c foreach循环
介绍 (Introduction)
The foreach loop in C++ or more specifically, range-based for loop was introduced with the C++11. This type of for loop structure eases the traversal over an iterable data set. It does this by eliminating the initialization process and traversing over each and every element rather than an iterator. So let us dig into the respective foreach loop structure.
C ++ 11中引入了foreach循环,或更具体地说, 基于范围的for循环 。 这种类型的for循环结构简化了对可迭代数据集的遍历。 它通过消除初始化过程并遍历每个元素而不是遍历迭代器来做到这一点。 因此,让我们深入研究各自的foreach循环结构。
C ++中的foreach循环工作 (Working of the foreach loop in C++)
So basically a for-each loop iterates over the elements of arrays, vectors, or any other data sets. It assigns the value of the current element to the variable iterator declared inside the loop. Let us take a closer look at the syntax:
因此,基本上每个循环都遍历数组 , 向量或任何其他数据集的元素。 它将当前元素的值分配给循环内声明的变量迭代器。 让我们仔细看一下语法:
for(type variable_name : array/vector_name)
{
loop statements
...
}
As we can see:
如我们所见:
- During the loop initialization, the elemental variable declaration is the part where we need to declare the variable which will iterate over the array or vector. Here, the
type
is the data type of the variable_name 在循环初始化期间, 元素变量声明是我们需要声明将在数组或向量上迭代的变量的部分。 在这里,type
是variable_name的数据类型 - array/vector name is the name of the respective data set over which the loop will iterate, 数组/向量名称是循环将在其上进行迭代的各个数据集的名称,
- loop statements are the different operations which the user can choose to perform over the corresponding elements with the use of the iterating variable. 循环语句是用户可以使用迭代变量选择对相应元素执行的不同操作。
Note: It is suggested to keep the data type of the variable the same as that of the array or vector. If the data type is not the same, then the elements are going to be type-casted and then stored into the variable.
注意:建议使变量的数据类型与数组或向量的数据类型相同。 如果数据类型不同,则元素将被类型转换,然后存储到变量中。
foreach循环的例子 (Examples of foreach loop)
1. C ++中的数组foreach循环示例 (1. Example of foreach loop for Arrays in C++)
The code given below illustrates the use of the for-each loop in C++,
下面给出的代码说明了C ++中for-each循环的用法,
#include<iostream>
using namespace std;
int main()
{
int arr[]={1,2,3,4,5}; //array initialization
cout<<"The elements are: ";
for(int i : arr)
{
cout<<i<<" ";
}
return 0;
}
Output:
输出 :
The elements are: 1 2 3 4 5
Let’s break down the code and look at it line-by-line:
让我们分解代码,逐行看一下:
- An array
arr[]
is initialized with some values {1 , 2 , 3 , 4 , 5} 数组arr[]
被初始化为一些值{1、2、3、4、5} - Inside the loop structure, ‘i’ is the variable that stores the value of the current array element 在循环结构内部, “ i”是存储当前数组元素值的变量
arr
is the array name which also serves as the base address of the respective arrayarr
是数组名称,它也用作相应数组的基地址- As we can see, printing ‘i’ for each iteration gives us the corresponding array elements in contrast to the array indices in case of normal for loop 如我们所见,在正常的for循环的情况下,每次迭代打印'i'会给我们相应的数组元素,而不是数组索引
Please note: While declaring the variable ‘i‘ we could also use the auto
datatype instead of int
. This ensures that the type of the variable is deduced from the array type, and no data type conflicts occur.
请注意 :在声明变量' i '时,我们也可以使用auto
数据类型代替int
。 这样可以确保从数组类型推导变量的类型,并且不会发生数据类型冲突。
For example:
例如:
#include<iostream>
using namespace std;
int main()
{
int array[]={1,4,7,4,8,4};
cout<<"The elements are: ";
for(auto var : array)
{
cout<<var<<" ";
}
return 0;
}
Output:
输出 :
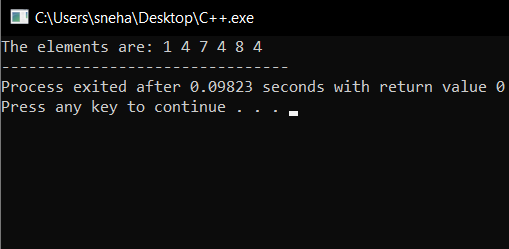
2. C ++中向量的foreach循环示例 ( 2. Example of foreach loop for Vectors in C++ )
The following code illustrates the use of the for-each loop for iterating over a vector
.
以下代码说明了for-each循环在vector
进行迭代的用法。
#include<iostream>
#include<vector>
using namespace std;
int main()
{
vector<int> vec={11,22,33,44,55,66};
cout<<"The elements are: ";
for(auto var : vec)
{
cout<<var<<" ";
}
return 0;
}
Output:
输出 :
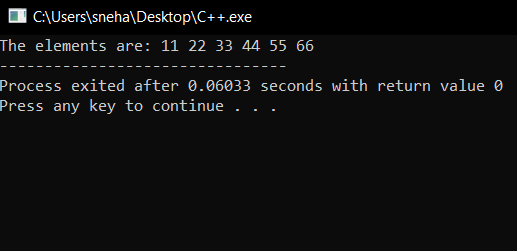
The for-each loop for vector works in the same way as it does for an array. Furthermore, the only differences are the vector declaration, initialization and the different operations that can be performed over it.
向量的for-each循环的工作方式与数组相同。 此外,唯一的区别是向量声明,初始化以及可以对其执行的不同操作。
C ++中foreach循环的优缺点 (Advantages and Disadvantages of the foreach loop in C++)
1. foreach循环的优点 (1. Advantages of foreach loop)
- It eliminates the possibility of errors and makes the code more readable. 它消除了错误的可能性,并使代码更具可读性。
- Easy to implement 易于实施
- Does not require pre-initialization of the iterator 不需要预先初始化迭代器
2. foreach循环的缺点 (2. Disadvantages of foreach loop)
- Cannot directly access the corresponding element indices 无法直接访问相应的元素索引
- Cannot traverse the elements in reverse order 无法以相反顺序遍历元素
- It doesn’t allow the user to skip any element as it traverses over each one of them 它不允许用户跳过任何遍历每个元素的元素
结论 (Conclusion)
The foreach loop in C++ has its own pros and cons. The code is easy to read but it restricts some of the actions that the normal for loop offers. Hence, it completely depends on the user what he/she wants the loop to perform and choose accordingly.
C ++中的foreach循环有其优点和缺点。 该代码易于阅读,但是它限制了普通for循环提供的某些操作。 因此,这完全取决于用户他/她希望循环执行什么并相应地选择。
参考资料 (References)
- https://stackoverflow.com/questions/16504062/how-to-make-the-for-each-loop-function-in-c-work-with-a-custom-class https://stackoverflow.com/questions/16504062/how-to-make-the-for-each-loop-function-in-c-work-with-a-custom-class
翻译自: https://www.journaldev.com/36227/foreach-loop-c-plus-plus
c foreach循环