decorator
Python decorator is a function that helps to add some additional functionalities to an already defined function. Python decorator is very helpful to add functionality to a function that is implemented before without making any change to the original function. Decorator is very efficient when want to give an updated code to an existing code.
Python装饰器是一个函数,可以帮助向已定义的函数添加一些其他功能。 Python装饰器在不对原始功能进行任何更改的情况下将功能添加到之前实现的功能中非常有用。 想要将更新的代码提供给现有代码时,Decorator非常有效。
Python装饰器 (Python Decorator)
Hope all of you are fine and spending a very good time with python. Today we will learn about python decorator. As I defined earlier, python decorator is nothing but a decorator that decorates a function. For example, you have a flower vase in your home. Now you want to decorate it with some flower and some ornaments. Then you will be called as decorator and the flower and ornaments will be called additional functionalities.
希望大家都很好,并在python上度过了愉快的时光。 今天,我们将了解python装饰器。 正如我之前定义的,python装饰器不过是装饰函数的装饰器。 例如,您家中有一个花瓶。 现在,您想用一些花和一些装饰品来装饰它。 然后,您将被称为装饰器,花和装饰品将被称为附加功能。
Python Decorator基本结构 (Python Decorator Basic Structure)
Let’s have a look at the basic structure of a python decorator function.
让我们看一下python装饰器函数的基本结构。
# example of decorator
def sampleDecorator(func):
def addingFunction():
# some new statments or flow control
print("This is the added text to the actual function.")
# calling the function
func()
return addingFunction
@sampleDecorator
def actualFunction():
print("This is the actual function.")
actualFunction()
Above code will produce following output:
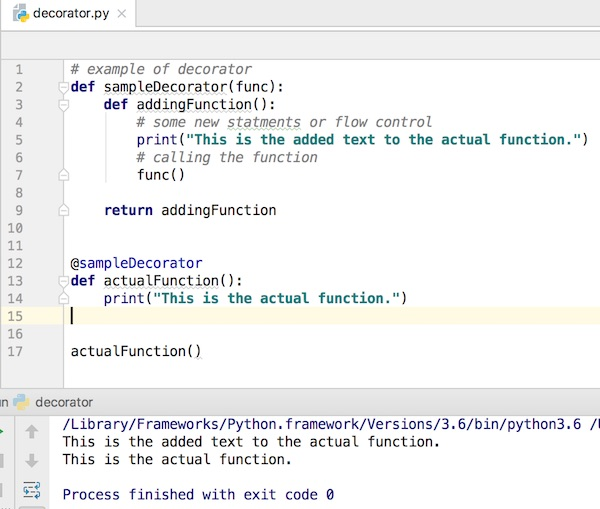
上面的代码将产生以下输出:
This is the added text to the actual function.
This is the actual function.
In the above code, in line 12 there is a special character @, which mainly indicates that the function which is defined just below it, will be decorated using the sampleDecorator
function.
在上面的代码中,在第12行中有一个特殊字符@,主要表示在其正下方定义的函数将使用sampleDecorator
函数进行修饰。
The above code can be interpreted as the following code assume that we don’t have line 12. Then if we want to get the same flavour then we have to override line 17 as following:
上面的代码可以解释为以下代码假定我们没有第12行。然后,如果要获得相同的样式,则必须重写第17行,如下所示:
actualFunction = sampleDecorator(actualFunction)
actualFunction()
Then you will get the same output as before. Observing the above two line what can you assume? We are mainly overwriting the function actualFunction
using the sampleDecorator
function where we are passing the actualFunction
as argument of the decorator function.
然后,您将获得与以前相同的输出。 观察以上两行,您可以假设什么? 我们主要是使用sampleDecorator
函数覆盖函数actualFunction
,在sampleDecorator
函数中,我们将actualFunction
作为装饰器函数的参数传递。
带有单个参数的Python Decorator示例 (Python Decorator Example with single argument)
Consider the following code where the function we want to decorate, do have an argument. Notice how we are implementing the decorator python method. Remember the example of flower vase. We will give, the number of flowers that we want to put in.
