介绍 (Introduction)
当许多人想到 WebBrowser control, they immediately think of a control which allows the viewing and navigation of web pages. While this is true, it's also very useful for displaying your own custom User Interface (UI). Those members that have used WebBrowser控件时,他们立即想到允许查看和导航网页的控件。 确实如此,但对于显示您自己的自定义用户界面(UI)也非常有用。 那些使用 QuickEE will have seen it in action - it is the basis of the Question View pane. QuickEE的成员将会看到它的实际效果-它是“问题视图”窗格的基础。In this article, I will explain how you can raise events from the WebBrowsers DHTML document, and have them handled by your application. For instance, a user might click an HTML <button> object and your application program can then take action to handle that click.
在本文中,我将解释如何从WebBrowsers DHTML文档引发事件,并由应用程序处理事件。 例如,用户可能单击HTML <button>对象,然后您的应用程序可以采取措施来处理该单击。
设定
(Setting it up
)
DHTML与您的应用程序之间的交互是由组件对象模型(COM)处理的,因此在处理事件之前,需要确保包含客户代码的类对COM可见。 有两种方法可以实现此目的。 使您的程序集或类对COM交互可见。
1.使您的[i]组件[/ i] COM可见 (1. Make your [i]assembly[/i] COM-Visible)
一种选择是通过转到“程序集信息”对话框(“项目”>“ [AppName]属性”>“应用程序”选项卡>“程序集信息”)使整个程序集对COM可见,并确保选中“使程序集COM-可见”复选框: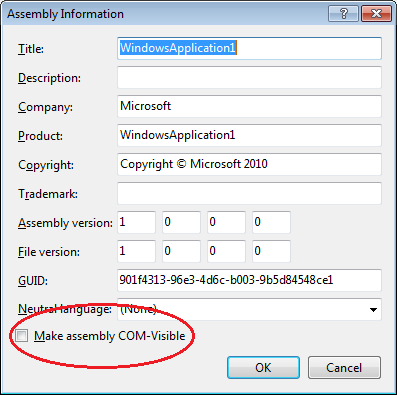
...OR...
...要么...
2.使[i]类[/ i]成为COM-Visible (2. Make the [i]class[/i] COM-Visible)
第二个(也是推荐的)方法是使父类仅对COM可见。 在大多数情况下,父类将是包含WebBrowser控件的Form的类。 这是通过将ComVisibleAttribute添加到类中来完成的。<System.Runtime.InteropServices.ComVisible(True)> _
Public Class Form1
....
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
WebBrowser1.ObjectForScripting = Me
End Sub
将文档添加到您的WebBrowser (Adding the Document to your WebBrowser)
QuickEE使用 Extensible Stylesheet Language Transformations (XSLT) to transform an XML document to HTML, however you can use whatever method you desire. For the purposes of this article, we will use simple HTML and add it to the WebBrowser control with its DocumentText property. 可扩展样式表语言转换(XSLT)将XML文档转换为HTML,但是您可以使用所需的任何方法。 出于本文的目的,我们将使用简单HTML并将其带有DocumentText属性添加到WebBrowser控件中。Before adding the HTML to the WebBrowser, it's important to know how to raise the events to be handled by your application. This is done by calling the DHTML window.external object, along with the name and arguments of the appropriate routine in your application.
在将HTML添加到WebBrowser之前,重要的是要知道如何引发由应用程序处理的事件。 这是通过调用DHTML window.external对象以及应用程序中适当例程的名称和参数来完成的。
Here's an example:
这是一个例子:
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.0 Transitional//EN">
<htm>
<body>
<button onclick="window.external.SimpleCall()">Call an application routine from script</button><br />
<button onclick="window.external.ParameterCall(99, 'some text')">Call an application routine from script, with parameters</button><br />
<br />
<input type="text" value="Some text" id="txtTest"/><br />
<button onclick="window.external.ReferenceCall('txtTest')">Call an application routine from script, sending an elements ID</button><br />
<br />
Reference the calling element<input type="checkbox" Checked="Checked" value="test" onclick="window.external.ReferenceCall2(this)"/>
</body>
</html>
WebBrowser1.DocumentText = HTMLstring
处理代码中的脚本调用 (Handling the Script calls in your code)
Now that you have your WebBrowser set up with the right properties and a document, it's time to add the routines to your application. As mentioned earlier, the WebBrowsers parent class needs to be COM-visible. The reason for this is it will contain the routines which are called by the window.external object of the browser's DOM.
