mysql 显示数据库大小
In this tutorial, I will show you how to set up a simple database that holds images and how to re-size and display those images on your website. Here is what the result of this tutorial will look like:
在本教程中,我将向您展示如何建立一个保存图像的简单数据库,以及如何调整这些图像的大小并在您的网站上显示这些图像。 这是本教程的结果如下所示:
http://www.patsmitty.com/ExpertsExchange/BlobArticle.php http://www.patsmitty.com/ExpertsExchange/BlobArticle.phpThe example I am going to use stores screen-shots (.jpeg) of websites. For instance the screen-shot of www.facebook.com looks like this:
我将使用的示例存储网站的屏幕截图(.jpeg)。 例如, www.facebook.com的屏幕截图如下所示:
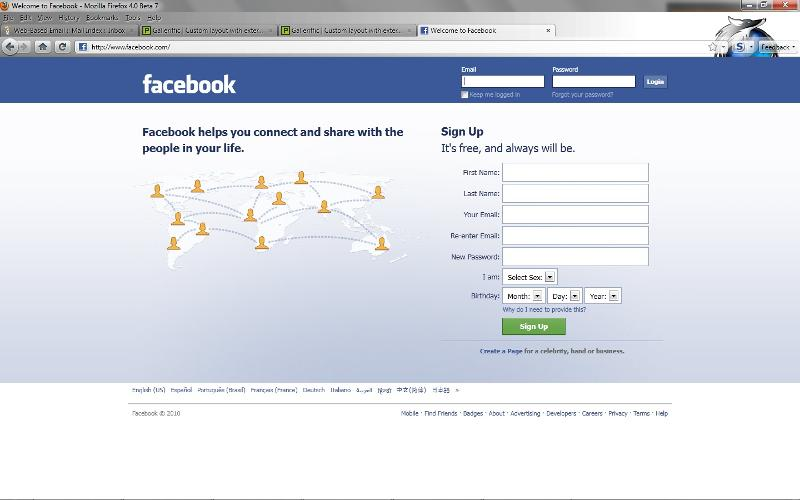
So, here is the to-do list:
因此,这是待办事项清单:
1.创建MySQL数据库 (1. Create the MySQL Database)
使用名为“ tblBlob”的表创建一个MySQL数据库,该表具有以下4个字段:blob_id
blob_id
blob_name
blob_name
blob_url
blob_url
blob_binary
blob_binary
Here is the MySQL syntax for creating this table:
这是用于创建此表MySQL语法:
CREATE TABLE `tblBlob` (
`blob_id` INTEGER UNSIGNED NOT NULL AUTO_INCREMENT,
`blob_name` VARCHAR(85) NOT NULL,
`blob_url` VARCHAR(185) NOT NULL,
`blob_binary` LONGBLOB NOT NULL,
PRIMARY KEY (`blob_id`)
)
ENGINE = InnoDB;
2.创建一个PHP脚本来检索和调整图像大小 (2. Create a PHP script for retrieving and re-sizing the image)
将此脚本称为“ doImage.php”。 将会发生的是,从显示图像和链接的主页(我们将在下一步创建),将图像标签内的“ src”设置为以下内容:echo('<img src="doImage.php?blob_id=1&resize=225,225" />');
Notice the two request-variables inside the url: blob_id=1 and resize=225,225. The first one, blob_id=1, retrieves the blob in the database whose blob_id is 1. So if you wanted the 15th blob in the database, you would change that 1 to a 15. (We will dynamically set this variable -- I only went into this for explaining purposes) The second variable, resize=225,225, will size the image to 225 x 225 (h x w). It won't change the blob in the database, it is only for viewing purposes. If you want to just display the original image's size, you can leave this out completely which would give you the following url:
请注意url中的两个请求变量:blob_id = 1和resize = 225,225。 第一个blob_id = 1,用于检索数据库中blob_id为1的blob。因此,如果您想要数据库中的第15个blob,可以将其更改为15。(我们将动态设置此变量-我仅第二个变量resize = 225,225会将图像的尺寸调整为225 x 225(hxw)。 它不会更改数据库中的Blob,仅用于查看目的。 如果您只想显示原始图像的大小,则可以完全省略此大小,这将为您提供以下网址:
echo('<img src="doImage.php?blob_id=1" />');
The contents of doImage.php need to look like this:
doImage.php的内容应如下所示:
<?php
$blob_id = $_REQUEST['blob_id']; //gets the request variable from the url that contains the id of the blob that we want to retrieve from the database
mysql_connect('database host-name', 'database user-name', 'password') OR DIE('Unable to connect to database! Please try again later.');
mysql_select_db('database-name');
$sql = "SELECT blob_name, blob_binary FROM tblBlob WHERE blob_id = '$blob_id'";
$result = mysql_query($sql) or exit("QUERY FAILED!");
list($blob_name, $blob_url, $blob_binary) = mysql_fetch_array($result);
header("Content-type: image/jpeg");
header("Content-Disposition: attachment; filename= $blob_name");
if ($_REQUEST['resize'] != "" && $_REQUEST['resize'] != null) { //resizes the images if the url contains
$dimensions = explode(",", $_REQUEST['resize']);
echo resize($blob_binary, $dimensions[0], $dimensions[1]);
} else {
echo $blob_binary;
}
function resize($blob_binary, $desired_width, $desired_height) { // simple function for resizing images to specified dimensions from the request variable in the url
$im = imagecreatefromstring($blob_binary);
$new = imagecreatetruecolor($desired_width, $desired_height) or exit("bad url");
$x = imagesx($im);
$y = imagesy($im);
imagecopyresampled($new, $im, 0, 0, 0, 0, $desired_width, $desired_height, $x, $y) or exit("bad url");
imagedestroy($im);
imagejpeg($new, null, 85) or exit("bad url");
echo $new;
}
?>
This script takes the first variable (blob_id) and retrieves that blob from the database. If there is a resize variable, the script will re-size the retrieved image.
