java实现链表
A linked list is a linear data structure that contains a sequence of elements such that each element has reference to the next element in the sequence.
链表是一个线性数据结构,包含一个元素序列,这样每个元素都可以引用序列中的下一个元素。
Each element in the linked list is called “Node”. Each Node contains a data element and a Node next which points to the next node in the linked list.
链接列表中的每个元素称为“节点”。 每个节点都包含一个数据元素和一个下一个Node,它指向链表中的下一个节点。
In a linked list we can create as many nodes as we want according to our requirement.
在链表中,我们可以根据需要创建任意数量的节点。
为什么需要链接列表? (Why do we need a Linked List?)
Although you must have seen Arrays data structure earlier, they too are linear data structure but they pose certain limitations such as:
尽管您可能早先已经看过数组数据结构,但是它们也是线性数据结构,但是它们具有某些局限性,例如:
- They are static in nature and hence their size cannot be changed. 它们本质上是静态的,因此它们的大小无法更改。
- They need contiguous memory to store their values. 他们需要连续的内存来存储其值。
To tackle these limitations Linked list offers the following features:
解决这些限制链接列表提供以下功能:
- DYNAMIC MEMORY ALLOCATION i.e. the memory allocation is done at the run time by the compiler. 动态内存分配,即编译器在运行时完成内存分配。
- Each individual Node can have any block of memory and it is linked to other nodes by the address of the block, thus no contiguous memory blocks are required. 每个单独的节点可以具有任何内存块,并且通过该块的地址链接到其他节点,因此不需要连续的内存块。
链表的一般实现 (General Implementation of Linked List)
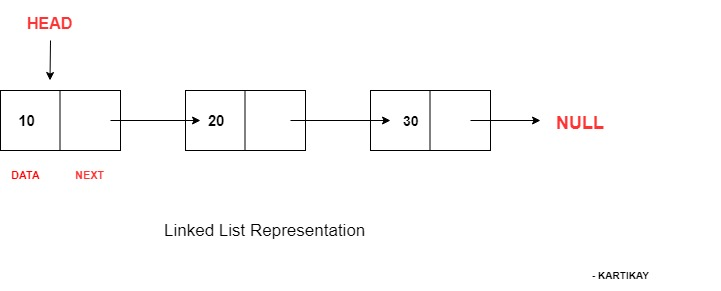
Linked List
链表
- The Linked list class contains a private class Node and an Object of Node class (called as Head) as one of its Properties. 链接列表类包含一个私有类Node和一个Node对象类(称为Head)作为其属性之一。
- The Node head points to the starting of the Linked List. Node头指向链接列表的开始。
- Every Node contains a data element and a Node next which points to the next node in the Linked List. 每个节点都包含一个数据元素和一个下一个节点,该节点指向链接列表中的下一个节点。
- The last nodes point to null. 最后的节点指向空。
- In newer variations, we also have a tail Node element which helps us to reduce the time complexity of operations to be performed easily. 在较新的变体中,我们还有一个尾节点元素,它可以帮助我们减少易于执行的操作的时间复杂性。
链表操作 (Linked List Operations)
A Linked List implementation must have following common purpose methods.
链表实现必须具有以下通用方法。
- getFirst: It returns the data (if any, else it throws an exception) present at the first Node. It simply returns the “this.head.data” provided “if(this. Size!=0)” i.e. if the size of the Linked list is not zero. getFirst :它返回第一个Node上存在的数据(如果有的话,否则抛出异常)。 它只是返回“ if( this。Size != 0) ”所提供的“ this.head.data ”,即,如果链接列表的大小不为零。
- getLast: It returns the data (if any, else it throws an exception) present at the last Node. It simply returns the “this.tail.data” provided “if(this. Size!=0)” i.e. if the size of the Linked list is not zero. getLast :它返回最后一个节点上存在的数据(如果有的话,否则抛出异常)。 它只是返回“ if( this。Size != 0) ”所提供的“ this.tail.data ”,即,如果链接列表的大小不为零。
- getAt: It takes an Integer index as an argument and if the index is valid(i.e. if index>=0 and index <size of the Linked list) it returns the data of the element present at that index. getAt :它使用一个整数索引作为参数,并且如果索引有效(即,如果index> = 0且index <链接列表的大小),则它返回该索引处存在的元素的数据 。
- getNodeAt: It takes an Integer index as an argument and if the index is valid(i.e. if index>=0 and index <size of the Linked list) it returns the Node at that index. getNodeAt :它将一个整数索引作为参数,并且如果索引有效(即,如果index> = 0且index <链接列表的大小),则返回该索引处的Node 。
- addLast: It takes an input of type of which Linked list is, and then appends it at the last position of the Linked list, thereby increasing the size by ‘+1’ and pointing the “tail” at the newly added Node. addLast :接受链接列表类型的输入,然后将其追加到链接列表的最后一个位置,从而将大小增加“ +1”,并将“尾部”指向新添加的Node。
- addFirst: It takes an input of type of which Linked list is, and then appends it at the First position of the Linked list, thereby increasing the size by ‘+1’ and pointing the “head” at the newly added Node. addFirst :它接受链接列表类型的输入,然后将其追加到链接列表的第一个位置,从而将大小增加“ +1”,并将“ head”指向新添加的Node。
