Project Lombok is a very useful tool for java projects to reduce boiler plate code.
Lombok项目对于Java项目来说是非常有用的工具,可以减少样板代码。
问题陈述 (Problem Statement)
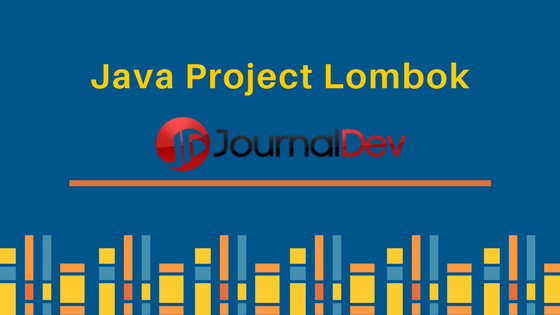
In Java vs. other language debate, the first knock which you get from other language supporters is that Java requires lot of boiler plate code and you just cannot get over it, and you are defenceless. The same issue is also reported in multiple platforms and developer communities.
在Java与其他语言的争论中,您从其他语言支持者那里得到的第一声敲门声是Java需要大量样板代码,而您却无法克服它,而且您毫无防备。 在多个平台和开发人员社区中也报告了相同的问题。
Let’s see a sample of code which has boiler plate code.
让我们看一下具有样板代码的代码示例。
package com.askrakesh.java.manage_boilerplate;
import java.time.LocalDate;
public class Person {
String firstName;
String lastName;
LocalDate dateOfBirth;
public Person(String firstName, String lastName, LocalDate dateOfBirth) {
super();
this.firstName = firstName;
this.lastName = lastName;
this.dateOfBirth = dateOfBirth;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public LocalDate getDateOfBirth() {
return dateOfBirth;
}
public void setDateOfBirth(LocalDate dateOfBirth) {
this.dateOfBirth = dateOfBirth;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((dateOfBirth == null) ? 0 : dateOfBirth.hashCode());
result = prime * result + ((firstName == null) ? 0 : firstName.hashCode());
result = prime * result + ((lastName == null) ? 0 : lastName.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
Person other = (Person) obj;
if (dateOfBirth == null) {
if (other.dateOfBirth != null)
return false;
} else if (!dateOfBirth.equals(other.dateOfBirth))
return false;
if (firstName == null) {
if (other.firstName != null)
return false;
} else if (!firstName.equals(other.firstName))
return false;
if (lastName == null) {
if (other.lastName != null)
return false;
} else if (!lastName.equals(other.lastName))
return false;
return true;
}
@Override
public String toString() {
return "Person [firstName=" + firstName + ", lastName=" + lastName + "dateOfBirth=" + dateOfBirth + "]";
}
}
A class should have getter-setters for the instance variables, equals
& hashCode
method implementation, all field constructors and an implementation of toString
method. This class so far has no business logic and even without it is 80+ lines of code. This is insane.
一个类应该具有实例变量的getter-setter方法, equals
和hashCode
方法的实现,所有字段的构造函数以及toString
方法的实现。 到目前为止,此类没有业务逻辑,即使没有80余行代码也是如此。 疯了吧。
Lombok计划 (Project Lombok)
Project Lombok is a java library that automatically plugs into your editor and build tools and helps reduce the boiler plate code. Let’s see how to setup Lombok project first.
Lombok项目是一个Java库,可自动插入您的编辑器和构建工具,并有助于减少样板代码。 让我们看看如何首先设置Lombok项目。
Java Project Lombok如何工作? (How does Java Project Lombok work?)
Lombok has various annotations which can be used within our code that is be processed during the compile time and appropriate code expansion would take place based on the annotation used.
Lombok具有各种注释,可以在我们的代码内使用这些注释,这些注释在编译期间进行处理,并且将根据所使用的注释对代码进行适当的扩展。
Lombok only does the code reduction in view time, after the compiling the byte code is injected with all the boiler plate. This helps keeping our codebase small, clean and easy to read and maintain.
在编译字节码与所有样板一起注入之后,Lombok仅减少查看时间的代码。 这有助于使我们的代码库保持小巧,整洁,易于阅读和维护。
LombokMaven项目 (Project Lombok Maven)
Adding Lombok in your project is simple. Just add the below dependency in your maven project pom.xml file.