该脚本采用第一个变量(blob_id),并从数据库中检索该blob。 如果有调整大小的变量,脚本将调整检索到的图像的大小。
This is all we need to do with this script. It is as easy as that! Lastly we need to create the main page that will display all the images with their links.
这就是我们需要使用此脚本进行的所有操作。 就是这么简单! 最后,我们需要创建显示所有图像及其链接的主页。
3.创建主页 (3. Create Main Page)
创建一个php网页,并根据需要命名。Inside the body of your php page, insert the following code that grabs the ids, names, and urls from your database, then sets the image sources to the respective url I discussed in the last step:
在您的php页面的主体内,插入以下代码,以从数据库中获取ID,名称和url,然后将图像源设置为我在上一步中讨论的相应url:
<?php
$blob_id = $_REQUEST['blob_id']; //gets the request variable from the url that contains the id of the blob that we want to retrieve from the databse
mysql_connect('db host', 'db user-name', 'password') OR DIE('Unable to connect to database! Please try again later.');
mysql_select_db('db-name');
$sql = "SELECT blob_id, blob_name, blob_url FROM tblBlob";
$result = mysql_query($sql) or exit("QUERY FAILED!");
if ($result):
while ($row = mysql_fetch_array($result)):
echo('<h2><a href="' . $row['blob_url'] . '">' . $row['blob_name'] . '</a></h2>');
echo('<br />');
echo('<img alt="' . $row['blob_name'] . '" src="doImage.php?blob_id=' . $row['blob_id'] . '&resize=280,215" />');
echo('<br /><hr />');
endwhile;
endif;
?>
4.加载样本数据 (4. Load Sample Data)
将一些样本数据放入数据库中。 我拍摄了6个不同网站的屏幕截图,并将它们放入我的数据库中。 这是我的数据库内容的屏幕截图: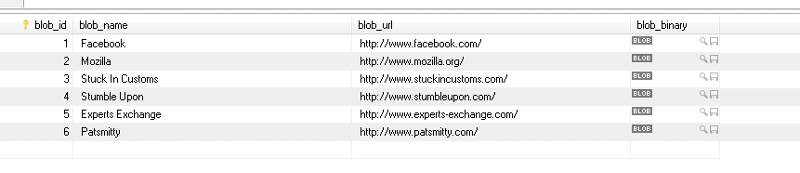
5.修复连接字符串并将两个文件放在同一目录中 (5. Fix Connection Strings and Place Both Files In Same Directory)
进入两个文件并修复MySQL连接字符串以连接到数据库。Now place both files in the same directory and navigate to the main page that you created. Here is what my example looks like:
现在,将两个文件放在同一目录中,并导航到创建的主页。 这是我的示例:
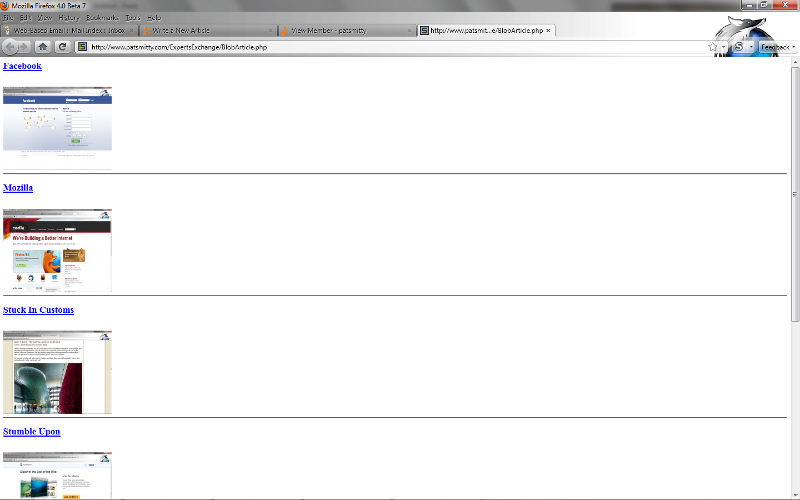
You now have a working example of how to display and resize images from your MySQL database onto your website!
现在,您将获得一个有效的示例,该示例如何显示MySQL数据库中的图像并调整其大小到您的网站上!
mysql 显示数据库大小