++枚举和c语言枚举