在您的项目中添加Lombok很简单。 只需在您的maven项目pom.xml文件中添加以下依赖项即可。
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.16.20</version>
</dependency>
在IDE(Eclipse)中添加Lombok插件 (Adding the Lombok Plugin in IDE (Eclipse))
Here are the installation steps for Windows:
这是Windows的安装步骤:
- Downloaded jar from https://projectlombok.org/download or use the jar which is downloaded from your maven build. 从https://projectlombok.org/download下载的jar或使用从您的maven版本下载的jar。
- Execute command in terminal:
java -jar lombok.jar
在终端中执行命令:java -jar lombok.jar
- This command will open window as show in the picture below, install and quit the installer and restart eclipse. 该命令将打开如下图所示的窗口,安装并退出安装程序,然后重新启动eclipse。
If you are on MacOS, then following are the steps to use Lombok in your project.
如果您使用的是MacOS,则以下是在项目中使用Lombok的步骤。
- Copy lombok.jar into
Eclipse.app/Contents/MacOS
directory. 将lombok.jar复制到Eclipse.app/Contents/MacOS
目录中。 - Add
-javaagent:lombok.jar
to the end ofEclipse.app/Contents/Eclipse/eclipse.ini
file. 将-javaagent:lombok.jar
添加到Eclipse.app/Contents/Eclipse/eclipse.ini
文件的末尾。 - Restart Eclipse and enable “Annotation Processing” in project properties as shown in below image.
Lombok的Eclipse概述 (Lombok’s peek in Eclipse outline)
After installation let’s check how we can see our reduced boiler plate code? I have recreated the same class as PersonLombok
. Eclipse outline which displays getter and setter for firstName. This was done based on the Lombok’s @Getter
& @Setter
annotation set for instance variable firstName.
安装后,让我们检查一下如何查看减少的样板代码? 我已经重新创建了与PersonLombok
相同的类。 Eclipse大纲,显示firstName的getter和setter。 这是基于Lombok为实例变量firstName设置的@Getter
和@Setter
批注完成的 。
Lombok的Java字节码窥视 (Lombok’s peek in Java byte code)
We can check the addition of getter & setter methods for the firstName from class bytecode.

我们可以检查类字节码中firstName的getter和setter方法的添加。
Lombok项目注释 (Project Lombok Annotations)
Project Lombok provides many annotation which helps to reduce the boiler plate code in various scenarios. Let’s go through a few of them.
Lombok项目提供了许多注释,可帮助减少各种情况下的样板代码。 让我们来看看其中的一些。
构造器注释 (Constructor Annotation)
@AllArgsConstructor public class PersonLombok { @NonNull String firstName; String lastName; LocalDate dateOfBirth; public static void main(String[] args) { new PersonLombok(null, "Kumar", LocalDate.now()); } }
Above code injects the following in the class:
- A constructor with all arguments by @AllArgsConstructor
- Null check during when passing an argument in the Constructor by @NonNull. @NonNull annotation can also be used when passing an argument as a parameter to a method
Here’s the result of the program run.
@RequiredArgsConstructor
generates a constructor with 1 parameter for each field that requires special handling. All non-initialized final fields get a parameter, as well as any fields that are marked as@NonNull
that aren’t initialized where they are declared.@AllArgsConstructor public class PersonLombok { @NonNull String firstName; String lastName; LocalDate dateOfBirth; public static void main(String[] args) { new PersonLombok(null, "Kumar", LocalDate.now()); } }
上面的代码在该类中注入了以下内容:
- 具有@AllArgsConstructor的所有参数的构造函数
- @NonNull在构造函数中传递参数时进行空检查。 将参数作为参数传递给方法时,也可以使用@NonNull批注
这是程序运行的结果。
@RequiredArgsConstructor
为需要特殊处理的每个字段生成一个带有1个参数的构造函数。 所有未初始化的final字段都将获取一个参数,以及所有未声明其位置的,标记为@NonNull
字段。Getter / Setter批注 (Getter/Setter Annotations)
These annotations can be used either at the field or class level. If you want fine grained control then use it at field level. When used at class level all the getter/setters are created. Let’s work on the class we had created above.
