A Matrix is a rectangular array. The elements are arranged in the rows and columns. In this tutorial, we will look at some matrix programs in Java.
矩阵是矩形数组。 元素按行和列排列。 在本教程中,我们将介绍一些Java矩阵程序。
矩阵的图形表示 (Graphical Representation of Matrix)
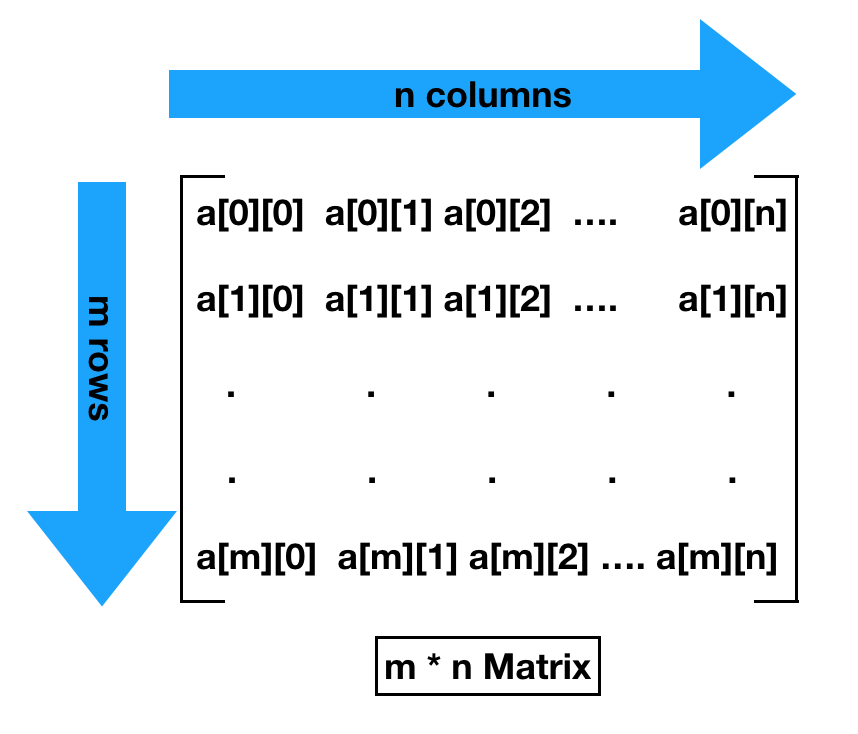
Matrix
矩阵
Java矩阵 (Matrix in Java)
We can implement a matrix using two dimensional array in Java. The element at row “r” and column “c” can be accessed using index “array[r]“.
我们可以使用Java中的二维数组来实现矩阵。 可以使用索引“ array [r]”访问“ r”行和“ c”列的元素。
Java矩阵程序 (Matrix Programs in Java)
Since we are using two-dimensional arrays to create a matrix, we can easily perform various operations on its elements. In this tutorial, we will learn how to create a matrix from user input. Then we will add, subtract, and multiply two matrices and print the result matrix on the console.
由于我们使用二维数组来创建矩阵,因此我们可以轻松地对其元素执行各种操作。 在本教程中,我们将学习如何根据用户输入创建矩阵。 然后,我们将对两个矩阵进行加,减和乘运算,并将结果矩阵打印在控制台上。
1.加两个矩阵 (1. Adding Two Matrix)
Here is the simple program to populate two matrices from the user input. Then add its elements at the corresponding indices to get the addition of the matrices. Finally, we will print the sum of the matrices.
这是从用户输入中填充两个矩阵的简单程序。 然后将其元素添加到相应的索引处以获得矩阵的相加。 最后,我们将打印矩阵的总和。
package com.journaldev.examples;
import java.util.Scanner;
public class MatrixPrograms {
public static void main(String[] args) {
System.out.println("Please enter the rows in the matrix");
Scanner sc = new Scanner(System.in);
int row = sc.nextInt();
System.out.println("Please enter the columns in the matrix");
int column = sc.nextInt();
int[][] first = new int[row][column];
int[][] second = new int[row][column];
for (int r = 0; r < row; r++) {
for (int c = 0; c < column; c++) {
System.out.println(String.format("Enter first[%d][%d] integer", r, c));
first[r] = sc.nextInt();
}
}
for (int r = 0; r < row; r++) {
for (int c = 0; c < column; c++) {
System.out.println(String.format("Enter second[%d][%d] integer", r, c));
second[r] = sc.nextInt();
}
}
// close the scanner
sc.close();
// print both matrices
System.out.println("First Matrix:\n");
print2dArray(first);
System.out.println("Second Matrix:\n");
print2dArray(second);
// sum of matrices
sum(first, second);
}
// below code doesn't take care of exceptions
private static void sum(int[][] first, int[][] second) {
int row = first.length;
int column = first[0].length;
int[][] sum = new int[row][column];
for (int r = 0; r < row; r++) {
for (int c = 0; c < column; c++) {
sum[r] = first[r] + second[r];
}
}
System.out.println("\nSum of Matrices:\n");
print2dArray(sum);
}
private static void print2dArray(int[][] matrix) {
for (int r = 0; r < matrix.length; r++) {
for (int c = 0; c < matrix[0].length; c++) {
System.out.print(matrix[r] + "\t");
}
System.out.println();
}
}
}

Adding Two Matrices
加两个矩阵
2.减去两个矩阵 (2. Subtracting Two Matrices)
Here is the function to subtraction second matrix elements from the first matrix and then print the result matrix.
这是从第一个矩阵中减去第二个矩阵元素,然后打印结果矩阵的函数。
private static void subtract(int[][] first, int[][] second) {
int row = first.length;
int column = first[0].length;
int[][] sum = new int[row][column];
for (int r = 0; r < row; r++) {
for (int c = 0; c < column; c++) {
sum[r] = first[r] - second[r];
}
}
System.out.println("\nSubtraction of Matrices:\n");
print2dArray(sum);
}
3.将两个矩阵相乘 (3. Multiplying Two Matrices)
Below method will multiply the matrix elements and print the result matrix.
下面的方法将矩阵元素相乘并打印结果矩阵。
private static void multiply(int[][] first, int[][] second) {
int row = first.length;
int column = first[0].length;
int[][] sum = new int[row][column];
for (int r = 0; r < row; r++) {
for (int c = 0; c < column; c++) {
sum[r] = first[r] * second[r];
}
}
System.out.println("\nMultiplication of Matrices:\n");
print2dArray(sum);
}
Reference: Wikipedia
参考: 维基百科
翻译自: https://www.journaldev.com/32012/matrix-programs-in-java