- addAt: It takes an input of type of which Linked list is and it also takes an Integer index (let it be equal to ‘k’) as arguments, and then appends it (data) at the kth position of the Linked list, thereby increasing the size by ‘+1’. In case the element to be added is “First” or “Last” then it calls “addFirst” or “addLast” respectively. addAt :它接受链接列表类型的输入,还接受一个整数索引(使其等于“ k”)作为参数,然后将其(数据)附加到链接列表的第k个位置,从而将大小增加“ +1”。 如果要添加的元素是“ First ”或“ Last ”,则分别调用“ addFirst ”或“ addLast ”。
- removeFirst: It removes the first element of a Linked list (Provided the list is not an empty list, in which it throws an exception) and moves the “head” to point towards the 2nd element (if any ) and decreases the size by ‘1’. Also, it returns the removed data. removeFirst :删除链接列表的第一个元素(假设列表不是空列表,在其中抛出异常),并将“ head”指向第二个元素(如果有的话),并减小大小'1'。 同样,它返回删除的数据。
- removeLast: It removes the last element of the Linked list (Provided the list is not an empty list, in which it throws an exception) and moves the “tail” to point towards the 2nd last element (if any ) and decreases the size by ‘1’. Also, it returns the removed data. removeLast :删除链接列表的最后一个元素(假设列表不是一个空列表,在其中抛出异常),然后将“ tail”移动到最后一个第二个元素(如果有的话),并减小其大小按“ 1”。 同样,它返回删除的数据。
- removeAt: It takes an Integer index (let’s say k) as an argument and if the index is valid(i.e. if index>=0 and index <size of Linked list) removes the ‘kth’ element of Linked list (Provided the list is not an empty list, in which it throws an exception) and decreases the size by ‘1’. Also, it returns the removed data. In case the element to be removed is “First” or “Last” then it calls “removeFirst” or “removeLast” respectively. removeAt :它使用一个整数索引(比如说k)作为参数,并且如果索引有效(即,如果index> = 0且index <链接列表的大小),则删除链接列表的“ kth”元素(假设列表为(不是一个空列表,它会引发异常)并将大小减小1。 同样,它返回删除的数据。 如果要删除的元素是“ First ”或“ Last ”,则分别调用“ removeFirst ”或“ removeLast ”。
- display: This method prints the whole Linked list by traversing it once starting from a pointer which points to “head” until it points to “null”. display :此方法通过从指向“ head”的指针开始直到指向“ null”的指针遍历,来打印整个Linked列表。
Java中的链表实现 (Linked List Implementation in Java)
package com.journaldev.ds;
public class LinkedList {
private class Node {
int data;
Node next;
}
private Node head;
private Node tail;
private int size;
public int size() {
return this.size;
}
public int getFirst() throws Exception {
if (this.size == 0) {
throw new Exception("LL is Empty.");
}
return this.head.data;
}
public int getLast() throws Exception {
if (this.size == 0) {
throw new Exception("LL is Empty.");
}
return this.tail.data;
}
public int getAt(int idx) throws Exception {
if (this.size == 0) {
throw new Exception("LL is Empty.");
}
if (idx < 0 || idx >= this.size) {
throw new Exception("Invalid Index.");
}
Node temp = this.head;
for (int i = 1; i <= idx; i++) {
temp = temp.next;
}
return temp.data;
}
private Node getNodeAt(int idx) throws Exception {
if (this.size == 0) {
throw new Exception("LL is Empty.");
}
if (idx < 0 || idx >= this.size) {
throw new Exception("Invalid Index.");
}
Node temp = this.head;
for (int i = 1; i <= idx; i++) {
temp = temp.next;
}
return temp;
}
public void addLast(int item) {
// create
Node nn = new Node();
nn.data = item;
nn.next = null;
// attach
if (this.size > 0)
this.tail.next = nn;
// dm update
if (this.size == 0) {
this.head = nn;
this.tail = nn;
this.size += 1;
} else {
this.tail = nn;
this.size += 1;
}
}
public void addFirst(int item) {
// create
Node nn = new Node();
nn.data = item;
nn.next = null;
// attach
nn.next = this.head;
// dm update
if (this.size == 0) {
this.head = nn;
this.tail = nn;
this.size++;
} else {
this.head = nn;
this.size++;
}
}
public void addAt(int item, int idx) throws Exception {
if (idx < 0 || idx > this.size) {
throw new Exception("Invalid Index.");
}
if (idx == 0) {
addFirst(item);
} else if (idx == this.size) {
addLast(item);
} else {
// create