@AllArgsConstructor @Getter @Setter public class PersonLombok { String firstName; String lastName; LocalDate dateOfBirth; }
这些注释可以在字段级别或类级别使用。 如果您想要细粒度的控制,请在现场使用它。 在类级别使用时,将创建所有的getter / setter。 让我们继续上面创建的类。
等于,hashCode和toString批注 (equals, hashCode and toString annotations)
It’s recommended to override the
hashCode()
andequals()
methods while creating a class. In Lombok we have@EqualsAndHashCode
annotation which injects code for equals() & hashCode() method as they go together. Additionally a@ToString
annotation provides a toString() implementation. Let’s see this:@AllArgsConstructor @Getter @Setter @EqualsAndHashCode @ToString public class PersonLombok { String firstName; String lastName; LocalDate dateOfBirth; }
We now have achieved to create Person class without any boiler plate code with help of Lombok annotations. However it gets even better we can replace all the annotations used in the above class with
@Data
and get the same functionality.建议在创建类时重写
hashCode()
和equals()
方法。 在Lombok中,我们有@EqualsAndHashCode
批注,当它们一起使用时,它们会为equals()和hashCode()方法注入代码。 另外,@ToString
批注提供了toString()实现。 让我们看看这个:现在,借助Lombok批注,我们无需使用任何模板代码即可创建Person类。 但是,更好的是,我们可以用
@Data
替换上述类中使用的所有注释,并获得相同的功能。基于设计模式的注释 (Design Pattern based Annotations)
@Builder
lets you automatically produce the code required to have your class be instantiable using builder pattern.@Builder public class Employee { String firstName; String lastName; LocalDate dateOfBirth; public static void main(String[] args) { Employee emp = new EmployeeBuilder().firstName("Rakesh") .lastName("Kumar") .dateOfBirth(LocalDate.now()) .build(); } }
@Delegate
generates delegate methods that forward the call to this field on which annotation is used.“Favour Composition over Inheritance“, but this creates a lot of boiler plate code similar to Adapter Pattern. Lombok’s took the clue from Groovy’s annotation by the same name while implementing this functionality. Let’s see an implementation:
Lombok provides functionality for fine grained control in all the annotations.
@Builder
允许您自动生成使用builder模式可实例化您的类所需的代码。@Builder public class Employee { String firstName; String lastName; LocalDate dateOfBirth; public static void main(String[] args) { Employee emp = new EmployeeBuilder().firstName("Rakesh") .lastName("Kumar") .dateOfBirth(LocalDate.now()) .build(); } }
@Delegate
生成委托方法,该方法将调用转发到使用注释的该字段。“ 在继承上偏爱组成 ”,但这会创建许多类似于Adapter Pattern的样板代码。 Lombok在实现此功能时从同名的Groovy注释中获得了线索。 我们来看一个实现:
Lombok在所有注释中提供了用于细粒度控制的功能。
Boiler-Plate:Java架构师在听吗? (Boiler-Plate: Are Java Architects Listening?)
Yes, they are. You need to understand unlike other languages, Java has taken utmost care of upgrading the language such that they do not break any existing codebase which are in older versions of java. This itself is a huge task and cannot be undermined.
对,他们是。 您需要了解与其他语言不同的地方,Java已竭尽全力升级该语言,以使它们不会破坏Java旧版本中的任何现有代码库。 这本身是一项艰巨的任务,不能被破坏。
They are already modifying and building better type inference capabilities in the language which has been rolled out. One of the important features planned for Java 10 is Local-Variable Type Inference. Though the feature has more to do with adding dynamic typing than the boiler plate, but it is a small drop in the Ocean to manage the boiler-plate code.
他们已经在使用已经推出的语言来修改和构建更好的类型推断功能。 Java 10计划的重要功能之一是Local-Variable Type Inference 。 尽管此功能与添加动态类型相比,比样板更多,但是在管理样板代码方面,Ocean只是一小部分。
摘要 (Summary)
Reducing boiler plate code helps in better readability, less code also means less error. Project Lombok is heavily used today in almost all the major organizations. We provided you with the most useful features from Lombok. Hope you give it a try.
减少样板代码有助于提高可读性,更少的代码也意味着更少的错误。 如今,Lombok计划在几乎所有主要组织中都大量使用。 我们为您提供了Lombok最有用的功能。 希望您能尝试一下。
Source Code: You can visit my Github link to download complete source code used in this tutorial.
源代码 :您可以访问我的Github链接来下载本教程中使用的完整源代码。