vbs脚本读取安装软件列表
Microsoft has implemented the Windows Update as a three-stage process. This is the key to understand how Windows Update works. The process needs to find out which new updates are available for each Microsoft product, download and install them all.
Microsoft已将Windows Update分为三个阶段实施。 这是了解Windows Update如何工作的关键。 该过程需要找出每个Microsoft产品都有哪些新更新,然后下载并安装所有更新。
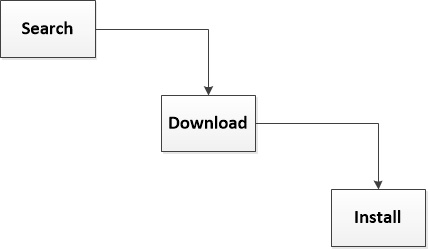
- by using the control panel 通过使用控制面板
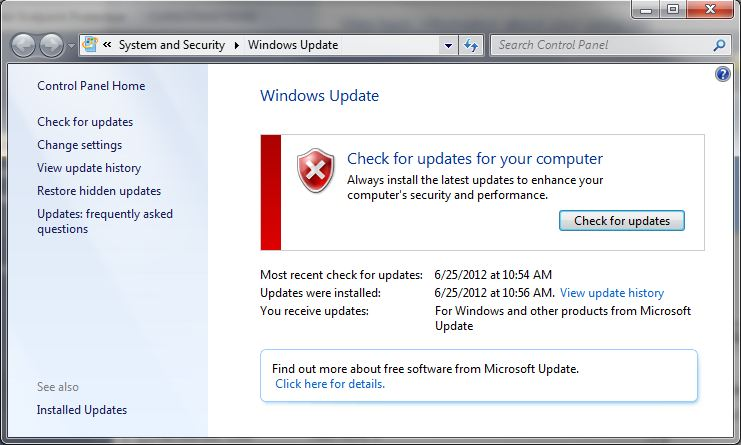
- or by using the wuauclt tool. 或使用wuauclt工具。
WuAuClt /DetectNow
So, there is a lack of native tools to start and complete the update process at will from a command prompt or from a scheduled task. To meet this need I created a script that executes the installation process from start to finish without user interaction. The script follows the three-stages process described previously, by using special windows functions published on MSDN for managing windows updates.
因此,缺少从命令提示符或计划任务随意启动和完成更新过程的本机工具。 为了满足此需求,我创建了一个脚本,该脚本从头到尾执行安装过程,而无需用户干预。 该脚本通过使用MSDN上发布的用于管理Windows更新的特殊Windows函数来遵循上述三个阶段的过程。
The script algorithm is the following:
脚本算法如下:
- Verify if there is a pending reboot, if so: reboot right now unless the /nr option was entered. 验证是否有挂起的重启,如果有的话:立即重启,除非输入了/ nr选项。
- Search for updates. 搜索更新。
- If there are new updates then download them all. 如果有新更新,请全部下载。
- Install all downloaded updates. 安装所有下载的更新。
- Reboot if it is needed, unless the /nr option was entered.. 如果需要,请重新引导,除非输入了/ nr选项。
On Error Resume Next
WScript.StdOut.Write "*****************************************************************" & vbCrLf
WScript.StdOut.Write "*** Forced install of all pending updates ***" & vbCrLf
WScript.StdOut.Write "*****************************************************************" & vbCrLf
WScript.StdOut.Write "** How to use this script **" & vbCrLf
WScript.StdOut.Write "*****************************************************************" & vbCrLf
WScript.StdOut.Write "** cscript DoUpdate.vbs [/nr] **" & vbCrLf
WScript.StdOut.Write "** **" & vbCrLf
WScript.StdOut.Write "** [/nr] Never reboot (default is to reboot if needed) **" & vbCrLf
WScript.StdOut.Write "*****************************************************************" & vbCrLf
' See if can auto-reboot.
DoReboot = True
if WScript.Arguments.Count <> 0 then
for i = 0 to WScript.Arguments.Count - 1
strInput1 = Lcase(Trim(WScript.Arguments(i)))
if (strInput1 = "/nr") then
DoReboot = False 'Do not reboot even if it is necessary.
end if
next
end if
'*******************************************************************
' Create needed objects.
