c++语句switch语句
The Switch Statement in C++ acts as a control statement and select a particular matching condition from a set of conditions.
C ++中的Switch语句用作控制语句,并从一组条件中选择特定的匹配条件。
These statements are an easy substitute to the monotonous if-else statements.
这些语句很容易替代单调的if-else语句。
It selects one block with the matching case-label out of all the blocks in the code.
它从代码中的所有块中选择一个带有匹配大小写标签的块。
Switch statements are helpful when we have a set of multiple conditions and the user needs to select one of them based on the matching condition.
当我们有一组多个条件并且用户需要根据匹配条件选择其中一个条件时,switch语句会很有用。
Syntax:
句法:
switch(expression/condition) {
case constant_value1:
// statements
break;
case constant_value2:
// statements
break;
.
.
case constant_valueN:
//statements
break;
default:
// statements
}
C ++中的switch语句的工作 (Working of Switch Statement in C++)
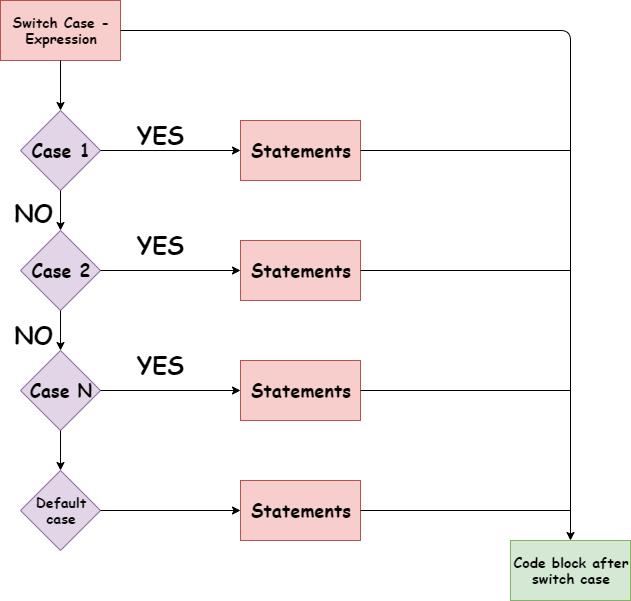
The switch statement works upon the expression input by the user to check for a match.
switch语句根据用户输入的表达式来检查匹配项。
As soon as an expression is encountered by the compiler, it searches for a matching value of the expression with the case labels.
编译器一旦遇到表达式, 便会搜索带有大小写标签的表达式的匹配值。
If a match is found, the piece of code within that particular block associated with the matched case label is executed.
如果找到匹配项,则执行与匹配的案例标签关联的特定块内的代码 。
Thus, the switch statement is taken into consideration only once upon the match to be found.
因此,仅在找到匹配项后才考虑switch语句。
“ break”关键字的意义 (Significance of “break” keyword )
As soon as a match is found with the case label, the break statement gets executed.
一旦找到与case标签匹配的内容,就会执行break语句。
The break statements terminate the execution of the code in the block, following the statement and get the execution point out of the switch statement.
break语句在语句之后终止块中代码的执行,并从switch语句中获取执行点 。
Thus, it neglects and terminates the execution of the rest of the code and comes out of the switch statement.
因此,它忽略并终止了其余代码的执行,并退出了switch语句。
“默认”关键字的意义 (Significance of “default” keyword)
The default keyword is responsible for executing the piece of code if no matching case labels are found.
如果找不到匹配的大小写标签,则 default关键字负责执行这段代码。
在switch语句中为案例标签选择数据类型 (Choosing data type for the case labels in switch statements)
- The data type of case labels of the switch statement in C++ can be either an integer or character type. C ++中switch语句的case标签的数据类型可以是整数或字符类型。
- The case label can be either a constant variable or a constant expression. case标签可以是一个常量变量或一个常量表达式。
要记住的要点 ( Points to Remember )
- The case label can be of integer, short, long or character data type. 大小写标签可以是整数,短,长或字符数据类型。
- Switch statements do not support float or double type case labels. switch语句不支持浮点或双精度型大小写标签。
- The data type of the expression and the case label needs to be the same. 表达式的数据类型和大小写标签必须相同。
- The case label cannot be an expression or a variable. It can be an integer value such as 1, 2, 3, so on or a character such as ‘A’, ‘w’, so on. 案例标签不能是表达式或变量。 它可以是整数值,例如1、2、3等,也可以是字符,例如'A','w'等。
- Duplicate case label values turn out as error and are not allowed. 重复的案例标签值显示为错误,因此是不允许的。
- The default statement is completely optional and doesn’t affect the flow of execution of switch cases. 默认语句是完全可选的,不会影响切换案例的执行流程。
- Any statement that is written above the switch case labels never gets executed. 开关格标签上方写的任何语句都不会执行。
- The position of the default statement does not affect the execution and can be placed anywhere within the code. 默认语句的位置不影响执行,可以放置在代码内的任何位置。
了解C ++中的开关情况 (Understanding switch case in c++)
Example:
例:
# include <iostream>
using namespace std;
int main()
{
char choice;
float input1, input2;
cout << "Enter the input values:\n";
cin >> input1;
cin >> input2;
cout << "Choose the operation to be performed from (+, -, *, /) : ";
cin >> choice;
switch(choice) #expression
{
case '+': # case-label
cout << "Performing Addition operation on the input values..\n";
cout << input1+input2;
break;
case '-':
cout << "Performing Subtraction operation on the input values..\n";
cout << input1-input2;
break;
case '*':
cout << "Performing Multiplication operation on the input values..\n";
cout << input1*input2;
break;
case '/':
cout << "Performing Division operation on the input values..\n";
cout << input1/input2;
break;
default:
cout << "Choose the correct operation to be performed.";
}
return 0;
}
Output:
输出:
Enter the input values:
10
10
Choose the operation to be performed from (+, -, *, /) : /
Performing Division operation on the input values..
1
In the above code snippet, the input operation is division (/). So, the expression (input) checks for the matching case label.
在以上代码段中,输入操作为除(/)。 因此,表达式(输入)检查匹配的案例标签。
As soon as a match is encountered, the flow of control execution shifts to the particular block and the division operation is performed.
一旦遇到匹配,控制执行流程就会转移到特定的块并执行除法运算。
结论 (Conclusion)
Thus, in this article, we have understood the working of Switch Statements in the C++ language.
因此,在本文中,我们了解了C ++语言中的Switch语句的工作。
参考 (Reference)
翻译自: https://www.journaldev.com/34910/switch-statement-in-c-plus-plus
c++语句switch语句