Node nn = new Node();
nn.data = item;
nn.next = null;
// attach
Node nm1 = getNodeAt(idx - 1);
Node np1 = nm1.next;
nm1.next = nn;
nn.next = np1;
// dm
this.size++;
}
}
public int removeFirst() throws Exception {
if (this.size == 0) {
throw new Exception("LL is empty.");
}
Node temp = this.head;
if (this.size == 1) {
this.head = null;
this.tail = null;
this.size = 0;
} else {
this.head = this.head.next;
this.size--;
}
return temp.data;
}
public int removeLast() throws Exception {
if (this.size == 0) {
throw new Exception("LL is empty.");
}
Node temp = this.tail;
if (this.size == 1) {
this.head = null;
this.tail = null;
this.size = 0;
} else {
Node sm2 = getNodeAt(this.size - 2);
sm2.next = null;
this.tail = sm2;
this.size--;
}
return temp.data;
}
public int removeAt(int idx) throws Exception {
if (this.size == 0) {
throw new Exception("LL is empty.");
}
if (idx < 0 || idx >= this.size) {
throw new Exception("Invalid Index.");
}
if (idx == 0) {
return removeFirst();
} else if (idx == this.size - 1) {
return removeLast();
} else {
Node nm1 = getNodeAt(idx - 1);
Node n = nm1.next;
Node np1 = n.next;
nm1.next = np1;
this.size--;
return n.data;
}
}
public void display() {
System.out.println("----------------------");
Node temp = this.head;
while (temp != null) {
System.out.print(temp.data + " ");
temp = temp.next;
}
System.out.println(".");
System.out.println("----------------------");
}
public static void main(String[] args) throws Exception {
LinkedList list = new LinkedList();
list.addLast(10);
list.addLast(20);
list.addLast(30);
list.addLast(40);
// this will display the list
list.display();
// first element i.e.10 should be printed
System.out.println(list.getFirst());
// last element i.e.40 should be printed
System.out.println(list.getLast());
// element at 3rd index i.e.40 should be printed
System.out.println(list.getAt(3));
// a memory address of a node should be printed
System.out.println(list.getNodeAt(3));
// 10 should be removed and printed
System.out.println(list.removeFirst());
// 40 should be removed and printed
System.out.println(list.removeLast());
// list without 10 and 40 should be printed
list.display();
// 100 should be added at first
list.addFirst(100);
list.display();
// 30 should be removed
list.removeAt(2);
// 300 should be added at 2nd index
list.addAt(300, 2);
list.display();
}
}
Output
输出量

LinkedLIst Implementation
链接式实施
NOTE: Java provides implementation of LinkedList. For actual programming, use the official implementation.
注意:Java提供了LinkedList的实现。 对于实际编程,请使用官方实现。
链表操作时间复杂度 (Linked List Operations Time Complexity)
Operations | Time Complexity |
---|---|
getFirst | O(1) |
getLast | O(1) {Note: It is because we have ‘tail’ pointer too , else it would have been O(n) } |
getAt | O(n) |
getNodeAt | O(n) |
addLast | O(1) {Note: It is because we have ‘tail’ pointer too , else it would have been O(n) } |
addFirst | O(1) |
addAt | O(n) |
removeFirst | O(1) |
removeLast | O(1) {Note: It is because we have ‘tail’ pointer too , else it would have been O(n) } |
removeAt | O(n) |
Display | O(n) |
运作方式 | 时间复杂度 |
---|---|
getFirst | O(1) |
getLast | O(1){注意:这也是因为我们也有“ tail”指针,否则将是O(n)} |
getAt | 上) |
getNodeAt | 上) |
addLast | O(1){注意:这也是因为我们也有“ tail”指针,否则将是O(n)} |
addFirst | O(1) |
addAt | 上) |
removeFirst | O(1) |
removeLast | O(1){注意:这也是因为我们也有“ tail”指针,否则将是O(n)} |
removeAt | 上) |
显示 | 上) |
结论 (Conclusion)
LinkedList is one of the most popular data structure. It’s used a lot in storing data sequentially. We have provided the basic implementation of Linked List. But it’s not optimized for production usage. Please use the language-specific implementation of the Linked List in actual programming.
LinkedList是最流行的数据结构之一。 在顺序存储数据中使用了很多方法。 我们提供了链接列表的基本实现。 但是它并未针对生产用途进行优化。 请在实际编程中使用特定于语言的链接表实现。
翻译自: https://www.journaldev.com/30176/introduction-to-linked-list-java-implementation
java实现链表