Python itertools module is very useful in creating efficient iterators. In almost every program you write with any programming language, one of the task which is usually always present is Iteration. Traversing sequence of objects and manipulating them is very common.
Python itertools模块在创建有效的迭代器时非常有用。 在使用任何编程语言编写的几乎每个程序中,迭代始终是经常存在的任务之一。 遍历对象序列并对其进行操作非常普遍。
Many times while doing these common operations, we miss out on managing memory usage of the variables, size of the sequence being iterated and create a risk of inefficient code usage. With itertools
module in Python, this can be prevented from happening with its functions.
很多时候,在执行这些通用操作时,我们会错过管理变量的内存使用情况,要迭代的序列大小以及创建代码使用效率低下的风险。 使用Python中的itertools
模块,可以防止其功能发生这种情况。
Python itertools模块 (Python itertools module)
Python itertools module provide us various ways to manipulate the sequence while we are traversing it. Some of the most commons examples are shared here.
Python itertools 模块为我们提供了多种遍历序列的方式。 这里共享一些最常见的示例。
Python itertools链() (Python itertools chain())
Python itertools chain()
function just accepts multiple iterable and return a single sequence as if all items belongs to that sequence.
Python itertools chain()
函数仅接受多个iterable并返回单个序列,就好像所有项目都属于该序列一样。
Syntax for chain works as:
链的语法如下:
itertools.chain(*sequence)
Let’s put this in an example:
让我们举个例子:
from itertools import *
for value in chain([12.3, 2.5, 34.13], ['JournalDev', 'Python', 'Java']):
print(value)
The output will be:
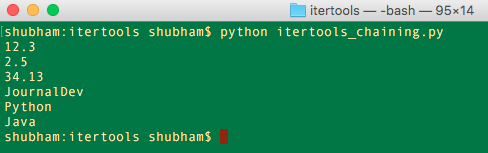
输出将是:
It doesn’t matter if sequences passed were for different data type.
传递的序列是否用于不同的数据类型都没有关系。
使用islice()和count()自动执行序列 (Automating sequences with islice() and count())
Itertools islice()
and count()
functions provide us an easy to make quick iterable and slice them through.
Itertools的islice()
和count()
函数使我们可以轻松实现快速迭代并切分它们。
Let’s provide a sample code snippet:
让我们提供一个示例代码片段:
from itertools import *
for num in islice(count(), 4):
print(num)
print('I stopped at 4.')
for num in islice(count(), 15, 20):
print(num)
print('I started at 15 and stopped at 20.')
for num in islice(count(), 5, 50, 10):
print(num)
print('I started at 5 and leaped by 10 till 50.')
The output will be:
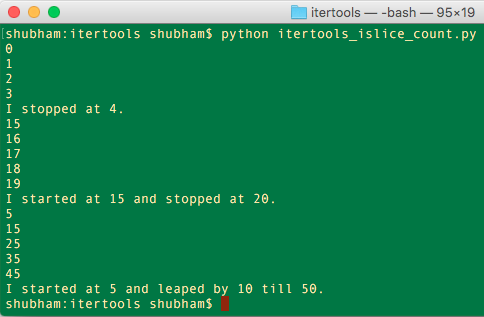
输出将是:
使用tee()克隆序列 (Cloning sequences with tee())
The best way through which we can make a clone of a sequence is using tee()
function. Remember that original sequence cannot be used once we have cloned into into 2 sequences.
克隆序列的最佳方法是使用tee()
函数。 请记住,一旦我们克隆为2个序列,就无法使用原始序列。
Let’s put this in an example:
让我们举个例子:
from itertools import *
single_iterator = islice(count(), 3)
cloned1, cloned2 = tee(single_iterator)
for num in cloned1:
print('cloned1: {}'.format(num))
for num in cloned2:
print('cloned2: {}'.format(num))
The output will be:
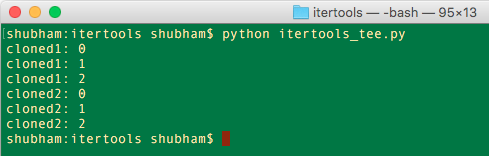
输出将是:
使用cycle()在序列中循环 (Cycling through sequences with cycle())
We can even iterate through a sequence has it was infinite. This works just like a circular Linked List.
我们甚至可以遍历一个无限的序列。 这就像循环的链表一样工作。
Syntax for cycle
works as:
cycle
语法如下:
itertools.cycle(sequence)
Let’s provide a sample code snippet:
让我们提供一个示例代码片段:
from itertools import *
index = 0
for item in cycle(['Python', 'Java', 'Ruby']):
index += 1
if index == 12:
break
print(index, item)
The output will be:
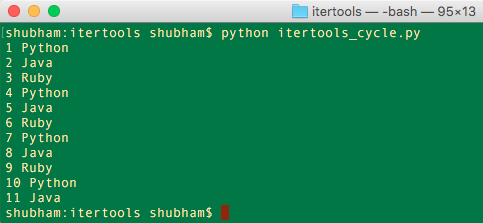
输出将是:
使用accumulate()进行累加运算 (Accumulating operations with accumulate())
With accumulate()
function, we can perform mathematical operations with a sequence and return the results. Like adding the numbers to the previous value in the sequence. Let’s put this in an example:
使用accumulate()
函数,我们可以按顺序执行数学运算并返回结果。 就像将数字加到序列中的前一个值。 让我们举个例子:
from itertools import *
data = accumulate(range(10))
for item in data:
print(item)
The output will be:
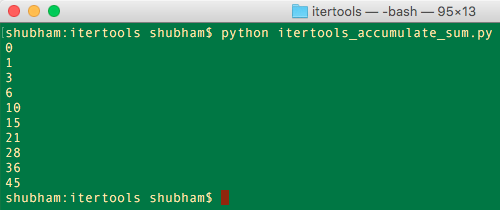
输出将是:
Let’s try another operator like multiplication
as:
让我们尝试另一个像multiplication
这样的multiplication
符:
from itertools import *
import operator
data = accumulate(range(1, 5), operator.mul)
for item in data:
print(item)
The output will be:
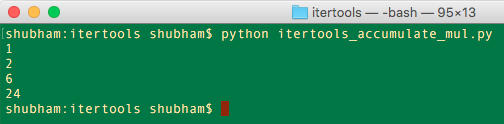
输出将是:
用dropwhile()过滤项目 (Filter items with dropwhile())
With dropwhile()
function, we can filer sequence items until a condition becomes False. Once it becomes False, it stops the filter process.
