android自定义进度条
Custom progress bar in android application gives it a personal touch. In this tutorial, we’ll create a custom progress bar by implementing a spinning logo icon in our application. Most of the time, we end up using a ProgressBar as the loading icon while the data gets loaded. Going by the current trend, apps like Reddit, UBER, Foodpanda and Twitter have replaced the commonly used Progress Bar with their application’s icon as the loading icon. This gives their application as well as logo brand a touch that makes them stand out from the rest.
android应用程序中的自定义进度栏使其具有个人风格。 在本教程中,我们将通过在应用程序中实现旋转徽标图标来创建自定义进度栏。 大多数情况下,在加载数据时,我们最终使用ProgressBar作为加载图标。 按照当前的趋势,Reddit,UBER,Foodpanda和Twitter之类的应用已将其常用的进度栏替换为应用程序的图标作为加载图标。 这为他们的应用程序和徽标品牌提供了一种触感,使它们与众不同。
Android中的自定义进度栏 (Custom Progress Bar in Android)
Let’s see the classical way of showing a loading icon in our application’s activity.
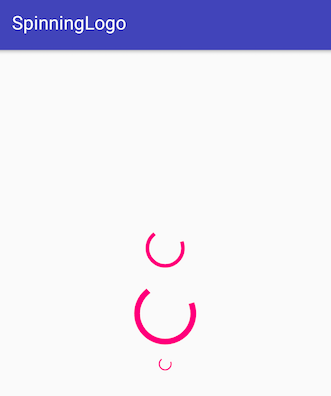
让我们看看在应用程序的活动中显示加载图标的经典方式。
The code for the layout above should look like this:
上面布局的代码应如下所示:
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="https://schemas.android.com/apk/res/android"
xmlns:app="https://schemas.android.com/apk/res-auto"
xmlns:tools="https://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.journaldev.spinninglogo.MainActivity">
<ProgressBar
android:id="@+id/progressBarLarge"
style="?android:attr/progressBarStyleLarge"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ProgressBar
android:id="@+id/progressBarSmall"
style="?android:attr/progressBarStyleSmall"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toBottomOf="@+id/progressBarLarge" />
<ProgressBar
android:id="@+id/progressBarMedium"
style="?android:attr/progressBarStyle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="8dp"
app:layout_constraintBottom_toTopOf="@+id/progressBarLarge"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent" />
</android.support.constraint.ConstraintLayout>
We’ve set three circular ProgressBars that rotate endlessly in the above layout. Now let’s try to add a ProgressBar that spins an icon indeterminately.
我们设置了三个圆形的ProgressBar,它们在上述布局中不断旋转。 现在,让我们尝试添加一个不确定地旋转图标的ProgressBar。
自定义进度栏Android Studio项目结构 (Custom Progress Bar Android Studio Project Structure)
Android自定义进度条码 (Android Custom Progress Bar Code)
The ProgressBar class contains an attribute indeterminateDrawable
which replaces the default indicator with the drawable specified. Let’s see what happens when we place an icon in the ProgressBar.
ProgressBar类包含一个indeterminateDrawable
属性,该属性用指定的drawable替换默认指示器。 让我们看看在进度栏中放置一个图标会发生什么。
The code for activity_main.xml looks like this:
activity_main.xml的代码如下所示:
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="https://schemas.android.com/apk/res/android"
xmlns:app="https://schemas.android.com/apk/res-auto"
xmlns:tools="https://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.journaldev.spinninglogo.MainActivity">
<ProgressBar
android:id="@+id/progressBar"
style="?android:attr/progressBarStyle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:indeterminateDrawable="@mipmap/ic_launcher"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</android.support.constraint.ConstraintLayout>
The output that the above layout reflects in our application is given below.
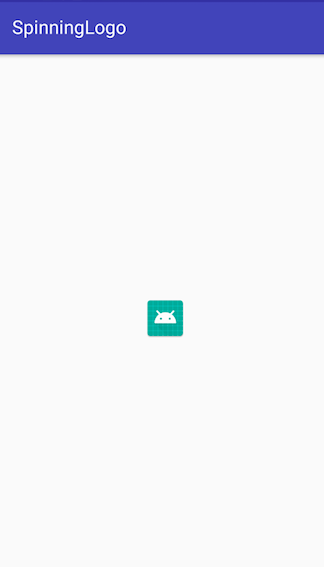
上面的布局在我们的应用程序中反映的输出如下。
Oops! What’s wrong with the ProgressBar? Why isn’t it rotating?
糟糕! ProgressBar有什么问题? 为什么不旋转?
Well we need to set a RotateDrawable as the value of the attribute.
好吧,我们需要将RotateDrawable设置为属性的值。
A RotateDrawable
is defined in the xml by encapsulating the current drawable and assigning it the angle and degrees of rotation. The tag <rotate> is used to do so in the xml as shown below.