现在,您已经为WebBrowser设置了正确的属性和文档,是时候将例程添加到应用程序了。 如前所述,WebBrowsers父类需要是COM可见的。 原因是它将包含由浏览器DOM的window.external对象调用的例程。
The first two calls ('SimpleCall' and 'ParameterCall') are easy to handle....
前两个调用(“ SimpleCall”和“ ParameterCall”)很容易处理...。
Public Sub SimpleCall()
MsgBox("Simple Call")
End Sub
Public Sub ParameterCall(ByVal i As Integer, ByVal s As String)
MsgBox(String.Format("i = {0}, s = {1}", i, s))
End Sub
The third call ('ReferenceCall') requires some special handling because it references an element within the HTML Document. This code obtains an element object from an ID and displays its value in a message box:
第三个调用(“ ReferenceCall”)需要一些特殊处理,因为它引用了HTML文档中的一个元素。 此代码从ID获取元素对象,并在消息框中显示其值:
Public Sub ReferenceCall(ByVal cid As String)
Dim c As HtmlElement = WebBrowser1.Document.GetElementById(cid)
MsgBox(c.GetAttribute("value"))
End Sub
MSHTML object. This requires adding a reference to your project (to Microsoft.mshtml, which is located under the .Net tab of the Add Reference dialog)
MSHTML对象。 这需要添加对项目的引用(到Microsoft.mshtml,位于“添加引用”对话框的.Net选项卡下)
Public Sub ReferenceCall2(ByVal c As Object)
MsgBox(CType(c, mshtml.IHTMLInputElement).checked)
End Sub
结论 (Conclusion)
如您所见,在应用程序中处理DHTML事件相当容易。 显然,您可以为此做更多的事情,尤其是添加MSHTML参考。 在QuickEE中,我使用此技术来提供功能丰富的UI,并且在您自己的应用程序中执行类似操作很容易。 完整的项目代码
(Full Project Code
)
<System.Runtime.InteropServices.ComVisible(True)> _
Public Class Form1
Private HTMLstring As String = "<!DOCTYPE HTML PUBLIC ""-//W3C//DTD HTML 4.0 Transitional//EN"">" & _
"<html>" & _
"<body>" & _
"<button onclick=""window.external.SimpleCall()"">Call an application routine from script</button><br />" & _
"<button onclick=""window.external.ParameterCall(99, 'some text')"">Call an application routine from script, with parameters</button><br />" & _
"<br />" & _
"<input type=""text"" value=""Some text"" id=""txtTest""/><br />" & _
"<button onclick=""window.external.ReferenceCall('txtTest')"">Call an application routine from script, sending an elements ID</button><br />" & _
"<br/>" & _
"Reference the calling element<input type=""checkbox"" Checked=""Checked"" onclick=""window.external.ReferenceCall2(this)""/>" & _
"</body>" & _
"</html>"
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
WebBrowser1.ObjectForScripting = Me
WebBrowser1.ScriptErrorsSuppressed = False
WebBrowser1.DocumentText = HTMLstring
End Sub
Public Sub SimpleCall()
MsgBox("Simple Call")
End Sub
Public Sub ParameterCall(ByVal i As Integer, ByVal s As String)
MsgBox(String.Format("i = {0}, s = {1}", i, s))
End Sub
Public Sub ReferenceCall(ByVal cid As String)
Dim c As HtmlElement = WebBrowser1.Document.GetElementById(cid)
MsgBox(c.GetAttribute("value"))
End Sub
Public Sub ReferenceCall2(ByVal c As Object)
MsgBox(CType(c, mshtml.IHTMLInputElement).checked)
End Sub
End Class