java 方法 示例
Java Method Reference was introduced in Java 8, along with lambda expressions. The method reference is a shortcut way to create lambda expressions when it just calls a method.
Java方法参考是与lambda表达式一起在Java 8中引入的。 方法引用是在调用方法时创建lambda表达式的快捷方式。
什么是Java方法参考? (What is Java Method Reference?)
The method reference allows us to create a lambda expression from an existing method. It’s used when the lambda expression is calling a function and doing nothing else. The JVM takes care of creating the lambda expression by mapping the input variables to the method arguments.
方法参考允许我们从现有方法创建lambda表达式。 当lambda表达式正在调用函数而不执行其他任何操作时,将使用它。 JVM通过将输入变量映射到方法参数来创建lambda表达式。
方法参考语法 (Method Reference Syntax)
The method reference has two parts – class/object and method/constructor. They are separated by double colons (::). There are no additional parameters passed with the method reference.
方法参考包括两部分-类/对象和方法/构造函数。 它们之间用双冒号(::)分隔。 方法引用没有传递其他参数。
方法引用的类型 (Types of Method References)
There are four types of method references in Java.
Java中有四种类型的方法引用。
- Static Method Reference: its syntax is Class::StaticMethodName 静态方法参考:其语法为Class :: StaticMethodName
- Reference to an Object instance method: the syntax is Object::instanceMethodName 引用对象实例方法:语法为Object :: instanceMethodName
- Reference to an instance method of an arbitrary object of specific type: the syntax is Class::instanceMethodName 引用特定类型的任意对象的实例方法:语法为Class :: instanceMethodName
- Constructor Reference: its syntax is ClassName::new 构造函数参考:其语法为ClassName :: new
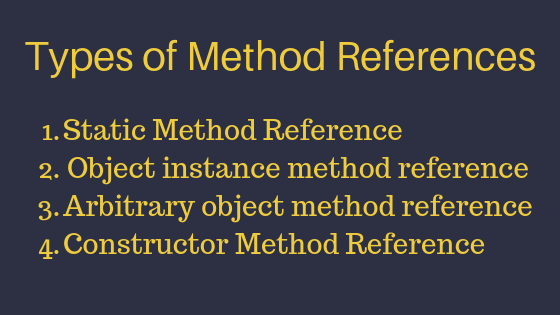
Java Method Reference Types
Java方法参考类型
Java方法参考示例 (Java Method Reference Examples)
Let’s look at the examples of all the four types of method references. We will first create a lambda expression and then use the method reference to have the same effect.
让我们看一下所有四种类型的方法引用的示例。 我们将首先创建一个lambda表达式 ,然后使用方法引用产生相同的效果。
1.静态方法参考 (1. Static Method Reference)
Let’s say we have a functional interface as below.
假设我们有一个如下所示的功能接口 。
@FunctionalInterface
interface Counter {
int count(Object[] objArray);
}
We have a Utils class to get the number of elements in an array.
我们有一个Utils类来获取数组中元素的数量。
class Utils {
public static int countElements(Object[] array) {
return array.length;
}
}
We will use a lambda expression to call the Utils class countElements() method to get the number of elements in an array.
我们将使用lambda表达式调用Utils类的countElements()方法来获取数组中的元素数。
Integer[] intArray = { 1, 2, 3, 4, 5 };
// lambda expression
Counter counter2 = array -> Utils.countElements(array);
System.out.println(counter2.count(intArray)); // 5
Here, the lambda expression is simply calling the Utils class static method and not doing anything else. This is a perfect place to use a method reference to call the static method.
在这里,lambda表达式只是调用Utils类的静态方法,而不做其他任何事情。 这是使用方法引用调用静态方法的理想场所。
Counter counter = Utils::countElements;
2.对象实例方法参考 (2. Object Instance Method Reference)
Let’s change the Utils class implementation and create an instance method.
让我们更改Utils类的实现并创建一个实例方法。
class Utils {
public int count(Object[] array) {
return array.length;
}
}
Now, we will create a lambda expression calling this instance method.
现在,我们将创建一个lambda表达式来调用此实例方法。
Utils ut = new Utils();
Counter counter1 = array -> ut.count(array);
We can replace the lambda expression with instance method reference.
我们可以将lambda表达式替换为实例方法参考。
Counter counter1 = ut::count;
We can instantiate the Utils class in the method reference itself.
我们可以在方法引用本身中实例化Utils类。
Counter counter1 = new Utils()::count;
3.引用任意对象的实例方法 (3. Reference to the instance method of an arbitrary object)
Sometimes, we call a method of an argument in the lambda expression. In that case, we can use a method reference to call an instance method of an arbitrary object of a specific type.
有时,我们在lambda表达式中调用参数的方法。 在这种情况下,我们可以使用方法引用来调用特定类型的任意对象的实例方法。
Let’s say we have a lambda expression like this.
假设我们有一个这样的lambda表达式。
String[] strArray = { "A", "E", "I", "O", "U", "a", "e", "i", "o", "u" };
Arrays.sort(strArray, (s1, s2) -> s1.compareToIgnoreCase(s2));
Here, the lambda expression is simply calling a method. So, we can use method reference here. But, the method is called on an arbitrary object of String. So, we can call the instance method by referring to the String class.
在这里,lambda表达式只是调用一个方法。 因此,我们可以在此处使用方法参考。 但是,该方法在String的任意对象上调用。 因此,我们可以通过引用String类来调用实例方法。
Arrays.sort(strArray, String::compareToIgnoreCase);
4.构造方法参考 (4. Constructor Method Reference)
The Stream collect() method accepts a Supplier argument. The supplier should return a new instance every time. So, the lambda expression must call the constructor. Let’s look at a simple example.
Stream collect()方法接受Supplier参数。 供应商应每次返回一个新实例。 因此,lambda表达式必须调用构造函数。 让我们看一个简单的例子。
List<Integer> intList = List.of(1, 2, 3, 4, 5);
String concat1 = intList.parallelStream().collect(
() -> new StringBuilder(),
(x, y) -> x.append(y),
(a, b) -> a.append(b)).toString();
Here, we can replace “() -> new StringBuilder()” to a constructor method reference.
在这里,我们可以将“()-> new StringBuilder()”替换为构造函数方法参考。
String concat1 = intList.parallelStream().collect(
StringBuilder::new,
(x, y) -> x.append(y),
(a, b) -> a.append(b)).toString();
Well, we don’t have to stop here. The other two lambda expressions can also be replaced by a method reference.
好吧,我们不必在这里停下来。 其他两个lambda表达式也可以用方法引用代替。
String concat1 = intList.parallelStream().collect(
StringBuilder::new,
StringBuilder::append,
StringBuilder::append).toString();
参考 (Reference)
java 方法 示例