c语言编程字符串
Strings in C programming are an array of characters with a NULL character ('\0'
) appended at the end index. Unlike character arrays, a c-string is a sequence of characters that are treated by the compiler as a single item. But just like an array, a string can be traversed along with its indices.
C编程中的字符串是一个字符数组,在末尾索引后附加一个NULL字符( '\0'
)。 与字符数组不同,c字符串是由编译器视为单个项目的一系列字符。 但是就像数组一样,字符串也可以遍历其索引。
1.用C声明字符串 (1. Declaration of Strings in C)
Declaration of a String in C is exactly the same as in the case of an Array of characters.
用C声明字符串与使用字符数组的情况完全相同。
char String_Name[No.of.elements];
char String_Name [元素数量];
Note: here the number of elements should include the NULL character ('\0'
) too.
注意:这里的元素数量也应包含NULL字符( '\0'
)。
char demo[10];
2. C语言中字符串的初始化 (2. The Initialization of Strings in C)
A string in C could be initialized in different ways. When creating a string by specifying individual characters like example 2 and 4 below, you also make sure that the NULL character is added to it.
C中的字符串可以用不同的方式初始化。 通过指定单个字符(如下面的示例2和4)来创建字符串时,还应确保将NULL字符添加到其中。
- char string[20]=”JournalDev”; char string [20] =” JournalDev”;
- char string[20]= { ‘J’ , ‘o’, ‘u’ , ‘r’ , ‘n’ , ‘a’ , ‘l’ , ‘D’ , ‘e’ , ‘v’ , ‘\0’ }; char string [20] = {'J','o','u','r','n','a','l','D','e','v','\ 0 '};
- char string[]=”JournalDev”; char string [] =” JournalDev”;
- char string[]= { ‘J’ , ‘o’, ‘u’ , ‘r’ , ‘n’ , ‘a’ , ‘l’ , ‘D’ , ‘e’ , ‘v’ , ‘\0’ }; char string [] = {'J','o','u','r','n','a','l','D','e','v','\ 0' };
For both 3rd and 4th cases the number of elements is not initially mentioned. In these situations, the size of the array is by-default set to the total number of elements(including '\0'
).
对于第3和第4种情况,最初没有提到元素的数量。 在这些情况下,默认情况下,数组的大小设置为元素总数(包括'\0'
)。
3.在C中遍历一个字符串 (3. Traversing a String in C)
For example, let us take a look at how a string can be traversed similar to an array.
例如,让我们看一下如何像数组一样遍历字符串。
char string[20]="JournalDev";
char ch;
int i=0;
ch=string[i];
while(ch!=NULL)
{
printf("%c",ch);
i++;
ch=string[i];
}
Output:
输出:
JournalDev
4.用C读取字符串(使用scanf()gets()或fgets()) (4. Reading String in C (using scanf() gets() or fgets()))
Both scanf() or gets() functions are useful for reading a string from the user. But, unfortunately, the scanf()
function only reads a sequence of characters until it encounters a newline, space, or tab, etc. Whereas, gets()
reads the sequence of characters until a newline(or enter key). Further, fgets()
is used to avoid the buffer overflow possible due to gets()
. Let’s take a look at how all the above-mentioned functions work.
scanf()或gets()函数都可用于从用户读取字符串。 但是,不幸的是, scanf()
函数仅读取字符序列,直到遇到换行符,空格或制表符等为止。而gets()
读取字符序列直到换行符(或Enter键)。 此外, fgets()
用于避免由于gets()
可能导致的缓冲区溢出 。 让我们看一下上述所有功能是如何工作的。
For example,
例如,
#include<stdio.h>
int main()
{
char string_input[20];
printf("Enter the string: ");
scanf("%s", string_input);
puts(string_input);
}
Output:
输出:
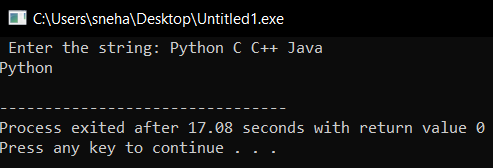
As you can see, due to the presence of whitespaces in between the string, scanf()
takes in only the characters before the first space. This can be avoided by the gets()
function.
如您所见,由于字符串之间存在空格 ,所以scanf()
仅接受第一个空格之前的字符。 可以通过gets()
函数避免这种情况。
#include<stdio.h>
int main()
{
char string_input[20];
printf("Enter the string: ");
gets(string_input);
puts(string_input);
}
Output:
输出:
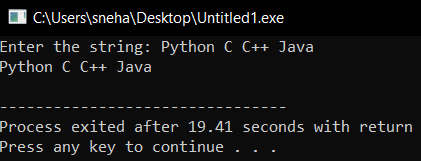
Again using fgets()
function,
再次使用fgets()
函数,
#include<stdio.h>
int main()
{
char string_input[20];
printf("Enter the string: ");
fgets(string_input,sizeof(string_input),stdin);
puts(string_input);
}
Output:
输出:

5. C字符串库函数 (5. C String Library functions)
The standard C library incorporates many in-built string oriented functions. All these functions are available inside the string.h
header file. So, including the string.h
header file is going to be useful for easier string manipulation.
标准的C库包含许多内置的面向字符串的函数。 所有这些功能在string.h
头文件中均可用。 因此,包含string.h
头文件对于更容易的字符串操作将很有用。
Some common functions are,
一些常见的功能是
strlen(string)
– This function returns the length of the passed string or the total number of elements in the string,strlen(string)
–此函数返回传递的字符串的长度或字符串中元素的总数,strcmp(string1,string2)
– This function compares two string. It returns 0 if the two strings are equal, and else it returns a non-zero value,strcmp(string1,string2)
–此函数比较两个字符串。 如果两个字符串相等,则返回0,否则返回非零值,strcat(string1,string2)
– This function concatenates two given strings. It Appends string2 to the end of string1 and stores the result to string1,strcat(string1,string2)
–此函数连接两个给定的字符串。 它将string2追加到string1的末尾,并将结果存储到string1,strcpy(string1,string2)
– This function copies the contents of the string2 into the string1,strcpy(string1,string2)
–此函数将string2的内容复制到string1中,strrev(string)
– This function reverses the string inside,strrev(string)
–此函数反转内部的字符串,- And many more. 还有很多。
For further reading – Check all the previous tutorials on C Programming on Journaldev.
要进一步阅读,请参阅Journaldev上有关C编程的所有先前教程。
6. 参考: (6. References:)
- https://en.wikibooks.org/wiki/C_Programming/String_manipulation https://zh.wikibooks.org/wiki/C_Programming/String_manipulation
- How to create an array of strings in C(Stack Overflow) 如何在C中创建字符串数组(堆栈溢出)
翻译自: https://www.journaldev.com/35071/strings-in-c-programming
c语言编程字符串