'*******************************************************************
Set updateSession = CreateObject("Microsoft.Update.Session")
Set updateSearcher = updateSession.CreateUpdateSearcher()
Set updateDownloader = updateSession.CreateUpdateDownloader()
Set updateInstaller = updateSession.CreateUpdateInstaller()
Set ComputerStatus = CreateObject("Microsoft.Update.SystemInfo")
Set objShell = CreateObject("WScript.Shell")
' Step 1: Verify if there is a pending reboot, if so: reboot right now.
If ComputerStatus.RebootRequired then
WScript.StdOut.Write "This computer needs to reboot before start searching for updates." & vbCrLf
if DoReboot then
WScript.StdOut.Write "Rebooting in 5 seconds." & vbCrLf
strErrorCode = objShell.Run("shutdown.exe -r -f -t 05",0,True)
end if
WScript.Sleep 3000
WScript.Quit 1
End if
' Step 2: Search for updates.
WScript.StdOut.Write "Wait while searching updates." & vbCrLf
Set updateSearch = updateSearcher.Search("IsInstalled=0")
If updateSearch.ResultCode <> 2 Then
WScript.StdOut.Write "Searching has failed with error code: " & updateSearch.ResultCode & vbCrLf
WScript.Sleep 3000
WScript.Quit 1
End If
' Step 3: If there are new updates download them all.
If updateSearch.Updates.Count = 0 Then
WScript.StdOut.Write "No new updates. Finishing in 3 seconds." & vbCrLf
WScript.Sleep 3000
WScript.Quit 2
End If
WScript.StdOut.Write "Wait while downloading " & updateSearch.Updates.Count & " update(s)." & vbCrLf
updateDownloader.Updates = updateSearch.Updates
Set downloadResult = updateDownloader.Download()
If downloadResult.ResultCode <> 2 Then
WScript.StdOut.Write "The download has failed with error code: " & downloadResult.ResultCode & vbCrLf
WScript.Sleep 3000
WScript.Quit 1
End If
WScript.StdOut.Write "Download completed." & vbCrLf
' Step 4: Install all downloaded updates.
WScript.StdOut.Write "Installing updates ..." & vbCrLf
updateInstaller.Updates = updateSearch.Updates
Set installationResult = updateInstaller.Install()
If installationResult.ResultCode <> 2 Then
WScript.StdOut.Write "The installation has failed with error code: " & installationResult.ResultCode & vbCrLf
WScript.Sleep 3000
WScript.Quit 1
End If
' Step 5: Reboot if its needed.
If ComputerStatus.RebootRequired then
WScript.StdOut.Write "This computer needs to reboot to complete the installation." & vbCrLf
if DoReboot then
WScript.StdOut.Write "Rebooting in 5 seconds." & vbCrLf
strErrorCode = objShell.Run("shutdown.exe -r -f -t 05",0,True)
end if
WScript.Sleep 3000
WScript.Quit 1
Else
WScript.StdOut.Write "Script completed." & vbCrLf
WScript.Sleep 3000
WScript.Quit 2
End if
This is a Visual Basic Script that you can use freely. The script has an optional argument to control the reboot behavior of the computer: with /nr the script will not reboot even if it is needed by the update installation to complete. If you run the script without arguments it will always reboot whenever is needed. If the computer needs to reboot before start to search for updates, it will reboot at the moment you run the script.
这是您可以自由使用的Visual Basic脚本。 该脚本具有一个可选参数来控制计算机的重新启动行为:使用/ nr ,即使更新安装需要完成该脚本,该脚本也不会重新启动。 如果运行不带参数的脚本,它将始终在需要时重新启动。 如果计算机需要重新启动才能开始搜索更新,则它将在您运行脚本时重新启动。
To run the script you must use the CSCRIPT.EXE native application. For example:
若要运行脚本,您必须使用CSCRIPT.EXE本机应用程序。 例如:
CScript //Nologo DoUpdate.vbs /nr
CScript //Nologo \\FQDN.Domain.Name\NetLogon\DoUpdate.vbs /nr
Thanks for reading and good luck to everyone.
感谢您的阅读和祝大家好运。
vbs脚本读取安装软件列表