c ++ 继承
Hi! This time we are here with the topic – Inheritance in C++.
嗨! 这次我们将讨论主题– C ++中的继承 。
As we know Inheritance is a very important concept or aspect in Object-Oriented Programming.
众所周知,继承是面向对象编程中非常重要的概念或方面。
什么是继承? (What is Inheritance?)
Theoretically, C++ Inheritance is the capability of one class of things to inherit capabilities or properties from another class.
从理论上讲, C ++继承是一类事物从另一类继承能力或属性的能力。
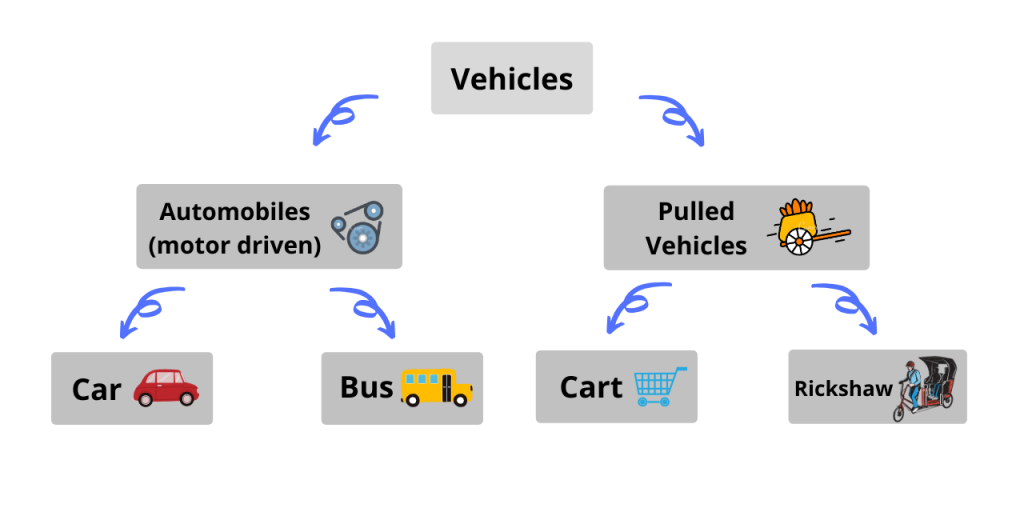
Now consider the above Vehicles example. Here, the “Car” inherits some of its properties from the class “Automobiles” which again inherits some properties from the “Vehicles” class. This capability of passing down properties is very useful for supporting the OOP approach.
现在考虑上面的车辆示例。 在这里, “汽车”继承了“汽车”类的某些属性,而汽车又继承了“汽车”类的一些属性。 传递属性的这种能力对于支持OOP方法非常有用。
Hence, C++ Inheritance helps us build closer to the real-world model. The principle behind here is that each subclass shares common characteristics with the class from which it is derived.
因此,C ++继承可以帮助我们更接近实际模型。 此处的原理是,每个子类都与其派生类具有共同的特征。
Again for the example above, ‘Automobiles’ and ‘Pulled Vehicles’ are sub-classes of ‘Vehicles’. On the other hand, ‘Vehicles’ is the base class for them.
再次对于上面的例子中,“汽车”和“拉车辆”是“汽车”的子类。 另一方面, “车辆”是它们的基类。
Similarly, ‘Automobiles’ is the base-class of ‘Car’ and ‘Bus’. And ‘Car’ and ‘Bus’ are sub-classes of ‘Automobiles’.
同样, “汽车”是“汽车”和“公共汽车”的基本类别。 而“汽车”和“公共汽车”是“汽车”的子类。
C ++继承 (C++ Inheritance)
Syntax for C++ Single Inheritance is given below:
C ++单一继承的语法如下:
class derived_class_name: access_mode base_class_name
{
/*body or properties*/
};
Here, derived_class_name
is the name of the sub-class and base_class_name
is the name of the base class. The access_mode
or the access specifier defines which members of the base class are accessible from the derived classes. You can follow the given table for C++ access specifier definitions.
在此, derived_class_name
是子类的名称,而base_class_name
是基类的名称。 access_mode
或access指定符定义可从派生类访问基类的哪些成员。 您可以按照给定的表格获取C ++访问说明符定义。
Access Mode | public | protected | private |
members of the same class | Yes | Yes | Yes |
members of derived class | Yes | Yes | No |
not members | Yes | No | No |
存取模式 | 上市 | 受保护的 | 私人的 |
同班同学 | 是 | 是 | 是 |
派生类的成员 | 是 | 是 | 没有 |
不是会员 | 是 | 没有 | 没有 |
Considering the ‘Vehicles’ example again(this time just the ‘Vehicles’, ‘Automobiles’ and ‘Pulled Vehicles’ classes). We can represent this C++ single level inheritance as shown in the code snippet below.