通过封装当前可绘制对象并为其分配旋转角度和角度,在xml中定义了RotateDrawable
。 标记<rotate>用于在xml中这样做,如下所示。
The code for the progress_icon.xml
RotateDrawable is given below.
下面给出了progress_icon.xml
RotateDrawable的代码。
<?xml version="1.0" encoding="utf-8"?>
<layer-list xmlns:android="https://schemas.android.com/apk/res/android" >
<item>
<rotate
android:drawable="@mipmap/ic_launcher"
android:fillAfter="true"
android:fromDegrees="0"
android:pivotX="50%"
android:pivotY="50%"
android:toDegrees="360" />
</item>
</layer-list>
The attribute android:fillAfter
indicates that the transformation is applied after the
animation is over.
android:fillAfter
属性指示在
动画结束了。
android:toDegrees
value can be increased or decreased to change the speed of rotation. Generally it’s recommended to set it in multiples of 360.
android:toDegrees
值可以增加或减少以改变旋转速度。 通常,建议将其设置为360的倍数。
Let’s set the above drawable in the ProgressBar present in the activity_main.xml.
让我们在activity_main.xml中存在的ProgressBar中设置上述可绘制对象。
<ProgressBar
android:id="@+id/progressBar"
style="?android:attr/progressBarStyle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:indeterminateDrawable="@drawable/progress_icon"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
The output reflected in the application is shown below.
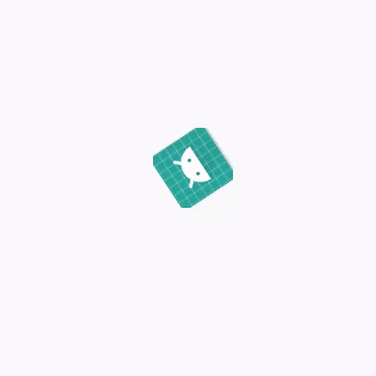
应用程序中反映的输出如下所示。
Let’s create a basic application that displays a string in a TextView from an ArrayList after a delay.
让我们创建一个基本的应用程序,该应用程序在延迟后在ArrayList的TextView中显示一个字符串。
The code for the xml layout file activity_main.xml
is given below.
xml布局文件activity_main.xml
的代码如下。
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="https://schemas.android.com/apk/res/android"
xmlns:app="https://schemas.android.com/apk/res-auto"
xmlns:tools="https://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.journaldev.spinninglogo.MainActivity">
<ProgressBar
android:id="@+id/progressBar"
style="?android:attr/progressBarStyle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:indeterminateDrawable="@drawable/progress_icon"
android:visibility="gone"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="32dp"
android:text="TAP ME TO GET A RANDOM QUOTE"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent" />
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="A Greeting Message Awaits You"
android:textSize="24sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</android.support.constraint.ConstraintLayout>
Take note of the efficient use of ConstraintLayout in the above code.
请注意上面代码中ConstraintLayout的有效使用。
The code for the MainActivity.java is given below.
MainActivity.java的代码如下。
package com.journaldev.spinninglogo;
import android.os.Handler;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.ProgressBar;
import android.widget.TextView;
import java.util.ArrayList;
import java.util.List;
public class MainActivity extends AppCompatActivity {
TextView textView;
List<String> quotesList;
ProgressBar progressBar;
int i = 0;
Handler handler = new Handler();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
quotesList = new ArrayList<>();
quotesList.add("Hi");
quotesList.add("Happy New Year");
quotesList.add("Hope you have a good day");
quotesList.add("Merry Christmas");
Button btnTap = findViewById(R.id.button);
textView = findViewById(R.id.textView);
progressBar = findViewById(R.id.progressBar);
btnTap.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
progressBar.setVisibility(View.VISIBLE);
textView.setVisibility(View.GONE);
handler.postDelayed(new Runnable() {
@Override
public void run() {
if (i == quotesList.size())
i = 0;
textView.setVisibility(View.VISIBLE);
textView.setText(quotesList.get(i++));
progressBar.setVisibility(View.GONE);
}
}, 3000);
}
});
}
}
The output of the above application in action is given below.
下面给出了上面应用程序的输出。
Note: Ignore the flickering rotation in the gifs. It’s absolutely smooth when running on a device.
注意:忽略gifs中的闪烁旋转。 在设备上运行时绝对流畅。
This brings an end to this tutorial. We’ve implemented a RotationDrawable inside a ProgressBar to achieve a spinning logo like indicator. This should be good for showing a loading progress in apps.
本教程到此结束。 我们已经在ProgressBar内实现了RotationDrawable,以实现类似指示器的旋转徽标。 这对于显示应用程序的加载进度应该很好。
You can download the final Android Custom Progress Bar project from the link below.
您可以从下面的链接下载最终的Android Custom Progress Bar项目 。
Reference: API Doc
参考: API文档
翻译自: https://www.journaldev.com/17821/custom-progress-bar-android
android自定义进度条