This article is the part of a series of articles that intends to demonstrate ‘
本文是旨在演示“
How to build a content management system如何建立内容管理系统This article shows how a single PHP file can be used to act as an interface to the content within our database, controlling which views are displayed and actions are performed.
本文说明如何使用单个PHP文件充当数据库中内容的接口,控制显示哪些视图和执行操作。
The first thing to note is that unlike other websites, there will only ever be one file presented to the web user, the index.php file. Every click, navigation or action that is performed on the website, will be directed back towards the same file. This file will then act as the controller for the whole site and depending upon what input is given, the file will determine what view is presented back to the user.
首先要注意的是,与其他网站不同,将只有一个文件呈现给Web用户,即index.php文件。 在网站上执行的每次单击,导航或操作都将被定向回同一文件。 然后,该文件将充当整个站点的控制器,并且根据给出的输入内容,文件将确定向用户呈现哪些视图。
With this focus on one single file, we then gain greater control over exactly what happens every time an action is performed on the site. The following steps are performed every time the index.php file is called.
通过将重点放在一个文件上,我们可以更好地控制每次在站点上执行操作时所发生的确切情况。 每次调用index.php文件时,将执行以下步骤。
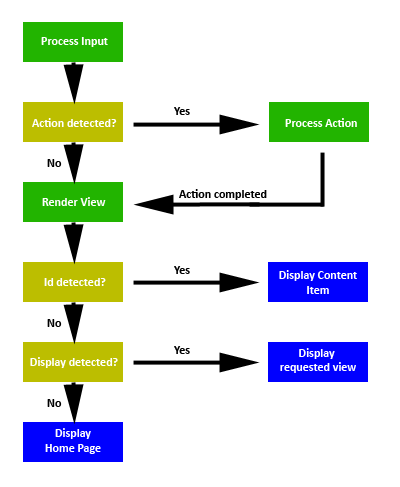
Process Input
流程输入
Input is sent to the system in one of two different ways.
输入以两种不同方式之一发送到系统。
The first of which is by appending parameters to the URL i.e. index.php?id=1. In this example, the parameter being passed is [id] and it has the value of 1. We access this using the $_GET[]‘id’] command.
首先是通过将参数附加到URL,即index.php?id = 1。 在此示例中,要传递的参数为[id],其值为1。我们使用$ _GET []'id']命令进行访问。
The second method uses a HTML form to POST data back to the system. With this method, the data is packaged in the HTTP header request, so it is not visible to the user. We access data sent by this method using $_POST[]‘id’] in our code. This is the preferred method, when performing any action that will make changes to our content.
第二种方法使用HTML表单将数据回传到系统。 使用此方法,数据被打包在HTTP标头请求中,因此用户看不到它。 我们在代码中使用$ _POST []'id']访问通过此方法发送的数据。 当执行任何会更改我们内容的操作时,这是首选方法。
Irrespective of which method is used, we process the input and store each parameter in a variable by the same name. So in the first example, we can access the PHP variable $id if it has been sent.
无论使用哪种方法,我们都会处理输入并将每个参数以相同的名称存储在变量中。 因此,在第一个示例中,我们可以访问PHP变量$ id(如果已发送)。
Process Actions
流程动作
If the input that has been processed in the step above, requests a change to be made to some of our content items, then that action is then carried out at this stage.
如果在以上步骤中已处理的输入请求对某些内容项进行更改,则在此阶段执行该操作。
Possible actions might be:
可能的操作可能是:
- Add an item 新增项目
- Edit an item 编辑项目
- Delete an item 删除项目
- Process login 流程登录
- Process Logout 流程注销
The more you develop your system, the greater this list will become. For each of the actions, you will create a new block of code, which will only executed should the correct set of input be presented.
您开发的系统越多,此列表将变得越多。 对于每个动作,您将创建一个新的代码块,仅在提供正确的输入集时才执行。
Render View
渲染视图
In this final step, the system determines what view should be shown to the user.
在最后一步中,系统确定应向用户显示哪个视图。
The system needs to determine, does the user want to:
系统需要确定用户是否要:
- View content items, or 查看内容项,或
- Access the management area 进入管理区
To determine if a user wants to view a content item, the system looks out for one of two combinations of input:
为了确定用户是否要查看内容项,系统会寻找两种输入组合之一:
- Only [id] – in which case we assume navigation type and translate to [type] and [typeid]
(i.e. index.php?id=1 -> translates nav [id=1] from table) 仅[id] –在这种情况下,我们假设导航类型并将其转换为[type]和[typeid]
- Both [type] and [typeid] – in which case we deliver specified content item
(i.e. index.php?type=page&typeid=1 -> deliver page [id=1]) [type]和[typeid] –在这种情况下,我们交付指定的内容项
We determine if the user wants to access this area by searching for the [display] parameter in the address bar. If this is found, we then display the requested view.
