函数重载函数的引用算重载吗
介绍 (Introduction)
Today in this tutorial, we are going to understand the concept of Function Overloading in C++.
今天,在本教程中,我们将了解C ++中的函数重载的概念。
So now let’s get started.
现在开始吧。
什么是函数重载? (What is Function Overloading?)
The C++ programming language provides its users with a very useful feature that is function overloading. It allows the users to use the same name for two or more functions with a different number or types of arguments they accept.
C ++编程语言为其用户提供了一个非常有用的功能,即函数重载 。 它允许用户对两个或多个函数使用相同的名称,并接受不同数量或类型的参数。
For example,
例如,
int demo(int);
int demo(int, int);
int demo(float);
Here we have used the same function name demo
for declaration of three different functions. Notice all of them take different number or type of arguments. So, all these functions can be used in a single program and the function call would be solely dependent on the type and number of arguments passed.
在这里,我们使用相同的函数名称demo
来声明三个不同的函数。 请注意,它们全部采用不同数量或类型的参数。 因此,所有这些函数都可以在单个程序中使用,并且函数调用将完全取决于传递的参数的类型和数量。
For instance, the below function (though having different return types) would raise an error if used with the above functions.
例如,如果将以下函数(尽管返回类型不同)与上述函数一起使用,则会引发错误 。
float demo(int)
This is because it takes the same type and number of argument as of the first function declared earlier.
这是因为它采用与先前声明的第一个函数相同的参数类型和数量。
示例–在C ++中使用函数重载计算周长 (Example – Calculating perimeter using Function Overloading in C++)
Now let us look at an easy example where we try to calculate the perimeter of a square/triangle/rectangle on the basis of the user’s choice by implementing the function overloading concept in C++.
现在,让我们看一个简单的示例,在该示例中,我们尝试通过在C ++中实现函数重载概念,根据用户的选择来计算正方形/三角形/矩形的周长。
#include<iostream>
using namespace std;
float peri(float x)
{
return 4*x;
}
float peri(float a, float b, float c)
{
return (a+b+c);
}
float peri(float l, float b)
{
return 2*(l+b);
}
int main()
{
float x, y, z;
int ch;
cout<<"::: Menu for calculating perimeter :::"<<endl;
cout<<"1. Square"<<endl;
cout<<"2. Triangle"<<endl;
cout<<"3. Rectangle"<<endl;
cout<<"Enter your choice(1/2/3) : ";
cin>>ch;
switch(ch)
{
case 1: cout<<"\n\nEnter edge for the Square: ";
cin>>x;
cout<<"\nPerimeter = "<<peri(x)<<" units";
break;
case 2: cout<<"\n\nEnter 1st side length: ";
cin>>x;
cout<<"\nEnter 2nd side length: ";
cin>>y;
cout<<"\nEnter 3rd side length: ";
cin>>z;
cout<<"\nPerimeter = "<<peri(x,y,z)<<" units";
break;
case 3: cout<<"\n\nEnter length for the Rectangle: ";
cin>>x;
cout<<"\nEnter breadth for the Rectangle: ";
cin>>y;
cout<<"\nPerimeter = "<<peri(x,y)<<" units";
break;
default: cout<<"Wrong Choice!";
exit(0);
}
return 0;
}
Outputs:
输出:
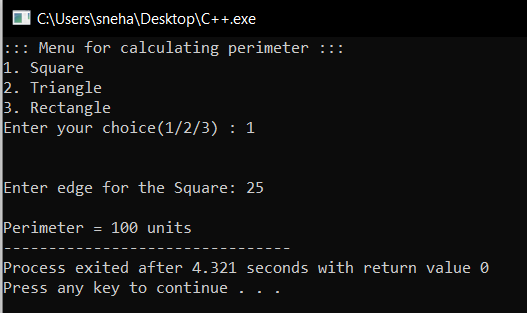

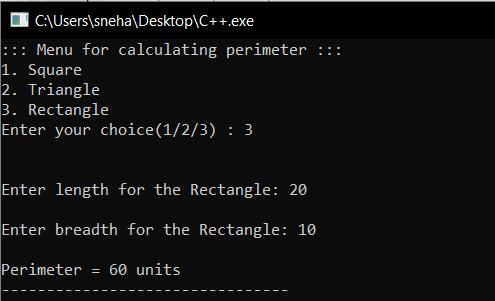
Here, we use a switch-case to calculate the perimeter of any one of the squares, triangles, or rectangles. ch
is the user’s choice which determines the shape for which the data input is to be taken.
在这里,我们使用开关盒来计算正方形,三角形或矩形中任何一个的周长。 ch
是用户的选择,它决定要进行数据输入的形状。
After taking the user’s choice inside the switch-case
we take the data for the different shapes and pass them to the peri()
function in order to print the corresponding perimeter.
在switch-case
进行用户选择之后,我们获取不同形状的数据,并将其传递给peri()
函数,以打印相应的周长。
In this case, the function call for peri()
totally depends on the number of arguments that are passed to it. Hence on a single parameter, the compiler automatically calls the first function and returns the perimeter for a square.
在这种情况下,对peri()
的函数调用完全取决于传递给它的参数数量。 因此,对于单个参数,编译器会自动调用第一个函数,并返回一个正方形的周长。
And accordingly, for two and three parameters, the corresponding functions to calculate the perimeter for a rectangle and a triangle are called respectively.
因此,对于两个和三个参数,分别调用计算矩形和三角形周长的相应函数。
结论 (Conclusion)
So in this tutorial, we learned the concept of function overloading in C++. For any further questions, feel free to use the comments below.
因此,在本教程中,我们学习了C ++ 中函数重载的概念 。 如有其他疑问,请随时使用以下评论。
参考资料 (References)
- Polymorphism in Java – Journal Dev Post, Java中的多态性 – Journal Dev Post,
- Polymorphism in C++ – StackOverflow Question, C ++中的多态性 – StackOverflow问题,
- A confusion about c++ function overloading – StackOverflow Question. 关于c ++函数重载的困惑 – StackOverflow问题。
翻译自: https://www.journaldev.com/38988/function-overloading-in-c-plus-plus
函数重载函数的引用算重载吗