再次考虑“车辆”示例(这次仅是“车辆”,“汽车”和“牵引车”类)。 我们可以表示此C ++单级继承,如下面的代码片段所示。
#include<iostream>
#include<cstring>
using namespace std;
class Vehicles //base-class
{
// public members
public:
int No_of_wheels;
void set_wheels(int w)
{
No_of_wheels = w;
}
};
class Automobiles : public Vehicles // sub-class(inherits from Vehicles)
{
// members
public:
string fuel;
void set_fuel_type(string str)
{
fuel = str;
}
};
class pulled_vehicles : public Vehicles // sub-class(inherits from Vehicles)
{
// members
public:
string puller;
void set_puller(string str)
{
puller = str;
}
};
int main()
{
Automobiles A1; // Automobiles object created
A1.set_wheels(4); // member function of Vehicles
A1.set_fuel_type("Petrol"); // member function of Automobiles
pulled_vehicles P1; // pulled_vehicles object created
P1.set_wheels(3); // member function of Vehicles
P1.set_puller("Horse"); // member function of pulled_vehicles
// A1 properties
cout<<"A1:"<<endl;
cout<<"No. of wheels = "<<A1.No_of_wheels<<endl;
cout<<"Fule Type = "<<A1.fuel<<endl;
// P1 properties
cout<<"\nP1:"<<endl;
cout<<"No. of wheels = "<<P1.No_of_wheels<<endl;
cout<<"Pulled by = "<<P1.puller<<endl;
return 0;
}
Output:
输出:
A1:
No. of wheels = 4
Fule Type = Petrol
P1:
No. of wheels = 3
Pulled by = Horse
C ++多重继承 (C++ Multiple Inheritance)
A class can also be derived from more than one base class. In that case, the subclass inherits the properties from all the base-classes. This feature in C++ is termed as Multiple Inheritance.
一个类也可以从多个基类派生。 在这种情况下,子类将从所有基类继承属性。 在C ++中,此功能称为多重继承 。
For using this C++ feature, the base classes along with their access modes should be separated by a comma(','
). The syntax for doing so can be given as:
为了使用此C ++功能,基类及其访问模式应使用逗号( ','
)分隔。 这样做的语法可以给出为:
class derived_class_name: access_mode1 base_class_name1, access_mode2 base_class_name2, ... , access_modeN base_class_nameN
{
/*body or properties*/
};
Now let us take an example where a class(C
) inherits properties from two other classes(base-classes A
and B
). We can visualize this simple multiple inheritance model as shown below.
现在让我们举一个例子,一个类C
从其他两个类(基类A
和B
)继承属性。 我们可以可视化此简单的多继承模型,如下所示。

#include<iostream>
#include<cstring>
using namespace std;
class A //base-class for C
{
public:
void A_print()
{
cout<<"We are in class A"<<endl;
}
};
class B // Another base-class for C
{
public:
void B_print()
{
cout<<"We are in class B"<<endl;
}
};
class C : public A, public B // Multiple Inheritance
{
//body
};
int main()
{
C c1; // object of class C
c1.A_print();
c1.B_print();
return 0;
}
Output:
输出:
We are in class A
We are in class B
As you can see, here we are able to access the public
members of the base classes A
and B
from C
. Hence, it is clear that C
inherits all the properties from its base classes(A and B).
如您所见,在这里我们可以从C
访问基类A
和B
的public
成员。 因此,很明显, C
从其基类(A和B)继承了所有属性。
C ++中继承的重要性 (Importance of Inheritance in C++)
- Real-world Resemblance – The concept of Inheritance in any OOP language gives a user the capability to express inheritance relationship which makes it ensure the closeness with the real-world models(as mentioned earlier), 真实世界的相似性 –任何OOP语言中的继承概念都使用户能够表达继承关系,从而确保与真实世界模型的紧密联系(如前所述),
- Reusability – It also allows the addition of additional features to an existing class without modifying it, 可重用性 –它还允许在不修改现有类的情况下向其添加其他功能,
- Transitive nature – Inheritance is transitive. For example, if class
A
inherits properties of another classB
, then all the sub-classes ofA
will automatically inherit the properties ofB
. This property is called transitive nature of Inheritance. 传递性 –继承是传递性的。 例如,如果类A
另一个类的继承属性B
,则所有的子类A
都会自动继承的属性B
。 此属性称为继承的传递性。
加起来 (Summing Up)
So, that’s it for today. Hope you had a clear understanding of the concept of Inheritance in C++.
所以,今天就这样。 希望您对C ++中的继承概念有清楚的了解。
We recommend you to go through our C++ Tutorial for more info.
我们建议您阅读我们的C ++教程以获取更多信息。
For any further questions related to this topic, feel free to use the comments below.
对于与该主题有关的任何其他问题,请随时使用以下评论。
参考资料 (References)
- OOPS Interview Questions and Answers, OOPS面试问答 ,
- OOPS Concepts in Java – OOPS Concepts Example, Java中的OOPS概念– OOPS概念示例 ,
- Friendship and inheritance – Documentation, 友谊与继承 –文档,
- Friend Function in C++. C ++中的Friend函数 。
翻译自: https://www.journaldev.com/41077/inheritance-in-c-plus-plus
c ++ 继承