考虑以下代码,我们要装饰的函数确实有一个参数 。 注意我们如何实现装饰器python方法。 记住花瓶的例子。 我们将提供想要放入的花朵数量。
def flowerDecorator(vasel):
def newFlowerVase(n):
print("We are decorating the flower vase.")
print("You wanted to keep %d flowers in the vase." % n)
vasel(n)
print("Our decoration is done")
return newFlowerVase
@flowerDecorator
def flowerVase(n):
print("We have a flower vase.")
flowerVase(6)
Can you assume the output?? The output of the above code will be:
你能假设输出吗? 上面代码的输出将是:
We are decorating the flower vase.
You wanted to keep 6 flowers in the vase.
We have a flower vase.
Our decoration is done
了解python装饰器参数代码 (Understanding the python decorator argument code)
Now let’s simulate the above code. First in line 18, we call the function flowerVase(6)
. Then flow goes to line 13 and sees that this function is being decorated by another function named flowerDecorator
. So it goes to line 1, where the flowerVase
is sent as an argument.
现在让我们模拟上面的代码。 首先在第18行,我们调用函数flowerVase(6)
。 然后流程转到第13行,并看到此函数正在由另一个名为flowerDecorator
函数flowerDecorator
。 因此,它转到第1行,其中flowerVase
作为参数发送。
Notice that flowerVase takes a parameter. In the flowerDecorator
function, we are defining a new function named newFlowerVase
that will wrap the actual function and notice this function will have the exactly same number or parameter that the actual function ( flowerVase ) takes.
请注意,flowerVase具有一个参数。 在flowerDecorator
函数中,我们定义了一个名为newFlowerVase
的新函数,该函数将包装实际函数,并注意此函数将具有与实际函数(flowerVase)完全相同的数字或参数。
Then we are adding some new functionalities (printing some message) to it and calling the actual function with parameter which was sent as argument to the flowerDecorator
. And then finally we returned the newFlowerVase
which received by line 18 and we get the output as we decorated it.
然后,我们向其中添加一些新功能(打印一些消息),并调用带有参数的实际函数,该参数作为参数发送给flowerDecorator
。 最后,我们返回了第18行接收到的newFlowerVase
,并在装饰它时得到输出。
This is how a python function decorator work. If your actual function having more than one arguments then the inner function of the decorator will have the same number of parameter.
这就是python函数装饰器的工作方式。 如果您的实际函数具有多个参数,则装饰器的内部函数将具有相同数量的参数。
Python Decorator Example处理异常 (Python Decorator Example to handle exception)
The following code will print the value of an array with respect to its index.
以下代码将打印数组相对于其索引的值。
array = ['a', 'b', 'c']
def valueOf(index):
print(array[index])
valueOf(0)
This will output:
这将输出:
a
But what if we call the function for,
但是如果我们调用该函数,
valueOf(10)
This will output:
这将输出:
Traceback (most recent call last):
File "D:/T_Code/Pythongenerator/array.py", line 4, in
valueOf(10)
File "D:/T_Code/Pythongenerator/array.py", line 3, in valueOf
print(array[index])
IndexError: list index out of range
As we haven’t handled whether the given index value exceeds the size of the array or not. So now we will decorate this function as following:
由于我们尚未处理给定的索引值是否超过数组的大小。 因此,现在我们将装饰此功能如下:
array = ['a', 'b', 'c']
def decorator(func):
def newValueOf(pos):
if pos >= len(array):
print("Oops! Array index is out of range")
return
func(pos)
return newValueOf
@decorator
def valueOf(index):
print(array[index])
valueOf(10)
Now it will output as below image.
现在它将输出如下图。
So now we have handled the possible error of array out of bound exception. That’s all about python decorator example and how it works.
因此,现在我们已经处理了数组超出范围异常的可能错误。 这就是关于python decorator示例及其工作方式的全部内容。
翻译自: https://www.journaldev.com/14932/python-decorator-example
decorator