python gc
Python garbage collection is the memory management mechanism in python. Let’s look into different aspects of garbage collection and how python garbage collection works.
Python垃圾回收是python中的内存管理机制。 让我们研究一下垃圾收集的不同方面以及python垃圾收集的工作方式。
什么是垃圾回收? (What is Garbage Collection?)
Garbage collection is the process of cleaning shared computer memory which is currently being put to use by a running program when that program no longer needs that memory. With Garbage collection, that chunk of memory is cleaned so that other programs (or same program) can use it again.
垃圾收集是清除共享计算机内存的过程,当该程序不再需要该内存时,该内存将由正在运行的程序使用。 通过垃圾回收,该内存块被清除,以便其他程序(或同一程序)可以再次使用它。
Garbage collection is a memory management feature in many programming languages. In this lesson, we will study how this mechanism works in Python.
垃圾回收是许多编程语言中的内存管理功能。 在本课程中,我们将研究此机制在Python中的工作方式。
Python垃圾回收 (Python Garbage Collection)

The process of memory management in Python is straightforward. Python handles its objects by keeping a count to the references each object have in the program, which means, each object stores how many times it is referenced in the program. This count is updated with the program runtime and when it reaches zero, this means it is not reachable from the program anymore. Hence, the memory for this object can be reclaimed and be freed by the interpreter.
Python中的内存管理过程非常简单。 Python通过保持对每个对象在程序中的引用计数来处理其对象,这意味着每个对象存储在程序中被引用的次数。 此计数随程序运行时更新,并且当计数为零时,这意味着不再可以从程序中访问它。 因此,该对象的内存可以被解释器回收并释放。
Let’s study python garbage collection with the help of an example:
让我们借助示例研究python垃圾回收:
class User(object):
def __del__(self):
print("No reference left for {}".format(self))
user1 = User()
user2 = user1
user3 = user1
In this example, we made a class and 3 reference variables pointing to the same object. Let’s visualise this:

在此示例中,我们制作了一个类和3个引用变量指向同一对象。 让我们将其可视化:
Now we let the variables user1, user2, and user3 point to None instead of the User instance.
现在,让变量user1,user2和user3指向None而不是User实例。
>>> user1 = None
>>> user2 = None
>>> user3 = None
No reference left for <__main__.User object at 0x212bee9d9>
With above code, the references have changed to:
使用上面的代码,引用已更改为:
After we assigned the last variable user3
to None
, the object is garbage collected and this calls the __del__
function.
在将最后一个变量user3
分配给None
,该对象将被垃圾回收,这将调用__del__
函数。
垃圾收集如何随实现而变化 (How Garbage collection varies with implementation)
Garbage collection is a mechanism which varies with Python implementations like CPython, Jython or IronPython.
垃圾收集是一种机制,它随诸如CPython,Jython或IronPython之类的Python实现而变化。
- C Implementation of Python uses reference counting to track unreachable objects. It doesn’t track the objects at each line of execution instead, it periodically executes a cycle detection algorithm which looks for inaccessible objects and cleans them. Python的C实现使用引用计数来跟踪无法访问的对象。 它不会在执行的每一行都跟踪对象,而是定期执行循环检测算法,以查找无法访问的对象并清除它们。
- Jython uses the JVM’s garbage collector. The same applies to IronPython which uses the CLR garbage collector Jython使用JVM的垃圾收集器。 同样适用于使用CLR垃圾收集器的IronPython
If you want to study about the gc interface, do have a look at the Python docs.
如果您想研究gc接口 ,请查看Python文档。
Python Force垃圾收集 (Python Force Garbage Collection)
As we studied above, the Garbage collection runs automatically as the program is under execution, sometimes, we might want to run the Garbage collection at a specific time. We can do this by calling collect()
function. Let’s try to define a LinkedList class to demonstrate this:
如上文所述,Garbage集合会在程序执行时自动运行,有时,我们可能希望在特定时间运行Garbage集合。 我们可以通过调用collect()
函数来实现。 让我们尝试定义一个LinkedList类来演示这一点:
class LinkedList(object):
def __init__(self, name):
self.name = name
self.next = None
def set_next(self, next):
print('Linking nodes %s.next = %s' % (self, next))
self.next = next
def __repr__(self):
return '%s(%s)' % (self.__class__.__name__, self.name)
Once that is done, we can start constructing their objects and trigger Garbage collection manually:
完成后,我们可以开始构造它们的对象并手动触发垃圾回收:
# Constructing a circular LinkedList
a = LinkedList('1')
b = LinkedList('2')
c = LinkedList('3')
a.set_next(b)
b.set_next(c)
c.set_next(a)
# Remove references to the LinkedList nodes in this module's namespace
a = b = c = None
# Show the effect of garbage collection
for i in range(2):
print('Collecting %d ...' % i)
n = gc.collect()
print('Unreachable objects:', n)
print('Remaining Garbage:', pprint.pprint(gc.garbage))
print
When we run this, the output will be:
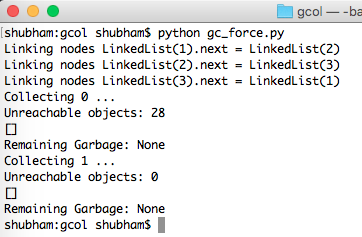
当我们运行它时,输出将是:
In this example, the cyclic LinkedList objects are cleared as soon as garbrge collection runs the first time.
在此示例中,第一次运行Garbrge收集时,便会清除循环LinkedList对象。
Python垃圾收集摘要 (Python Garbage Collection Summary)
Here, let’s provide some final ways through which we can optimise the use of Garbage collection:
在这里,让我们提供一些最终方法,通过这些方法可以优化垃圾回收的使用:
- Do not force collect garbage too many times. This is because that even if are freeing the memory, it still takes time to evaluate an object’s eligibility for being Garbage collected. 不要强行收集垃圾太多次。 这是因为即使释放内存,仍然需要花费时间来评估对象是否符合垃圾收集条件。
- If you want to manually manage the Garbage Collection in your application, start doing it only after the app has completely started and then continue doing so in steady operations. 如果要在应用程序中手动管理垃圾收集,请仅在应用程序完全启动后才开始进行操作,然后在稳定运行时继续进行操作。
Garbage collection is a tricky mechanism if managed manually. To get it right, study the contract closely.
如果手动进行管理,则垃圾回收是一个棘手的机制。 为正确起见,请仔细研究合同。
翻译自: https://www.journaldev.com/17927/python-garbage-collection-gc
python gc