使用dropwhile()
函数,我们可以过滤序列项,直到条件变为False为止 。 一旦变为False,它将停止过滤过程。
Syntax for dropwhile
works as:
dropwhile
语法如下:
itertools.dropwhile(predicate, sequence)
Let’s provide a sample code snippet:
让我们提供一个示例代码片段:
from itertools import *
data = dropwhile(lambda x: x < 5, [3, 12, 7, 1, -5])
for item in data:
print(item)
The output will be:
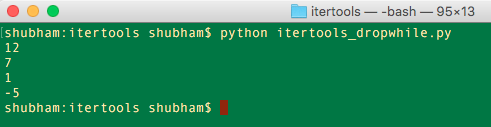
输出将是:
使用takewhile()过滤项目 (Filter items with takewhile())
With takewhile()
function, we can filer sequence items until a condition becomes True. Once it becomes True, it stops the filter process.
使用takewhile()
函数,我们可以过滤序列项,直到条件变为True为止 。 一旦变为True,它将停止过滤过程。
Syntax for takewhile
works as:
takewhile
语法如下:
itertools.takewhile(predicate, sequence)
Let’s put this in an example:
让我们举个例子:
from itertools import *
data = takewhile(lambda x: x < 5, [3, 12, 7, 1, -5])
for item in data:
print(item)
The output will be:

输出将是:
用Combines()进行组合 (Making combinations with combinations())
When it comes to making combinations of all the values in a list, custom logic can go wrong in any number of ways. Even here, itertools module has a rescue function.
在组合列表中的所有值时,自定义逻辑可能会以多种方式出错。 即使在这里,itertools模块也具有救援功能。
Syntax for combinations()
works as:
Combines combinations()
语法如下:
itertools.combinations(sequence, r)
Let’s provide a sample code snippet:
让我们提供一个示例代码片段:
from itertools import *
data = list(combinations('ABCD', 2))
for item in data:
print(item)
The output will be:
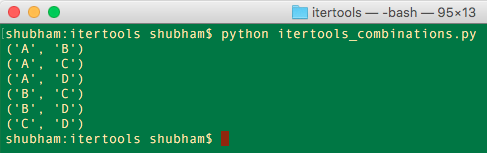
It is worth noticing that:
输出将是:
值得注意的是:
- If items in sequence are sorted, than combination will be sorted as well. 如果按顺序对项目进行排序,则组合也将进行排序。
- If items in sequence are unique, than combination data will not contain any duplicate combination. 如果序列中的项目是唯一的,则组合数据将不包含任何重复的组合。
重复的组合,与groups_with_replacement() (Repeated combinations with combinations_with_replacement())
This works just like the combinations()
function as shown above. Only difference that this can have repeatitions in combination data.
就像上面显示的combinations()
函数一样工作。 唯一的区别在于它可以在组合数据中重复。
Syntax for combinations_with_replacement
works as:
语法combinations_with_replacement
作品:
itertools.combinations_with_replacement(sequence, r)
Let’s put this in an example:
让我们举个例子:
from itertools import *
data = list(combinations_with_replacement('ABCD', 2))
for item in data:
print(item)
The output will be:
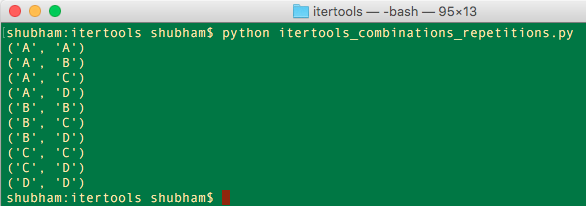
输出将是:
带有compress()的压缩过滤器 (Compression filter with compress())
Data compression is easy based on a Boolean list using the compress()
function.
使用compress()
函数基于布尔列表轻松进行数据压缩。
Syntax for compress works as:
compress的语法如下:
itertools.compress(sequence, selector)
Let’s provide a sample code snippet:
让我们提供一个示例代码片段:
from itertools import *
filtered = [True, False, False, True, True]
to_filter = 'PQRSTUVW'
data = list(compress(to_filter, filtered))
for item in data:
print(item)
The output will be:
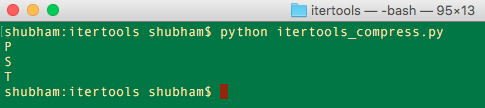
输出将是:
In this lesson, we learned about various ways through which we can iterate and manipulate sequences with python itertools
module.
在本课程中,我们学习了各种方法,可以通过这些方法使用python itertools
模块迭代和操作序列。
Reference: API Doc
参考: API文档