我们通过在地址栏中搜索[display]参数来确定用户是否要访问该区域。 如果找到了,我们将显示请求的视图。
Examples of the type of views display might be:
视图类型的显示示例可能是:
- List of items (with links to perform actions) 项目列表(带有执行操作的链接)
- Forms (to provide input) 表格(提供输入)
- Search box 搜索框
<html>
<body>
<h1><u>CMS Example #1</u></h1>
<ul>
<li>Find content item using 'nav' type <a href="index.php?id=1">click here</a>.</li>
<li>Dislay type 'page' with id '1' <a href="index.php?type=page&typeid=1">click here</a>.</li>
<li>Display login view <a href="index.php?display=login">click here</a>.</li>
</ul>
<?php
/* Enable error reporting */
error_reporting(E_ALL);
ini_set('display_errors', 1);
/* process input - create a variable for each input and assign the value*/
foreach($_REQUEST as $key => $value){ $$key = $value;}
/* process actions */
if(isset($action)){
echo "<p>PROCESSING ACTION...</p>";
if($action=="login"){
echo "<p>PROCESSING LOGIN...</p>";
unset($action);
echo "<p>LOGIN SUCCESS... REDIRECTING TO DASHBOARD</p>";
$display="dashboard";
}
}
/* find homepage */
$homepageid=1;
if(isset($display)){
if($display=="login") {
echo "<p>LOGIN VIEW<p>
<p>To login, please <a href='index.php?action=login'>click here</a>.";
}elseif($display=="dashboard") {
echo "<p>DASHBOARD VIEW</p>
<ul>
<li>To view all pages <a href='index.php?display=view&type=page'>click here</a>.</li>
<li>To add a new page <a href='index.php?display=add&type=page'>click here</a>.</li>
</ul>";
}elseif($display=="add") {
echo "<p>ADD VIEW</p>";
}elseif($display=="view") {
echo "<p>DISPLAY ALL VIEW</p>";
}
}elseif(isset($id)||(isset($type)&&isset($typeid))){
/* process nav to determine type & typeid */
if(isset($id)){
echo "<p>TRANSLATING NAV TYPE</p>";
/*for example only assign type=page and typeid=1*/
$type="page";
$typeid="1";
unset($id);
}
/* display content item */
echo "<p>DISPLAY CONTENT ITEM</p>";
echo "TYPE=".$type;
echo "ID=".$typeid;
}else{
/* display home page */
echo "<p>HOME PAGE</p>";
}
?>
</body>
</html>
In short, the code does the following:
简而言之,该代码执行以下操作:
- Enables error handling 启用错误处理
- Processes input 处理输入
- Processes actions 处理动作
- Finds the home page 查找主页
- Output display if requested 输出显示(如果需要)
- Translates navigation if detected 如果检测到则翻译导航
- Outputs content item if detected 如果检测到输出内容项
As you can imagine however, no user will be given access to these views without us having confirmed that they are authorised to see it.
您可以想象,如果没有我们确认他们有权查看这些视图,则不会授予任何用户访问这些视图的权限。
To summarize, in this section we have shown how a single PHP file can control what is output is presented to the user, based on the input received, thus creating a single interface that we will later connect to other functionality, that will enable us to access and manipulate the content within our system.
总而言之,在本节中,我们展示了单个PHP文件如何基于接收到的输入来控制向用户呈现的输出,从而创建了一个单个接口,稍后我们将其连接到其他功能,从而使我们能够访问和操作我们系统中的内容。
In the next article 'How to build a CMS: Displaying the content' we will show how the system identifies, retrieves and displays the requested content item, and also how we use the navigation layer to hide non-essential and possibly restricted content from the user.
在下一篇文章`` 如何构建CMS:显示内容 ''中,我们将展示系统如何识别,检索和显示所请求的内容项目,以及我们如何使用导航层从以下位置隐藏不必要的内容以及可能受限制的内容用户。
翻译自: https://www.experts-exchange.com/articles/20119/How-to-build-a-CMS-Defining-the-system.html